Inheritance consists of creating a class whose primary
definition or behavior is based on another class. In other words, inheritance
starts by having a class that can provide behavior that other classes can
improve on.
Practical Learning: Introducing Inheritance
|
|
- Start Microsoft Visual C# and create a new
Console Application named RealEstate4
- To create a new class, in the Class View, right-click the name of
the project, position the mouse on Add and click Class...
- Set the Name to Property and press Enter
- Change the file as follows:
using System;
namespace RealEstate4
{
public enum PropertyCondition
{
Unknown,
Excellent,
Good,
NeedsRepair,
BadShape
}
public class Property
{
private string propNbr;
private PropertyCondition cond;
private int beds;
private float baths;
private int yr;
private decimal val;
public Property()
{
}
public string PropertyNumber
{
get { return propNbr; }
set
{
if (propNbr == "")
propNbr = "N/A";
else
propNbr = value;
}
}
public PropertyCondition Condition
{
get { return cond; }
set { cond = value; }
}
public int Bedrooms
{
get
{
if (beds <= 1)
return 1;
else
return beds;
}
set { beds = value; }
}
public float Bathrooms
{
get { return baths; }
set { baths = value; }
}
public int YearBuilt
{
get { return yr; }
set { yr = value; }
}
public decimal Value
{
get { return val; }
set { val = value; }
}
}
}
|
- To create a new class, in the Solution Explorer, right-click the name of
the project, position the mouse on Add and click Class...
- Set the Name to PropertyListing and press Enter
- Change the file as follows:
using System;
namespace RealEstate4
{
public enum PropertyType
{
Unknown,
SingleFamily,
Townhouse,
Condominium
}
class PropertyListing
{
private Property prop;
private PropertyType tp;
public Property ListProperty
{
get { return prop; }
set { prop = value; }
}
public PropertyType Type
{
get { return tp; }
set { tp = value; }
}
public PropertyListing()
{
prop = new Property();
}
public void CreateListing()
{
Console.WriteLine(" =//= Altair Realty =//=");
Console.WriteLine("-=- Property Creation -=-");
Console.WriteLine("\nTypes of Properties");
Console.WriteLine("1. Single Family");
Console.WriteLine("2. Townhouse");
Console.WriteLine("3. Condonium");
Console.WriteLine("4. Don't Know");
Console.Write("Enter Type of Property: ");
int propType = int.Parse(Console.ReadLine());
Console.Write("\nEnter Property #: ");
ListProperty.PropertyNumber = Console.ReadLine();
Console.WriteLine("\nProperties Conditions");
Console.WriteLine("1. Excellent");
Console.WriteLine("2. Good (may need minor repair)");
Console.WriteLine("3. Needs Repair");
Console.Write("4. In Bad Shape (property needs ");
Console.WriteLine("major repair or rebuild)");
Console.Write("Enter Property Condition: ");
int condition = int.Parse(Console.ReadLine());
if (condition == 1)
ListProperty.Condition = PropertyCondition.Excellent;
else if (condition == 2)
ListProperty.Condition = PropertyCondition.Good;
else if (condition == 3)
ListProperty.Condition = PropertyCondition.NeedsRepair;
else if (condition == 4)
ListProperty.Condition = PropertyCondition.BadShape;
else
ListProperty.Condition = PropertyCondition.Unknown;
switch ((PropertyType)propType)
{
case PropertyType.SingleFamily:
Type = PropertyType.SingleFamily;
break;
case PropertyType.Townhouse:
Type = PropertyType.Townhouse;
break;
case PropertyType.Condominium:
Type = PropertyType.Condominium;
break;
default:
Type = PropertyType.Unknown;
break;
}
Console.Write("\nHow many bedrooms? ");
ListProperty.Bedrooms = int.Parse(Console.ReadLine());
Console.Write("How many bathrooms? ");
ListProperty.Bathrooms = float.Parse(Console.ReadLine());
Console.Write("Year built: ");
ListProperty.YearBuilt = int.Parse(Console.ReadLine());
Console.Write("Property Value: ");
ListProperty.Value = decimal.Parse(Console.ReadLine());
}
public void ShowProperty()
{
Console.WriteLine("==================================");
Console.WriteLine(" =//=//= Altair Realty =//=//=");
