If you happen to have a record you don't need or don't find
necessary in a table, you can remove that record from the table. To start, you
must establish what record you want to delete. Once again, you would need a way
to uniquely identify a record. For our video collection, you can use the shelf
number column. Once you have located the undesired record, you can delete it.
To assist you with removing a record, the DataRow
class is equipped with a method named Delete. Its syntax is simply:
public void Delete();
This method must be called by the record that wants to be
deleted. Here is an example:
|
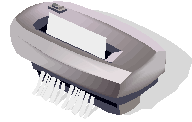 |
using System;
using System.IO;
using System.Xml;
using System.Data;
using System.Collections;
namespace VideoCollection3
{
public class VideoCollection
{
. . . No Change
public void DeleteVideo()
{
if (File.Exists(strFilename))
{
bool found = false;
string strShelfNumber = "AA-000";
dsVideos.ReadXml(strFilename);
Console.WriteLine("\nHere is the current list of videos");
Console.WriteLine("========================================");
Console.WriteLine(" Video Collection");
Console.WriteLine("=========================================");
foreach (DataRow vdo in tblVideos.Rows)
{
Console.WriteLine("Shelf #: {0}", vdo["ShelfNumber"]);
Console.WriteLine("Title: {0}", vdo["Title"]);
Console.WriteLine("Director: {0}", vdo["Director"]);
Console.WriteLine("(c) Year: {0}", vdo["Year"]);
Console.WriteLine("Length: {0:C}", vdo["Length"]);
Console.WriteLine("Rating: {0}", vdo["Rating"]);
Console.WriteLine("-----------------------------------------");
}
Console.Write("Enter the shelf number of the video you want to delete: ");
strShelfNumber = Console.ReadLine();
foreach (DataRow vdo in tblVideos.Rows)
{
string str = (string)vdo["ShelfNumber"];
if (str == strShelfNumber)
{
found = true;
Console.WriteLine("\n-----------------------------------------");
Console.WriteLine("Here is the video you want to delete");
Console.WriteLine("1. Title: {0}", vdo["Title"]);
Console.WriteLine("2. Director: {0}", vdo["Director"]);
Console.WriteLine("3. (c) Year: {0}", vdo["Year"]);
Console.WriteLine("4. Length: {0}", vdo["Length"]);
Console.WriteLine("5. Rating: {0}", vdo["Rating"]);
Console.WriteLine("-----------------------------------------");
Console.Write("Do you still want to delete this video (y/n)? ");
char ans = char.Parse(Console.ReadLine());
if ((ans == 'y') || (ans == 'Y'))
{
vdo.Delete();
dsVideos.WriteXml(strFilename);
Console.WriteLine("The video has been deleted!");
}
return;
}
}
if (found == false)
{
Console.WriteLine("No video with that shelf number was found");
return;
}
}
}
}
}
Removing a Row From the Collection of Records |
|
Besides the DataRow class, the DataRowCollection
class provides its own means of deleting a record from a table. To delete a record, you can call the
DataRowCollection.Remove() method. Its syntax is:
public void Remove(DataRow row);
This method takes as argument a DataRow object and
checks whether the table contains it. If that record exists, it gets deleted,
including all of its entries for each column. Here is an example:
using System;
using System.IO;
using System.Xml;
using System.Data;
using System.Collections;
namespace VideoCollection3
{
public class VideoCollection
{
. . . No Change
public void DeleteVideo()
{
if (File.Exists(strFilename))
{
bool found = false;
string strShelfNumber = "AA-000";
dsVideos.ReadXml(strFilename);
Console.WriteLine("\nHere is the current list of videos");
Console.WriteLine("========================================");
Console.WriteLine(" Video Collection");
Console.WriteLine("=========================================");
foreach (DataRow vdo in tblVideos.Rows)
{
Console.WriteLine("Shelf #: {0}", vdo["ShelfNumber"]);
Console.WriteLine("Title: {0}", vdo["Title"]);
Console.WriteLine("Director: {0}", vdo["Director"]);
Console.WriteLine("(c) Year: {0}", vdo["Year"]);
Console.WriteLine("Length: {0:C}", vdo["Length"]);
Console.WriteLine("Rating: {0}", vdo["Rating"]);
Console.WriteLine("-----------------------------------------");
}
Console.Write("Enter the shelf number of the video you want to delete: ");
strShelfNumber = Console.ReadLine();
foreach (DataRow vdo in tblVideos.Rows)
{
string str = (string)vdo["ShelfNumber"];
if (str == strShelfNumber)
{
found = true;
Console.WriteLine("\n-----------------------------------------");
Console.WriteLine("Here is the video you want to delete");
Console.WriteLine("1. Title: {0}", vdo["Title"]);
Console.WriteLine("2. Director: {0}", vdo["Director"]);
Console.WriteLine("3. (c) Year: {0}", vdo["Year"]);
Console.WriteLine("4. Length: {0}", vdo["Length"]);
Console.WriteLine("5. Rating: {0}", vdo["Rating"]);
Console.WriteLine("-----------------------------------------");
Console.Write("Do you still want to delete this video (y/n)? ");
char ans = char.Parse(Console.ReadLine());
if ((ans == 'y') || (ans == 'Y'))
{
tblVideos.Rows.Remove(vdo);
dsVideos.WriteXml(strFilename);
Console.WriteLine("The video has been deleted!");
}
return;
}
}
if (found == false)
{
Console.WriteLine("No video with that shelf number was found");
return;
}
}
}
}
}
Deleting a Record by its Index |
|
When calling the DataRowCollection.Remove() method, you must
pass an exact identification of the record. If you don't have that
identification, you can delete a record based on its index. To do this, you
would call the DataRowCollection.RemoveAt() method. Its syntax is:
public void RemoveAt(int index);
This method takes as argument the index of the record you
want to delete. If a record with that index exists, it would be deleted.
Deleting all Records of a Table |
|
To delete all records of a table, call the DataRowCollection.Clear()
method. Its syntax is:
public void Clear();
This method is used to clear the table of all records.
|
|