|
We saw that you can get the result of a LINQ statement
from the Select section. In reality, the Select statement
simply indicates that the result is ready and it hands it to the other parts
of the program. Instead of getting the result directly from the Select
statement, you can first store it in a local LINQ variable. This allows you
to treat the result as a variable that you can then manipulate before
getting the final result.
|
To create a local variable in the LINQ statement, you
can use the Let operator. You must use it before the Select
statement to hold the result. Here is an example:
Imports System.Linq
Imports System.Collections.Generic
Public Class Employee
Public EmployeeNumber As Integer
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
Public Sub New(Optional ByVal Number As Integer = 0,
Optional ByVal FName As String = "John",
Optional ByVal LName As String = "Doe",
Optional ByVal salary As Double = 0D)
EmployeeNumber = Number
FirstName = FName
LastName = LName
HourlySalary = salary
End Sub
End Class
Module Exercise
Public Function Main() As Integer
Dim Employees() As Employee =
{
New Employee(971974, "Patricia", "Katts", 24.68),
New Employee(208411, "Raymond", "Kouma", 20.15),
New Employee(279374, "Hélène", "Mukoko", 15.55),
New Employee(707912, "Bertrand", "Yamaguchi", 24.68),
New Employee(294800, "Peter", "Mukoko", 18.85),
New Employee(971394, "Gertrude", "Monay", 20.55)
}
Dim FullNames = From Empls
In Employees
Let FullName = Empls.LastName + ", " + Empls.FirstName
Select FullName
For Each Empl In FullNames
Console.WriteLine(Empl)
Next
Console.WriteLine()
Return 0
End Function
End Module
This would produce:
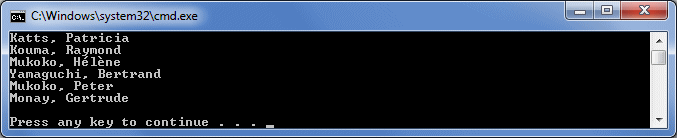
If you need a Where condition but your Let
variable would be used only to hold the final result, you can declare that
Let variable after the Where statement. Here is an example:
Dim FullNames = From Empls
In Employees
Where Empls.LastName = "Mukoko"
Let FullName = Empls.LastName & ", " & Empls.FirstName
Select FullName
This would produce:

You can create the Let variable before the
Where statement and you would get the same result:
Dim FullNames = From Empls
In Employees
Let FullName = Empls.LastName & ", " & Empls.FirstName
Where Empls.LastName = "Mukoko"
Select FullName