Console.WriteLine("-=-=-=- Properties Listing -=-=-=-");
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
ListProperty.PropertyNumber);
Console.WriteLine("Property Type: {0}", Type);
switch (Type)
{
case PropertyType.SingleFamily:
case PropertyType.Townhouse:
Type = PropertyType.SingleFamily;
break;
case PropertyType.Condominium:
break;
}
Console.WriteLine("Condition: {0}",
ListProperty.Condition);
Console.WriteLine("Bedrooms: {0}",
ListProperty.Bedrooms);
Console.WriteLine("Bathrooms: {0}",
ListProperty.Bathrooms);
Console.WriteLine("Year Built: {0}",
ListProperty.YearBuilt);
Console.WriteLine("Market Value: {0:C}",
ListProperty.Value);
Console.WriteLine("==================================");
}
}
}
|
- Access the Program.cs file and change it as follows:
using System;
namespace RealEstate4
{
class Program
{
static void Main()
{
PropertyListing listing = new PropertyListing();
listing.CreateListing();
Console.WriteLine("\n");
listing.ShowProperty();
Console.WriteLine();
}
}
}
|
- Execute the application and test it. Here is an example:
=//= Altair Realty =//=
-=- Property Creation -=-
Types of Properties
1. Single Family
2. Townhouse
3. Condominium
4. Don't Know
Enter Type of Property: 2
Enter Property #: 66DG8P
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 2
How many bedrooms? 2
How many bathrooms? 1.0
Year built: 1994
Property Value: 325880.75
==================================
=//=//= Altair Realty =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 66DG8P
Property Type: Townhouse
Condition: Good
Bedrooms: 2
Bathrooms: 1
Year Built: 1994
Market Value: $325,880.75
==================================
Press any key to continue . . .
|
- Close the DOS window
As you may have guessed, in order to implement
inheritance, you must first have a class that provides the fundamental
definition or behavior you need. There is nothing magical about such a
class. It could appear exactly like any of the classes we have used so
far. Here is an example:
using System;
class Circle
{
private double _radius;
public double Radius
{
get
{return _radius;
}
set
{
if( _radius < 0 )
_radius = 0.00;
else
_radius = value;
}
}
public double Diameter
{
get
{
return Radius * 2;
}
}
public double Circumference
{
get
{
return Diameter * 3.14159;
}
}
public double Area
{
get
{
return Radius * Radius * 3.14159;
}
}
}
|
 |
class Exercise
{
public static int Main()
{
Circle c = new Circle();
c.Radius = 25.55;
Console.WriteLine("Circle Characteristics");
Console.WriteLine("Side: {0}", c.Radius);
Console.WriteLine("Diameter: {0}", c.Diameter);
Console.WriteLine("Circumference: {0}", c.Circumference);
Console.WriteLine("Area: {0}", c.Area);
return 0;
}
}
This would produce:
Circle Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 2050.837805975
Press any key to continue
The above class is used to process a circle. It can
request or provide a radius, then it calculates the circumference and
the area. Now, suppose you want to create a class for a
sphere. You could start from scratch as we have done so far. On the other
hand, since a sphere is primarily a 3-dimensional circle, and if you have
a class for a circle already, you can simply create your sphere class that
uses the already implemented behavior of a circle class.
Creating a class that is based on another class is
also referred to as deriving a class from another. The first class serves
as parent or base. The class that is based on another class is also
referred to as child or derived. To create a class based on another, you
use the following formula:
class NewChild : BaseClass
{
// Body of the new class
}
In this formula, you start with the class keyword
followed by a name for your class. On the right side of the name of your
class, you must type a colon :, followed by the name of the class
that will serve as parent. Of course, the BaseClass class must have been
defined; that is, the compiler must be able to find its definition.
