In this example, we will calculate the overtime worked by an employee of a
company. To do this, we will consider each week day with 8 regular hours. If an
employee works more than 8 hours in one day, any time over that is considered
overtime. In our payroll simulation, we will cover two weeks but each week has
its separate calculation. After collecting the time for both weeks and
performing the calculation, we will display the number of regular hours, the
number of hours worked overtime, the amount pay for the regular hours, and the
amount pay for overtime, if any. At the end, we will display the total net pay.
Practical
Learning: Introducing the Date Picker
|
|
- Start a new Windows Application named GCSPayroll1
- Set the form's Icon to Drive:\Program Files\Microsoft Visual Studio
.NET 2003\Common7\Graphics\icons\Office\CRDFLE03.ICO
- Design the form as follows:
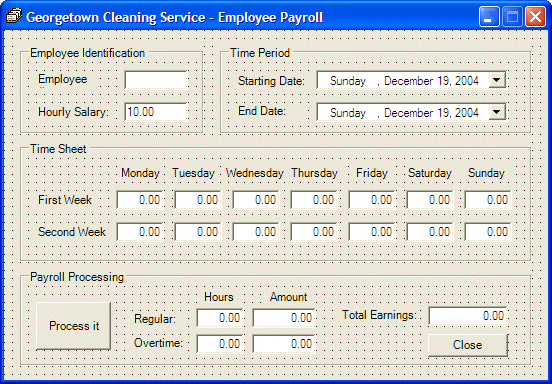 |
Control |
Name |
Text |
Other Properties |
GroupBox |
 |
|
Employee Identification |
|
Label |
 |
|
Employee #: |
|
TextBox |
 |
txtEmployeeNbr |
|
|
Label |
 |
|
Hourly Salary: |
|
TextBox |
 |
txtHourlySalary |
|
|
GroupBox |
 |
|
Time Period |
|
Label |
 |
Starting Date: |
|
|
DateTimePicker |
 |
dtpStartingDate |
|
|
Label |
 |
End Date: |
|
|
DateTimePicker |
 |
dtpEndDate |
|
|
GroupBox |
 |
|
Time Sheet |
|
Label |
 |
|
Monday |
|
Label |
 |
|
Tuesday |
|
Label |
 |
|
Wednesday |
|
Label |
 |
|
Thursday |
|
Label |
 |
|
Friday |
|
Label |
 |
|
Saturday |
|
Label |
 |
|
Sunday |
|
Label |
 |
|
First Week: |
|
TextBox |
 |
txtMonday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtTuesday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtWednesday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtThursday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtFriday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSaturday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSunday1 |
0.00 |
TextAlign: Right |
Label |
 |
|
Second Week: |
|
TextBox |
 |
txtMonday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtTuesday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtWednesday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtThursday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtFriday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSaturday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSunday2 |
0.00 |
TextAlign: Right |
GroupBox |
 |
|
Payroll Processing |
|
Label |
 |
|
Hours |
|
Label |
 |
|
Amount |
|
Button |
 |
btnProcessIt |
Process It |
|
Label |
 |
|
Regular |
|
TextBox |
 |
txtRegularHours |
0.00 |
TextAlign: Right |
TextBox |
 |
txtRegularAmount |
0.00 |
TextAlign: Right |
Label |
 |
|
Total Earnings |
|
TextBox |
 |
txtTotalEarnings |
0.00 |
TextAlign: Right |
Label |
 |
|
Overtime |
|
TextBox |
 |
txtOvertimeHours |
0.00 |
TextAlign: Right |
TextBox |
 |
txtOvertiimeAmount |
0.00 |
TextAlign: Right |
Button |
 |
btnClose |
|
|
|
- Double-click the Close button and implement its Click event as follows:
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
|
- Execute it to test it and then close the form
- Double-click an unoccupied area of the form and implement its Load event
as follows:
private void Form1_Load(object sender, System.EventArgs e)
{
DateTime todayDate = DateTime.Today;
DateTime twoWeeksAgo = todayDate.AddDays(-14);
this.dtpStartingDate.Value = twoWeeksAgo;
}
|
- Return to the form and click the top DateTimePicker control
- In the Properties window, click the Events button
and double-click the CloseUp field
- Implement the event as follows:
private void dtpStartingDate_CloseUp(object sender, System.EventArgs e)
{
// Get the starting date
DateTime dteStart = this.dtpStartingDate.Value;
// Find out if the user selected a day that is not Monday
if( dteStart.DayOfWeek != DayOfWeek.Monday )
{
MessageBox.Show("The date you selected in invalid\n" +
"The time period should start on a Monday");
this.dtpStartingDate.Focus();
}
}
|
- Return to the form and click the other DateTimePicker control
- In the Properties window, click the Events button
and double-click the CloseUp field
- Implement the event as follows:
private void dtpEndDate_CloseUp(object sender, System.EventArgs e)
{
// Get the starting date
DateTime dteStart = this.dtpStartingDate.Value;
// Get the ending date
DateTime dteEnd = this.dtpEndDate.Value;
// Make sure the first day of the period is Monday
if( dteStart.DayOfWeek != DayOfWeek.Monday )
{
MessageBox.Show("The starting date you selected in invalid\n" +
"The time period should start on a Monday");
this.dtpStartingDate.Focus();
}
// Find the number of days that separate the start and end
TimeSpan timeDifference = dteEnd.Subtract(dteStart);
