 |
Characteristics and Properties of Web Controls |
|
Fundamental Boolean Properties
Introduction
A Boolean property is one that has a True or a False value. In a form, the value is assigned as a case-insensitive string to an attribute of the same name. In the code, you can refer to the property and compare its value to True or False.
A Read-Only Text Box
A text box is read-only if the visitor can only see or select it but he cannot change its value. To support this characteristic, the TextBox is equipped with a property named ReadOnly.
Checking a Check Box
Probably the most fundamental characteristic of a check box is its ability to be checked or not. This is controlled by a member named Checked. To check the control, add an attribute named Checked to the <asp:CheckBox> control and set its value to "True". Here is an example:
<form id="frmRestaurant" runat="server">
<asp:CheckBox id="chkSpicy"
Text="Food is spicy?"
Checked="True"
runat="server"></asp:CheckBox>
</form>
On the other hand, to find out whether the check box is
checked or not, get the value of that attribute.
Automatically Posting a Check Box
Obviously, to use a check box, the visitor must click it. When this happens, the control sends a message to the server, in a Click event. To support this, the CheckBox class is equipped with a member named AutoPostBack, which accompanies an attribute of the same name. Therefore, to specify that a check box must automatically send a click notification to the server, set this attribute as "True".
Checking a Radio Button
To use a radio button, a webpage visitor must click it. To support this action, the RadioButtonList class has a Boolean member named AutoPostBack. If you set it to True, when the control is clicked, it sends a notification to the server. The associated event
is named SelectedIndexChanged. You can use that event ti identify the radio button that was clicked. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub rblPayrollPeriodSelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
lblSelection.Text = "Index of Radio Button Selected: " & rblPayrollPeriod.SelectedIndex
End Sub
</script>
<title>Payroll Evaluation</title>
</head>
<body>
<h3>Payroll Evaluation</h3>
<form id="frmPayroll" runat="server">
<p><b>Payroll Frequency</b></p>
<table>
<tr>
<td style="width: 150px">
<asp:RadioButtonList id="rblPayrollPeriod" AutoPostBack="true"
OnSelectedIndexChanged="rblPayrollPeriodSelectedIndexChanged" runat="server">
<asp:ListItem>Weekly</asp:ListItem>
<asp:ListItem>Biweekly</asp:ListItem>
<asp:ListItem>Semimonthly</asp:ListItem>
<asp:ListItem>Monthly</asp:ListItem>
</asp:RadioButtonList></td>
<td>
<asp:Label id="lblSelection" runat="server"></asp:Label>
</td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of using the webpage:
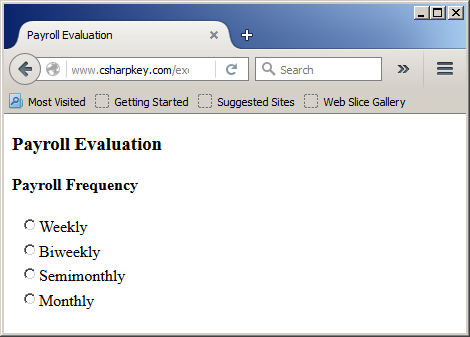
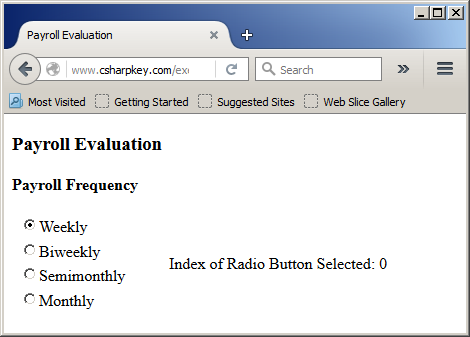
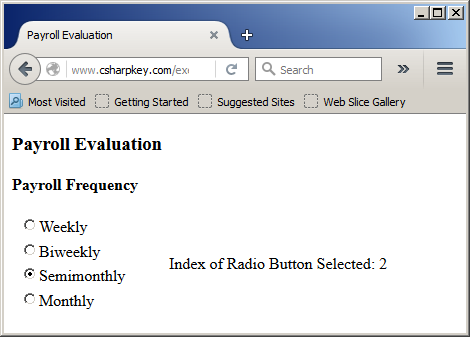
This means that, to find out
what button was clicked, compare the values of the RadioButtonList.SelectedIndex
