 |
Built-In Collection Classes: The ArrayList Class |
|
Overview of .NET Collections |
|
The ICollection Interface |
|
|
To provide a common abstract techniques of creating
and using lists, the .NET Framework provides the ICollection
interface. This interface is equipped with a property to keep track of
the number of items in a list: the Count property. It is also
equipped with a method to copy the items from a list to an array.
|
Introduction to the ArrayList Class
|
|
To support the creation of any kind of list, the Microsoft
.NET Framework provides the ArrayList class. The ArrayList class
implements the ICollection interface. This class can be used to add,
locate, or remove an item from a list. The class provides many other valuable
operations routinely done on a list.
Because of its flexibility, ArrayList
is the most used class to create lists of items of any kind in a .NET
application. Besides the ability to handle any type of list, ArrayList is
a good class to study the theory of a collection. The routine operations found
in the ArrayList class are the same found in many other collection-based
classes you would use when creating commercial or graphical applications.
|
|
Besides the ability to create a collection, the ArrayList
class has the built-in mechanism for serialization.
The ArrayList class is defined in the
System.Collections namespace. Therefore, in order to use the ArrayList
class in your application, you can first include the System.Collections
namespace in the file that would perform ArrayList operations.
Application: Introducing the ArrayList Class
|
|
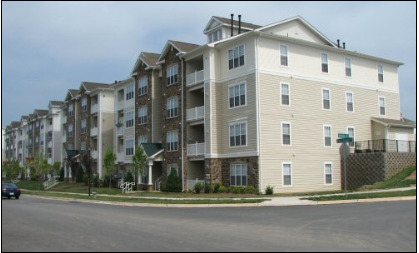
- Start Microsoft Visual Studio and create a new Console Application named
RealEstate5
- To save the project, on the Standard toolbar, click the Save All button

- Accept the suggestions and click Save
- To create a new class, in the Solution Explorer, right-click RealEstate5
-> Add -> Class...
- Set the Name to Property and click Add
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
public enum PropertyCondition
{
Unknown,
Excellent,
Good,
NeedsRepair,
BadShape
}
[Serializable]
public class Property
{
private string propNbr;
private PropertyCondition cond;
private short beds;
private float baths;
private int yr;
private decimal val;
public string PropertyNumber
{
get { return propNbr; }
set
{
if (propNbr == "")
propNbr = "N/A";
else
propNbr = value;
}
}
public string PropertyType;
public PropertyCondition Condition
{
get { return cond; }
set { cond = value; }
}
public short Bedrooms
{
get
{
if (beds <= 1)
return 1;
else
return beds;
}
set { beds = value; }
}
public float Bathrooms
{
get { return (baths <= 0) ? 0.00f : baths; }
set { baths = value; }
}
public int YearBuilt
{
get { return yr; }
set { yr = value; }
}
public decimal Value
{
get { return (val <= 0) ? 0.00M : val; }
set { val = value; }
}
}
}
- To create a new class, in the Class View, right-click RealEstate5 -> Add
-> Class...
- Set the Name to Condominium and press Enter
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
[Serializable]
public class Condominium : Property
{
private bool handicap;
public Condominium()
{
this.PropertyType = "Condominium";
}
public bool HandicapAccessible
{
get { return handicap; }
set { handicap = value; }
}
}
}
- To create a new class, on the main menu, click Project -> Add Class...
- Set the Name to HouseType and click Add
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
[Serializable]
public class HouseType : Property
{
private short nbrOfStories;
private bool basement;
private bool garage;
public short Stories
{
get { return nbrOfStories; }
set { nbrOfStories = value; }
}
public bool FinishedBasement
{
get { return basement; }
set { basement = value; }
}
public bool IndoorGarage
{
get { return garage; }
set { garage = value; }
}
}
}
- To create a new class, in the Solution Explorer, right-click RealEstate5
-> Add -> Class...
- Set the Name to Townhouse and press Enter
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
[Serializable]
public class Townhouse : HouseType
{
private bool comm;
public Townhouse()
{
this.PropertyType = "Townhouse";
}
public bool IsCommunityManaged
{
get { return this.comm; }
set { this.comm = value; }
}
}
}
- To create a new class, in the Class View, right-click RealEstate5 -> Add
-> Class...
- Set the Name to SingleFamily and click Add
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
[Serializable]
public class SingleFamily : HouseType
{
public SingleFamily()
{
this.PropertyType = "Single Family";
}
}
}
- Save all
After declaring an ArrayList variable, it is empty.
As objects are added to it, the list grows. The list can grow tremendously as
you wish. The number of items of the list is managed through the memory it
occupies and this memory grows as needed. The number of items that the memory
allocated is currently using is represented by the ArrayList.Capacity
property. This will usually be the least of your concerns.
If for some reason, you want to intervene and control the
number of items that your ArrayList list can contain, you can manipulate
the Capacity property. For example, you can assign it a constant to set
the maximum value that the list can contain. Once again, you will hardly have
any reason to use the Capacity property: the compiler knows what to do
with it.
If you set a fixed size on an ArrayList list, you may
not be able to add a new item beyond the limit. In fact, if you attempt to do
this, you may receive an error. A safe way is to check whether the list is fixed
before performing a related operation. To find out whether a list is fixed, you
can check the ArrayList variable's IsFixedSize property.
One of the reason for creating a list is to be able to add
items to it, edit its items, retrieve an items, or delete items from it. These
are the default operations. You can still limit these operations as you judge
them unnecessary. For example, you may create a list and then initialize it with
the items that you want the list to only have. If you don't intend to have the
user adding items to it, you can create the list as read-only. To do this, you
can call the ArrayList.ReadOnly() method. It is overloaded with two
versions as follows:
public static ArrayList ReadOnly(ArrayList);
public static IList ReadOnly(IList);
This method is static. This means that you don't need to
declare an instance of ArrayList to call them. Instead, to make the list
read-only, call the ArrayList.ReadOnly() method and pass your
ArrayList variable to it.
