 |
Introduction to GUI Applications |
|
|
Graphical user interfaces are quite easy to create in Java,
using Swing. We will review the steps to create a GUI application using NetBeans.
|
Practical
Learning: Starting the Application
|
|
- Start NetBeans
- To create a new application, on the main menu, click File -> New
Project...
- In the first page of the wizard, click Next
- In the second page of the wizard, change the Project Name to GUI101
- Click Finish
Probably the first thing you need on a graphical application
is called a form. In Swing, this is represented by a class named
JFrame.
To create a frame, you can derive a class from JFrame.
You can then instantiate the class when necessary. To display the frame to the
user, you can call the setVisible() method of the
JFrame class. You can use the other methods to manage the frame.
Practical
Learning: Creating a Frame
|
|
- To indicate that our main class is derived from JFrame, change the code
as follows:
package gui101;
import javax.swing.*;
public class Main extends JFrame {
public static void main(String[] args) {
Main GUI = new Main();
GUI.setVisible(true);
}
}
- Execute the application (you can press F6)
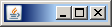
- Close the form and return to your programming environment
- To show a title and to specify the size of the frame, change the code as
follows:
package gui101;
import javax.swing.*;
public class Main extends JFrame {
public static void main(String[] args) {
Main GUI = new Main();
GUI.setTitle("Graphical User Interface");
GUI.setSize(300, 200);
GUI.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
GUI.setVisible(true);
}
}
- Execute the application (you can press F6)
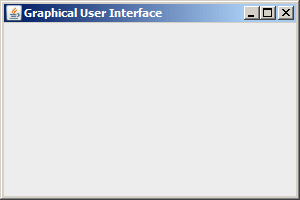
- Close the form and return to your programming environment
A graphical application is made useful by equipping it with
Windows controls, and there are many of them. You can create each control
individually. Once they are ready, you can add them to a panel (an object based
on the JPanel class). Once the panel is ready, add it to the frame variable.
Practical
Learning: Adding Controls to a Frame
|
|
- To add a button to the frame, change the code
as follows:
package gui101;
import javax.swing.*;
public class Main extends JFrame {
JButton btnOK;
JPanel pnlHolder;
public Main() {
pnlHolder = new JPanel();
btnOK = new JButton("OK");
pnlHolder.add(btnOK);
this.add(pnlHolder);
}
public static void main(String[] args) {
Main GUI = new Main();
GUI.setTitle("Graphical User Interface");
GUI.setSize(300, 200);
GUI.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
GUI.setVisible(true);
}
}
- Execute the application
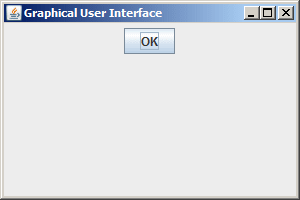
- Close the form and return to your programming environment
|
|