Techniques of Passing Arguments
|
|
Passing an Argument By Value
|
|
When calling a procedure that takes
an argument, we were supplying a value for that argument. When this is done,
the procedure that is called makes a copy of the value of the argument and
makes that copy available to the calling procedure. That way, the argument
itself is not accessed. This is referred to as passing an argument by value.
This can be reinforced by typing the ByVal keyword on the left side
of the argument. Here is an example:
Private Sub Welcome(ByVal strLanguage As String)
MsgBox("Welcome to the wonderful world of " & strLanguage)
End Sub
If you create a procedure that takes an argument by
value and you have used the ByVal keyword on the argument, when
calling the procedure, you don't need to use the ByVal keyword; just
the name of the argument is enough, as done in the examples on arguments so
far. Here is an example:
Public Module Exercise
Public Function Main() As Integer
Dim ComputerLanguage As String = "Visual Basic"
Welcome(ComputerLanguage)
Return 0
End Function
Private Sub Welcome(ByVal strLanguage As String)
MsgBox("Welcome to the wonderful world of " & strLanguage)
End Sub
End Module
This would produce:
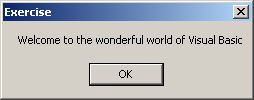
Passing an Argument By
Reference
|
|
An alternative to passing an argument as done so far is
to pass the address of the argument to the procedure. When this is done, the
procedure doesn't receive a simple copy of the value of the argument: the
argument is accessed by its address. That is, at its memory address. With
this technique, any action carried on the argument will be kept. If the
value of the argument is modified, the argument would now have the new
value, dismissing or losing the original value it had. This technique is
referred to as passing an argument by reference. Consider the following
program:
Public Module Exercise
Private Function Addition#(ByVal Value1 As Double, ByVal Value2 As Double)
Value1 = InputBox("Enter First Number: ")
Value2 = InputBox("Enter Second Number: ")
Addition = Value1 + Value2
End Function
Public Function Main() As Integer
Dim Result As String
Dim Number1, Number2 As Double
Result = Addition(Number1, Number2)
MsgBox(Number1 & " + " & Number2 & " = " & Result)
Return 0
End Function
End Module
Here is an example of running the program:
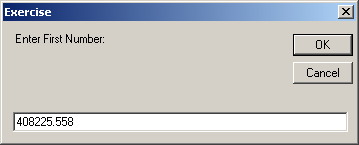
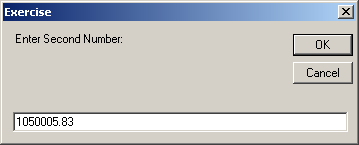
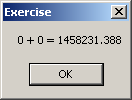
Notice that, although the values of the arguments were
changed in the Addition() procedure, at the end of the procedure, they lose
the value they got in the function. If you want a procedure to change the
value of an argument, you can pass the argument by reference.
To pass an argument by reference, on its left, type the
ByRef keyword. This is done only when defining the procedure. When
the procedure finishes with the argument, the argument would keep
whatever modification was made on its value. Now consider the same program
as above but with arguments passed by reference:
Public Module Exercise
Private Function Addition#(ByRef Value1 As Double, ByRef Value2 As Double)
Value1 = InputBox("Enter First Number: ")
Value2 = InputBox("Enter Second Number: ")
Addition = Value1 + Value2
End Function
Public Function Main() As Integer
Dim Result As String
Dim Number1, Number2 As Double
Result = Addition(Number1, Number2)
MsgBox(Number1 & " + " & Number2 & " = " & Result)
Return 0
End Function
End Module
Here is an example of running the program:
Using this technique, you can pass as many arguments by
reference and as many arguments by value as you want. As you may guess
already, this technique is also used to make a procedure return a value,
which a regular procedure cannot do. Furthermore, passing arguments by
reference allows a procedure to return as many values as possible while a
regular function can return only one value.