As we will see in the next sections, some operations cannot
be performed on a read-only list. To perform such operations, you can first find
out whether an ArrayList list is read-only. This is done by checking its
IsReadOnly property.
The primary operation performed on a list is to create one.
One of the biggest advantages of using a linked list is that you don't have to
specify in advance the number of items of the list as done for an array. You can
just start adding items. The ArrayList class makes this possible with the
Add() method. Its syntax is:
public virtual int Add(object value);
The argument of this method is the value to add to the list.
If the method succeeds with the addition, it returns the position where the
value was added in the list. This is usually the last position in the list. If
the method fails, the compiler would throw an error. One of the errors that
could result from failure of this operation would be based on the fact that
either a new item cannot be added to the list because the list is read-only, or
the list was already full prior to adding the new item. Normally, a list can be
full only if you had specified the maximum number of items it can contain using
the ArrayList.Capacity property. As mentioned above, the list can be made
read-only by passing its variable to the ArrayList.ReadOnly() method
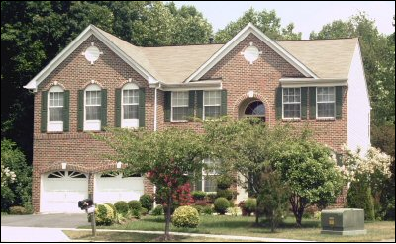
Application: Adding Items to an ArrayList List
|
|
- To create an inventory, on the main menu, click Project -> Add Class...
- Set the Name to PropertyManagement and press Enter
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
using System.Collections;
using System.Runtime.Serialization.Formatters.Binary;
namespace RealEstate5
{
public class PropertyManagement
{
private Condominium Condo;
private Townhouse TownHome;
private SingleFamily House;
private ArrayList Condominiums;
private ArrayList Townhouses;
private ArrayList SingleFamilies;
private string strpropertyNumber;
private string strPropertiesDirectory;
public PropertyManagement()
{
Condo = new Condominium();
TownHome = new Townhouse();
House = new SingleFamily();
Condominiums = new ArrayList();
Townhouses = new ArrayList();
SingleFamilies = new ArrayList();
strpropertyNumber = "000000";
strPropertiesDirectory = @"C:\Altair Realtors\Properties";
try
{
// If a directory for the properties has not yet
// been created, then create them
Directory.CreateDirectory(strPropertiesDirectory);
}
catch (DirectoryNotFoundException)
{
Console.WriteLine("The directory could " +
"not be created");
}
}
public PropertyCondition GetPropertyCondition()
{
short condition = 0;
try
{
Console.WriteLine("\nProperties Conditions");
Console.WriteLine("1. Excellent");
Console.WriteLine("2. Good (may need minor repair)");
Console.WriteLine("3. Needs Repair");
Console.Write("4. In Bad Shape (property needs ");
Console.WriteLine("major repair or rebuild)");
Console.Write("Enter Property Condition: ");
condition = short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the condition of the property is not valid");
}
if (condition == 1)
return PropertyCondition.Excellent;
else if (condition == 2)
return PropertyCondition.Good;
else if (condition == 3)
return PropertyCondition.NeedsRepair;
else if (condition == 4)
return PropertyCondition.BadShape;
else
return PropertyCondition.Unknown;
}
public void CreateProperty()
{
char propType = '0';
Console.WriteLine("\n=======================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("-=- Property Creation -=-");
Console.WriteLine("------------------------");
// We will make sure that no two
// properties have the same number
Console.Write("\nEnter Property #: ");
this.strpropertyNumber = Console.ReadLine();
try
{
Console.WriteLine("\nTypes of Properties");
Console.WriteLine("1. Condominium");
Console.WriteLine("2. Townhouse");
Console.WriteLine("3. Single Family");
Console.Write("Enter Type of Property: ");
propType = char.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the type of property is invalid");
}
switch (propType)
{
case '1':
CreateCondominium();
break;
case '2':
CreateTownhouse();
break;
case '3':
CreateSingleFamily();
break;
default:
Console.WriteLine("Invalid Choice!!!");
break;
}
}
public void CreateCondominium()
{
char answer = 'n';
Condo.propertyNumber = strpropertyNumber;
Condo.Condition = GetPropertyCondition();
try
{
Console.Write("\nHow many bedrooms? ");
Condo.Bedrooms = short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the number of bedrooms is not good");
}
try
{
Console.Write("How many bathrooms? ");
Condo.Bathrooms =
float.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid value");
}
try
{
Console.Write("Year built: ");
Condo.YearBuilt =
int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The house cannot have " +
"built in that year");
}
Console.Write("\nIs the building " +
"accessible to handicapped (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
Condo.HandicapAccessible = true;
else
Condo.HandicapAccessible = false;
try
{
Console.Write("Condominium Value: ");
Condo.Value = decimal.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Property Value");
}
Condominiums.Add(Condo);
ShowTitle();
ShowCondominium();
SaveCondominium();
}
public void CreateTownhouse()
{
char answer = 'n';
this.TownHome.propertyNumber = strpropertyNumber;
this.TownHome.Condition = GetPropertyCondition();
try
{
Console.Write("\nHow many stories (levels)? ");
this.TownHome.Stories =
short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The number of stories " +
"you entered is not valid");
}
try
{
Console.Write("How many bedrooms? ");
this.TownHome.Bedrooms =
short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the number of bedrooms is not good");
}
try
{
Console.Write("How many bathrooms? ");
this.TownHome.Bathrooms =
float.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid bathroom number.");
}
Console.Write("Does it have an indoor " +
"car garage (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
this.TownHome.IndoorGarage = true;
else
this.TownHome.IndoorGarage = false;
Console.Write("Is the basement finished(y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
this.TownHome.FinishedBasement = true;
else
this.TownHome.FinishedBasement = false;
try
{
Console.Write("Year built: ");
this.TownHome.YearBuilt =
int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The house cannot have " +
