Implementing Authentication |
|
There is always more than one way to solve a problem
in logic and in math. When it comes to an application also, you have
many options:
- You can create the first form of your application as the log in
dialog box. In this case, if the employee successfully logs, then the
actual application would display
- You can add the code to call a log in dialog box to the first form.
If the employee successfully logs in, then the rest of the application
would show
In all cases, you must decide when and how the employee
would log in and what to do in case of successful or unsuccessful log in.
Practical
Learning: Implementing Authentication
|
|
- To create a file, on the main menu, click Project -> Add
New Item...
- In the middle list, click Code File
- Set the Name to Authenticator
- Click Add
- In the empty document, type the following:
using System;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
using System.ComponentModel;
public class Authenticator : Form
{
private Label lblUsername;
public TextBox txtUsername;
private Label lblPassword;
public TextBox txtPassword;
private Button btnOK;
private Button btnCancel;
public Authenticator()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblUsername = new Label();
txtUsername = new TextBox();
txtPassword = new TextBox();
lblPassword = new Label();
btnOK = new Button();
btnCancel = new Button();
this.SuspendLayout();
lblUsername.AutoSize = true;
lblUsername.Location = new Point(12, 19);
lblUsername.Size = new Size(58, 13);
lblUsername.TabIndex = 0;
lblUsername.Text = "Username:";
txtUsername.Location = new Point(87, 16);
txtUsername.Size = new Size(100, 20);
txtUsername.TabIndex = 1;
lblPassword.AutoSize = true;
lblPassword.Location = new Point(12, 45);
lblPassword.Size = new Size(56, 13);
lblPassword.TabIndex = 2;
lblPassword.Text = "Password:";
txtPassword.Location = new Point(87, 42);
txtPassword.PasswordChar = '*';
txtPassword.Size = new Size(100, 20);
txtPassword.TabIndex = 3;
btnOK.DialogResult = System.Windows.Forms.DialogResult.OK;
btnOK.Location = new Point(31, 78);
btnOK.Size = new Size(75, 23);
btnOK.TabIndex = 4;
btnOK.Text = "OK";
btnOK.UseVisualStyleBackColor = true;
btnCancel.DialogResult = System.Windows.Forms.DialogResult.Cancel;
btnCancel.Location = new Point(112, 78);
btnCancel.Size = new Size(75, 23);
btnCancel.TabIndex = 5;
btnCancel.Text = "Cancel";
btnCancel.UseVisualStyleBackColor = true;
Controls.Add(lblUsername);
Controls.Add(txtUsername);
Controls.Add(lblPassword);
Controls.Add(txtPassword);
Controls.Add(btnOK);
Controls.Add(btnCancel);
AcceptButton = btnOK;
CancelButton = btnCancel;
ClientSize = new Size(206, 115);
FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
MaximizeBox = false;
MinimizeBox = false;
ShowInTaskbar = false;
StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
Text = "Fun Department Store - Log In";
ResumeLayout(false);
}
}
- In the Solution Explorer, double-click Authenticator.cs
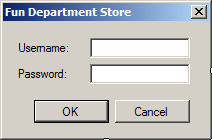
- Access the Switchboard.cs file
- Create a method named LogInToTheApplication and change the SwitchboardLoaded event as follows:
private void LogInToTheApplication()
{
bool usernamePasswordMatch = false;
Authenticator dlgLogIn = new Authenticator();
string strPasswordFromDialogBox = "", strPasswordFromDatabase = "";
string strUsernameFromDialogBox = "", strUsernameFromDatabase = "";
// Display the Autheticator dialog box.
// If the user clicks Cancel, simply close the application
if (dlgLogIn.ShowDialog() == System.Windows.Forms.DialogResult.Cancel)
Close();
else // If the user clicks OK
{
// If the employee doesn't enter a username, display a message box...
if (dlgLogIn.txtUsername.Text == "")
{
MessageBox.Show("You must enter a user name.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// ... and close the application
Close();
}
// If the employee doesn't enter a password, display a message box...
if (dlgLogIn.txtPassword.Text == "")
{
MessageBox.Show("You must type a password.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// ... and close the application
Close();
}
// Get the user name of the dialog box
strUsernameFromDialogBox = dlgLogIn.txtUsername.Text;
// Get the password of the dialog box
strPasswordFromDialogBox = dlgLogIn.txtPassword.Text;
// To connect to the database, after the following Data Source= expression,
// type \\, followed by the name of the server (ours is named Expression),
// followed by \\, followed by the name of the folder where the database
// is installed (ours is named Fun Department Store) (of course, you must
// have shared that folder), followed by \\, and followed by the name of
// the database, in this case FunDS
using (OleDbConnection conFunDS =
new OleDbConnection(@"Provider=Microsoft.ACE.OLEDB.12.0;" +
@"Data Source=\\Expression\\Fun Department Store\\FunDS.accdb"))
{
// Get the records of all employees
OleDbCommand cmdFunDS = new OleDbCommand("SELECT * FROM Employees;", conFunDS);
// Create a data adapater to retrieve the employees
OleDbDataAdapter odaEmployees = new OleDbDataAdapter(cmdFunDS);
// Create a data set of employees
DataSet dsEmployees = new DataSet("EmployeesSet");
// Fill the data set with the Employees records
odaEmployees.Fill(dsEmployees);
// Open the connection
conFunDS.Open();
// Navigate to each record
for (int i = 0; i < dsEmployees.Tables[0].Rows.Count; i++)
{
// Get a reference to the current record
DataRow rcdStoreItem = dsEmployees.Tables[0].Rows[i];
// When you are on a record, get the username of the employee
strUsernameFromDatabase = rcdStoreItem["Username"].ToString();
// and the password
strPasswordFromDatabase = rcdStoreItem["UserPassword"].ToString();
// Compare the current username to the username of the dialog box
// and the current password to the password of the dialog box.