Based
on the above formula, you can create a sphere class based on the earlier
mentioned Circle class as follows: class Sphere : Circle
{
// The class is ready
}
After deriving a class, it becomes available and you
can use it just as you would any other class. Here is an example:
using System;
class Circle
{
private double _radius;
public double Radius
{
get
{
if( _radius < 0 )
return 0.00;
else
return _radius;
}
set
{
_radius = value;
}
}
public double Diameter
{
get
{
return Radius * 2;
}
}
public double Circumference
{
get
{
return Diameter * 3.14159;
}
}
public double Area
{
get
{
return Radius * Radius * 3.14159;
}
}
}
class Sphere : Circle
{
}
class Exercise
{
public static int Main()
{
Circle c = new Circle();
c.Radius = 25.55;
Console.WriteLine("Circle Characteristics");
Console.WriteLine("Side: {0}", c.Radius);
Console.WriteLine("Diameter: {0}", c.Diameter);
Console.WriteLine("Circumference: {0}", c.Circumference);
Console.WriteLine("Area: {0}", c.Area);
Sphere s = new Sphere();
s.Radius = 25.55;
Console.WriteLine("\nSphere Characteristics");
Console.WriteLine("Side: {0}", s.Radius);
Console.WriteLine("Diameter: {0}", s.Diameter);
Console.WriteLine("Circumference: {0}", s.Circumference);
Console.WriteLine("Area: {0}", s.Area);
return 0;
}
}
This would produce:
Circle Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 2050.837805975
Sphere Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 2050.837805975
Press any key to continue
When a class is based on another class, all public (we
will also introduce another inheritance-oriented keyword for this issue)
members of the parent class are made
available to the derived class that can use them as easily. While other
methods and classes can also use the public members of a class, the
difference is that the derived class can call the public members of the
parent as if they belonged to the derived class. That is, the child class
doesn't have to "qualify" the public members of the parent class
when these public members are used in the body of the derived class. This
is illustrated in the following program:
using System;
class Circle
{
private double _radius;
public double Radius
{
get
{
if( _radius < 0 )
return 0.00;
else
return _radius;
}
set
{
_radius = value;
}
}
public double Diameter
{
get
{
return Radius * 2;
}
}
public double Circumference
{
get
{
return Diameter * 3.14159;
}
}
public double Area
{
get
{
return Radius * Radius * 3.14159;
}
}
public void ShowCharacteristics()
{
Console.WriteLine("Circle Characteristics");
Console.WriteLine("Side: {0}", Radius);
Console.WriteLine("Diameter: {0}", Diameter);
Console.WriteLine("Circumference: {0}", Circumference);
Console.WriteLine("Area: {0}", Area);
}
}
class Sphere : Circle
{
public void ShowCharacteristics()
{
// Because Sphere is based on Circle, you can access
// any public member(s) of Circle without qualifying it(them)
Console.WriteLine("\nSphere Characteristics");
Console.WriteLine("Side: {0}", Radius);
Console.WriteLine("Diameter: {0}", Diameter);
Console.WriteLine("Circumference: {0}", Circumference);
Console.WriteLine("Area: {0}\n", Area);
}
}
class Exercise
{
public static int Main()
{
Circle c = new Circle();
c.Radius = 25.55;
c.ShowCharacteristics();
Sphere s = new Sphere();
s.Radius = 25.55;
s.ShowCharacteristics();
return 0;
}
}
This would produce the same result.
Practical Learning: Inheriting |
|
- On the main menu, click Project -> Add -> Class...
- Set the Name to HouseType and press Enter
- To derive a class, change the file as follows:
using System;
namespace RealEstate4
{
class HouseType : Property
{
private int nbrOfStories;
private bool basement;
private bool garage;
public int Stories
{
get { return nbrOfStories; }
set { nbrOfStories = value; }
}
public bool FinishedBasement
{
get { return basement; }
set { basement = value; }
}
public bool IndoorGarage
{
get { return garage; }
set { garage = value; }
}
}
}
|
- On the main menu, click Project -> Add -> Class...
