Practical
Learning: Creating an Enumeration Property |
|
- Access the ShoppingStore.cs file and change it as follows:
using System;
namespace DepartmentStore2
{
public enum ItemCategory
{
Unspecified,
Women,
Men,
Girls,
Boys,
Babies
}
class ShoppingItem
{
private long itemNo;
private ItemCategory cat;
private string nm;
private string sz;
private decimal price;
// A property for the stock number of an item
public long ItemNumber
{
get
{
return itemNo;
}
set
{
if (itemNo <= 0)
itemNo = 0;
else
itemNo = value;
}
}
// A property for the category of item
public ItemCategory Category
{
get
{
return cat;
}
set
{
cat = value;
}
}
// A property for the name of an item
public string Name
{
get
{
return nm;
}
set
{
if (nm == "")
nm = "Item no Description";
else
nm = value;
}
}
// A property for size of a merchandise
public string Size
{
get
{
if( sz == "0" )
return "Unknown Size or Fits All";
else
return sz;
}
set
{
sz = value;
}
}
// A property for the marked price of an item
public decimal UnitPrice
{
get
{
return price;
}
set
{
if (price < 0)
price = 0.00M;
else
price = value;
}
}
public static ShoppingItem Read()
{
int category = 0;
ShoppingItem shop = new ShoppingItem();
Console.Write("Item #: ");
shop.itemNo = long.Parse(Console.ReadLine());
Console.WriteLine("Store Items Categories");
Console.WriteLine("\t1. Women");
Console.WriteLine("\t2. Men");
Console.WriteLine("\t3. Girls");
Console.WriteLine("\t4. Boys");
Console.WriteLine("\t5. Babies");
Console.Write("Enter the Category: ");
category = int.Parse(Console.ReadLine());
if (category == 1)
shop.Category = ItemCategory.Women;
else if (category == 2)
shop.Category = ItemCategory.Men;
else if (category == 3)
shop.Category = ItemCategory.Girls;
else if (category == 4)
shop.Category = ItemCategory.Boys;
else if (category == 5)
shop.Category = ItemCategory.Babies;
else
shop.Category = ItemCategory.Unspecified;
Console.Write("Item Name: ");
shop.Name = Console.ReadLine();
Console.Write("Item Size (Enter 0 if unknown): ");
shop.Size = Console.ReadLine();
Console.Write("Unit Price: ");
shop.UnitPrice = decimal.Parse(Console.ReadLine());
return shop;
}
public static void Write(ShoppingItem item)
{
Console.WriteLine("Item #: {0}", item.ItemNumber);
Console.WriteLine("Category: {0}", item.Category);
Console.WriteLine("Description: {0}", item.Name);
Console.WriteLine("Item Size: {0}", item.Size);
Console.WriteLine("Unit Price: {0:C}", item.UnitPrice);
}
}
}
|
- Execute the application and test it. Here is an example:
/-/Arrington Department Store/-/
Enter the following pieces of
information about the sale item
Item #: 624008
Store Items Categories
1. Women
2. Men
3. Girls
4. Boys
5. Babies
Enter the Category: 3
Item Name: Scotta Miniskirt
Item Size (Enter 0 if unknown): 11
Unit Price: 35.95
================================
/-/Arrington Department Store/-/
--------------------------------
Item #: 624008
Category: Girls
Description: Scotta Miniskirt
Item Size: 11
Unit Price: $35.95
Press any key to continue . . .
|
|
- Close the DOS window
Remember that, after creating a class, it becomes a data
type in its own right. We have seen that you could declare a variable from it,
you could pass it as argument, and you could return it from a method. As a
normal data type, a class can be validate. This means that its value can be
evaluated, rejected, or retrieved. Based on these characteristics of a class,
you can create a property from it.
To create a property that is based on a class, primarily
follow the same formulas we have applied to the other properties. The most
important aspect to remember is that the class is composite. That is, it is
(likely) made of fields of various types.
Practical
Learning: Creating a Property of a Class Type |
|
- Access the ShoppingItem.cs file and change it as follows:
using System;
namespace DepartmentStore2
{
public enum ItemCategory
{
Unspecified,
Women,
Men,
Girls,
Boys,
Babies
}
class ShoppingItem
{
private long itemNo;
private ItemCategory cat;
private string nm;
private string sz;
private decimal price;
// A property for the stock number of an item
public long ItemNumber
{
get
{
return itemNo;
}
set
{
itemNo = value;
}
}
// A property for the category of item
public ItemCategory Category
{
get
{
return cat;
}
set
{
cat = value;
}
}
// A property for the name of an item
public string Name
{
get
{
return nm;
}
set
{
if (nm == "")
nm = "Item no Description";
else
nm = value;
}
}
// A property for size of a merchandise
public string Size
{
get
{
if (sz == "0")
return "Unknown Size or Fits All";
else
return sz;
}
set
{
sz = value;
}
}
// A property for the marked price of an item
public decimal UnitPrice
{
get
{
return price;
}
set
{
if (price < 0)
price = 0.00M;
else
price = value;
}
}
}
}
|
- To create a new class, in the Solution Explorer, right-click the name of
the project, position the mouse on Add and click Class...
