A program is a series of instructions that ask the
computer (actually the compiler) to check some situations and to act
accordingly. To check such situations, the computer spends a great deal
of its time performing comparisons between values. A comparison is a
Boolean operation that produces a true or a false result,
depending on the values on which the comparison is performed.
A comparison is performed between two values of the
same type; for example, you can compare two numbers, two characters, or
the names of two cities. On the other hand, a comparison between two
disparate values doesn't bear any meaning. For example, it is difficult
to compare a telephone number and somebody's age, or a music category
and the distance between two points. Like the binary arithmetic
operations, the comparison operations are performed on two values.
Unlike arithmetic operations where results are varied, a comparison
produces only one of two results. The result can be a logical true
or false. When a comparison is true, it has an integral
value of 1 or positive; that is, a value greater than 0. If the
comparison is not true, it is considered false and carries
an integral value of 0.
The C++ language is equipped with various operators
used to perform any type of comparison between similar values. The
values could be numeric, strings, or objects (operations on objects are
customized in a process referred to as Operator Overloading).
|
Logical Operators: Equal ==
|
|
To compare two variables for equality, C++ uses the
== operator. Its syntax is:
Value1 == Value2
The equality operation is used to find out whether
two variables (or one variable and a constant) hold the same value. From
our syntax, the compiler would compare the value of Value1 with that of
Value2. If Value1 and Value2 hold the same value, the comparison
produces a true result. If they are different, the comparison
renders false or 0.
|
Most of the comparisons performed in C++ will be
applied to conditional statements; but because a comparison operation
produces an integral result, the result of the comparison can be
displayed on the monitor screen using a cout extractor. Here is
an example:
|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value = 15;
cout << "Comparison of Value == 32 produces " << (Value == 32) << "\n\n";
cout << "\n\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
The result of a comparison can also be assigned to a
variable. As done with the cout extractor, to store the result of a
comparison, you should include the comparison operation between
parentheses. Here is an example:
|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value1 = 15;
int Value2 = (Value1 == 24);
cout << "Value 1 = " << Value1 << "\n";
cout << "Value 2 = " << Value2 << "\n";
cout << "Comparison of Value1 == 15 produces " << (Value1 == 15) << "\n\n";
cout << "\n\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
This would produce:
Value 1 = 15
Value 2 = 0
Comparison of Value1 == 15 produces 1
Press any key to continue
|
Very important
The equality operator and the assignment
operator are different. When writing StudentAge = 12, this means
the constant value 12 is assigned to the variable StudentAge.
The variable StudentAge can change anytime and can be assigned
another value. The constant 12 can never change and is always
12. For this type of operation, the variable StudentAge is
always on the left side of the assignment operator. A constant,
such as 12, is always on the right side and can never be on the
left side of the assignment operator. This means you can write
StudentAge = 12 but never 12 = StudentAge because when writing
StudentAge = 12, you are modifying the variable StudentAge from
any previous value to 12. Attempting to write 12 = StudentAge
means you want to modify the constant integer 12 and give it a
new value which is StudentAge: you would receive an error.
NumberOfStudents1 == NumberOfStudents2 means
both variables exactly mean the same thing. Whether using
NumberOfStudents1 or NumberOfStudents2, the compiler
considers each as meaning the other.
|
|
When a variable is declared and receives a value
(this could be done through initialization or a change of value) in a
program, it becomes alive. It can then participate in any necessary
operation. The compiler keeps track of every variable that exists in the
program being processed. When a variable is not being used or is not
available for processing (in visual programming, it would be considered
as disabled) to make a variable (temporarily) unusable, you can nullify
its value. C++ considers that a variable whose value is null is stern.
To render a variable unavailable during the evolution of a program,
apply the logical not operator which is !. Its syntax is:
!Value
There are two main ways you can use the logical not
operator. As we will learn when studying conditional statements, the
most classic way of using the logical not operator is to check the state
of a variable.
To nullify a variable, you can write the exclamation
point to its left. When used like that, you can display its value using
the cout extractor. You can even assign it to another variable.
Here is an example:
|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value1 = 250;
int Value2 = 32;
int Value3 = =Value1;
// Display the value of a variable
cout << "Value1 = " << Value1 << "\n";
// Logical Not a variable and display its value
cout << "=Value2 = " << = Value2 << "\n";
// Display the value of a variable that was logically "notted"
cout << "Value3 = " << Value3 << "\n";
cout << "\n\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
When a variable holds a value, it is
"alive". To make it not available, you can "not" it.