// If they match, ...
if (strUsernameFromDatabase.Equals(strUsernameFromDialogBox) &&
strPasswordFromDatabase.Equals(strPasswordFromDialogBox))
{
usernamePasswordMatch = true;
// ... display the switchboard
break;
}
// If there is no match, continue to the next record,
// up to the end of the table
}
// If there was a match for username/password, display the Switchboard
// If there was no match for username/password,
// Let the employee know ...
if (usernamePasswordMatch == false)
{
MessageBox.Show("The username and password combination did " +
"not match any of the employees",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// ... and close the application
Close();
}
}
}
}
private void SwitchboardLoaded(object sender, EventArgs e)
{
LogInToTheApplication();
}
- To test the application, press F5
- When the dialog box comes up, click Cancel
- Execute the application again
- When the dialog box displays, type the user name as Pasquale and press
Enter
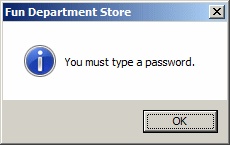
- Read the message box and click OK
- Execute the application again
- When the dialog box displays, type the user name as opasquale and press
Tab
- Type the password as L'Italiano and press Enter
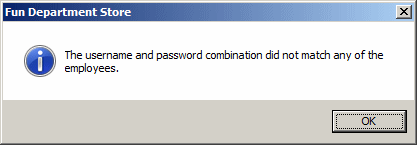
- Read the message box and press Enter
- Execute the application again
- Type the username as mtownsend and press Tab
- Type the password as Password4 and pass Enter
- Read the message box and click OK
- Execute the application again
- In the username of the dialog box, type mtownsend and press Tab
- Type the password as Password5
- Click OK
- Click the View Store Inventory button
- Close the forms
- To create a new file, on the main menu, click Project -> Add
New Item...
- In the middle list, click Code File
- Set the Name to CreateStoreItem
- Click Add
- In the empty document, type the following:
using System;
using System.Data;
using System.Drawing;
using System.Data.OleDb;
using System.Windows.Forms;
using System.ComponentModel;
public class CreateStoreItem : Form
{
private Label lblItemNumber;
private TextBox txtItemNumber;
private Label lblArrivalDate;
private MaskedTextBox txtArrivalDate;
private Label lblManufacturer;
private TextBox txtManufacturer;
private Label lblCategory;
private ComboBox cbxCategories;
private Label lblSubCategory;
private ComboBox cbxSubCategories;
private Label lblItemName;
private TextBox txtItemName;
private Label lblItemSize;
private TextBox txtItemSize;
private Label lblUnitPrice;
private TextBox txtUnitPrice;
private Label lblDiscountRate;
private TextBox txtDiscountRate;
private Label lblPercent;
private Label lblSaleStatus;
private ComboBox cbxSaleStatus;
private Button btnReset;
private Button btnCreate;
private Button btnClose;
public CreateStoreItem()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblItemNumber = new Label();
txtItemNumber = new TextBox();
lblArrivalDate = new Label();
txtArrivalDate = new MaskedTextBox();
lblManufacturer = new Label();
txtManufacturer = new TextBox();
lblCategory = new Label();
cbxCategories = new ComboBox();
lblSubCategory = new Label();
cbxSubCategories = new ComboBox();
lblItemName = new Label();
txtItemName = new TextBox();
lblItemSize = new Label();
txtItemSize = new TextBox();
lblUnitPrice = new Label();
txtUnitPrice = new TextBox();
lblDiscountRate = new Label();
txtDiscountRate = new TextBox();
lblPercent = new Label();
lblSaleStatus = new Label();
cbxSaleStatus = new ComboBox();
btnReset = new Button();
btnCreate = new Button();
btnClose = new Button();
SuspendLayout();
lblItemNumber.AutoSize = true;
lblItemNumber.Location = new Point(11, 14);
lblItemNumber.Size = new Size(40, 13);
lblItemNumber.TabIndex = 0;
lblItemNumber.Text = "Item #:";
txtItemNumber.Location = new Point(95, 11);
txtItemNumber.Size = new Size(96, 20);
txtItemNumber.TabIndex = 1;
lblArrivalDate.AutoSize = true;
lblArrivalDate.Location = new Point(223, 14);
lblArrivalDate.Size = new Size(65, 13);
lblArrivalDate.TabIndex = 2;
lblArrivalDate.Text = "Arrival Date:";
txtArrivalDate.Location = new Point(303, 11);
txtArrivalDate.Mask = "00/00/0000";
txtArrivalDate.Size = new Size(121, 20);
txtArrivalDate.TabIndex = 3;
txtArrivalDate.ValidatingType = typeof(System.DateTime);
lblManufacturer.AutoSize = true;
lblManufacturer.Location = new Point(11, 43);
lblManufacturer.Size = new Size(73, 13);
lblManufacturer.TabIndex = 4;
lblManufacturer.Text = "Manufacturer:";
txtManufacturer.Location = new Point(95, 40);
txtManufacturer.Size = new Size(330, 20);
txtManufacturer.TabIndex = 5;
lblCategory.AutoSize = true;
lblCategory.Location = new Point(11, 72);
lblCategory.Size = new Size(52, 13);
lblCategory.TabIndex = 6;
lblCategory.Text = "Category:";
cbxCategories.FormattingEnabled = true;
cbxCategories.Items.AddRange(new object[] {
"Men", "Girls", "Boys",
"Babies", "Women", "Other"});
cbxCategories.Location = new Point(95, 70);
cbxCategories.Size = new Size(116, 21);
cbxCategories.TabIndex = 7;
lblSubCategory.AutoSize = true;
lblSubCategory.Location = new Point(223, 73);
lblSubCategory.Size = new Size(74, 13);
lblSubCategory.TabIndex = 8;
lblSubCategory.Text = "Sub-Category:";
cbxSubCategories.FormattingEnabled = true;
cbxSubCategories.Items.AddRange(new object[] {
"Skirts", "Pants", "Shirts", "Shoes",
"Blouse", "Beauty", "Dresses", "Clothing",
"Sweater", "Watches", "Handbags", "Miscellaneous"});
cbxSubCategories.Location = new Point(303, 70);
cbxSubCategories.Size = new Size(121, 21);
cbxSubCategories.TabIndex = 9;
lblItemName.AutoSize = true;