Practical
Learning: Passing Arguments by Reference
|
|
- To pass an argument by reference, change the file as follows:
Module Geometry
Private Sub GetValues(ByRef Length As Double, ByRef Width As Double)
Length = InputBox("Enter the length:")
Width = InputBox("Enter the width:")
End Sub
Private Function CalculatePerimeter(ByVal Length As Double,
ByVal Width As Double) As Double
CalculatePerimeter = (Length + Width) * 2
End Function
Private Function CalculateArea(ByVal Length As Double, _
ByVal Width As Double) As Double
CalculateArea = Length * Width
End Function
Private Sub ShowCharacteristics(ByVal Length As Double,
ByVal Width As Double)
Dim Result As String
Result = "=-= Rectangle Characteristics =-=" & vbCrLf &
"Length: " & vbTab & vbTab & CStr(Length) & vbCrLf &
"Width: " & vbTab & vbTab & CStr(Width) & vbCrLf &
"Perimeter: " & vbTab &
CalculatePerimeter(Length, Width) & vbCrLf &
"Area: " & vbTab & vbTab &
CalculateArea(Length, Width)
MsgBox(Result)
End Sub
Public Function Main() As Integer
Dim L As Double, W As Double
GetValues(L, W)
ShowCharacteristics(L, W)
Return 0
End Function
End Module
- To execute the program, on the Standard toolbar, click the Start
Debugging button

- Enter the length as 24.55 and the width as
22.85
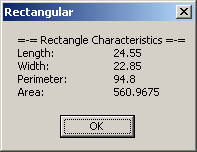
- Close the message box and return to your programming environment
Other Techniques of Passing Arguments
|
|
If you create a procedure that takes one or more
arguments, whenever you call that procedure, you must provide a value for
the argument(s). Otherwise,, you would receive an error. If such an argument
is passed with the same value over and over again, you may be tempted to
remove the argument altogether. In some cases, although a certain argument
is passed with the same value most of the time, you still have situations in
which you want the user to decide whether to pass a value or not for the
argument, you can declare the value optional. In other words, you can create
the argument with a default value so that the user can call the procedure
without passing a value for the argument, thus passing a value only when
necessary. Such an argument is called default or optional.
Imagine you write a procedure that will be used to
calculate the final price of an item after discount. The procedure would
need the discount rate in order to perform the calculation. Such a procedure
could look like this:
Function CalculateNetPrice#(ByVal DiscountRate As Double)
Dim OrigPrice#
OrigPrice = InputBox("Please enter the original price:")
Return OrigPrice - (OrigPrice * DiscountRate / 100)
End Function
Since this procedure expects an argument, if you do not
supply it, the following program would not compile:
Public Module Exercise
Function CalculateNetPrice#(ByVal DiscountRate As Double)
Dim OrigPrice#
OrigPrice = InputBox("Please enter the original price:")
Return OrigPrice - (OrigPrice * DiscountRate / 100)
End Function
Public Function Main() As Integer
Dim FinalPrice#
Dim Discount# = 15 ' That is 25% = 25
FinalPrice = CalculateNetPrice(Discount)
MsgBox("Final Price = " & FinalPrice)
Return 0
End Function
End Module
Here is an example of running the program:
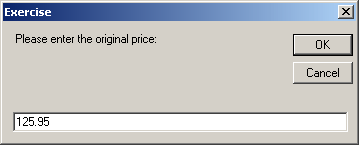
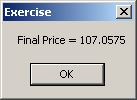
Most of the time, a procedure such as ours would use the
same discount rate over and over again. Therefore, instead of supplying an
argument all the time, you can define an argument whose value would be used
whenever the function is not provided with the argument.
