An enumeration is a series of constant integers that each has
a specific position in the list and can be recognized by a meaningful name.
To create an enumeration, you use the enum
keyword, followed by the name of the enumeration, followed by a name for each
item of the list. The name of the enumeration and the name of each item of the
list follows the rules we
reviewed for names. The formula of creating an enumeration is:
enum Series_Name {Item1, Item2, Item_n};
Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
enum HouseType { Unknown, SingleFamily, TownHouse, Condominium }
</script>
<title>Active Exercise</title>
</head>
<body>
<h3>Active Exercise</h3>
<p></p>
</body>
</html>
After creating an enumerator, each item in the list is
referred to as a member of the enumerator. To access a member of an enumeration,
type the name of the enumeration, followed by a period, followed by the desired
member. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
enum HouseType { Unknown, SingleFamily, TownHouse, Condominium }
</script>
<title>Active Exercise</title>
</head>
<body>
<h3>Active Exercise</h3>
<%
Response.Write("<pre>Member Index: ");
Response.Write(HouseType.Unknown);
Response.Write("</pre><pre>Member Index: ");
Response.Write(HouseType.SingleFamily);
Response.Write("</pre><pre>Member Index: ");
Response.Write(HouseType.TownHouse);
Response.Write("</pre><pre>Member Index: ");
Response.Write(HouseType.Condominium);
%>
</body>
</html>
This would produce:
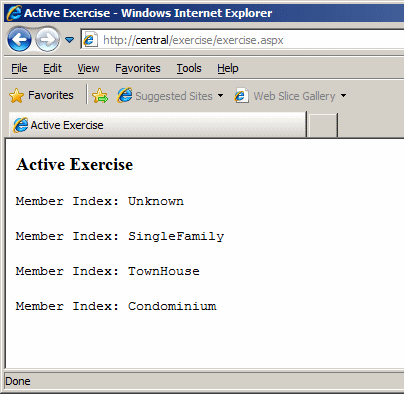
Each member is assigned a constant number. The members are
counted starting at 0, then 1, etc. By default, the first member in the
enumerationr is assigned the number 0, the second is 1, etc. This is referred to
as the index. In our HouseType enumeration,
the Unknown member has an index 0. The SingleFamily member has an index of 1.
The TownHouse member has an index of 2.
You can specify or change the numbers to your liking when
you create the enumeration but once the enumeration has been created, whether
you specified these numbers or not, they cannot be changed.
To make the list start at a specific number, assign the
starting value to the first item in the list. Here is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
enum HouseType { Unknown = 5, SingleFamily, TownHouse, Condominium }
</script>
<title>Active Exercise</title>
</head>
<body>
<h3>Active Exercise</h3>
<%
Response.Write("<pre>Member Index: ");
Response.Write(HouseType.Unknown);
Response.Write("</pre><pre>Member Index: ");
Response.Write(HouseType.SingleFamily);
Response.Write("</pre><pre>Member Index: ");
Response.Write(HouseType.TownHouse);
Response.Write("</pre><pre>Member Index: ");
Response.Write(HouseType.Condominium);
%>
</body>
</html>
Enumerations are regularly used when performing comparisons.