lblItemName.Location = new Point(11, 104);
lblItemName.Size = new Size(61, 13);
lblItemName.TabIndex = 10;
lblItemName.Text = "Item Name:";
txtItemName.Location = new Point(95, 100);
txtItemName.Size = new Size(329, 20);
txtItemName.TabIndex = 11;
lblItemSize.AutoSize = true;
lblItemSize.Location = new Point(12, 132);
lblItemSize.Size = new Size(30, 13);
lblItemSize.TabIndex = 12;
lblItemSize.Text = "Size:";
txtItemSize.Location = new Point(95, 129);
txtItemSize.Size = new Size(96, 20);
txtItemSize.TabIndex = 13;
lblUnitPrice.AutoSize = true;
lblUnitPrice.Location = new Point(224, 135);
lblUnitPrice.Size = new Size(56, 13);
lblUnitPrice.TabIndex = 14;
lblUnitPrice.Text = "Unit Price:";
txtUnitPrice.Location = new Point(304, 132);
txtUnitPrice.Size = new Size(121, 20);
txtUnitPrice.TabIndex = 15;
txtUnitPrice.Text = "0.00";
txtUnitPrice.TextAlign = HorizontalAlignment.Right;
lblDiscountRate.AutoSize = true;
lblDiscountRate.Location = new Point(11, 161);
lblDiscountRate.Size = new Size(78, 13);
lblDiscountRate.TabIndex = 16;
lblDiscountRate.Text = "Discount Rate:";
txtDiscountRate.Location = new Point(95, 158);
txtDiscountRate.Size = new Size(96, 20);
txtDiscountRate.TabIndex = 17;
txtDiscountRate.Text = "0.00";
txtDiscountRate.TextAlign = HorizontalAlignment.Right;
lblPercent.AutoSize = true;
lblPercent.Font = new Font("Microsoft Sans Serif", 9.75F,
FontStyle.Bold, GraphicsUnit.Point, 0);
lblPercent.Location = new Point(191, 161);
lblPercent.Size = new Size(21, 16);
lblPercent.TabIndex = 18;
lblPercent.Text = "%";
lblSaleStatus.AutoSize = true;
lblSaleStatus.Location = new Point(224, 164);
lblSaleStatus.Name = "lblSaleStatus";
lblSaleStatus.Size = new Size(64, 13);
lblSaleStatus.TabIndex = 19;
lblSaleStatus.Text = "Sale Status:";
cbxSaleStatus.FormattingEnabled = true;
cbxSaleStatus.Items.AddRange(new object[] {
"Sold", "In Stock", "On Display",
"Processing", "Other"});
cbxSaleStatus.Location = new Point(304, 161);
cbxSaleStatus.Size = new Size(121, 21);
cbxSaleStatus.TabIndex = 20;
btnReset.Location = new Point(125, 193);
btnReset.Size = new Size(95, 23);
btnReset.TabIndex = 23;
btnReset.Text = "Reset";
btnReset.UseVisualStyleBackColor = true;
btnReset.Click += new System.EventHandler(btnResetClicked);
btnCreate.Location = new Point(226, 193);
btnCreate.Size = new Size(108, 23);
btnCreate.TabIndex = 21;
btnCreate.Text = "Create";
btnCreate.UseVisualStyleBackColor = true;
btnCreate.Click += new System.EventHandler(btnCreateClicked);
btnClose.Location = new Point(340, 193);
btnClose.Size = new Size(83, 23);
btnClose.TabIndex = 22;
btnClose.Text = "Close";
btnClose.UseVisualStyleBackColor = true;
btnClose.Click += new System.EventHandler(btnClose_Click);
Controls.Add(lblItemNumber);
Controls.Add(txtItemNumber);
Controls.Add(lblArrivalDate);
Controls.Add(txtArrivalDate);
Controls.Add(lblManufacturer);
Controls.Add(txtManufacturer);
Controls.Add(lblCategory);
Controls.Add(cbxCategories);
Controls.Add(lblSubCategory);
Controls.Add(cbxSubCategories);
Controls.Add(lblItemName);
Controls.Add(txtItemName);
Controls.Add(lblItemSize);
Controls.Add(txtItemSize);
Controls.Add(lblUnitPrice);
Controls.Add(txtUnitPrice);
Controls.Add(lblDiscountRate);
Controls.Add(txtDiscountRate);
Controls.Add(lblPercent);
Controls.Add(lblSaleStatus);
Controls.Add(cbxSaleStatus);
Controls.Add(btnCreate);
Controls.Add(btnReset);
Controls.Add(btnClose);
ClientSize = new Size(437, 230);
FormBorderStyle = FormBorderStyle.FixedDialog;
MaximizeBox = false;
MinimizeBox = false;
ShowInTaskbar = false;
StartPosition = FormStartPosition.CenterScreen;
Text = "Fun Department Store - Create New Store Item";
Load += new System.EventHandler(CreateStoreItemLoaded);
ResumeLayout(false);
PerformLayout();
}
private void btnResetClicked(object sender, EventArgs e)
{
txtItemNumber.Text = "";
txtArrivalDate.Text = DateTime.Today.ToShortDateString();
txtManufacturer.Text = "";
cbxCategories.Text = "";
cbxSubCategories.Text = "";
txtItemName.Text = "";
txtItemSize.Text = "";
txtUnitPrice.Text = "0.00";
txtDiscountRate.Text = "0.00";
cbxSaleStatus.Text = "In Stock";
}
private void CreateStoreItemLoaded(object sender, EventArgs e)
{
// When the form opens, reset it
btnResetClicked(sender, e);
}
private void btnCreateClicked(object sender, EventArgs e)
{
double unitPrice = 0.00;
double discountRate = 0.00;
// Make sure the user entered an item number.
// If not, don't do anything
if (txtItemNumber.Text.Length == 0)
{
MessageBox.Show("You must provide a (unique) item number.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
// On a related issue, for the simplicity of this exercise,
// We are not writing code to check whether the user provided
// a duplicate item number. If the user does, the application
// itself would throw an exception. In another article, we may have
// to take care of that problem. For now, we will ignore it
// Make sure the user entered a name for the new item.
// If not, don't do anything
if (txtItemName.Text.Length == 0)
{
MessageBox.Show("You must provide a name for the item.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
// Make sure the user provided a price for the item
if (txtUnitPrice.Text.Length == 0)
{
MessageBox.Show("You must provide a price for the item.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
// Check if the user provided a valid price...
try
{
unitPrice = double.Parse(txtUnitPrice.Text);
}
catch (FormatException)
{
// ... if not, use 0.00
}
// Check if the user provided a valid discount rate...
