 |
Static Fields, Methods, and Classes |
|
|
Normally, to access a class in another method, you declare a
variable for it. A
variable you have declared of a class is also called an instance of the class.
In the same way, you can declare various instances of the same class as
necessary.
|
Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
public class Book
{
public string Title;
public string Author;
public short YearPublished;
public int NumberOfPages;
public char CoverType;
}
</script>
<title>Exercise</title>
</head>
<body>
<%
Book written = new Book();
Book bought = new Book();
%>
</body>
</html>
Each one of these instances gives you access to the members
of the class but each instance holds the particular values of the members of its
instance. Consider the results of the following program:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
public class Book
{
public string Title;
public string Author;
public short YearPublished;
public int NumberOfPages;
public char CoverType;
}
</script>
<title>Exercise</title>
</head>
<body>
<%
Book first = new Book();
first.Title = "Psychology and Human Evolution";
first.Author = "Jeannot Lamm";
first.YearPublished = 1996;
first.NumberOfPages = 872;
first.CoverType = 'H';
Response.Write("<pre>Book Characteristics<br />");
Response.Write("Title: " + first.Title + "<br />");
Response.Write("Author: " + first.Author + "<br />");
Response.Write("Year: " + first.YearPublished + "<br />");
Response.Write("Pages: " + first.NumberOfPages + "<br />");
Response.Write("Cover: " + first.CoverType + "</pre>");
%>
<%
Book second = new Book();
second.Title = "C# First Step";
second.Author = "Alexandra Nyango";
second.YearPublished = 2004;
second.NumberOfPages = 604;
second.CoverType = 'P';
Response.Write("<pre>Book Characteristics<br />");
Response.Write("Title: " + second.Title + "<br />");
Response.Write("Author: " + second.Author + "<br />");
Response.Write("Year: " + second.YearPublished + "<br />");
Response.Write("Pages: " + second.NumberOfPages + "<br />");
Response.Write("Cover: " + second.CoverType + "</pre>");
%>
</body>
</html>
This would produce:
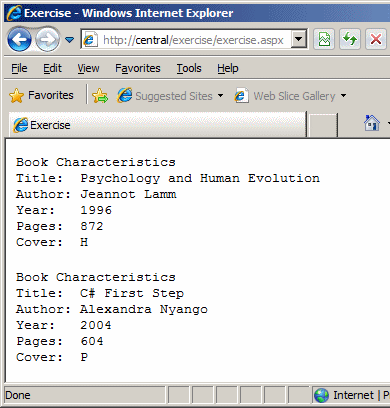
All of the member variables and methods of classes we have
used so far are referred to as instance members because, in order to
access them, you must have an instance of a class declared in another class in
which you want to access them.
In your application, you can declare a class member
and refer to it regardless of which instance of an object you are using. Such a
member variable is called static. To declare a member variable of a class as
static, type the static keyword on its left. Here is
an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
public class Book
{
public static string Title;
public static string Author;
public short YearPublished;
public int NumberOfPages;
public char CoverType;
}
</script>
<title>Exercise</title>
</head>
<body>
<%
Book first = new Book();
Book.Title = "Psychology and Human Evolution";
Book.Author = "Jeannot Lamm";
first.YearPublished = 1996;
first.NumberOfPages = 872;
first.CoverType = 'H';
Response.Write("<pre>Book Characteristics<br />");
Response.Write("Title: " + Book.Title + "<br />");
Response.Write("Author: " + Book.Author + "<br />");
Response.Write("Year: " + first.YearPublished + "<br />");
Response.Write("Pages: " + first.NumberOfPages + "<br />");
Response.Write("Cover: " + first.CoverType + "</pre>");
%>
<%
Book second = new Book();
Book.Title = "C# First Step";
Book.Author = "Alexandra Nyango";
second.YearPublished = 2004;
second.NumberOfPages = 604;
second.CoverType = 'P';
Response.Write("<pre>Book Characteristics<br />");
Response.Write("Title: " + Book.Title + "<br />");
Response.Write("Author: " + Book.Author + "<br />");
Response.Write("Year: " + second.YearPublished + "<br />");
Response.Write("Pages: " + second.NumberOfPages + "<br />");
Response.Write("Cover: " + second.CoverType + "</pre>");
%>
</body>
</html>
Notice that when a member variable has been declared as
static, you don't need an instance of the class to access that member variable
outside of the class. Based on this, if you declare all members of a class as
static, you don't need to declare a variable of their class in order to access
them.
|