- Set the Name to DepartmentStore and click Add
- Change the file as follows:
using System;
namespace DepartmentStore2
{
class DepartmentStore
{
private int qty;
private ShoppingItem itm;
public int Quantity
{
get { return qty; }
set
{
if (qty <= 0)
qty = 0;
else
qty = value;
}
}
public ShoppingItem SaleItem
{
get { return itm; }
set
{
if (itm == null)
{
itm.ItemNumber = 0;
itm.Category = ItemCategory.Unspecified;
itm.Name = "Unknown";
itm.Size = "0";
itm.UnitPrice = 0.00M;
}
else
itm = value;
}
}
public DepartmentStore()
{
itm = new ShoppingItem();
}
public void ProcessOrder()
{
int category;
Console.WriteLine("/-/Arrington Department Store/-/");
Console.WriteLine("Enter the following pieces of");
Console.WriteLine("information about the sale item");
Console.Write("Item #: ");
itm.ItemNumber = long.Parse(Console.ReadLine());
Console.WriteLine("Store Items Categories");
Console.WriteLine("\t1. Women");
Console.WriteLine("\t2. Men");
Console.WriteLine("\t3. Girls");
Console.WriteLine("\t4. Boys");
Console.WriteLine("\t5. Babies");
Console.Write("Enter the Category: ");
category = int.Parse(Console.ReadLine());
if (category == 1)
itm.Category = ItemCategory.Women;
else if (category == 2)
itm.Category = ItemCategory.Men;
else if (category == 3)
itm.Category = ItemCategory.Girls;
else if (category == 4)
itm.Category = ItemCategory.Boys;
else if (category == 5)
itm.Category = ItemCategory.Babies;
else
itm.Category = ItemCategory.Unspecified;
Console.Write("Item Name: ");
itm.Name = Console.ReadLine();
Console.Write("Item Size (Enter 0 if unknown): ");
itm.Size = Console.ReadLine();
Console.Write("Unit Price: ");
itm.UnitPrice = decimal.Parse(Console.ReadLine());
Console.Write("How many samples of ");
Console.Write(itm.Name);
Console.Write(": ");
qty = int.Parse(Console.ReadLine());
}
public void DisplayReceipt()
{
decimal totalPrice = itm.UnitPrice * Quantity;
Console.WriteLine("\n================================");
Console.WriteLine("/-/Arrington Department Store/-/");
Console.WriteLine("--------------------------------");
Console.WriteLine("Item #: {0}", itm.ItemNumber);
Console.WriteLine("Category: {0}", itm.Category);
Console.WriteLine("Description: {0}", itm.Name);
Console.WriteLine("Item Size: {0}", itm.Size);
Console.WriteLine("Unit Price: {0:C}", itm.UnitPrice);
Console.WriteLine("Quantity: {0}", Quantity);
Console.WriteLine("Total Price: {0:C}\n", totalPrice);
Console.WriteLine("\n================================");
}
}
}
|
- Access the Program.cs file and change it as follows:
using System;
namespace DepartmentStore2
{
class Program
{
static void Main()
{
DepartmentStore store = new DepartmentStore();
store.ProcessOrder();
store.DisplayReceipt();
}
}
}
|
- Execute the application and test it. Here is an example:
/-/Arrington Department Store/-/
Enter the following pieces of
information about the sale item
Item #: 444412
Store Items Categories
1. Women
2. Men
3. Girls
4. Boys
5. Babies
Enter the Category: 1
Item Name: Stretch Cotton Shirt
Item Size (Enter 0 if unknown): 14
Unit Price: 55.95
How many samples of Stretch Cotton Shirt: 2
================================
/-/Arrington Department Store/-/
--------------------------------
Item #: 444412
Category: Women
Description: Stretch Cotton Shirt
Item Size: 14
Unit Price: $55.95
Quantity: 2
Total Price: $111.90
================================
Press any key to continue . . .
|
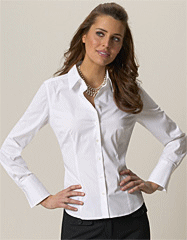 |
- Close the DOS window
|
|