Practical
Learning: Introducing Dictionary Collections
|
|
- Start Microsoft Visual Basic and create a new Windows Forms Application named BethesdaCarRental1
- To add a new form to the project, in the Solution Explorer, right-click
BethesdaCarRental1 -> Add -> Windows Form...
- Set the Name to RentalRates and press Enter
- Add a ListView to the form and create its Columns as follows:
(Name) |
Text |
TextAlign |
Width |
colCategory |
Category |
|
90 |
colDaily |
Daily |
Right |
|
colWeekly |
Weekly |
Right |
|
colMonthly |
Monthly |
Right |
|
colWeekend |
Weekend |
Right |
|
- Create its Items as follows:
ListViewItem |
SubItems |
SubItems |
SubItems |
SubItems |
|
Text |
Text |
Text |
Text |
Economy |
35.95 |
32.75 |
28.95 |
24.95 |
Compact |
39.95 |
35.75 |
32.95 |
28.95 |
Standard |
45.95 |
39.75 |
35.95 |
32.95 |
Full Size |
49.95 |
42.75 |
38.95 |
35.95 |
Mini Van |
55.95 |
50.75 |
45.95 |
42.95 |
SUV |
55.95 |
50.75 |
45.95 |
42.95 |
Truck |
42.75 |
38.75 |
35.95 |
32.95 |
Van |
69.95 |
62.75 |
55.95 |
52.95 |
- Complete the design of the form as follows:

- To add a new form to the application, in the Solution Explorer, right-click
BethesdaCarRental1 -> Add -> Windows Form...
- Set the Name to Employees and click Add
- From the Toolbox, add a ListView to the form
- While the new list view is still selected, in the Properties window, click
the ellipsis button of the Columns field and create the columns as follows:
(Name) |
Text |
TextAlign |
Width |
colEmployeeNumber |
Empl # |
|
45 |
colFirstName |
First Name |
|
65 |
colLastName |
Last Name |
|
65 |
colFullName |
Full Name |
|
140 |
colTitle |
Title |
|
120 |
colHourlySalary |
Hourly Salary |
Right |
75 |
- Design the form as follows:
 |
Control |
Text |
Name |
Other Properties |
ListView |
|
lvwEmployees |
Anchor: Top, Bottom, Left, Right
FullRowSelect: True
GridLines: True
View: Details |
Button |
New Employee... |
btnNewEmployee |
|
Button |
Close |
btnClose |
|
|
- To add another form to the application, in the Solution Explorer, right- click
BethesdaCarRental1
-> Add ->
Windows Form...
- Set the Name to EmployeeEditor and click Add
- Design the form as follows:
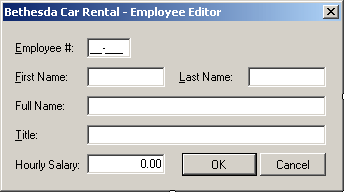 |
Control |
Text |
Name |
Properties |
Label |
&Employee #: |
|
|
MaskedTextBox |
|
txtEmployeeNumber |
Mask: 00-000
Modifiers: public |
Label |
First Name: |
|
|
TextBox |
|
txtFirstName |
Modifiers: public |
Label |
Last Name: |
|
|
TextBox |
|
txtLastName |
Modifiers: public |
Label |
Full Name: |
|
|
TextBox |
|
txtFullName |
Enabled: False
Modifiers: public |
Label |
Title: |
|
|
TextBox |
|
txtTitle |
Modifiers: public |
Label |
Hourly Salary: |
|
|
TextBox |
|
txtHourlySalary |
Modifiers: public
TextAlign: Right |
Button |
OK |
btnOK |
DialogResult: OK |
Button |
Cancel |
btnCancel |
DialogResult: Cancel |
Form |
|
|
AcceptButton: btnOK
CancelButton: btnCancel
FormBorderStyle: FixedDialog
MaximizeBox: False
MinimizeBox: False
ShowInTaskbar: False |
|
- Right-click the form and click View Code
- In the Class Name combo box, select txtLastName
- In the Method Name combo box, select Leave and implement the event as follows:
REM This code is used to create and display the customer's full name
Private Sub txtLastNameLeave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtLastName.Leave
Dim strFirstName As String = txtFirstName.Text
Dim strLastName As String = txtLastName.Text
Dim strFullName As String
If strFirstName.Length = 0 Then
strFullName = strLastName
Else
strFullName = strLastName & ", " & strFirstName
End If
txtFullName.Text = strFullName
End Sub
- To add a new class to the project, on the main menu, click Project
-> Add Class...