"built in that year");
}
try
{
Console.Write("Is it community managed (y/n)? ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
this.TownHome.IsCommunityManaged = true;
else
this.TownHome.IsCommunityManaged = false;
}
catch (FormatException)
{
Console.WriteLine("Invalid Answer");
}
try
{
Console.Write("Property Value: ");
this.TownHome.Value = decimal.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Property Value");
}
Townhouses.Add(this.TownHome);
ShowTitle();
ShowTownhouse();
SaveTownhouse();
}
public void CreateSingleFamily()
{
char answer = 'n';
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("-=- Property Creation -=-");
this.House.propertyNumber = strpropertyNumber;
House.Condition = GetPropertyCondition();
try
{
Console.Write("\nHow many stories (levels)? ");
House.Stories = short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The number of stories you " +
"entered is not allowed");
}
try
{
Console.Write("How many bedrooms? ");
House.Bedrooms =
short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the number of bedrooms is not good");
}
try
{
Console.Write("How many bathrooms? ");
House.Bathrooms =
float.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid number of bathrooms");
}
try
{
Console.Write("Does it have an indoor " +
"car garage (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.IndoorGarage = true;
else
House.IndoorGarage = false;
}
catch (FormatException)
{
Console.WriteLine("Invalid Indoor Car Garage Answer");
}
try
{
Console.Write("Is the basement finished(y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.FinishedBasement = true;
else
House.FinishedBasement = false;
}
catch (FormatException)
{
Console.WriteLine("Invalid Basement Answer");
}
try
{
Console.Write("Year built: ");
House.YearBuilt =
int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The house cannot have " +
"built in that year");
}
try
{
Console.Write("House Value: ");
House.Value = decimal.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Property Value");
}
SingleFamilies.Add(House);
ShowTitle();
ShowSingleFamily();
SaveSingleFamily();
}
public void ShowTitle()
{
Console.WriteLine("==================================");
Console.WriteLine(" =//=//= Altair Realtors =//=//=");
Console.WriteLine("-=-=-=- Properties Listing -=-=-=-");
}
public void ShowCondominium()
{
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
Condo.propertyNumber);
Console.WriteLine("Property Type: {0}",
Condo.PropertyType);
Console.WriteLine("Condition: {0}",
Condo.Condition);
Console.WriteLine("Bedrooms: {0}",
Condo.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
Condo.Bathrooms);
Console.WriteLine("Year Built: {0}",
Condo.YearBuilt);
Console.WriteLine("Handicapped Accessible Building: {0}",
Condo.HandicapAccessible);
Console.WriteLine("Market Value: {0:C}",
Condo.Value);
Console.WriteLine("----------------------------------");
}
public void ShowTownhouse()
{
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
TownHome.propertyNumber);
Console.WriteLine("Property Type: {0}",
TownHome.PropertyType);
Console.WriteLine("Stories: {0}",
TownHome.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
TownHome.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
TownHome.FinishedBasement);
Console.WriteLine("Condition: {0}",
TownHome.Condition);
Console.WriteLine("Bedrooms: {0}",
TownHome.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
TownHome.Bathrooms);
Console.WriteLine("Year Built: {0}",
TownHome.YearBuilt);
Console.WriteLine("Community Managed? {0}",
TownHome.IsCommunityManaged);
Console.WriteLine("Market Value: {0:C}",
TownHome.Value);
Console.WriteLine("----------------------------------");
}
public void ShowSingleFamily()
{
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
House.propertyNumber);
Console.WriteLine("Property Type: {0}",
House.PropertyType);
Console.WriteLine("Stories: {0}",
House.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
House.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
House.FinishedBasement);
Console.WriteLine("Condition: {0}",
House.Condition);
Console.WriteLine("Bedrooms: {0}",
House.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
House.Bathrooms);
Console.WriteLine("Year Built: {0}",
House.YearBuilt);
Console.WriteLine("Market Value: {0:C}",
House.Value);
Console.WriteLine("----------------------------------");
}
public void SaveCondominium()
{
FileStream fsCondo = null;
BinaryFormatter bfCondo = new BinaryFormatter();
string strFilename = strPropertiesDirectory +
@"\Condominiums.alr";
try
{
fsCondo = new FileStream(strFilename,
FileMode.Create,
FileAccess.Write);
bfCondo.Serialize(fsCondo, Condominiums);
}
finally
{
fsCondo.Close();
}
}
public void SaveTownhouse()
{
FileStream fsTown = null;
BinaryFormatter bfTown =
new BinaryFormatter();
string strFilename = strPropertiesDirectory +
@"\Townhouses.alr";
try
{
fsTown = new FileStream(strFilename,
FileMode.Create,
FileAccess.Write);
bfTown.Serialize(fsTown, Townhouses);
}
finally
{
fsTown.Close();
}
}
public void SaveSingleFamily()
{
FileStream fsHouse = null;
BinaryFormatter bfHouse = new BinaryFormatter();
string strFilename = strPropertiesDirectory +
@"\SingleFamilies.alr";
try
{
fsHouse = new FileStream(strFilename,
FileMode.Create,
FileAccess.Write);
bfHouse.Serialize(fsHouse,
SingleFamilies);
}
finally
{
fsHouse.Close();
}
}
}
}
- Access the Program.cs file and change it as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
public class Program
{
static void Main(string[] args)
{
var answer = 'q';
var listing = new PropertyManagement();
// Display the title
Console.WriteLine("=================================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("----------------------------------");
do
{
// Ask the user to select an option
try
{
Console.WriteLine("What do you want to do?");
Console.WriteLine("1. Create a property");
Console.WriteLine("0. Quit");
Console.Write("Your Choice? ");
answer = char.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Choice!!!");
}
switch (answer)
{
case '1':
listing.CreateProperty();
break;
default:
break;
}
} while(answer == '1');
Console.WriteLine();
}
}
}
- Execute the application and continually create the following properties:
=================================
=//= Altair Realtors =//=
----------------------------------
What do you want to do?