member and take the appropriate action. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Sub BtnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim incomeTax As Double
Dim employeeName As String
Dim hourlySalary, timeWorked
Dim frequency As Integer
Dim grossSalary, netPay
pnlPreparation.Visible = False
pnlPresentation.Visible = True
incomeTax = 0.00
employeeName = TxtEmployeeName.Text
hourlySalary = CDbl(TxtHourlySalary.Text)
timeWorked = CDbl(TxtTimeWorked.Text)
frequency = RblPayrollFrequency.SelectedIndex
grossSalary = hourlySalary * timeWorked
If frequency = 0 Then
incomeTax = 99.1 + (grossSalary * 0.25)
ElseIf frequency = 1 Then
incomeTax = 35.5 + (grossSalary * 0.15)
ElseIf frequency = 2 Then
incomeTax = 38.4 + (grossSalary * 0.15)
Else ' If frequency == 3)
incomeTax = 76.8 + (grossSalary * 0.15)
End If
netPay = grossSalary - incomeTax
lblEmployeeName.Text = employeeName
lblHourlySalary.Text = hourlySalary
lblTimeWorked.Text = timeWorked
lblGrossSalary.Text = grossSalary
If frequency = 0 Then
lblPayrollFrequency.Text = "Weekly"
ElseIf frequency = 1 Then
lblPayrollFrequency.Text = "Biweekly"
ElseIf frequency = 2 Then
lblPayrollFrequency.Text = "Semimonthly"
Else ' If frequency == 3 then
lblPayrollFrequency.Text = "Monthly"
End If
lblIncomeTax.Text = FormatNumber(incomeTax)
lblNetPay.Text = FormatNumber(netPay)
End Sub
</script>
<style type="text/css">
#tblPreparation { width: 450px; }
#tblPresentation { width: 300px; }
.main-title
{
font-size: 1.28em;
font-weight: bold;
text-align: center;
font-family: Georgia, Garamond, 'Times New Roman'Payroll Preparation
}
</style>
</head>
<body>
<div id="whole">
<form id="frmPayroll" runat="server">
<asp:Label id="lblMainTitle" CssClass="main-title"
runat="server" Text="Payroll Preparation"></asp:Label>
<asp:Panel id="pnlPreparation" Visible="true" runat="server">
<table id="tblPreparation">
<tr>
<td><p><b>Employee/Time Information</b></p>
<table>
<tr>
<td style="width: 160px;">Employee Name:</td>
<td>
<asp:TextBox id="TxtEmployeeName"
runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>Hourly Salary:</td>
<td>
<asp:TextBox id="TxtHourlySalary" runat="server"
style="width: 50px"></asp:TextBox>
</td>
</tr>
<tr>
<td>Time Worked:</td>
<td>
<asp:TextBox id="TxtTimeWorked" runat="server"
style="width: 50px"></asp:TextBox>
</td>
</tr>
<tr>
<td> </td>
<td> </td>
</tr>
</table>
</td>
<td style="width: 140px;"><p><b>Payroll Frequency</b></p>
<asp:RadioButtonList id="RblPayrollFrequency" runat="server">
<asp:ListItem>Weekly</asp:ListItem>
<asp:ListItem>Biweekly</asp:ListItem>
<asp:ListItem>Semimonthly</asp:ListItem>
<asp:ListItem>Monthly</asp:ListItem>
</asp:RadioButtonList>
</td>
</tr>
<tr>
<td colspan="2">
<p style="text-align: center">
<asp:Button id="BtnCalculate" runat="server" style="height: 32px;"
Text="Calculate" style="width: 182px"
OnClick="BtnCalculateClick" /></p>
</td>
</tr>
</table>
</asp:Panel>
<asp:Panel id="pnlPresentation" Visible="false" runat="server">
<table id="tblPresentation">
<tr>
<td>Employee Name:</td>
<td><asp:Label id="lblEmployeeName"
runat="server"></asp:Label></td>
</tr>
<tr>
<td>Hourly Salary:</td>