- Set the Name to Condominium and press Enter
- To create another class based on the Property class, change the file as follows:
using System;
namespace RealEstate4
{
class Condominium : Property
{
private bool handicap;
public bool HandicapAccessible
{
get { return handicap; }
set { handicap = value; }
}
}
}
|
- Access the PropertyListing.cs file file and change it as follows:
using System;
namespace RealEstate4
{
public enum PropertyType
{
Unknown,
SingleFamily,
Townhouse,
Condominium
}
class PropertyListing
{
private Property prop;
private HouseType hse;
private Condominium cond;
private PropertyType tp;
public Property ListProperty
{
get { return prop; }
set { prop = value; }
}
public HouseType House
{
get { return hse; }
set { hse = value; }
}
public Condominium Condo
{
get { return cond; }
set { cond = value; }
}
public PropertyType Type
{
get { return tp; }
set { tp = value; }
}
public PropertyListing()
{
prop = new Property();
hse = new HouseType();
cond = new Condominium();
}
public void CreateListing()
{
char answer;
Console.WriteLine(" =//= Altair Realty =//=");
Console.WriteLine("-=- Property Creation -=-");
Console.WriteLine("\nTypes of Properties");
Console.WriteLine("1. Single Family");
Console.WriteLine("2. Townhouse");
Console.WriteLine("3. Condonium");
Console.WriteLine("4. Don't Know");
Console.Write("Enter Type of Property: ");
int propType = int.Parse(Console.ReadLine());
Console.Write("\nEnter Property #: ");
ListProperty.PropertyNumber = Console.ReadLine();
Console.WriteLine("\nProperties Conditions");
Console.WriteLine("1. Excellent");
Console.WriteLine("2. Good (may need minor repair)");
Console.WriteLine("3. Needs Repair");
Console.Write("4. In Bad Shape (property needs ");
Console.WriteLine("major repair or rebuild)");
Console.Write("Enter Property Condition: ");
int condition = int.Parse(Console.ReadLine());
if (condition == 1)
ListProperty.Condition = PropertyCondition.Excellent;
else if (condition == 2)
ListProperty.Condition = PropertyCondition.Good;
else if (condition == 3)
ListProperty.Condition = PropertyCondition.NeedsRepair;
else if (condition == 4)
ListProperty.Condition = PropertyCondition.BadShape;
else
ListProperty.Condition = PropertyCondition.Unknown;
switch ((PropertyType)propType)
{
case PropertyType.SingleFamily:
Type = PropertyType.SingleFamily;
Console.Write("\nHow many stories (levels)? ");
House.Stories = int.Parse(Console.ReadLine());
Console.Write("Does it have an indoor car garage (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.IndoorGarage = true;
else
House.IndoorGarage = false;
Console.Write("Is the basement finished(y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.FinishedBasement = true;
else
House.FinishedBasement = false;
break;
case PropertyType.Townhouse:
Type = PropertyType.Townhouse;
Console.Write("\nHow many stories (levels)? ");
House.Stories = int.Parse(Console.ReadLine());
Console.Write("Does it have an indoor car garage (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.IndoorGarage = true;
else
House.IndoorGarage = false;
Console.Write("Is the basement finished(y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.FinishedBasement = true;
else
House.FinishedBasement = false;
break;
case PropertyType.Condominium:
Type = PropertyType.Condominium;
Console.Write("\nIs the building accessible to handicapped (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
Condo.HandicapAccessible = true;
else
Condo.HandicapAccessible = false;
break;
default:
Type = PropertyType.Unknown;
break;
}
Console.Write("\nHow many bedrooms? ");
ListProperty.Bedrooms = int.Parse(Console.ReadLine());
Console.Write("How many bathrooms? ");
ListProperty.Bathrooms = float.Parse(Console.ReadLine());
Console.Write("Year built: ");
ListProperty.YearBuilt = int.Parse(Console.ReadLine());
Console.Write("Property Value: ");
ListProperty.Value = decimal.Parse(Console.ReadLine());
}
public void ShowProperty()
{
Console.WriteLine("==================================");
Console.WriteLine(" =//=//= Altair Realty =//=//=");
Console.WriteLine("-=-=-=- Properties Listing -=-=-=-");
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
ListProperty.PropertyNumber);
Console.WriteLine("Property Type: {0}", Type);
switch(Type)
{
case PropertyType.SingleFamily:
case PropertyType.Townhouse:
Type = PropertyType.SingleFamily;
Console.WriteLine("Stories: {0}",
House.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
House.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
House.FinishedBasement);
break;
case PropertyType.Condominium:
Console.WriteLine("Handicapped Accessible Building: {0}",
Condo.HandicapAccessible);
break;
}
Console.WriteLine("Condition: {0}", ListProperty.Condition);
Console.WriteLine("Bedrooms: {0}", ListProperty.Bedrooms);
Console.WriteLine("Bathrooms: {0}", ListProperty.Bathrooms);
Console.WriteLine("Year Built: {0}", ListProperty.YearBuilt);
Console.WriteLine("Market Value: {0:C}", ListProperty.Value);
}
}
}
|
- Execute the application and test it. Here is an example:
=//= Altair Realty =//=
-=- Property Creation -=-
Types of Properties
1. Single Family
2. Townhouse
3. Condonium
4. Don't Know
Enter Type of Property: 1
Enter Property #: 4LP804
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 3
How many stories (levels)? 3
Does it have an indoor car garage (y/n): y
Is the basement finished(y/n): n
How many bedrooms? 4
How many bathrooms? 2.5
Year built: 1996
Property Value: 640885.80
==================================
=//=//= Altair Realty =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 4LP804
Property Type: SingleFamily
Stories: 3
Has Indoor Car Garage: True
Finished Basement: False
Condition: NeedsRepair
Bedrooms: 4
Bathrooms: 2.5
Year Built: 1996
Market Value: $640,885.80
Press any key to continue . . .