1. Create a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 000001
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 1
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 1
How many bedrooms? 1
How many bathrooms? 1
Year built: 1960
Is the building accessible to handicapped (y/n): n
Condominium Value: 10000
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 000001
Property Type: Condominium
Condition: Excellent
Bedrooms: 1
Bathrooms: 1.00
Year Built: 1960
Handicapped Accessible Building: False
Market Value: $10,000.00
----------------------------------
What do you want to do?
1. Create a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 000002
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 2
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 1
How many stories (levels)? 1
How many bedrooms? 1
How many bathrooms? 1
Does it have an indoor car garage (y/n): n
Is the basement finished(y/n): n
Year built: 1960
Is it community managed (y/n)? n
Property Value: 20000
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 000002
Property Type: Townhouse
Stories: 1
Has Indoor Car Garage: False
Finished Basement: False
Condition: Excellent
Bedrooms: 1
Bathrooms: 1.00
Year Built: 1960
Community Managed? False
Market Value: $20,000.00
----------------------------------
What do you want to do?
1. Create a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 000003
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 3
=//= Altair Realtors =//=
-=- Property Creation -=-
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 1
How many stories (levels)? 1
How many bedrooms? 1
How many bathrooms? 1
Does it have an indoor car garage (y/n): n
Is the basement finished(y/n): n
Year built: 1960
House Value: 30000
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 000003
Property Type: Single Family
Stories: 1
Has Indoor Car Garage: False
Finished Basement: False
Condition: Excellent
Bedrooms: 1
Bathrooms: 1.00
Year Built: 1960
Market Value: $30,000.00
----------------------------------
What do you want to do?
1. Create a property
0. Quit
Your Choice? 0
Press any key to continue . . .
- Close the DOS window
The Number of Items in the List
|
|
When using a list, at any time, you should be able to know
the number of items that the list contains:
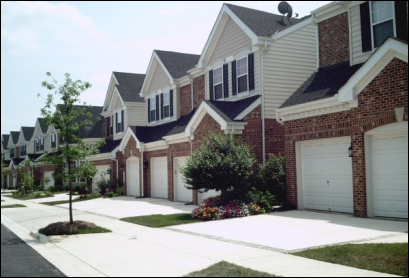
This information is provided by the
ArrayList.Count property. The Capacity and the Count have this in common:
the value of each increases as the list grows and the same value decreases if
the list shrinks. It is important to know that, although they look alike, there
are various differences between the capacity of a list and the number of items
it contains. Capacity is a read/write property. This means that, as we
saw above, you can assign a value to the capacity to fix the number of items
that the list can contain. You can also retrieve the value of the Capacity. The
Count is read-only because it is used by the compiler to count the
current number of items of the items and this counting is performed without your
intervention.
Once a list is ready, you can perform different types of
operations on it. Besides adding items, one of the most regular operations
performed on a list consists of locating and retrieving its items. You have
various options. To retrieve a single item based on its position, you can apply
the square brackets of arrays to the variable. Like a normal array, an
ArrayList list is zero-based. Another issue to keep in mind is that the
ArrayList[] returns an Object value. Therefore, you may have to cast this
value to your type of value to get it right.
Besides using the index to access an item from the list,
ArrayList class implements the IEnumerable.GetEnumerator() method.
For this reason, you can use the foreach loop to access each member of
the collection.
Application: Retrieving Items From an ArrayList List
|
|
- Change the PropertyManagement.cs file as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
using System.Collections;
using System.Runtime.Serialization.Formatters.Binary;
namespace RealEstate5
{
public class PropertyManagement
{
private Condominium Condo;
private Townhouse TownHome;
private SingleFamily House;
ArrayList Condominiums;
ArrayList Townhouses;
ArrayList SingleFamilies;
string strpropertyNumber;
string strPropertiesDirectory;
public PropertyManagement()
{
Condo = new Condominium();
TownHome = new Townhouse();
House = new SingleFamily();
Condominiums = new ArrayList();
Townhouses = new ArrayList();
SingleFamilies = new ArrayList();
strpropertyNumber = "000000";
strPropertiesDirectory = @"C:\Altair Realtors\Properties";
try
{
// If a directory for the properties has not yet
// been created, then create them
Directory.CreateDirectory(strPropertiesDirectory);
}
catch (DirectoryNotFoundException)
{
Console.WriteLine("The directory could not be created");
}
}
public PropertyCondition GetPropertyCondition()
{
short condition = 0;
try
{
Console.WriteLine("\nProperties Conditions");
Console.WriteLine("1. Excellent");
Console.WriteLine("2. Good (may need minor repair)");
Console.WriteLine("3. Needs Repair");
Console.Write("4. In Bad Shape (property needs ");
Console.WriteLine("major repair or rebuild)");
Console.Write("Enter Property Condition: ");
condition = short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the condition of the property is not valid");
}
if (condition == 1)
return PropertyCondition.Excellent;
else if (condition == 2)
return PropertyCondition.Good;
else if (condition == 3)
return PropertyCondition.NeedsRepair;
else if (condition == 4)
return PropertyCondition.BadShape;
else
return PropertyCondition.Unknown;
}
public void CreateProperty()
{
char propType = '0';
bool found = false;
FileStream fsProperties = null;
BinaryFormatter bfProperties = new BinaryFormatter();
Console.WriteLine("\n=======================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("-=- Property Creation -=-");
Console.WriteLine("------------------------");
// We will make sure that no two
// properties have the same number
Console.Write("\nEnter Property #: ");
this.strpropertyNumber = Console.ReadLine();
try
{
fsProperties = new FileStream(strPropertiesDirectory +
@"\Condominiums.alr",
FileMode.Open,
FileAccess.Read);
Condominiums =
(ArrayList)bfProperties.Deserialize(fsProperties);