<td><asp:Label id="lblHourlySalary" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Time Worked:</td>
<td><asp:Label id="lblTimeWorked" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Raw Salary:</td>
<td><asp:Label id="lblGrossSalary" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Payroll Period:</td>
<td><asp:Label id="lblPayrollFrequency"
runat="server"></asp:Label></td>
</tr>
<tr>
<td>Income Tax:</td>
<td><asp:Label id="lblIncomeTax" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>Net Pay:</td>
<td><asp:Label id="lblNetPay" runat="server"></asp:Label></td>
</tr>
</table>
</asp:Panel>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
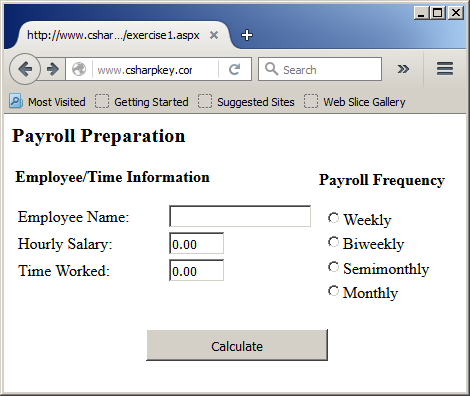
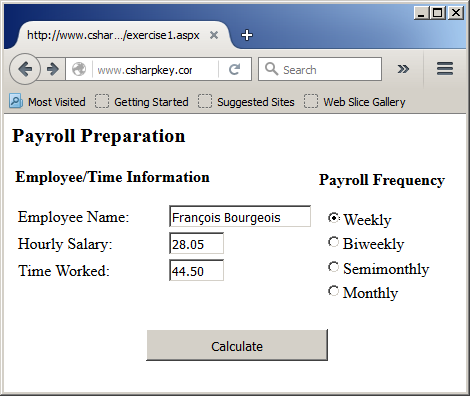
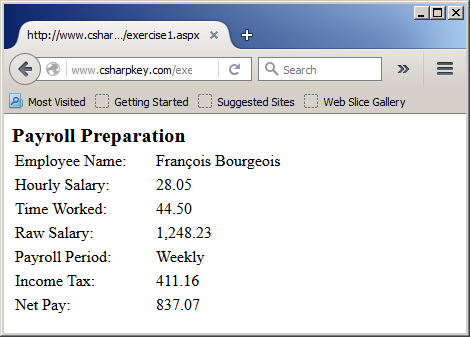
Using Web Controls in Conditional Statements
A Text Box Losing Focus
When a user of a webform clicks in a text box, or when the caret lands in a text box, the control is said to have focus. To use another control or do something else on the webpage, the visitor can press Tab or click somewhere else. When this happens, the text box is said to have lost focus, and this action fires an event.
When a text box loses focus, to let you send a notification to the server and possibly take action, the TextBox class is equipped with a Boolean member named AutoPostBack. To take advantage of the lost focus event of a text box, set this member to True.
A Drop Down List/Combo Box
To use a combo box, a webpage visitor can select one of
the items in the list. There are various ways you can find out what item was
selected. You can access the Text value of the SelectedItem member to find out what item was select. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim hourlySalary As Double = txtHourlySalary.Text
Dim weeklyTime As Double = txtWeeklyTime.Text
Dim weeklySalary As Double = hourlySalary * weeklyTime
Dim incomeTax As Double = 99.10 + (weeklySalary * 0.25)
If ddlMaritalStatus.SelectedItem.Text = "Married" Then
incomeTax = 35.50 + (weeklySalary * 0.15)
End If
Dim netPay As Double = weeklySalary - incomeTax
txtIncomeTax.Text = incomeTax
txtNetPay.Text = netPay
End Sub
</script>
<title>Payroll Evaluation</title>
</head>
<body>
<h3 style="text-align: center">Payroll Evaluation</h3>
<form id="frmPayroll" runat="server">
<table align="center" id="tblPayroll">
<tr>
<td>
<asp:Label id="lblEmployeeeName" runat="server"
Text="Employeee Name:"></asp:Label>
</td>
<td>
<asp:TextBox id="txtEmployeeeName" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label id="lblMaritalStatus" runat="server"
Text="Marital Status:"></asp:Label>
</td>
<td>
<asp:DropDownList id="ddlMaritalStatus" Width="120px" runat="server">
<asp:ListItem>Single</asp:ListItem>
<asp:ListItem>Married</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td>
<asp:Label id="lblHourlySalary" runat="server"
Text="Hourly Salary:"></asp:Label>
</td>
<td>
<asp:TextBox id="txtHourlySalary" runat="server"
Width="75px"></asp:TextBox></td>
</tr>
<tr>
<td>
<asp:Label id="lblWeeklyTime" runat="server" Text="Weekly Time:"></asp:Label>