try
{
discountRate = double.Parse(txtDiscountRate.Text);
}
catch (FormatException)
{
// ... if not, use 0.00
}
// Connect to the database
// To connect to the database, after the following Data Source= expression,
// type \\, followed by the name of the server (ours is named Expression),
// followed by \\, followed by the name of the folder where the database
// is installed (ours is named Fun Department Store) (of course, you must
// have shared that folder), followed by \\, and followed by the name of
// the database, in this case FunDS
using (OleDbConnection conFunDS =
new OleDbConnection(@"Provider=Microsoft.ACE.OLEDB.12.0;" +
@"Data Source=\\Expression\\Fun Department Store\\FunDS.accdb"))
{
string strSQLInsert = "INSERT INTO StoreItems(ItemNumber, ArrivalDate, " +
"Manufacturer, Category, SubCategory, ItemName, " +
"ItemSize, UnitPrice, DiscountRate, SaleStatus) " +
"VALUES(" + txtItemNumber.Text + ", '" +
txtArrivalDate.Text + "', '" +
txtManufacturer.Text + "', '" +
cbxCategories.Text + "', '" +
cbxSubCategories.Text + "', '" +
txtItemName.Text + "', '" +
txtItemSize.Text + "', " +
unitPrice + ", " +
discountRate + ", '" +
cbxSaleStatus.Text + "');";
// Generate a command to create a record
OleDbCommand cmdStoreItems = new OleDbCommand(strSQLInsert, conFunDS);
conFunDS.Open();
cmdStoreItems.ExecuteNonQuery();
// Once the item has been created, let the user know
MessageBox.Show("The item has been created",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
btnResetClicked(sender, e);
}
}
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
}
- In the Solution Explorer, double-click Authenticator.cs
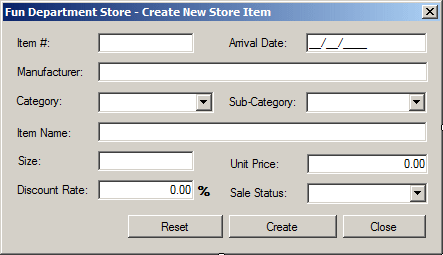
- To create a new file, on the main menu, click Project -> Add New Item
- In the middle list, make sure Code File is selected.
Change the name
to Employees
- Click Add
- In the empty document, type the following:
using System;
using System.IO;
using System.Data;
using System.Drawing;
using System.Data.OleDb;
using System.Windows.Forms;
using System.ComponentModel;
public class Employees : Form
{
private ColumnHeader colEmployeeNumber;
private ColumnHeader colFirstName;
private ColumnHeader colLastName;
private ColumnHeader colTitle;
private ColumnHeader colManager;
private ColumnHeader colHourlySalary;
private ColumnHeader colUsername;
private ColumnHeader colPassword;
private ListView lvwEmployees;
public GroupBox grpNewEmployee;
private Label lblEmployeeNumber;
private MaskedTextBox txtEmployeeNumber;
private Label lblFirstName;
private TextBox txtFirstName;
private Label lblLastName;
private TextBox txtLastName;
private Label lblTitle;
private TextBox txtTitle;
private Button btnReset;
private CheckBox chkManager;
private Label lblHourlySalary;
private TextBox txtHourlySalary;
private Button btnSubmit;
private Label lblUsername;
private TextBox txtUsername;
private Label lblPassword;
private TextBox txtPassword;
private Button btnClose;
public Employees()
{
InitializeComponent();
}
private void InitializeComponent()
{
colEmployeeNumber = new ColumnHeader();
colFirstName = new ColumnHeader();
colLastName = new ColumnHeader();
colTitle = new ColumnHeader();
colManager = new ColumnHeader();
colHourlySalary = new ColumnHeader();
colUsername = new ColumnHeader();
colPassword = new ColumnHeader();
lvwEmployees = new ListView();
grpNewEmployee = new GroupBox();
lblEmployeeNumber = new Label();
txtEmployeeNumber = new MaskedTextBox();
lblFirstName = new Label();
txtFirstName = new TextBox();
lblLastName = new Label();
txtLastName = new TextBox();
lblTitle = new Label();
txtTitle = new TextBox();
btnReset = new Button();
chkManager = new CheckBox();
lblHourlySalary = new Label();
txtHourlySalary = new TextBox();
btnSubmit = new Button();
lblUsername = new Label();
txtUsername = new TextBox();
lblPassword = new Label();
txtPassword = new TextBox();
btnClose = new Button();
grpNewEmployee.SuspendLayout();
SuspendLayout();
colEmployeeNumber.Text = "Employee #";
colEmployeeNumber.Width = 70;
colFirstName.Text = "First Name";
colFirstName.Width = 80;
colLastName.Text = "Last Name";
colLastName.Width = 80;
colTitle.Text = "Title";
colTitle.Width = 120;
colManager.Text = "Manager";
colHourlySalary.Text = "Salary";
colHourlySalary.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
colHourlySalary.Width = 50;
colUsername.Text = "Username";
colPassword.Text = "Password";
lvwEmployees.Columns.AddRange(new ColumnHeader[] {
colEmployeeNumber, colFirstName, colLastName, colTitle,
colManager, colHourlySalary, colUsername, colPassword});
lvwEmployees.FullRowSelect = true;
lvwEmployees.GridLines = true;
lvwEmployees.Location = new Point(12, 12);
lvwEmployees.Size = new Size(606, 191);
lvwEmployees.TabIndex = 0;
lvwEmployees.UseCompatibleStateImageBehavior = false;
lvwEmployees.View = System.Windows.Forms.View.Details;
grpNewEmployee.Controls.Add(lblEmployeeNumber);
grpNewEmployee.Controls.Add(txtEmployeeNumber);
grpNewEmployee.Controls.Add(lblFirstName);
grpNewEmployee.Controls.Add(txtFirstName);
grpNewEmployee.Controls.Add(lblLastName);
grpNewEmployee.Controls.Add(txtLastName);
grpNewEmployee.Controls.Add(lblTitle);
grpNewEmployee.Controls.Add(txtTitle);
grpNewEmployee.Controls.Add(btnReset);
grpNewEmployee.Controls.Add(chkManager);
grpNewEmployee.Controls.Add(lblHourlySalary);
grpNewEmployee.Controls.Add(txtHourlySalary);
grpNewEmployee.Controls.Add(btnSubmit);
grpNewEmployee.Controls.Add(lblUsername);
grpNewEmployee.Controls.Add(txtUsername);
grpNewEmployee.Controls.Add(lblPassword);
grpNewEmployee.Controls.Add(txtPassword);
grpNewEmployee.Location = new Point(12, 209);
grpNewEmployee.Size = new Size(605, 165);
grpNewEmployee.TabIndex = 1;
grpNewEmployee.TabStop = false;
grpNewEmployee.Text = "New Employee";
lblEmployeeNumber.AutoSize = true;
lblEmployeeNumber.Location = new Point(42, 29);
lblEmployeeNumber.Size = new Size(66, 13);
lblEmployeeNumber.TabIndex = 0;
lblEmployeeNumber.Text = "Employee #:";
txtEmployeeNumber.Location = new Point(124, 26);
txtEmployeeNumber.Mask = "00-000";
txtEmployeeNumber.Size = new Size(52, 20);
txtEmployeeNumber.TabIndex = 1;
lblFirstName.AutoSize = true;
lblFirstName.Location = new Point(42, 55);
lblFirstName.Size = new Size(60, 13);
lblFirstName.TabIndex = 2;
lblFirstName.Text = "First Name:";
txtFirstName.Location = new Point(124, 52);
txtFirstName.Size = new Size(100, 20);
txtFirstName.TabIndex = 3;
lblLastName.AutoSize = true;
lblLastName.Location = new Point(245, 55);
lblLastName.Size = new Size(61, 13);
lblLastName.TabIndex = 4;
lblLastName.Text = "Last Name:";
txtLastName.Location = new Point(327, 52);
txtLastName.Name = "txtLastName";
txtLastName.Size = new Size(100, 20);
txtLastName.TabIndex = 5;
lblTitle.AutoSize = true;
lblTitle.Location = new Point(42, 81);
lblTitle.Size = new Size(30, 13);
lblTitle.TabIndex = 6;
lblTitle.Text = "Title:";
txtTitle.Location = new Point(124, 78);
txtTitle.Name = "txtTitle";
