|
An animation is a series of pictures put together to
produce a video clip. It can be used to display the evolution of an ongoing
task to the user. This makes such tasks less boring. For example, making a
copy of a CD is usually a long process that can take minutes. To let the
user know when such a task is being performed, you can display an animation.
|
Microsoft Windows provides a few small animations you
can use for your applications. If you need an animation other than those
supplied, you may have to create it. Microsoft Visual C++ is not the place
to create an animation. You may need a graphics software to do this.
To use a regular animation, the video must be a
standard Microsoft Windows audio/video format: Audio Video Interleaved or
AVI. Therefore, it must be a file with the avi extension. If the file has
both audio and video, only the video part would be considered.
Practical
Learning: Introducing Animations
|
|
- To create a new application, press Ctrl + N
- Select MFC Application
- Set the Name to Video1
- Click OK
- In the first page of the wizard, click Next
- In the second page of the wizard, click Dialog Based and click
Next
- In the third page of the wizard, click set the Title to Video
Animation
- Click Finish
- Click TODO and press Delete
- Click the OK button and press Delete
- Change the caption of the Cancel button to &Close
Getting an Animation Control
|
|
An animation first originates from an avi file created
by an external application. Therefore, you must already have the video you
want to play in your application. To provide an animation for your
application, at design time, from the Toolbox, click the Animation Control
and click the desired area on the host.
The animator control is based on the
CAnimatorCtrl class. Therefore, if you want to programmatically
create an animation, you must first declare a variable of type, or a
pointer to, CAnimationCtrl. You can do this in the source
code of the dialog class. After declaring the variable or pointer, to
initialize the object, call its Create() member function.
Here is an example:
class CControlsDlg : public CDialog
{
// Construction
public:
CControlsDlg(CWnd* pParent = NULL); // standard constructor
~CControlsDlg();
. . .
private:
CAnimateCtrl *Player;
};
CControlsDlg::CControlsDlg(CWnd* pParent /*=NULL*/)
: CDialog(CControlsDlg::IDD, pParent)
{
//{{AFX_DATA_INIT(CControlsDlg)
// NOTE: the ClassWizard will add member initialization here
//}}AFX_DATA_INIT
Player = new CAnimateCtrl;
}
CControlsDlg::~CControlsDlg()
{
delete Player;
}
. . .
BOOL CControlsDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// TODO: Add extra initialization here
RECT Recto = { 5, 5, 360, 360 };
Player->Create(WS_CHILD | WS_VISIBLE,
Recto, this, 0x1884);
return TRUE; // return TRUE unless you set the focus to a control
// EXCEPTION: OCX Property Pages should return FALSE
}
Characteristics of the Animation Control
|
|
After creating the control, you must provide a video
to play. This is done by opening a video file using the
CAnimateCtrl::Open() member function. It is overloaded with two
versions as follows:
BOOL Open(LPCTSTR lpszFileName);
BOOL Open(UINT nID);
The first version expects the path of the video file.
Alternatively, you can first add the file as a resource to your project
and use its identifier as argument to the second version. Here is an
example:
BOOL CControlsDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// TODO: Add extra initialization here
RECT Recto = { 5, 5, 360, 360 };
Player->Create(WS_CHILD | WS_VISIBLE | ACS_TRANSPARENT,
Recto, this, 0x1884);
Player->Open("res\\clock.AVI");
return TRUE; // return TRUE unless you set the focus to a control
// EXCEPTION: OCX Property Pages should return FALSE
}
The Transparency of an Animation
|
|
In this case, the center of the video would match the
center of your rectangle, even though the animation may still not exactly
match the dimensions of your rectangle.
When playing the video, you have the option of
displaying the original background color of the video or seeing through.
When creating a video, its author can do it with transparency to allow
seeing the color of the host. In this case, to display the color of the
host while the video is playing, set the Transparent property to True. If
you are creating the control programmatically, add the
ACS_TRANSPARENT style:
BOOL CControlsDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// TODO: Add extra initialization here
RECT Recto = { 5, 5, 360, 360 };
Player->Create(WS_CHILD | WS_VISIBLE |
ACS_TRANSPARENT | ACS_AUTOPLAY,
Recto, this, 0x1884);
return TRUE; // return TRUE unless you set the focus to a control
// EXCEPTION: OCX Property Pages should return FALSE
}
Practical
Learning: Show an Animation
|
|
- From the Toolbox, click Animation Control
- Draw a rectangle from the upper left section of the dialog box to
the right-middle
- In the Properties window, change the following characteristics:
Auto Play: True
Center: True
Client Edge: False
ID:
IDC_ANIMATOR
Transparent: True
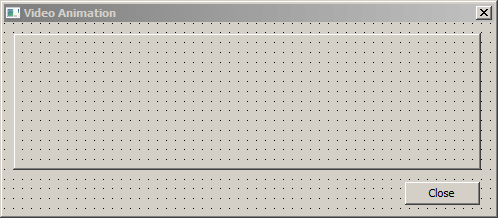
- On the dialog box, right-click the animator control and click Add
Variable
- In the Category combo box, make sure Control is selected.
In
the Variable Name, type m_Animator
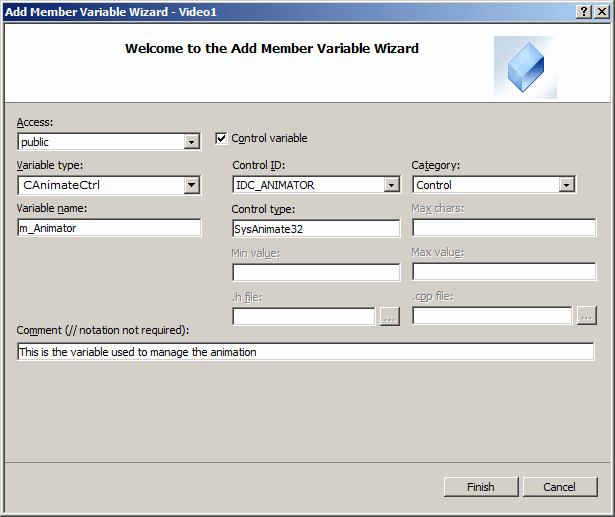
- Click Finish