</td>
<td>
<asp:TextBox id="txtWeeklyTime" runat="server" Width="75px"></asp:TextBox>
<asp:Button id="btnCalculate" runat="server"
OnClick="btnCalculateClick" Text="Calculate" Width="122px" />
</td>
</tr>
<tr>
<td>
<asp:Label id="lblIncomeTax" runat="server" Text="Income Tax:"></asp:Label>
</td>
<td>
<asp:TextBox id="txtIncomeTax" runat="server"
Width="75px"></asp:TextBox></td>
</tr>
<tr>
<td>
<asp:Label id="lblNetPay" runat="server" Text="Net Pay:"></asp:Label>
</td>
<td>
<asp:TextBox id="txtNetPay" runat="server"
style="width: 75px"></asp:TextBox></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of using the webpage:
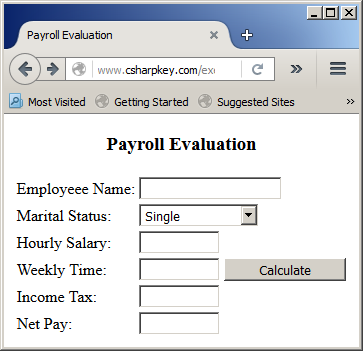
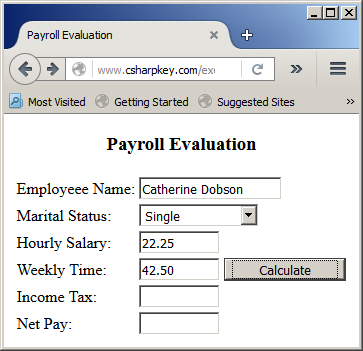
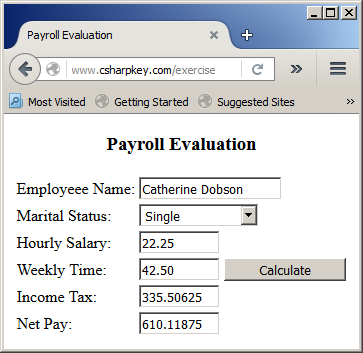
To support the item to be selected, the class is equipped
with a Boolean member named Selected. To specify the item to be selected
as the default, set this member to True.
Finding a Control in a Web Page
To let you find a control in a webpage, the Page class is equipped with a method named FindControl. It takes the identification of the control as a string argument. Once you have the control, you can use it. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub btnEvaluateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim discountRate As Double
Dim txtItemName As TextBox
Dim txtUnitPrice As TextBox
Dim discountAmount As Double
Dim txtDiscountRate As TextBox
Dim unitPrice As Double, salePrice As Double
txtItemName = Page.FindControl("txtOriginalItemName")
txtUnitPrice = Page.FindControl("txtOriginalUnitPrice")
txtDiscountRate = Page.FindControl("txtOriginalDiscountRate")
unitPrice = If(txtUnitPrice.Text = "", 0.00, txtUnitPrice.Text)
discountRate = If(txtDiscountRate.Text = "", 0.00, txtDiscountRate.Text)
If discountRate >= 1.00 Then
discountAmount = unitPrice * discountRate / 100.00
txtResultDiscountRate.Text = discountRate & "%"
Else
discountAmount = unitPrice * discountRate
txtResultDiscountRate.Text = (discountRate * 100.00) & "%"
End If
salePrice = unitPrice - discountAmount
txtResultItemName.Text = txtItemName.Text
txtResultUnitPrice.Text = unitPrice
txtDiscountAmount.Text = discountAmount
txtSalePrice.Text = salePrice
End Sub
</script>
<title>Hospice Store - Hospital Beds Sale</title>
</head>
<body>
<form id="frmStore" runat="server">
<div align="center">
<h3>Hospice Store - Hospital Beds Inventory</h3>
<table>
<tr>
<td>Item Name:</td>
<td>
<asp:TextBox id="txtOriginalItemName" Width="200px"
runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td>
<asp:TextBox id="txtOriginalUnitPrice"
runat="server" style="Width: 65px" /></td>
</tr>
<tr>
<td>Discount Rate:</td>
<td>
<asp:TextBox id="txtOriginalDiscountRate" style="Width: 65px" runat="server" />%
<asp:Button id="btnEvaluate" text = "Calculate" OnClick = "btnEvaluateClick"
runat="server"></asp:Button></td>
</tr>
</table>
<h3>Hospice Store - Hospital Beds Sale</h3>
<table>
<tr>
<td>Item Name:</td>
<td style="text-align: left">
<asp:TextBox id="txtResultItemName" Width="200px"