foreach (Condominium c in Condominiums)
{
if (c.propertyNumber == this.strpropertyNumber)
{
found = true;
}
}
}
catch (FileNotFoundException)
{
Console.WriteLine("The Condominiums list was not found");
}
finally
{
fsProperties.Close();
}
try
{
fsProperties = new FileStream(strPropertiesDirectory +
@"\Townhouses.alr",
FileMode.Open,
FileAccess.Read);
Townhouses =
(ArrayList)bfProperties.Deserialize(fsProperties);
foreach (Townhouse t in Townhouses)
{
if (t.propertyNumber == this.strpropertyNumber)
{
found = true;
}
}
}
finally
{
fsProperties.Close();
}
try
{
fsProperties = new FileStream(strPropertiesDirectory +
@"\SingleFamilies.alr",
FileMode.Open,
FileAccess.Read);
SingleFamilies =
(ArrayList)bfProperties.Deserialize(fsProperties);
foreach (SingleFamily s in SingleFamilies)
{
if (s.propertyNumber == this.strpropertyNumber)
{
found = true;
}
}
}
finally
{
fsProperties.Close();
}
if (found == true)
{
Console.WriteLine("A property with that " +
"number exists already");
return;
}
try
{
Console.WriteLine("\nTypes of Properties");
Console.WriteLine("1. Condominium");
Console.WriteLine("2. Townhouse");
Console.WriteLine("3. Single Family");
Console.Write("Enter Type of Property: ");
propType = char.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the type of property is invalid");
}
switch (propType)
{
case '1':
CreateCondominium();
break;
case '2':
CreateTownhouse();
break;
case '3':
CreateSingleFamily();
break;
default:
Console.WriteLine("Invalid Choice!!!");
break;
}
}
public void CreateCondominium()
{
char answer = 'n';
Condo.propertyNumber = strpropertyNumber;
Condo.Condition = GetPropertyCondition();
try
{
Console.Write("\nHow many bedrooms? ");
Condo.Bedrooms = short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the number of bedrooms is not good");
}
try
{
Console.Write("How many bathrooms? ");
Condo.Bathrooms =
float.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid value");
}
try
{
Console.Write("Year built: ");
Condo.YearBuilt =
int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The house cannot have " +
"built in that year");
}
Console.Write("\nIs the building " +
"accessible to handicapped (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
Condo.HandicapAccessible = true;
else
Condo.HandicapAccessible = false;
try
{
Console.Write("Condominium Value: ");
Condo.Value = decimal.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Property Value");
}
Condominiums.Add(Condo);
ShowTitle();
ShowCondominium();
SaveCondominium();
}
public void CreateTownhouse()
{
char answer = 'n';
this.TownHome.propertyNumber = strpropertyNumber;
this.TownHome.Condition = GetPropertyCondition();
try
{
Console.Write("\nHow many stories (levels)? ");
this.TownHome.Stories =
short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The number of stories " +
"you entered is not valid");
}
try
{
Console.Write("How many bedrooms? ");
this.TownHome.Bedrooms =
short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the number of bedrooms is not good");
}
try
{
Console.Write("How many bathrooms? ");
this.TownHome.Bathrooms =
float.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid bathroom number.");
}
Console.Write("Does it have an indoor " +
"car garage (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
this.TownHome.IndoorGarage = true;
else
this.TownHome.IndoorGarage = false;
Console.Write("Is the basement finished(y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
this.TownHome.FinishedBasement = true;
else
this.TownHome.FinishedBasement = false;
try
{
Console.Write("Year built: ");
this.TownHome.YearBuilt =
int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The house cannot have " +
"built in that year");
}
try
{
Console.Write("Is it community managed (y/n)? ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
this.TownHome.IsCommunityManaged = true;
else
this.TownHome.IsCommunityManaged = false;
}
catch (FormatException)
{
Console.WriteLine("Invalid Answer");
}
try
{
Console.Write("Property Value: ");
this.TownHome.Value =
decimal.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Property Value");
}
Townhouses.Add(this.TownHome);
ShowTitle();
ShowTownhouse();
SaveTownhouse();
}
public void CreateSingleFamily()
{
char answer = 'n';
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("-=- Property Creation -=-");
this.House.propertyNumber = strpropertyNumber;
House.Condition = GetPropertyCondition();
try
{
Console.Write("\nHow many stories (levels)? ");
House.Stories = short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The number of stories you " +
"entered is not allowed");
}
try
{
Console.Write("How many bedrooms? ");
House.Bedrooms =
short.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The value you entered for " +
"the number of bedrooms is not good");
}
try
{
Console.Write("How many bathrooms? ");
House.Bathrooms =
float.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid number of bathrooms");
}
try
{
Console.Write("Does it have an indoor " +
"car garage (y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.IndoorGarage = true;
else
House.IndoorGarage = false;
}
catch (FormatException)
{
Console.WriteLine("Invalid Indoor Car Garage Answer");
}
try
{
Console.Write("Is the basement finished(y/n): ");
answer = char.Parse(Console.ReadLine());
if ((answer == 'y') || (answer == 'Y'))
House.FinishedBasement = true;
else
House.FinishedBasement = false;
}
catch (FormatException)
{
Console.WriteLine("Invalid Basement Answer");
}
try
{
Console.Write("Year built: ");
House.YearBuilt =
int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("The house cannot have " +
"built in that year");
}
try
{
Console.Write("House Value: ");
House.Value = decimal.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Property Value");
}
SingleFamilies.Add(House);
ShowTitle();
ShowSingleFamily();
SaveSingleFamily();
}
public void ShowTitle()
{
Console.WriteLine("==================================");
Console.WriteLine(" =//=//= Altair Realtors =//=//=");
Console.WriteLine("-=-=-=- Properties Listing -=-=-=-");
}
public void ShowCondominium()
{
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
Condo.propertyNumber);
Console.WriteLine("Property Type: {0}",
Condo.PropertyType);
Console.WriteLine("Condition: {0}",
Condo.Condition);
Console.WriteLine("Bedrooms: {0}",