- Set the Class Name to Employee and click Add
- Change the Employee.vb file as follows:
<Serializable()> Public Class Employee
Public FirstName As String
Public LastName As String
Public Title As String
Public HourlySalary As Double
Public ReadOnly Property FullName()
Get
Return LastName & ", " & FirstName
End Get
End Property
REM This default constructor says that
REM the employee is not defined
Public Sub New()
FirstName = "Unknown"
LastName = "Unknown"
Title = "N/A"
HourlySalary = 0.0
End Sub
REM This constructor completely defines an employee
Public Sub New(ByVal fname As String, ByVal lname As String,
ByVal title As String, ByVal salary As Double)
FirstName = fname
LastName = lname
Title = Title
HourlySalary = salary
End Sub
End Class
- To add a new form to the application, on the main menu, click Project
-> Add Windows Forms
- Set the Name to Customers and press Enter
- From the Toolbox, add a ListView to the form
- While the new list view is still selected, in the Properties window, click
the ellipsis button of the Columns field and create the columns as follows:
(Name) |
Text |
Width |
colDrvLicNbr |
Driver's Lic. # |
100 |
colFullName |
Full Name |
100 |
colAddress |
Address |
160 |
colCity |
City |
100 |
colState |
State |
38 |
colZIPCode |
ZIP Code |
|
- Design the form as follows:
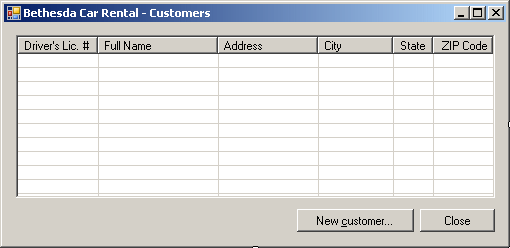 |
Control |
Text |
Name |
Other Properties |
ListView |
|
lvwCustomers |
View: Details
GridLines: True
FullRowSelect: True |
Button |
New Customer... |
btnNewCustomer |
|
Button |
Close |
btnClose |
|
|
- To add another form to the application, on the main menu, click Project
-> Add Windows Forms
- Set the Name to CustomerEditor and click Add
- Design the form as follows:
 |
Control |
Text |
Name |
Properties |
Label |
Driver's Lic. #: |
|
|
TextBox |
|
txtDrvLicNbr |
Modifiers: Public |
Label |
State: |
|
|
ComboBox |
|
cbxStates |
Modifiers: Public
Items: AL, AK, AZ, AR, CA, CO, CT, DE, DC, FL, GA, HI, ID, IL,
IN, IA, KS, KY, LA, ME, MD, MA, MI, MN, MS, MO, MT, NE, NV, NH,
NJ, NM, NY, NC, ND, OH, OK, OR, PA, RI, SC, SD, TN, TX, UT, VT,
VA, WA, WV, WI, WY |
Label |
Full Name: |
|
|
TextBox |
|
txtFullName |
Modifiers: Public |
Label |
Address: |
|
|
TextBox |
|
txtAddress |
Modifiers: Public |
Label |
City: |
|
|
TextBox |
|
txtCity |
Modifiers: Public |
Label |
ZIP Code: |
|
|
TextBox |
|
txtZIPCode |
Modifiers: Public |
Button |
OK |
btnOK |
DialogResult: OK |
Button |
Close |
btnCancel |
DialogResult: Cancel |
Form |
|
|
AcceptButton: btnOK
CancelButton: btnCancel
FormBorderStyle: FixedDialog
MaximizeBox: False
MinimizeBox: False
ShowInTaskbar: False |
|
- To add a new class to the project, on the main menu, click Project -> Add
Class...