ReadOnly="True" runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td style="text-align: left">
<asp:TextBox id="txtResultUnitPrice"
ReadOnly="True" runat="server" Width="65px" /></td>
</tr>
<tr>
<td>Discount Rate:</td>
<td style="text-align: left">
<asp:TextBox id="txtResultDiscountRate"
ReadOnly="True" Width="65px" runat="server" /></td>
</tr>
<tr>
<td>Discount Amount:</td>
<td style="text-align: left">
<asp:TextBox id="txtDiscountAmount" ReadOnly="True"
Width="65px" runat="server" /></td>
</tr>
<tr>
<td>Sale Price:</td>
<td style="text-align: left">
<asp:TextBox id="txtSalePrice" Width="65px"
ReadOnly="True" runat="server" /></td>
</tr>
</table>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
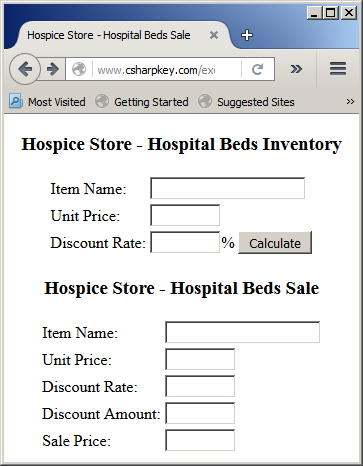
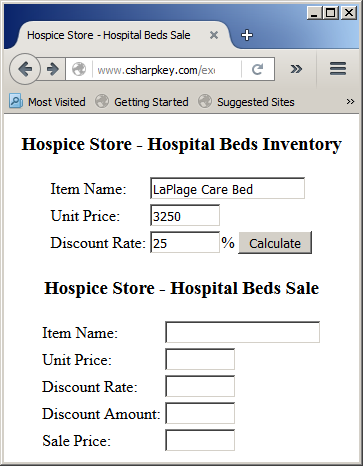
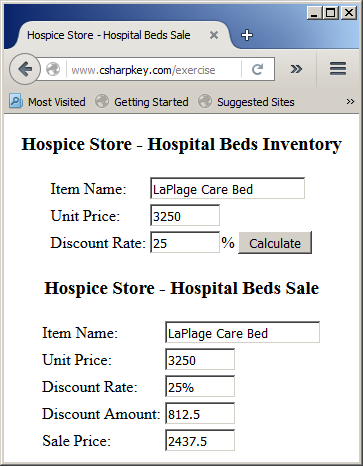
In the same way, if a webpage is displaying as a result of an action from another page, you can use the PreviousPage property to get a reference to a (the) control(s) from the previous page, and then use the control as if it were on the current page. Here is an example:
hospital1.aspx
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Hospice Store - Hospital Beds Sale</title>
</head>
<body>
<form id="frmStore" runat="server">
<div align="center">
<h3>Hospice Store - Hospital Beds Inventory</h3>
<table>
<tr>
<td>Item Name:</td>
<td>
<asp:TextBox id="txtOriginalItemName" Width="200px"
runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td>
<asp:TextBox id="txtOriginalUnitPrice"
runat="server" style="Width: 65px" /></td>
</tr>
<tr>
<td>Discount Rate:</td>
<td>
<asp:TextBox id="txtOriginalDiscountRate" style="Width: 65px" runat="server" />%
<asp:Button id="btnEvaluate" text = "Evaluate" PostBackUrl="hospital2.aspx"
runat="server"></asp:Button></td>
</tr>
</table>
</div>
</form>
</body>
</html>
hospital2.aspx
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim discountRate As Double
Dim txtItemName As TextBox
Dim txtUnitPrice As TextBox
Dim discountAmount As Double
Dim txtDiscountRate As TextBox
Dim unitPrice As Double, salePrice As Double
If Not IsNothing(Page.PreviousPage) Then
txtItemName = Page.PreviousPage.FindControl("txtOriginalItemName")
txtUnitPrice = Page.PreviousPage.FindControl("txtOriginalUnitPrice")
txtDiscountRate = Page.PreviousPage.FindControl("txtOriginalDiscountRate")
unitPrice = If(txtUnitPrice.Text = "", 0.00, txtUnitPrice.Text)
discountRate = If(txtDiscountRate.Text = "", 0.00, txtDiscountRate.Text)
If discountRate >= 1.0 Then
discountAmount = unitPrice * discountRate / 100.0
txtResultDiscountRate.Text = discountRate & "%"
Else
discountAmount = unitPrice * discountRate
txtResultDiscountRate.Text = (discountRate * 100.0) & "%"
End If
salePrice = unitPrice + discountAmount
txtResultItemName.Text = txtItemName.Text
txtResultUnitPrice.Text = unitPrice
txtDiscountAmount.Text = discountAmount
txtSalePrice.Text = salePrice
End If
End Sub
</script>
<title>Hospice Store - Hospital Beds Sale</title>
</head>
<body>
<form id="frmStore" runat="server">
<div align="center">
<h3>Hospice Store - Hospital Beds Sale</h3>