Condo.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
Condo.Bathrooms);
Console.WriteLine("Year Built: {0}",
Condo.YearBuilt);
Console.WriteLine("Handicapped Accessible Building: {0}",
Condo.HandicapAccessible);
Console.WriteLine("Market Value: {0:C}",
Condo.Value);
Console.WriteLine("----------------------------------");
}
public void ShowTownhouse()
{
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
TownHome.propertyNumber);
Console.WriteLine("Property Type: {0}",
TownHome.PropertyType);
Console.WriteLine("Stories: {0}",
TownHome.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
TownHome.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
TownHome.FinishedBasement);
Console.WriteLine("Condition: {0}",
TownHome.Condition);
Console.WriteLine("Bedrooms: {0}",
TownHome.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
TownHome.Bathrooms);
Console.WriteLine("Year Built: {0}",
TownHome.YearBuilt);
Console.WriteLine("Community Managed? {0}",
TownHome.IsCommunityManaged);
Console.WriteLine("Market Value: {0:C}",
TownHome.Value);
Console.WriteLine("----------------------------------");
}
public void ShowSingleFamily()
{
Console.WriteLine("----------------------------------");
Console.WriteLine("Property #: {0}",
House.propertyNumber);
Console.WriteLine("Property Type: {0}",
House.PropertyType);
Console.WriteLine("Stories: {0}",
House.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
House.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
House.FinishedBasement);
Console.WriteLine("Condition: {0}",
House.Condition);
Console.WriteLine("Bedrooms: {0}",
House.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
House.Bathrooms);
Console.WriteLine("Year Built: {0}",
House.YearBuilt);
Console.WriteLine("Market Value: {0:C}",
House.Value);
Console.WriteLine("----------------------------------");
}
public void SaveCondominium()
{
FileStream fsCondo = null;
BinaryFormatter bfCondo = new BinaryFormatter();
string strFilename = strPropertiesDirectory +
@"\Condominiums.alr";
try
{
fsCondo = new FileStream(strFilename,
FileMode.Create,
FileAccess.Write);
bfCondo.Serialize(fsCondo, Condominiums);
}
finally
{
fsCondo.Close();
}
}
public void SaveTownhouse()
{
FileStream fsTown = null;
BinaryFormatter bfTown = new BinaryFormatter();
string strFilename = strPropertiesDirectory +
@"\Townhouses.alr";
try
{
fsTown = new FileStream(strFilename,
FileMode.Create,
FileAccess.Write);
bfTown.Serialize(fsTown, Townhouses);
}
finally
{
fsTown.Close();
}
}
public void SaveSingleFamily()
{
FileStream fsHouse = null;
BinaryFormatter bfHouse =
new BinaryFormatter();
string strFilename = strPropertiesDirectory +
@"\SingleFamilies.alr";
try
{
fsHouse =
new FileStream(strFilename,
FileMode.Create,
FileAccess.Write);
bfHouse.Serialize(fsHouse, SingleFamilies);
}
finally
{
fsHouse.Close();
}
}
public void ShowProperties()
{
int choice = 0;
Console.WriteLine();
ShowTitle();
try
{
Console.WriteLine("What properties do you want to see?");
Console.WriteLine("1. One Particular Property");
Console.WriteLine("2. Condominiums Only");
Console.WriteLine("3. Townhouses Only");
Console.WriteLine("4. Single Families Only");
Console.WriteLine("5. All properties");
Console.WriteLine("6. None");
Console.Write("Your Choice? ");
choice = int.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Selection");
}
switch (choice)
{
case 1:
ShowParticularProperty();
break;
case 2:
ShowCondominiums();
break;
case 3:
ShowTownhouses();
break;
case 4:
ShowSingleFamilies();
break;
case 5:
ShowCondominiums();
ShowTownhouses();
ShowSingleFamilies();
break;
default:
break;
}
}
public void ShowParticularProperty()
{
bool found = false;
FileStream fsProperty = null;
BinaryFormatter bfProperty = new BinaryFormatter();
Console.WriteLine("\n=======================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("------------------------");
Console.Write("\nEnter Property #: ");
this.strpropertyNumber = Console.ReadLine();
try
{
fsProperty = new FileStream(strPropertiesDirectory +
@"\Condominiums.alr",
FileMode.Open,
FileAccess.Read);
Condominiums =
(ArrayList)bfProperty.Deserialize(fsProperty);
foreach (Condominium c in Condominiums)
{
if (c.propertyNumber == this.strpropertyNumber)
{
found = true;
Condo = c;
ShowCondominium();
return;
}
}
}
finally
{
fsProperty.Close();
}
try
{
fsProperty = new FileStream(strPropertiesDirectory +
@"\Townhouses.alr",
FileMode.Open,
FileAccess.Read);
Townhouses =
(ArrayList)bfProperty.Deserialize(fsProperty);
foreach (Townhouse t in Townhouses)
{
if (t.propertyNumber == this.strpropertyNumber)
{
found = true;
TownHome = t;
ShowTownhouse();
return;
}
}
}
finally
{
fsProperty.Close();
}
try
{
fsProperty = new FileStream(strPropertiesDirectory +
@"\SingleFamilies.alr",
FileMode.Open,
FileAccess.Read);
SingleFamilies =
(ArrayList)bfProperty.Deserialize(fsProperty);
foreach (SingleFamily s in SingleFamilies)
{
if (s.propertyNumber == this.strpropertyNumber)
{
found = true;
House = s;
ShowSingleFamily();
return;
}
}
}
finally
{
fsProperty.Close();
}
if (found == false)
{
Console.WriteLine("No property with that " +
"number was found in the database");
return;
}
}
public void ShowCondominiums()
{
FileStream fsProperty = null;
BinaryFormatter bfProperty = new BinaryFormatter();
try
{
fsProperty = new FileStream(strPropertiesDirectory +
@"\Condominiums.alr",
FileMode.Open,
FileAccess.Read);
Condominiums =
(ArrayList)bfProperty.Deserialize(fsProperty);
Console.WriteLine("\n=================================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("----------------------------------");
Console.WriteLine("Condominiums Listing");
Console.WriteLine("----------------------------------");
foreach (Condominium c in Condominiums)
{
Console.WriteLine("Property #: {0}",
c.propertyNumber);
Console.WriteLine("Condition: {0}",
c.Condition);
Console.WriteLine("Bedrooms: {0}",
c.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
c.Bathrooms);
Console.WriteLine("Year Built: {0}",
c.YearBuilt);
Console.WriteLine("Handicapped Accessible Building: {0}",
Condo.HandicapAccessible);
Console.WriteLine("Market Value: {0:C}",
c.Value);
Console.WriteLine("----------------------------------");
}
}
finally
{
fsProperty.Close();
}
}
public void ShowTownhouses()
{
FileStream fsProperty = null;
BinaryFormatter bfProperty = new BinaryFormatter();
try
{
