 |
Introduction to Arrays |
|
A Series of Similar Items |
|
|
An array is a
series of items of the same kind. It could be a group of numbers, a group of
cars, a group of words, etc but all items of the array must be of the same type.
|
To create an array, you can use the following formula:
DataType[] VariableName = new DataType[Number];
If you are working in Microsoft Visual Studio or Microsoft
Visual Web Developer, you can use the above formula or you can use the var keyword
as follows:
var VariableName = new DataType[Number];
The DataType can be a primitive data type (char, int, float, double,
decimal, string, etc). It can also be the name of a class. Like a normal variable, an array must have a name,
represented in our formula as VariableName. The
square brackets on the left of the assignment operator are used. The new operator
is required to reserve memory. The Number is used
to specify the number of items of the list.
Based on the above formula, here is an example of an
array variable:
<%@ Page Language="C#" %>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
double numbers = new double[5];
%>
</body>
</html>
Introduction to Initializing an Array
|
|
When creating an array, each item of the series is referred to as a member or an
element of
the array. Once the array has been created, each one of its members is
initialized with a 0 value. Most, if not all, of the time, you will need to
change the value of each member to a value of your choice. This is referred to
as initializing the array.
An array is primarily a variable and can be
initialized. There are two main ways you can initialize an array.
If you have declared an array as done above, to initialize it, you can access
each one of its members and assign it a desired but appropriate value.
The index of a member of
an array is written in its own square brackets. Here is an example:
<body>
<%
double[] numbers = new double[5];
numbers[0] = 12.44;
numbers[1] = 525.38;
numbers[2] = 6.28;
numbers[3] = 2448.32;
numbers[4] = 632.04;
%>
</body>
The value between square brackets must be known. For example, the value can be returned from a
function or a method. Here is an
example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
int GetIndex()
{
return 2;
}
</script>
<title>Exercise</title>
</head>
<body>
<%
double[] numbers = new double[5];
numbers[0] = 12.44;
numbers[1] = 525.38;
numbers[GetIndex()] = 6.28;
numbers[3] = 2448.32;
numbers[4] = 632.04;
%>
</body>
</html>
You can also initialize the array as
a whole when declaring it. To do this, on the right side of the declaration,
before the closing semi-colon, type the values of the array members between
curly brackets and separated by a comma. Here is an example:
<%
double[] numbers = new double[5] { 12.44, 525.38, 6.28, 2448.32, 632.04 };
%>
If you use this second technique, you don't have to specify
the number of items in the series. In this case, you can leave all square brackets
empty.
Other Techniques of Initializing an Array
|
|
We have initialized our arrays so far with values we specified
directly in the curly brackets. If you have declared and initialized some
variables of the same type, you can use them to initialize an array. The values can also come from constant variables.
The values can also come from calculations, whether the
calculation is from an initialized variable or made locally in the curly
brackets.
All of the arrays we have declared so far were using only
numbers. The numbers we used were decimal constants. If the array is made of
integers, you can use decimal, hexadecimal values, or a combination of both.
An array can have types of values of any of the data types
we have used so far. The rule to follow is that all members of the array must be
of the same type. For example, you can declare an array of Boolean values, as
long as all values can be evaluated to true or false.
Accessing the Members of an Array
|
|
After initializing an array, to access a member, you use
the square brackets. As done for normal variables, one of the
reasons of accessing a member of an array would be to display its value. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
double[] numbers = new double[5] { 12.44, 525.38, 6.28, 2448.32, 632.04 };
Response.Write(numbers[3]);
%>
</body>
</html>
This would produce:
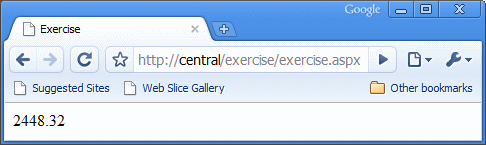
In the same way, you can access 1, a few or all members of
the array.
For an Indexed Member of the Array
|
|
Accessing each
member of the array using the square brackets allows you to access one, a few,
or each member. If you plan to access all members of the array instead of just
one or a few, you can use the for loop. The formula
to follow is:
for(DataType Initializer; EndOfRange; Increment) Do What You Want;
In this formula, the for keyword, the parentheses,
and the semi-colons are required. The DataType factor is used to specify
how you will count the members of the array.
The Initializer specifies how you would indicate the
starting of the count.
As seen in Lesson 12, this initialization could use an initialized int-based
variable.
The EndOfRange specifies how you would stop counting.
If you are using an array, it should combine a conditional operation (<, <=, >,
>=, or !=) with the number of members of the array minus 1.
The Increment factor specifies how you would move
from one index to the next.
Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
double[] numbers = new double[5] { 12.44, 525.38, 6.28, 2448.32, 632.04 };
for(int i = 0; i < 5; i++)
Response.Write(numbers[i] + "<br />");
%>
</body>
</html>
This would produce:
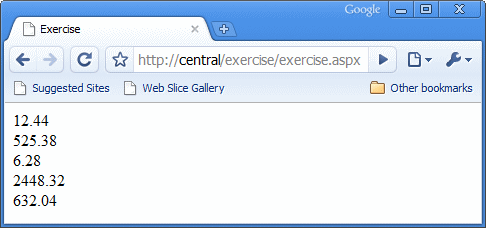
When using a for loop, you should pay attention to
the number of items you use. If you use a number n less than the total number of
members - 1, only the first n members of the array would be accessed. On the other hand, if you use a number of items higher than the number of
members minus one, you would get an IndexOutOfRangeException
exception. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
double[] numbers = new double[5] { 12.44, 525.38, 6.28, 2448.32, 632.04 };
for(int i = 0; i < 12; i++)
Response.Write(numbers[i] + "<br />");
%>
</body>
</html>
This would produce:
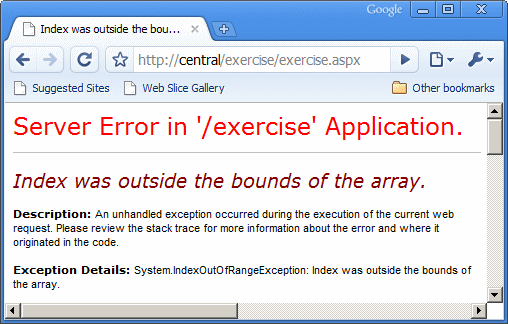
You could solve the above problem by using exception
handling to handle an IndexOutOfRangeException exception.
For Each Member in the Array
|
|
In a for loop, you should know the number of members
of the array. An alternative is to use the foreach operator. Its
formula is:
foreach (type identifier in expression) statement
The foreach and the in keywords are required.
The first factor of this syntax, type, can be var
or the
type of the members of the array. It can also be the name of
a class as we will learn in Lesson 23.
The identifier factor is a name of the variable you
will use.
The expression factor is the name of the array
variable. The statement is what you intend to do with the
identifier or as a result of accessing the member of the array. Like
a for loop that accesses all members of the array, the foreach
operator is used to access each array member, one at a time. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
double[] numbers = new double[5] { 12.44, 525.38, 6.28, 2448.32, 632.04 };
foreach(double d in numbers)
Response.Write(d + "<br />");
%>
</body>
</html>
|