|
- Return to Notepad
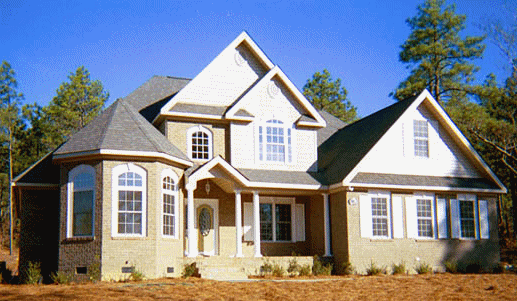
Implementation of Derived Members
|
|
You can notice in the above example that the derived
class produces the same results as the base class. In reality, inheritance
is used to solve various Object-Oriented Programming (OOP) problems. One of
them consists of customizing, adapting, or improving the behavior of a
feature (property or method, etc) of the parent class. For example,
although both the circle and the sphere have an area, their areas are not
the same. A circle is a flat surface but a sphere is a volume, which makes
its area very much higher. Since they use different formulas for
their respective areas, you should implement a new version of the area in
the sphere. Based on this, when deriving your class from another class,
you should be aware of the properties and methods of the base class so that,
if you know that the parent class has a certain behavior or a
characteristic that is not conform to the new derived class, you can do
something about that. A new version of the
area in the sphere can be calculated as follows:
using System;
class Circle
{
private double _radius;
public double Radius
{
get
{
if( _radius < 0 )
return 0.00;
else
return _radius;
}
set
{
_radius = value;
}
}
public double Diameter
{
get
{
return Radius * 2;
}
}
public double Circumference
{
get
{
return Diameter * 3.14159;
}
}
public double Area
{
get
{
return Radius * Radius * 3.14159;
}
}
}
class Sphere : Circle
{
public double Area
{
get
{
return 4 * Radius * Radius * 3.14159;
}
}
}
class Exercise
{
public static int Main()
{
Circle c = new Circle();
c.Radius = 25.55;
Console.WriteLine("Circle Characteristics");
Console.WriteLine("Side: {0}", c.Radius);
Console.WriteLine("Diameter: {0}", c.Diameter);
Console.WriteLine("Circumference: {0}", c.Circumference);
Console.WriteLine("Area: {0}", c.Area);
Sphere s = new Sphere();
s.Radius = 25.55;
Console.WriteLine("\nSphere Characteristics");
Console.WriteLine("Side: {0}", s.Radius);
Console.WriteLine("Diameter: {0}", s.Diameter);
Console.WriteLine("Circumference: {0}", s.Circumference);
Console.WriteLine("Area: {0}", s.Area);
return 0;
}
}
This would produce:
Circle Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 2050.837805975
Sphere Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 8203.3512239
Press any key to continue
Notice that, this time, the areas of both figures are
not the same even though their radii are similar.