fsProperty = new FileStream(strPropertiesDirectory +
@"\Townhouses.alr",
FileMode.Open,
FileAccess.Read);
Townhouses = (ArrayList)bfProperty.Deserialize(fsProperty);
Console.WriteLine("\n=================================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("----------------------------------");
Console.WriteLine("Townhouses Listing");
Console.WriteLine("----------------------------------");
foreach (Townhouse t in Townhouses)
{
Console.WriteLine("Property #: {0}",
t.propertyNumber);
Console.WriteLine("Stories: {0}",
t.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
t.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
t.FinishedBasement);
Console.WriteLine("Condition: {0}",
t.Condition);
Console.WriteLine("Bedrooms: {0}",
t.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
t.Bathrooms);
Console.WriteLine("Year Built: {0}",
t.YearBuilt);
Console.WriteLine("Community Managed? {0}",
t.IsCommunityManaged);
Console.WriteLine("Market Value: {0:C}",
t.Value);
Console.WriteLine("----------------------------------");
}
}
finally
{
fsProperty.Close();
}
}
public void ShowSingleFamilies()
{
FileStream fsProperty = null;
BinaryFormatter bfProperty = new BinaryFormatter();
try
{
fsProperty = new FileStream(strPropertiesDirectory +
@"\SingleFamilies.alr",
FileMode.Open,
FileAccess.Read);
SingleFamilies =
(ArrayList)bfProperty.Deserialize(fsProperty);
Console.WriteLine("\n================================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("----------------------------------");
Console.WriteLine("Single Families Listing");
Console.WriteLine("----------------------------------");
foreach (SingleFamily s in SingleFamilies)
{
Console.WriteLine("Property #: {0}",
s.propertyNumber);
Console.WriteLine("Stories: {0}",
s.Stories);
Console.WriteLine("Has Indoor Car Garage: {0}",
s.IndoorGarage);
Console.WriteLine("Finished Basement: {0}",
s.FinishedBasement);
Console.WriteLine("Condition: {0}",
s.Condition);
Console.WriteLine("Bedrooms: {0}",
s.Bedrooms);
Console.WriteLine("Bathrooms: {0:F}",
s.Bathrooms);
Console.WriteLine("Year Built: {0}",
s.YearBuilt);
Console.WriteLine("Market Value: {0:C}",
s.Value);
Console.WriteLine("----------------------------------");
}
}
finally
{
fsProperty.Close();
}
}
}
}
- Access the Program.cs file and change it as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RealEstate5
{
public class Program
{
static void Main(string[] args)
{
var answer = 'q';
var listing = new PropertyManagement();
// Display the title
Console.WriteLine("\n================================");
Console.WriteLine(" =//= Altair Realtors =//=");
Console.WriteLine("----------------------------------");
do
{
// Ask the user to select an option
try
{
Console.WriteLine("What do you want to do?");
Console.WriteLine("1. Create a property");
Console.WriteLine("2. Show the properties");
Console.WriteLine("3. Delete a property");
Console.WriteLine("0. Quit");
Console.Write("Your Choice? ");
answer = char.Parse(Console.ReadLine());
}
catch (FormatException)
{
Console.WriteLine("Invalid Choice!!!");
}
switch (answer)
{
case '1':
listing.CreateProperty();
Console.WriteLine("\n");
break;
case '2':
listing.ShowProperties();
break;
case '3':
break;
default:
break;
}
} while ((answer == '1') ||
(answer == '2') ||
(answer == '3'));
Console.WriteLine();
}
}
}
- Execute the application and test it. Here is an example:
================================
=//= Altair Realtors =//=
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 246185
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 1
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 2
How many bedrooms? 2
How many bathrooms? 2
Year built: 1996
Is the building accessible to handicapped (y/n): n
Condominium Value: 285660
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 246185
Property Type: Condominium
Condition: Good
Bedrooms: 2
Bathrooms: 2.00
Year Built: 1996
Handicapped Accessible Building: False
Market Value: $285,660.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 512664
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 2
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 1
How many stories (levels)? 3
How many bedrooms? 3
How many bathrooms? 2.5
Does it have an indoor car garage (y/n): n
Is the basement finished(y/n): y
Year built: 1992
Is it community managed (y/n)? y
Property Value: 435660
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 512664
Property Type: Townhouse
Stories: 3
Has Indoor Car Garage: False
Finished Basement: True
Condition: Excellent
Bedrooms: 3
Bathrooms: 2.50
Year Built: 1992
Community Managed? True
Market Value: $435,660.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 802664
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 3
=//= Altair Realtors =//=
-=- Property Creation -=-
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 1
How many stories (levels)? 3
How many bedrooms? 4
How many bathrooms? 3.5
Does it have an indoor car garage (y/n): y
Is the basement finished(y/n): y
Year built: 1995
House Value: 755820
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 802664
Property Type: Single Family
Stories: 3
Has Indoor Car Garage: True
Finished Basement: True
Condition: Excellent
Bedrooms: 4
Bathrooms: 3.50
Year Built: 1995
Market Value: $755,820.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 1
=======================
=//= Altair Realtors =//=
-=- Property Creation -=-
------------------------
Enter Property #: 693524
Types of Properties
1. Condominium
2. Townhouse
3. Single Family
Enter Type of Property: 3
=//= Altair Realtors =//=
-=- Property Creation -=-
Properties Conditions
1. Excellent
2. Good (may need minor repair)
3. Needs Repair
4. In Bad Shape (property needs major repair or rebuild)
Enter Property Condition: 3
How many stories (levels)? 2
How many bedrooms? 3
How many bathrooms? 2.5
Does it have an indoor car garage (y/n): n
Is the basement finished(y/n): n
Year built: 1964
House Value: 515665
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
----------------------------------
Property #: 693524
Property Type: Single Family
Stories: 2
Has Indoor Car Garage: False
Finished Basement: False
Condition: NeedsRepair
Bedrooms: 3
Bathrooms: 2.50
Year Built: 1964
Market Value: $515,665.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 0
Press any key to continue . . .