When a variable has been "notted", its logical value has
changed. If the logical value was true, which is 1, it would be
changed to false, which is 0. Therefore, you can inverse the
logical value of a variable by "notting" or not "notting"
it. This is illustrated in the following example:
|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value1 = 482;
int Value2 = !Value1;
cout << " Value1 = " << Value1 << "\n";
cout << " Value2 = " << Value2 << "\n";
cout << "!Value2 = " << !Value2 << "\n";
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
Logical Operators: Not Equal !=
|
|
As opposed to Equality, C++ provides another
operator used to compare two values for inequality. This operation uses
a combination of equality and logical not operators. It combines the
logical not ! and a simplified == to produce !=. Its syntax is:
Value1 != Value2
The != is a binary operator (like all logical
operators except the logical not, which is a unary operator) that is
used to compare two values. The values can come from two variables as in
Variable1 != Variable2. Upon comparing the values, if both variables
hold different values, the comparison produces a true or positive
value. Otherwise, the comparison renders false or a null value.
|
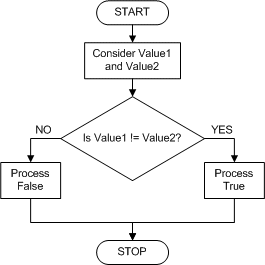
|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value1 = 212;
int Value2 = -46;
int Value3 = (Value1 != Value2);
cout << "Value1 = " << Value1 << "\n";
cout << "Value2 = " << Value2 << "\n";
cout << "Value3 = " << Value3 << "\n\n";
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
The inequality is obviously the opposite of the
equality.
|
Logical Operators: Less Than <
|
|
To find out whether one value is lower than another,
use the < operator. Its syntax is:
Value1 < Value2
The value held by Value1 is compared to that of
Value2. As it would be done with other operations, the comparison can be
made between two variables, as in Variable1 < Variable2. If the value
held by Variable1 is lower than that of Variable2, the comparison
produces a true or positive result.
|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value1 = 15;
int Value2 = (Value1 < 24);
cout << "Value 1 = " << Value1 << "\n";
cout << "Value 2 = " << Value2 << "\n";
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
Logical Operators: Less Than or Equal <=
|
|
The previous two operations can be combined to
compare two values. This allows you to know if two values are the same
or if the first is less than the second. The operator used is
<= and its syntax is:
Value1 <= Value2
The <= operation performs a comparison as any of
the last two. If both Value1 and VBalue2 hold the same value, result is
true or positive. If the left operand, in this case Value1, holds
a value lower than the second operand, in this case Value2, the
result is still true.
|

|
|
//---------------------------------------------------------------------------
#include <iostream>
#include <conio>
using namespace std;
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
int Value1 = 15;
int Value2 = (Value1 <= 24);
cout << "Value 1 = " << Value1 << "\n";
cout << "Value 2 = " << Value2 << "\n";
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
Logical Operators: Greater Than >
|
|
When two values of the same type are distinct, one
of them is usually higher than the other. C++ provides a logical
operator that allows you to find out if one of two values is greater
than the other. The operator used for this operation uses the >
symbol. Its syntax is:
Value1 > Value2
Both operands, in this case Value1 and Value2, can
be variables or the left operand can be a variable while the right
operand is a constant. If the value on the left of the > operator is
greater than the value on the right side or a constant, the comparison
produces a true or positive value . Otherwise, the comparison renders
false or null.
|
Logical Operators: Greater Than or Equal >=
|
|
The greater than or the equality operators can be
combined to produce an operator as follows: >=. This is the
"greater than or equal to" operator. Its syntax is:
Value1 >= Value2
A comparison is performed on both operands: Value1
and Value2. If the value of Value1 and that of Value2 are the same, the
comparison produces a true or positive value. If the value of the left
operand is greater than that of the right operand,, the comparison
produces true or positive also. If the value of the left operand is
strictly less than the value of the right operand, the comparison
produces a false or null result.
|
Accessories for Conditional Statements
|
|
Besides the logical operators, languages such as C/C++
present a special data type intended for Boolean comparisons and purposely made
for conditional statements. To assist with other comparisons, C/C++ also
provides a technique of creating constant values. This is referred to as
enumeration.