<table>
<tr>
<td>Item Name:</td>
<td style="text-align: left">
<asp:TextBox id="txtResultItemName" Width="200px"
ReadOnly="True" runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td style="text-align: left">
<asp:TextBox id="txtResultUnitPrice" ReadOnly="True"
runat="server" style="Width: 65px" /></td>
</tr>
<tr>
<td>Discount Rate:</td>
<td style="text-align: left">
<asp:TextBox id="txtResultDiscountRate" ReadOnly="True"
style="Width: 65px" runat="server" /></td>
</tr>
<tr>
<td>Discount Amount:</td>
<td style="text-align: left">
<asp:TextBox id="txtDiscountAmount" ReadOnly="True"
style="Width: 65px" runat="server" /></td>
</tr>
<tr>
<td>Sale Price:</td>
<td style="text-align: left">
<asp:TextBox id="txtSalePrice" ReadOnly="True"
style="Width: 65px" runat="server" /></td>
</tr>
</table>
</div>
</form>
</body>
</html>
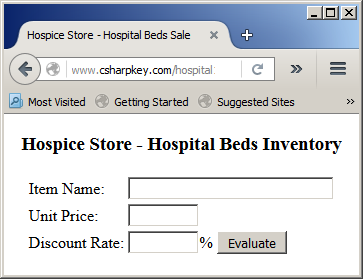
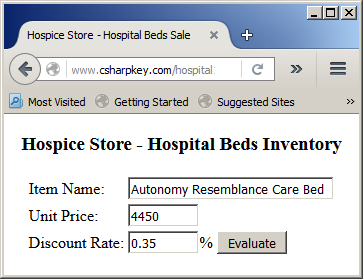
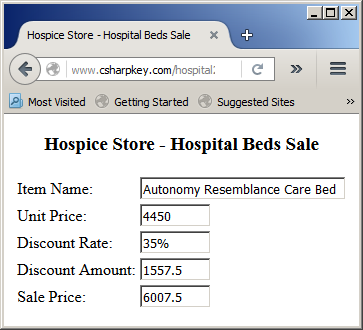
Common Characteristics and Properties of Web Controls
Introduction
CSS provides tremendous support for styles of webcontrols. Some of the characteristics include the size of a control, the font, the colors, etc. The .NET Framework also provides its own support of those characteristics as properties on the classes for those controls. There are other features that are not available in CSS, or are not handled the same way in CSS.
The Width of a Control
Besides the width style available in CSS, the classes of ASP.NET visual controls are equipped with a property named Width that can be used to control the length of a control. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim weeklySalary As Double
Dim hourlySalary As Double
Dim timeWorked As Double
Dim overtime As Double
Dim overtimePay As Double
weeklySalary = 0.0
hourlySalary = CDbl(txtHourlySalary.Text)
timeWorked = CDbl(txtTimeWorked.Text)
If timeWorked <= 40.0 Then
weeklySalary = hourlySalary * timeWorked
Else
overtime = timeWorked - 40.0
overtimePay = hourlySalary * 1.50 * overtime
weeklySalary = (hourlySalary * 40.0) + overtimePay
End If
txtWeeklySalary.Text = FormatNumber(weeklySalary)
End Sub
</script>
<title>Payroll Preparation</title>
</head>
<body>
<div align="center">
<form id="frmPayroll" runat="server">
<h3>Payroll Preparation</h3>
<table border=0>
<tr>
<td style="text-align: left">
<asp:Label ID="lblHourlySalary" runat="server"
Text="Hourly Salary:"></asp:Label>
</td>
<td style="text-align: left">
<asp:TextBox id="txtHourlySalary" Width="65px"
runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label ID="lblTimeWorked" runat="server"
Text="Time Worked:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtTimeWorked" runat="server" Width="65px" />
<asp:Button id="btnCalculate"
text = "Calculate"
OnClick = "btnCalculateClick"
runat="server"></asp:Button></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label ID="lblWeeklySalary" runat="server"
Text="Weekly Salary:"></asp:Label></td>
<td style="text-align: left">
<asp:TextBox id="txtWeeklySalary"
Width="65px" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html
|