- Close the DOS window
- Execute the application again and test different options. Here are
examples:
================================
=//= Altair Realtors =//=
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 2
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
What properties do you want to see?
1. One Particular Property
2. Condominiums Only
3. Townhouses Only
4. Single Families Only
5. All properties
6. None
Your Choice? 1
=======================
=//= Altair Realtors =//=
------------------------
Enter Property #: 802664
----------------------------------
Property #: 802664
Property Type: Single Family
Stories: 3
Has Indoor Car Garage: True
Finished Basement: True
Condition: Excellent
Bedrooms: 4
Bathrooms: 3.50
Year Built: 1995
Market Value: $755,820.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 2
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
What properties do you want to see?
1. One Particular Property
2. Condominiums Only
3. Townhouses Only
4. Single Families Only
5. All properties
6. None
Your Choice? 3
=================================
=//= Altair Realtors =//=
----------------------------------
Townhouses Listing
----------------------------------
Property #: 000002
Stories: 1
Has Indoor Car Garage: False
Finished Basement: False
Condition: Excellent
Bedrooms: 1
Bathrooms: 1.00
Year Built: 1960
Community Managed? False
Market Value: $20,000.00
----------------------------------
Property #: 512664
Stories: 3
Has Indoor Car Garage: False
Finished Basement: True
Condition: Excellent
Bedrooms: 3
Bathrooms: 2.50
Year Built: 1992
Community Managed? True
Market Value: $435,660.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 2
==================================
=//=//= Altair Realtors =//=//=
-=-=-=- Properties Listing -=-=-=-
What properties do you want to see?
1. One Particular Property
2. Condominiums Only
3. Townhouses Only
4. Single Families Only
5. All properties
6. None
Your Choice? 4
================================
=//= Altair Realtors =//=
----------------------------------
Single Families Listing
----------------------------------
Property #: 000003
Stories: 1
Has Indoor Car Garage: False
Finished Basement: False
Condition: Excellent
Bedrooms: 1
Bathrooms: 1.00
Year Built: 1960
Market Value: $30,000.00
----------------------------------
Property #: 802664
Stories: 3
Has Indoor Car Garage: True
Finished Basement: True
Condition: Excellent
Bedrooms: 4
Bathrooms: 3.50
Year Built: 1995
Market Value: $755,820.00
----------------------------------
Property #: 693524
Stories: 2
Has Indoor Car Garage: False
Finished Basement: False
Condition: NeedsRepair
Bedrooms: 3
Bathrooms: 2.50
Year Built: 1964
Market Value: $515,665.00
----------------------------------
What do you want to do?
1. Create a property
2. Show the properties
3. Delete a property
0. Quit
Your Choice? 0
Press any key to continue . . .
- Close the DOS window
Instead of the square brackets that allow you to retrieve an
item based on its position, you can look for an item based on its complete
definition. You have various options. You can first "build" an item and ask the
compiler to check whether any item in the list matches your definition. To
perform this search, you can call the ArrayList.Contains() method. Its
syntax is:
public virtual bool Contains(object item);
The item to look for is passed as argument to the method.
The compiler would look for exactly the item, using its definition, in the list.
If any detail of the argument fails to match any item of the ArrayList
list, the method would return false. If all characteristics of the argument
correspond to an item of the list, the method returns true.
Another option to look for an item in a list consists of
calling the ArrayList.BinarySearch() method. It is overloaded in three
versions and one of them uses the following syntax:
public virtual int BinarySearch(object value);
The item to look for is passed argument to the method.
As opposed to adding an item to a list, you may want to
remove one. To perform this operation, you have various options. You can ask the
compiler to look for an item in the list and if, or once, the compile finds it,
it would delete the item. To perform this type of deletion, you can call the
ArrayList.Remove() method. Its syntax is:
public virtual void Remove(object obj);
This method accepts as argument the item that you want to
delete from the list. To perform this operation, the list must not be read-only.
The Remove() method allows you to specify the exact
item you want to delete from a list. Another option you have consists of
deleting an item based on its position. This is done using the RemoveAt()
method whose syntax is:
public virtual void RemoveAt(int index);
With this method, the position of the item is passed as
argument. If the position is not valid because either it is lower or higher than
the current Count, the compiler would throw an
ArgumentOutOfRangeException exception.
To remove all items from a list at once, you can call the
ArrayList.Clear() method. Its syntax is:
public virtual void Clear();
|
|