To specify that an argument is
optional, when creating its procedure, type the Optional keyword to
the left of the argument's name and assign it the default value. Here is an
example:
Public Module Exercise
Function CalculateNetPrice#(Optional ByVal DiscountRate As Double = 20)
Dim OrigPrice#
OrigPrice = InputBox("Please enter the original price:")
Return OrigPrice - (OrigPrice * DiscountRate / 100)
End Function
Public Function Main() As Integer
Dim FinalPrice#
Dim Discount# = 15 ' That is 25% = 25
FinalPrice = CalculateNetPrice()
MsgBox("Final Price = " & FinalPrice)
Return 0
End Function
End Module
Here is an example of running the program:
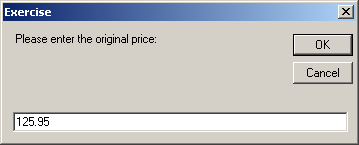

If a procedure takes more than one argument, you can
provide a default argument for each and select which ones would have default
values. If you want all arguments to have default values, when defining the
procedure , provide the Optional keyword for each and assign it the
desired default value. Here is an example:
Public Module Exercise
Function CalculateNetPrice#(Optional ByVal Tax As Double = 5.75,
Optional ByVal Discount As Double = 25,
Optional ByVal OrigPrice As Double = 245.55)
Dim DiscountValue As Double = OrigPrice * Discount / 100
Dim TaxValue As Double = Tax / 100
Dim NetPrice As Double = OrigPrice - DiscountValue + TaxValue
Dim Result As String
Result = "Original Price: " & vbTab & CStr(OrigPrice) & vbCrLf &
"Discount Rate: " & vbTab & CStr(Discount) & "%" & vbCrLf &
"Tax Amount: " & vbTab & CStr(Tax)
MsgBox(Result)
Return NetPrice
End Function
Public Function Main() As Integer
Dim FinalPrice As Double
FinalPrice = CalculateNetPrice()
MsgBox("Final Price: " & CStr(FinalPrice))
Return 0
End Function
End Module
This would produce:
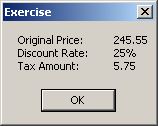
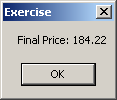
If a procedure takes more than one argument as above,
remember that some arguments can be specified as optional. In this case,
when calling the procedure, any argument that does not have a default value
must be passed with a value. When creating a procedure that takes more than
one argument, the argument(s) that has(have) default value(s) must be the
last in the procedure. This means that:
- If a procedure takes two arguments and one argument has a default
value, this optional argument must be the second
- If a procedure is taking three or more arguments and two or more
arguments have default values, these optional arguments must by placed
to the right of the non-optional argument(s).
Because of this, when calling any procedure in the
Visual Basic language, you must know what, if any, argument is optional and
which one is not.
If a procedure takes two arguments and one argument has
a default value, when calling this procedure, you can pass only one value.
In this case, the passed value would be applied on the first argument. If a
procedure takes more than two arguments and two or more arguments have a
default value, when calling this procedure, you can provide only the
value(s) of the argument that is (are) not optional. If you want to provide
the value of one of the arguments but that argument is not the first
optional, you can leave empty the position(s) of the other argument(s) but
remember to type a comma to indicate that the position is that of an
argument that has a default value. Here is an example:
Public Module Exercise
Function CalculateNetPrice(ByVal AcquiredPrice As Double,
ByVal MarkedPrice As Double,
Optional ByVal TaxRate As Double = 5.75,
Optional ByVal DiscountRate As Double = 25) As Double
Dim DiscountAmount As Double = MarkedPrice * DiscountRate / 100
Dim TaxAmount As Double = MarkedPrice * TaxRate / 100
Dim NetPrice As Double = MarkedPrice - DiscountAmount + TaxAmount
Dim Result As String
Result = "Price Acquired: " & vbTab & CStr(AcquiredPrice) & vbCrLf &
"Marked Price: " & vbTab & CStr(MarkedPrice) & vbCrLf &
"Discount Rate: " & vbTab & CStr(DiscountRate) & "%" & vbCrLf &
"Discount Amt: " & vbTab & CStr(DiscountAmount) & vbCrLf &
"Tax Rate: " & vbTab & CStr(TaxRate) & "%" & vbCrLf &
"Tax Amount: " & vbTab & CStr(TaxAmount)
MsgBox(Result)
Return NetPrice
End Function
Public Function Main() As Integer
Dim FinalPrice As Double
FinalPrice = CalculateNetPrice(225.55, 150.55, , 40)
MsgBox("Final Price: " & CStr(FinalPrice))
Return 0
End Function
End Module
This would produce:
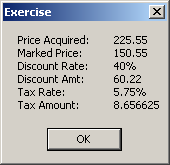
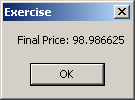
A program involves a great deal of names that represent
variables and procedures of various kinds. The compiler does not allow two
variables to have the same name in the same procedure (or in the same
scope). Although two procedures should have unique names in the same
program, you are allowed to use the same name for different procedures of
the same program following certain rules.
The ability to have various procedures with the same
name in the same program is referred to as overloading. The most important
rule about procedure overloading is to make sure that each one of these
procedures has a different number or different type(s) of arguments.
Practical
Learning: Overloading a Procedure
|
|
The moment of inertia is the ability of a beam to resist
bending. It is calculated with regard to the cross section of the beam.