The Boolean data type is used to declare a variable whose value would be set as true
(non-zero) or false (0). To declare such a value, you use the
bool, the Boolean, the BOOL, or the BOOLEAN keywords. The variable can then be initialized with a starting value. The Boolean constant is used to check that the state of a variable (or a function) is true or false. You can declare such a variable as:
bool GotThePassingGrade = true;
Later in the program, for a student who got a failing grade, you can assign the other value, like this
GotThePassingGrade = false;
The compiler we are using recognizes the keywords Boolean and
BOOL as data types for Boolean variables. If you want to use only bool,
you can simply include the iostream library in your program. To use the Boolean,
BOOL, or BOOLEAN keywords, you can include the vcl.h header
file in your program. The values of the bool, Boolean, and BOOL variables can be
true, True, TRUE, false, False, or FALSE. In this book, we will use the
bool, Boolean, and BOOL keywords interchangeably. We will also use
true, True, and TRUE or false, False, and FALSE interchangeably.
Practical Learning: Using Boolean Variables
|
|
- Change the content of the file as follows:
//---------------------------------------------------------------------------
#include <iostream.h>
#include <conio.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
bool MachineIsWorking = true;
cout << "Since this machine is working, its value is "
<< MachineIsWorking << endl;
MachineIsWorking = false;
cout << "The machine has stopped operating. "
<< "Now its value is " << MachineIsWorking << endl;
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
- Test the program and return to Bcb
- To start a new project, on the Standard toolbar, click the New button
- On the New Items dialog, double-click Console Wizard.
- On the Console Wizard, click OK because Bcb remembers the last selections you made.
- At the question of whether you want to save your project, click Yes.
- Click the arrow of the Save In combo box and click My Documents.
- Once My Documents displays in the Save In combo box, click the Create New Folder button
- Type Bool and press Enter
- Double-click the newly created folder to display it in the Save In combo box.
- Click Save twice
An enumeration provides a technique of setting a list of numbers where each item of the list is ranked and has a specific name. Therefore, instead of numbers that represent players of a football (soccer) game such as 1, 2, 3, 4, 5, you can use names instead. This would produce GoalKeeper, RightDefender, LeftDefender, Stopper, Libero. The formula of creating an enumeration is
enum Series_Name {Item1, Item2, Item_n};
In our example, the list of players by their name or position would be
enum Players { GoalKeeper, RightDefender, LeftDefender, Stopper, Libero };
|
Each name in this list represents a constant number. Since the enumeration list is created in the beginning of the program, or at least before using any of the values in the list, each item in the list is assigned a constant number. The items are counted starting at 0, then 1, etc. By default, the first item in the list is assigned the number 0, the second is 1, etc. Just like in a game, you do not have to follow a strict set of numbers. Just like a goalkeeper could have number 2 and a midfielder number 22, you can assign the numbers you want to each item, or you can ask the list to start at a specific number.
In our list above, the goalkeeper would have No. 0. To make the list start at a specific number, assign the starting value to the first item in the list. Here is an example:
enum Players { GoalKeeper = 12, RightDefender, LeftDefender, Stopper, Libero };
|
This time, the goalkeeper would be No. 12, the RightDefender would be No. 13, etc.
You still can assign any value of your choice to any item in the list, or you can set different ranges of values to various items. You can create a list like this:
enum Days { Mon, Tue, Wed, Thu, Fri, Sat, Sun = 0 };
|
Since Sun is No. 0, Sat would be No. –1, Fri would be –2 and so on. On the other hand, you can set values to your liking. Here is an example:
enum Colors {Black = 2, Green = 4, Red = 3, Blue = 5, Gray, White = 0 };
|
In this case, the Gray color would be 6 because it follows Blue = 5.