double fourteenDaysLater = timeDifference.Days;
if( (dteEnd.DayOfWeek != DayOfWeek.Sunday) || (fourteenDaysLater != 13) )
{
MessageBox.Show("The ending date you selected in invalid\n" +
"The time period should span 2 weeks and end on a Sunday");
this.dtpEndDate.Focus();
}
}
|
- Return to the form. Double-click the Process It button and implement its
Click event as follows:
private void btnPresseIt_Click(object sender, System.EventArgs e)
{
double monday1 = 0.00, tuesday1 = 0.00, wednesday1 = 0.00, thursday1 = 0.00,
friday1 = 0.00, saturday1 = 0.00, sunday1 = 0.00,
monday2 = 0.00, tuesday2 = 0.00, wednesday2 = 0.00, thursday2 = 0.00,
friday2 = 0.00, saturday2 = 0.00, sunday2 = 0.00;
double totalHoursWeek1, totalHoursWeek2;
double regHours1 = 0.00, regHours2 = 0.00, ovtHours1 = 0.00, ovtHours2 = 0.00;
double regAmount1 = 0.00, regAmount2 = 0.00, ovtAmount1 = 0.00, ovtAmount2 = 0.00;
double regularHours, overtimeHours;
double regularAmount, overtimeAmount, totalEarnings;
double hourlySalary = 0.00;
// Retrieve the hourly salary
try
{
hourlySalary = double.Parse(this.txtHourlySalary.Text);
}
catch(FormatException)
{
MessageBox.Show("The value you typed for the salary is invalid \n" +
"Please try again");
this.txtHourlySalary.Focus();
}
// Retrieve the value of each day worked
try
{
monday1 = double.Parse(this.txtMonday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtMonday1.Focus();
}
try
{
tuesday1 = double.Parse(this.txtTuesday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtTuesday1.Focus();
}
try
{
wednesday1 = double.Parse(this.txtWednesday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtWednesday1.Focus();
}
try
{
thursday1 = double.Parse(this.txtThursday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtThursday1.Focus();
}
try
{
friday1 = double.Parse(this.txtFriday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtFriday1.Focus();
}
try
{
saturday1 = double.Parse(this.txtSaturday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtSaturday1.Focus();
}
try
{
sunday1 = double.Parse(this.txtSunday1.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtSunday1.Focus();
}
try
{
monday2 = double.Parse(this.txtMonday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtMonday2.Focus();
}
try
{
tuesday2 = double.Parse(this.txtTuesday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtTuesday2.Focus();
}
try
{
wednesday2 = double.Parse(this.txtWednesday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtWednesday2.Focus();
}
try
{
thursday2 = double.Parse(this.txtThursday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtThursday2.Focus();
}
try
{
friday2 = double.Parse(this.txtFriday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtFriday2.Focus();
}
try
{
saturday2 = double.Parse(this.txtSaturday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtSaturday2.Focus();
}
try
{
sunday2 = double.Parse(this.txtSunday2.Text);
}
catch(FormatException)
{
MessageBox.Show("You typed an invalid value\n" +
"Please try again");
this.txtSunday2.Focus();
}
// Calculate the total number of hours for each week
totalHoursWeek1 = monday1 + tuesday1 + wednesday1 + thursday1 +
friday1 + saturday1 + sunday1;
totalHoursWeek2 = monday2 + tuesday2 + wednesday2 + thursday2 +
friday2 + saturday2 + sunday2;
// The overtime is paid time and half
double ovtSalary = hourlySalary * 1.5;
// If the employee worked under 40 hours, there is no overtime
if( totalHoursWeek1 < 40 )
{
regHours1 = totalHoursWeek1;
regAmount1 = hourlySalary * regHours1;
ovtHours1 = 0.00;
ovtAmount1 = 0.00;
} // If the employee worked over 40 hours, calculate the overtime
else if( totalHoursWeek1 >= 40 )
{
regHours1 = 40;
regAmount1 = hourlySalary * 40;
ovtHours1 = totalHoursWeek1 - 40;
ovtAmount1 = ovtHours1 * ovtSalary;
}
if( totalHoursWeek2 < 40 )
{
regHours2 = totalHoursWeek2;
regAmount2 = hourlySalary * regHours2;
ovtHours2 = 0.00;
ovtAmount2 = 0.00;
}
else if( totalHoursWeek2 >= 40 )
{
regHours2 = 40;
regAmount2 = hourlySalary * 40;
ovtHours2 = totalHoursWeek2 - 40;
ovtAmount2 = ovtHours2 * ovtSalary;
}
regularHours = regHours1 + regHours2;
overtimeHours = ovtHours1 + ovtHours2;
regularAmount = regAmount1 + regAmount2;
overtimeAmount = ovtAmount1 + ovtAmount2;
totalEarnings = regularAmount + overtimeAmount;
this.txtRegularHours.Text = regularHours.ToString("F");
this.txtOvertimeHours.Text = overtimeHours.ToString("F");
this.txtRegularAmount.Text = regularAmount.ToString("F");
this.txtOvertimeAmount.Text = overtimeAmount.ToString("F");
this.txtTotalEarnings.Text = totalEarnings.ToString("F");
}
|
- Execute the application to test it
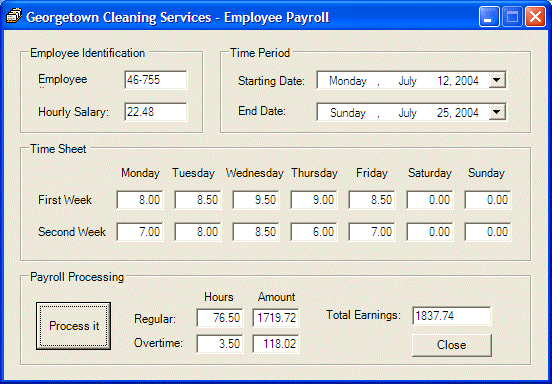
- After using it, close the form and return to your programming environment
|
|