Because it depends on the type of section of the beam, its calculation also
depends on the type of section of the beam. In this exercise, we will review
different formulas used to calculate the moment of inertia. Since this
exercise is for demonstration purposes, you do not need to be a Science
Engineering major to understand it.
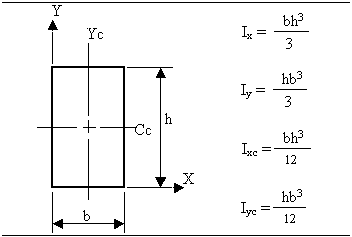
- To start a new project, on the main menu, click File -> New
Project...
- In the middle list, click Console Application
- Change the Name to MomentOfInertia1
- Click OK
- In the Solution Explorer, right-click Module1.vb and click Rename
- Type MomentOfInertia.vb and press Enter
- To calculate the moment of inertia of a rectangle, change the file
as follows:
Module MomentOfInertia
' Moment of Inertia
' Rectangle
Private Function MomentOfInertia(ByVal b As Double,
ByVal h As Double) As Double
Return b * h * h * h / 3
End Function
Public Sub Main()
Dim Base As Double, Height As Double
Base = InputBox("Enter the base of the Rectangle")
Height = InputBox("Enter the height of the Rectangle")
MsgBox("Moment of inertia with regard to the X axis" & vbCrLf &
"I = " & CStr(MomentOfInertia(Base, Height)) & "mm")
End Sub
End Module
- To execute the program, on the main menu, click Debug -> Start
Debugging
- Enter the base as 3.25
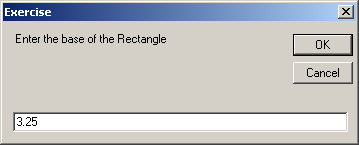
- Enter the height as 2.85
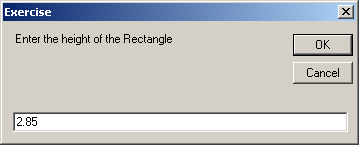
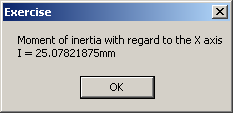
- Close the DOS window and return to your programming environment
- Here are the formulas to calculate the moment of inertia for a
semi-circle:
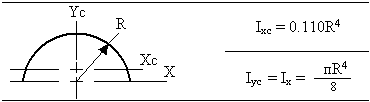
A circle, and thus a semi-circle, requires
only a radius. Since the other version of the MomentOfInertia()
function requires two arguments, we can overload it by providing
only one argument, the radius.
|
To calculate the moment of inertia of a rectangle, change the file as
follows:
Module MomentOfInertia
' Moment of Inertia
' Rectangle
Private Function MomentOfInertia(ByVal b As Double,
ByVal h As Double) As Double
Return b * h * h * h / 3
End Function
' Semi-Circle
Function MomentOfInertia(ByVal R As Double) As Double
Const PI As Double = 3.14159
Return R * R * R * R * PI / 8
End Function
Public Sub Main()
' Dim Base As Double, Height As Double
Dim Radius As Double
' Base = InputBox("Enter the base of the Rectangle:")
' Height = InputBox("Enter the height of the Rectangle")
' MsgBox("Moment of inertia with regard to the X axis" & vbCrLf & _
' "I = " & CStr(MomentOfInertia(Base, Height)) & "mm")
Radius = InputBox("Enter the radius of the semi-circle:")
MsgBox("Moment of inertia of a semi-circle with " &
"regard to the X axis:" & vbCrLf &
"I = " & CStr(MomentOfInertia(Radius)) & "mm")
End Sub
End Module
|
- To execute the program, on the Standard toolbar, click the Start
Debugging button

- Enter the radius as 6.35
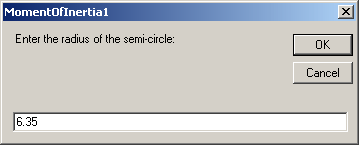
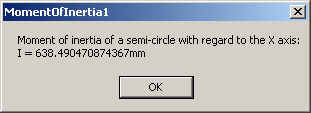
- Close the DOS window and return to your programming environment
- On the main menu, click File -> Close Solution (Microsoft Visual
Studio) or File -> Close Project (Microsoft Visual Basic 2010 Express)
- When asked whether you want to save, click Discard
|
|