Besides customizing member variables and methods of a
parent class, you can add new members as you wish. This is another
valuable feature of inheritance. In our example, while the circle is a flat
shape, a sphere has a volume. In this case, you may need to calculate the
volume of a sphere as a new method or property of the derived class. Here
is an example:
using System;
class Circle
{
private double _radius;
public double Radius
{
get
{
if( _radius < 0 )
return 0.00;
else
return _radius;
}
set
{
_radius = value;
}
}
public double Diameter
{
get
{
return Radius * 2;
}
}
public double Circumference
{
get
{
return Diameter * 3.14159;
}
}
public double Area
{
get
{
return Radius * Radius * 3.14159;
}
}
}
class Sphere : Circle
{
public double Area
{
get
{
return 4 * Radius * Radius * 3.14159;
}
}
public double Volume
{
get
{
return 4 * 3.14159 * Radius * Radius * Radius / 3;
}
}
}
class Exercise
{
public static int Main()
{
Circle c = new Circle();
c.Radius = 25.55;
Console.WriteLine("Circle Characteristics");
Console.WriteLine("Side: {0}", c.Radius);
Console.WriteLine("Diameter: {0}", c.Diameter);
Console.WriteLine("Circumference: {0}", c.Circumference);
Console.WriteLine("Area: {0}", c.Area);
Sphere s = new Sphere();
s.Radius = 25.55;
Console.WriteLine("\nSphere Characteristics");
Console.WriteLine("Side: {0}", s.Radius);
Console.WriteLine("Diameter: {0}", s.Diameter);
Console.WriteLine("Circumference: {0}", s.Circumference);
Console.WriteLine("Area: {0}", s.Area);
Console.WriteLine("Volume: {0}\n", s.Volume);
return 0;
}
}
This would produce:
Circle Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 2050.837805975
Sphere Characteristics
Side: 25.55
Diameter: 51.1
Circumference: 160.535249
Area: 8203.3512239
Volume: 209595.623770645
Press any key to continue
If you create a property or method in a derived class
and that property or method already exists in the parent class, when you
access the property or method in the derived class, you must make sure you
indicate what member you are accessing. To make this possible, the C#
language provides the base keyword. To access a property or method
of a parent class from the derived class, type the base keyword,
followed by the period operator, followed by the name of the property or
method of the base class.
Imagine you create a class used to process a circle as we
saw earlier. You can use this as the base class for a sphere. Both the circle and
the sphere have an area but their values are different. This means that, as
mentioned in our introduction to inheritance, when deriving the sphere class, you
would have to calculate a new area for the sphere.
If you create or declare a new member in a derived class and
that member has the same name as a member of the base class, when creating the
new member, you may want to indicate to the compiler that, instead of overriding
that same method that was defined in the base class, you want to create a brand
new and independent version of that method. When doing this, you would be asking
the compiler to hide the member of the base class that has the same name, when
the member of the derived class is invoked. To do this, type the new
keyword to its left. Here is an example:
using System;
class Circle
{
private double _radius;
public double Radius
{
get { return (_radius < 0) ? 0.00 : _radius; }
set { _radius = value; }
}
public double Diameter
{
get { return Radius * 2; }
}
public double Circumference
{
get { return Diameter * 3.14159; }
}
public double Area
{
get { return Radius * Radius * 3.14159; }
}
}
class Sphere : Circle
{
new public double Area
{
get { return 4 * Radius * Radius * 3.14159; }
}
public double Volume
{
get { return 4 * 3.14159 * Radius * Radius * Radius / 3; }
}
}
class Exercise
{
public static int Main()
{
Circle c = new Circle();
c.Radius = 25.55;
Console.WriteLine("Circle Characteristics");
Console.WriteLine("Side: {0}", c.Radius);
Console.WriteLine("Diameter: {0}", c.Diameter);
Console.WriteLine("Circumference: {0}", c.Circumference);
Console.WriteLine("Area: {0}", c.Area);
Sphere s = new Sphere();
s.Radius = 25.55;
Console.WriteLine("\nSphere Characteristics");
Console.WriteLine("Side: {0}", s.Radius);
Console.WriteLine("Diameter: {0}", s.Diameter);
Console.WriteLine("Circumference: {0}", s.Circumference);
Console.WriteLine("Area: {0}", s.Area);
Console.WriteLine("Volume: {0}\n", s.Volume);
return 0;
}
}
|
|