txtTitle.Size = new Size(303, 20);
txtTitle.TabIndex = 7;
btnReset.Location = new Point(447, 104);
btnReset.Size = new Size(97, 23);
btnReset.TabIndex = 15;
btnReset.Text = "Reset";
btnReset.UseVisualStyleBackColor = true;
btnReset.Click += new System.EventHandler(btnResetClicked);
chkManager.AutoSize = true;
chkManager.CheckAlign = ContentAlignment.MiddleRight;
chkManager.Location = new Point(45, 107);
chkManager.Size = new Size(91, 17);
chkManager.TabIndex = 8;
chkManager.Text = "Is a Manager:";
chkManager.UseVisualStyleBackColor = true;
lblHourlySalary.AutoSize = true;
lblHourlySalary.Location = new Point(245, 107);
lblHourlySalary.Size = new Size(72, 13);
lblHourlySalary.TabIndex = 9;
lblHourlySalary.Text = "Hourly Salary:";
txtHourlySalary.Location = new Point(327, 104);
txtHourlySalary.Size = new Size(100, 20);
txtHourlySalary.TabIndex = 10;
txtHourlySalary.Text = "0.00";
txtHourlySalary.TextAlign = HorizontalAlignment.Right;
btnSubmit.Location = new Point(447, 132);
btnSubmit.Size = new Size(97, 23);
btnSubmit.TabIndex = 16;
btnSubmit.Text = "Submit";
btnSubmit.UseVisualStyleBackColor = true;
btnSubmit.Click += new System.EventHandler(btnSubmitClicked);
lblUsername.AutoSize = true;
lblUsername.Location = new Point(42, 133);
lblUsername.Size = new Size(58, 13);
lblUsername.TabIndex = 11;
lblUsername.Text = "Username:";
txtUsername.Location = new Point(124, 130);
txtUsername.Size = new Size(100, 20);
txtUsername.TabIndex = 12;
lblPassword.AutoSize = true;
lblPassword.Location = new Point(245, 132);
lblPassword.Size = new Size(56, 13);
lblPassword.TabIndex = 13;
lblPassword.Text = "Password:";
txtPassword.Location = new Point(327, 130);
txtPassword.PasswordChar = '*';
txtPassword.Size = new Size(100, 20);
txtPassword.TabIndex = 14;
btnClose.Location = new Point(459, 384);
btnClose.Size = new Size(97, 23);
btnClose.TabIndex = 17;
btnClose.Text = "Close";
btnClose.UseVisualStyleBackColor = true;
btnClose.Click += new System.EventHandler(btnCloseClicked);
ClientSize = new Size(629, 414);
Controls.Add(grpNewEmployee);
Controls.Add(lvwEmployees);
Controls.Add(btnClose);
FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
MaximizeBox = false;
MinimizeBox = false;
ShowInTaskbar = false;
StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
Text = "Fun Department Store - Employees";
Load += new System.EventHandler(Employees_Load);
grpNewEmployee.ResumeLayout(false);
grpNewEmployee.PerformLayout();
ResumeLayout(false);
}
private void ShowEmployees()
{
lvwEmployees.Items.Clear();
// To connect to the database, after the following Data Source= expression,
// type \\, followed by the name of the server (ours is named Expression),
// followed by \\, followed by the name of the folder where the database
// is installed (ours is named Fun Department Store) (of course, you must
// have shared that folder), followed by \\, and followed by the name of
// the database, in this case FunDS
using (OleDbConnection conFunDS =
new OleDbConnection(@"Provider=Microsoft.ACE.OLEDB.12.0;" +
@"Data Source=\\Expression\\Fun Department Store\\FunDS.accdb"))
{
// Select all store items
OleDbCommand cmdFunDS = new OleDbCommand("SELECT * FROM Employees;", conFunDS);
OleDbDataAdapter odaFunDS = new OleDbDataAdapter(cmdFunDS);
DataSet dsEmployees = new DataSet("EmployeesSet");
odaFunDS.Fill(dsEmployees);
conFunDS.Open();
// Using the total number of records, display each in the list view
for (int i = 0; i < dsEmployees.Tables[0].Rows.Count; i++)
{
DataRow rcdEmployee = dsEmployees.Tables[0].Rows[i];
ListViewItem lviEmployee = new ListViewItem((i + 1).ToString());
lviEmployee.SubItems.Add(rcdEmployee["employeeNumber"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["FirstName"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["LastName"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["Title"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["Manager"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["HourlySalary"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["Username"].ToString());
lviEmployee.SubItems.Add(rcdEmployee["UserPassword"].ToString());
lvwEmployees.Items.Add(lviEmployee);
}
}
}
private void Employees_Load(object sender, EventArgs e)
{
ShowEmployees();
btnResetClicked(sender, e);
}
private void btnResetClicked(object sender, EventArgs e)
{
Random rndNumber = new Random();
txtEmployeeNumber.Text = rndNumber.Next(10000, 99999).ToString();
txtFirstName.Text = "";
txtLastName.Text = "";
txtTitle.Text = "";
chkManager.Checked = false;
txtHourlySalary.Text = "0.00";
txtUsername.Text = "";
txtPassword.Text = "";
}
private void btnSubmitClicked(object sender, EventArgs e)
{
double hourlySalary = 0.00;
try
{
hourlySalary = double.Parse(txtHourlySalary.Text);
}
catch (FormatException)
{
}
// Connect to the database
// To connect to the database, after the following Data Source= expression,
// type \\, followed by the name of the server (ours is named Expression),
// followed by \\, followed by the name of the folder where the database
// is installed (ours is named Fun Department Store) (of course, you must
// have shared that folder), followed by \\, and followed by the name of
// the database, in this case FunDS
using (OleDbConnection conFunDS =
new OleDbConnection(@"Provider=Microsoft.ACE.OLEDB.12.0;" +
@"Data Source=\\Expression\\Fun Department Store\\FunDS.accdb"))
{
string strSQLInsert = "INSERT INTO Employees(EmployeeNumber, FirstName, " +
"LastName, Title, Manager, HourlySalary, " +
"Username, UserPassword) VALUES('" +
txtEmployeeNumber.Text + "', '" +
txtFirstName.Text + "', '" +
txtLastName.Text + "', '" +
txtTitle.Text + "', " +
chkManager.Checked + ", " +
hourlySalary + ", '" +
txtUsername.Text + "', '" +
txtPassword.Text + "');";
// Generate a command to create a record
OleDbCommand cmdEmployees = new OleDbCommand(strSQLInsert, conFunDS);
conFunDS.Open();
cmdEmployees.ExecuteNonQuery();
MessageBox.Show("The employee has been created.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
btnResetClicked(sender, e);
}
}
private void btnCloseClicked(object sender, EventArgs e)
{
Close();
}
}
- Save all
We refer to contextual the ability to grant access or
some operations to one or more objects of an application to one or more
people. This may sound complicated but it really is not. Or the real
difficulty lies, not in programming, but in logic and organization
(design). One way to solve the problem would consist of making a list of
objects in your application, another list of people in your organization,
then specify who will have access to what and who would be denied access
to what. This would work perfectly fine in a small organization and a
small application that hardly changes, but this may not be practical in
larger scenarios. Another solution consists of creating groups of people,
adding people to those groups, and then deciding what group has access to
what.