- Set the Class Name to Customer and click Finish
- Change the Customer.vb file as follows:
<Serializable()> Public Class Customer
Public FullName As String
Public Address As String
Public City As String
Public State As String
Public ZIPCode As String
Public Sub New()
FullName = ""
Address = ""
City = ""
State = ""
ZIPCode = ""
End Sub
REM This constructor defines a customer
Public Sub New(ByVal fName As String,
ByVal adr As String, ByVal ct As String,
ByVal ste As String, ByVal zip As String)
FullName = fName
Address = adr
City = ct
State = ste
ZIPCode = zip
End Sub
End Class
- Save all
- Copy the following pictures to any folder somewhere on your computer (this
is simply an example of preparing the pictures of cars you would include in
your database application):
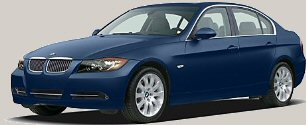 |
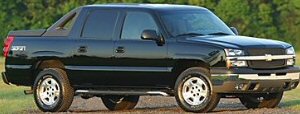 |
BMW: 335i |
Chevrolet Avalanche |
 |
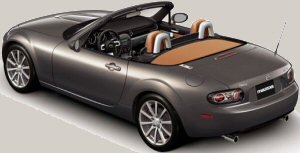 |
Honda Accord |
Mazda Miata |
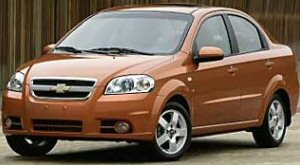 |
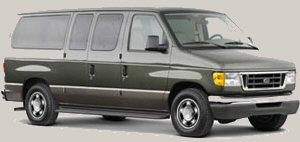 |
Chevrolet Aveo |
Ford E150XL |
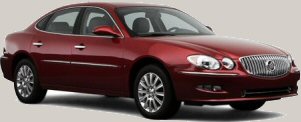 |
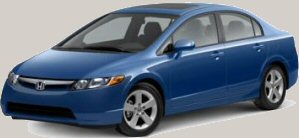 |
Buick Lacrosse |
Honda Civic |
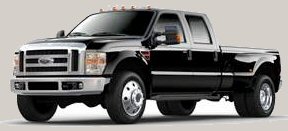 |
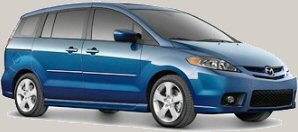 |
Ford F-150 |
Mazda Mazda5 |
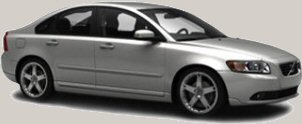 |
 |
Volvo S40 |
Land Rover LR3 |
- Return to your programming environment
- To add a new form to the application, in the Solution Explorer, right-click
BethesdaCarRental1
-> Add -> Windows Forms
- Set the Name to CarEditor and click Add
- Design the form as follows:
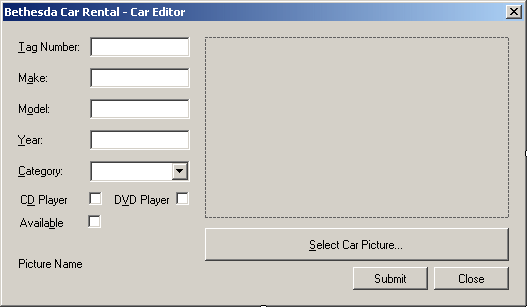 |
Control |
Text |
Name |
Other Properties |
Label |
Text # |
|
|
TextBox |
|
txtTagNumber |
|
Label |
Make: |
|
|
TextBox |
|
txtMake |
|
Label |
Model: |
|
|
TextBox |
|
txtModel |
|
Label |
Year: |
|
|
TextBox |
|
txtYear |
|
Label |
Category: |
|
|
ComboBox |
|
cboCategories |
DropDownStyle: DropDownList |
Items: |
Economy
Compact
Standard
Full Size
Mini Van
SUV
Truck
Van |
PictureBox |
|
pbxCar |
SizeMode: Zoom |
CheckBox |
CD Player |
chkCDPlayer |
CheckAlign: MiddleRight |
CheckBox |
DVD Player |
chkDVDPlayer |
CheckAlign: MiddleRight |
Button |
Select Car Picture... |
btnSelectPicture |
|
CheckBox |
Available |
chkAvailable |
CheckAlign: MiddleRight |
Label |
Picture Name |
lblPictureName |
|
Button |
Submit |
btnSubmit |
|
Button |
Close |
btnClose |
DialogResult: Cancel |
OpenFileDialog |
(Name): dlgOpen
Title: Select Item Picture
DefaultExt: jpg
Filter: JPEG Files (*.jpg,*.jpeg)|*.jpg|GIF Files (*.gif)|*.gif|Bitmap Files (*.bmp)|*.bmp|PNG Files (*.png)|*.png |
Form |
|
|
FormBorderStyle: FixedDialog
MaximizeBox: False
MinimizeBox: False
ShowInTaskbar: False |
|
- Double-click the Select Car Picture button and implement its event as