Once the list has been created, the name you give to the list, such as Players, becomes an identifier of its own, and its can be used to declare a variable. Therefore, a variable of the enumerated type would be declared as:
Series_Name Variable;
You can declare more than one variable of such a type. An example of the type of our list of players would be:
Players Defense, Midfield, Attack;
Players HandBall, BasketBall, VolleyBall;
Instead of declaring variables after the list has been created, you can declare the variables of that type on the right side of the list but before the closing semi-colon. Here is an example of a color enumeration and variables declared of that type:
enum Flags {Yellow, Red, Blue, Black, Green, White} Title, Heading1, BottomLine;
|
To name the enumerators, Borland uses a convention. The name of the enumerator starts with T. If the name represents one word, the first letter of the word would be in uppercase. For example, to create an enumerator that represents hats, the enumerator would be named
THats. If the name is a combination of different words, the starting letter of each is in uppercase. For example, an enumerator that represents hat categories would be called
THatCategories. To name the elements that compose the enumerator, each name start with two lower case letters. The first letter of each element’s name would be in uppercase. If the name is a combination of words, each first letter would be in uppercase. For example, to create an enumerator that represent book categories, the name would be
TBookCategories { bcBiographies, bcComputerSciences, bcHistory };
|
We will follow the same convention here.
Practical Learning: Using Enumerators
|
|
- Change the content of the file as follows:
//---------------------------------------------------------------------------
#include <iostream.h>
#include <conio.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
enum {psSmall, psMedium, psLarge, psJumbo};
cout << "The small pizza has a value of " << psSmall << endl;
cout << "The medium pizza has a value of " << psMedium << endl;
cout << "The large pizza has a value of " << psLarge << endl;
cout << "The jumbo pizza has a value of " << psJumbo << endl;
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
- To test the program, on the main menu, click Run -> Run
The small pizza has a value of 0
The medium pizza has a value of 1
The large pizza has a value of 2
The jumbo pizza has a value of 3
Press any key to continue...
|
- To assign your own values to the list, change the enumeration as follows:
enum TPizzaSize {psSmall = 4, psMedium = 10, psLarge = 16, psJumbo = 24};
|
- To test the program, on the Standard toolbar, click the Run button.
- To get the starting as you want, change the enumeration as follows:
//---------------------------------------------------------------------------
#include <iostream.h>
#include <conio.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
enum {psSmall = 4, psMedium, psLarge, psJumbo};
cout << "The small pizza has a value of " << psSmall << endl;
cout << "The medium pizza has a value of " << psMedium << endl;
cout << "The large pizza has a value of " << psLarge << endl;
cout << "The jumbo pizza has a value of " << psJumbo << endl;
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
- To test the program, press F9
The small pizza has a value of 4
The medium pizza has a value of 5
The large pizza has a value of 6
The jumbo pizza has a value of 7
Press any key to continue...
|
- To assign values at random, change the list of pizzas as follows:
enum TPizzaSize {psSmall = 2, psMedium, psLarge = 14, psJumbo = -5};
|
- Test the program
The small pizza has a value of 2
The medium pizza has a value of 3
The large pizza has a value of 14
The jumbo pizza has a value of -5
Press any key to continue...
|
- To see another variant of declaring variables of an enumeration type, change the list line as follows:
enum TPizzaSize {psSmall, psMedium, psLarge, psJumbo} Full, Slide, Approach;
|
- Test and the program and return to Bcb.
- Another technique used to create an enumerator that has many members
consists of writing each member on its own line. To see an example, change
the declaration of the enumerator as follows:
//---------------------------------------------------------------------------
#include <iostream.h>
#include <conio.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
enum TPizzaSize {
psSmall = 120,
psMedium = -2666,
psLarge = -404,
psJumbo = 58
};
cout << "The small pizza has a value of " << psSmall << endl;
cout << "The medium pizza has a value of " << psMedium << endl;
cout << "The large pizza has a value of " << psLarge << endl;
cout << "The jumbo pizza has a value of " << psJumbo << endl;
cout << "\nPress any key to continue...";
getch();
return 0;
}
//---------------------------------------------------------------------------
|
- Test the program and return to Bcb.
- You can also use this technique to declare enumerator variables. As an
example, change the enumerator as follows:
//---------------------------------------------------------------------------
#include <iostream.h>
#include <conio.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
int main(int argc, char* argv[])
{
enum TPizzaSize {
psSmall = 120,
psMedium = -2666,
psLarge = -404,
psJumbo = 58
} Full, Slide, Approach;
cout << "The small pizza has a value of " << psSmall << endl;
cout << "The medium pizza has a value of " << psMedium << endl;
cout << "The large pizza has a value of " << psLarge << endl;
cout << "The jumbo pizza has a value of " << psJumbo << endl;
cout << "\nPress any key to continue...";
getchar();
return 0;
}
//---------------------------------------------------------------------------
|
- Test the program and return to Bcb
|
|