In this example, we will use a field of the Employees
table to identify who can have access to the form used to create a new store
item.
Practical
Learning: Implementing Contextual Access
|
|
- Display the Switchboard.cs file
- Change the file as follows:
using System;
using System.Data;
using System.Drawing;
using System.Data.OleDb;
using System.Windows.Forms;
using System.ComponentModel;
public class Switchboard : Form
{
private Button btnStoreInventory;
private Button btnCreateStoreItem;
private Button btnEmployees;
private Button btnLogInUser;
private Button btnClose;
private bool employeeIsAManager;
public Switchboard()
{
InitializeComponent();
}
private void InitializeComponent()
{
btnStoreInventory = new Button();
btnCreateStoreItem = new Button();
btnEmployees = new Button();
btnLogInUser = new Button();
btnClose = new Button();
SuspendLayout();
btnStoreInventory.Font = new Font("Georgia", 18F, FontStyle.Bold,
GraphicsUnit.Point, 0);
btnStoreInventory.Location = new Point(11, 12);
btnStoreInventory.Size = new Size(227, 98);
btnStoreInventory.TabIndex = 21;
btnStoreInventory.Text = "View Store Inventory";
btnStoreInventory.UseVisualStyleBackColor = true;
btnStoreInventory.Click += new System.EventHandler(btnStoreInventoryClicked);
btnCreateStoreItem.Font = new Font("Georgia", 18F, FontStyle.Bold,
GraphicsUnit.Point, 0);
btnCreateStoreItem.Location = new Point(253, 12);
btnCreateStoreItem.Size = new Size(227, 98);
btnCreateStoreItem.TabIndex = 23;
btnCreateStoreItem.Text = "Create Store Item";
btnCreateStoreItem.UseVisualStyleBackColor = true;
btnCreateStoreItem.Click += new System.EventHandler(btnCreateStoreItemClicked);
btnEmployees.Font = new Font("Georgia", 18F, FontStyle.Bold, GraphicsUnit.Point, 0);
btnEmployees.Location = new Point(10, 128);
btnEmployees.Size = new Size(227, 98);
btnEmployees.TabIndex = 25;
btnEmployees.Text = "Employees";
btnEmployees.UseVisualStyleBackColor = true;
btnEmployees.Click += new System.EventHandler(btnEmployeesClicked);
btnLogInUser.Font = new Font("Georgia", 18F, FontStyle.Bold, GraphicsUnit.Point, 0);
btnLogInUser.Location = new Point(254, 128);
btnLogInUser.Size = new Size(227, 98);
btnLogInUser.TabIndex = 24;
btnLogInUser.Text = "Log in as a Different Employee";
btnLogInUser.UseVisualStyleBackColor = true;
btnLogInUser.Click += new System.EventHandler(btnLogInUserClicked);
btnClose.Font = new Font("Georgia", 18F, FontStyle.Bold,
GraphicsUnit.Point, 0);
btnClose.Location = new Point(10, 243);
btnClose.Size = new Size(470, 66);
btnClose.TabIndex = 22;
btnClose.Text = "Close Application";
btnClose.UseVisualStyleBackColor = true;
btnClose.Click += new System.EventHandler(btnCloseClicked);
ClientSize = new Size(493, 323);
Controls.Add(btnEmployees);
Controls.Add(btnLogInUser);
Controls.Add(btnCreateStoreItem);
Controls.Add(btnClose);
Controls.Add(btnStoreInventory);
StartPosition = FormStartPosition.CenterScreen;
Text = "Fun Department Store - Switchboard";
Load += new System.EventHandler(SwitchboardLoaded);
ResumeLayout(false);
}
private void btnStoreInventoryClicked(object sender, EventArgs e)
{
StoreInventory si = new StoreInventory();
si.ShowDialog();
}
private void btnCloseClicked(object sender, EventArgs e)
{
Close();
}
private void LogInToTheApplication()
{
bool usernamePasswordMatch = false;
bool currentEmployeeIsManager = false;
Authenticator dlgLogIn = new Authenticator();
string strPasswordFromDialogBox = "", strPasswordFromDatabase = "";
string strUsernameFromDialogBox = "", strUsernameFromDatabase = "";
// Display the Autheticator dialog box.
// If the user clicks Cancel, simply close the application
if (dlgLogIn.ShowDialog() == System.Windows.Forms.DialogResult.Cancel)
Close();
else // If the user clicks OK
{
// If the employee doesn't enter a username, display a message box...
if (dlgLogIn.txtUsername.Text == "")
{
MessageBox.Show("You must enter a user name.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// ... and close the application
Close();
}
// If the employee doesn't enter a password, display a message box...
if (dlgLogIn.txtPassword.Text == "")
{
MessageBox.Show("You must type a password.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// ... and close the application
Close();
}
// Get the user name of the dialog box
strUsernameFromDialogBox = dlgLogIn.txtUsername.Text;
// Get the password of the dialog box
strPasswordFromDialogBox = dlgLogIn.txtPassword.Text;
// To connect to the database, after the following Data Source= expression,
// type \\, followed by the name of the server (ours is named Expression),
// followed by \\, followed by the name of the folder where the database
// is installed (ours is named Fun Department Store) (of course, you must
// have shared that folder), followed by \\, and followed by the name of
// the database, in this case FunDS
using (OleDbConnection conFunDS =
new OleDbConnection(@"Provider=Microsoft.ACE.OLEDB.12.0;" +
@"Data Source=\\Expression\\Fun Department Store\\FunDS.accdb"))
{
// Get the records of all employees
OleDbCommand cmdFunDS = new OleDbCommand("SELECT * FROM Employees;", conFunDS);
// Create a data adapater to retrieve the employees
OleDbDataAdapter odaEmployees = new OleDbDataAdapter(cmdFunDS);
// Create a data set of employees
DataSet dsEmployees = new DataSet("EmployeesSet");
// Fill the data set with the Employees records
odaEmployees.Fill(dsEmployees);
// Open the connection
conFunDS.Open();
// Navigate to each record
for (int i = 0; i < dsEmployees.Tables[0].Rows.Count; i++)
{
// Get a reference to the current record
DataRow rcdEmployee = dsEmployees.Tables[0].Rows[i];
// Get the managing status of the employee
currentEmployeeIsManager = bool.Parse(rcdEmployee["Manager"].ToString());
// When you are on a record, get the username of the employee
strUsernameFromDatabase = rcdEmployee["Username"].ToString();
// and the password
strPasswordFromDatabase = rcdEmployee["UserPassword"].ToString();
// Compare the current username to the username of the dialog box
// and the current password to the password of the dialog box.
// If they match, ...
if (strUsernameFromDatabase.Equals(strUsernameFromDialogBox) &&
strPasswordFromDatabase.Equals(strPasswordFromDialogBox))
{
usernamePasswordMatch = true;
// Find out whether this employee is a manager.
// If so, make a reference...
if (currentEmployeeIsManager == true)
employeeIsAManager = true;
else
employeeIsAManager = false;
// ... and stop searching
break;
}
// If there is no match, continue to the next record,
// up to the end of the table
}
// If there was a match for username/password, display the Switchboard
// If there was no match for username/password,
// Let the employee know ...
if (usernamePasswordMatch == false)
{
MessageBox.Show("The username and password combination did " +
"not match any of the employees",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// ... and close the application
Close();
}
}
}
}
private void LogInAsADifferentEmployee()
{
bool currentEmployeeIsManager = false;
bool usernamePasswordMatch = false;
Authenticator dlgLogIn = new Authenticator();
string strPasswordFromDialogBox = "", strPasswordFromDatabase = "";
string strUsernameFromDialogBox = "", strUsernameFromDatabase = "";
// Display the Autheticator dialog box.
// If the user clicks Cancel, simply close the application
if (dlgLogIn.ShowDialog() == System.Windows.Forms.DialogResult.Cancel)
return;
else // If the user clicks OK
{
// If the employee doesn't enter a username, display a message box...
if (dlgLogIn.txtUsername.Text == "")
MessageBox.Show("You must enter a user name.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// If the employee doesn't enter a password, display a message box...
if (dlgLogIn.txtPassword.Text == "")
MessageBox.Show("You must type a password.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
// Get the user name of the dialog box
strUsernameFromDialogBox = dlgLogIn.txtUsername.Text;
// Get the password of the dialog box
strPasswordFromDialogBox = dlgLogIn.txtPassword.Text;
// To connect to the database, after the following Data Source= expression,
// type \\, followed by the name of the server (ours is named Expression),
// followed by \\, followed by the name of the folder where the database
// is installed (ours is named Fun Department Store) (of course, you must
// have shared that folder), followed by \\, and followed by the name of
// the database, in this case FunDS
using (OleDbConnection conFunDS =
new OleDbConnection(@"Provider=Microsoft.ACE.OLEDB.12.0;" +
@"Data Source=\\Expression\\Fun Department Store\\FunDS.accdb"))
{
// Get the records of all employees
OleDbCommand cmdFunDS = new OleDbCommand("SELECT * FROM Employees;", conFunDS);
// Create a data adapater to retrieve the employees
OleDbDataAdapter odaEmployees = new OleDbDataAdapter(cmdFunDS);
// Create a data set of employees
DataSet dsEmployees = new DataSet("EmployeesSet");
// Fill the data set with the Employees records
odaEmployees.Fill(dsEmployees);
// Open the connection
conFunDS.Open();
// Navigate to each record
for (int i = 0; i < dsEmployees.Tables[0].Rows.Count; i++)
{
// Get a reference to the current record
DataRow rcdEmployee = dsEmployees.Tables[0].Rows[i];
// Get the managing status of the employee
currentEmployeeIsManager = bool.Parse(rcdEmployee["Manager"].ToString());
// When you are on a record, get the username of the employee
strUsernameFromDatabase = rcdEmployee["Username"].ToString();
// and the password
strPasswordFromDatabase = rcdEmployee["UserPassword"].ToString();
// Compare the current username to the username of the dialog box
// and the current password to the password of the dialog box.
// If they match, ...
if (strUsernameFromDatabase.Equals(strUsernameFromDialogBox) &&
strPasswordFromDatabase.Equals(strPasswordFromDialogBox))
{
usernamePasswordMatch = true;
// Find out whether this employee is a manager.
// If so, make a reference.
if (currentEmployeeIsManager == true)
employeeIsAManager = true;
else
employeeIsAManager = false;
// ... display the switchboard
break;
}
// If there is no match, continue to the next record,
// up to the end of the table
}
// If there was a match for username/password, display the Switchboard
// If there was no match for username/password,
// Let the employee know ...
if (usernamePasswordMatch == false)
{
MessageBox.Show("The username and password combination did " +
"not match any of the employees",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
}
}
}
private void SwitchboardLoaded(object sender, EventArgs e)
{
employeeIsAManager = false;
LogInToTheApplication();
}
private void btnCreateStoreItemClicked(object sender, EventArgs e)
{
CreateStoreItem csi = new CreateStoreItem();
// Using the global canCreateStoreItem variable, find out
// whether the current user is a manager.
// If the user is, then display the Create Store Item form
if (employeeIsAManager == true)
csi.ShowDialog();
else // If the user is not, diaplay a message box
MessageBox.Show("You are not authorized to create store items.",
"Fun Department Store",
MessageBoxButtons.OK, MessageBoxIcon.Information);
}
private void btnLogInUserClicked(object sender, EventArgs e)
{
LogInAsADifferentEmployee();
}
private void btnEmployeesClicked(object sender, EventArgs e)
{
Employees staff = new Employees();
// If the user is a manager, enable the controls in the group box.
if (employeeIsAManager == true)
staff.grpNewEmployee.Enabled = true;
else // Otherwise, disable them
staff.grpNewEmployee.Enabled = false;
staff.ShowDialog();
}
}
public class DepartmentStore
{
public static int Main()
{
Application.Run(new Switchboard());
return 0;
}
}
- To test the application, press F5
- When the dialog box comes up, click Cancel. Notice that the application
did not continue
- Execute the application again
- When the dialog box displays, type the user name as stanley and press
Enter
- Type the password as Password5 and press Enter
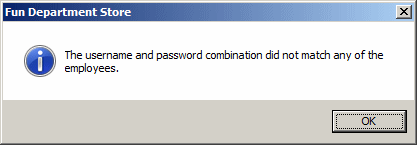
- Read the message box and press Enter
- Execute the application again
- Type the username as mtownsend and press Tab
- Type the password as Password5 and pass Enter
- Click View Store Inventory
- Close the form
- Click Create Store Item
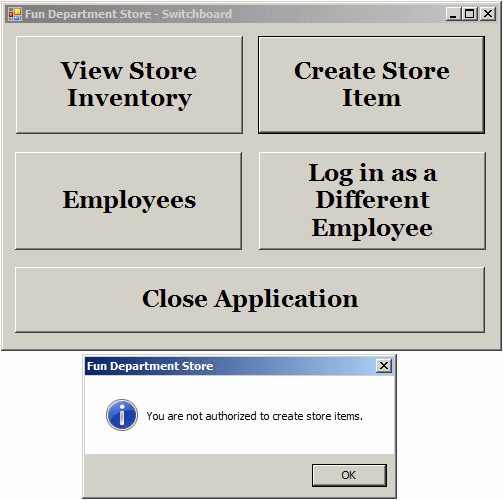
- Read the message and click OK
- Click Log in as a Different Employees
- In the username of the dialog box, type msamson and press Tab
- Type the password as password16 and press Enter
- Click Employees
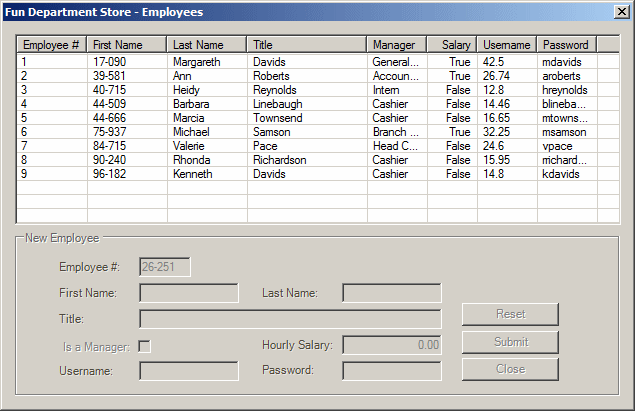
- Close the Employees form
- Click Log in as a Different Employees
- In the username of the dialog box, type msamson and press Tab
- Type the password as Password6 and press Enter
- Click Create Store Item
- Enter some values for the record
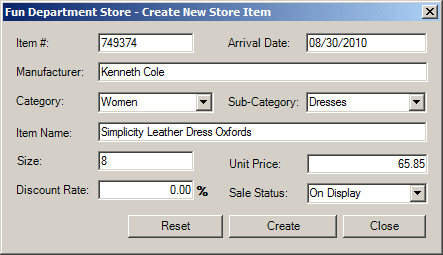
- Click Create
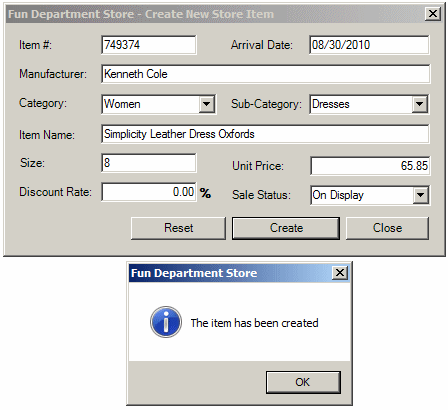
- Read the message box and click OK
- Close the form
- Click Employees
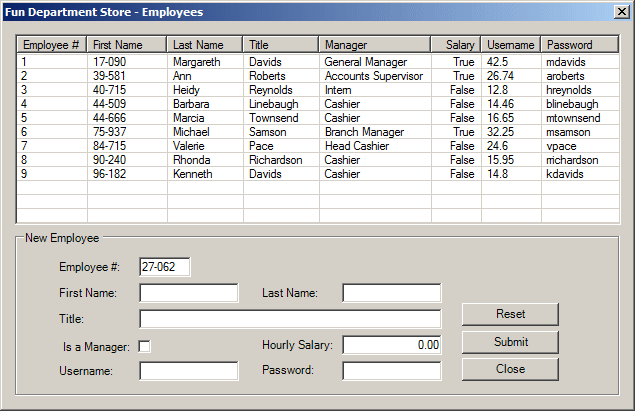
- Close the Employees form
- Click Log in as a Different Employees
- Click Cancel
- Click Log in as a Different Employees
- In the username of the dialog box, type kdavids and press Tab
- Type the password as Password9 and press Enter
- Click View Store Inventory
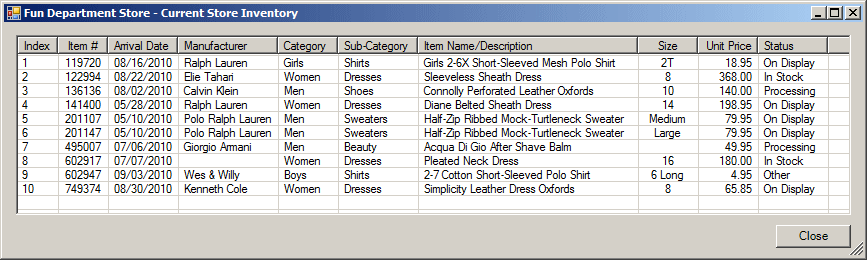
- Close the form
- Cick Create Store Iteam
- Read the message and click OK
- Close the Switchboard form
|
|