follows:
Private Sub btnSelectPictureClick(ByVal sender As System.Object,
ByVal e As System.EventArgs)
Handles btnSelectPicture.Click
If dlgPicture.ShowDialog() = DialogResult.OK Then
lblPictureName.Text = dlgPicture.FileName
pbxCar.Image = Image.FromFile(lblPictureName.Text)
End If
End Sub
- To add a new class to the project, on the main menu, click Project -> Add Class...
- Set the Class Name to Car and click Add
- Change the file as follows:
<Serializable()> Public Class Car
Public Make As String
Public Model As String
Public Year As Integer
Public Category As String
Public HasCDPlayer As Boolean
Public HasDVDPlayer As Boolean
Public IsAvailable As Boolean
Public Sub New()
Make = ""
Model = ""
Year = 0
Category = ""
HasCDPlayer = False
HasDVDPlayer = False
IsAvailable = False
End Sub
Public Sub New(ByVal mk As String, ByVal mdl As String,
ByVal yr As Integer, ByVal cat As String,
ByVal cd As Boolean, ByVal dvd As Boolean,
ByVal avl As Boolean)
Make = mk
Model = mdl
Year = yr
Category = cat
HasCDPlayer = cd
HasDVDPlayer = dvd
IsAvailable = avl
End Sub
End Class
- To add a new class to the project, on the main menu, click Project -> Add
Class...
- Set the Class Name to RentalOrder and press Enter
- Access the RentalOrder.vb file and change it as follows:
<Serializable()> Public Class RentalOrder
Public EmployeeNumber As String
REM The following flag will allow us to update/know whether
REM * The car is currently being used by the customer.
REM That is, if the car has been picked up by the customer
REM * The customer has brought the car back
Public OrderStatus As String
REM Because every car has a tag number
REM and that tag number will not change
REM during the lifetime of a car (while the car remains with our
REM car rental company, we will
REM use only the tag number here.
REM The other pieces of information will
REM be retrieved from the list of cars
Public CarTagNumber As String
REM We will identify the customer by the number
REM and the name on his or her driver's license
Public CustomerDrvLicNbr As String
Public CustomerName As String
REM Although he or she may keep the same driver's
REM license number for a lifetime, a customer who
REM rents cars at different times with this company
REM over months or years may have different addresses.
REM Therefore, although we will primarily retrieve the
REM customer's address from the list of customers,
REM we will give the clerk the ability to change this
REM information on a rental order. This means that the
REM same customer could have different information on
REM different rental orders.
REM For this reason, each rental order will its own set
REM of these pieces of information
Public CustomerAddress As String
Public CustomerCity As String
Public CustomerState As String
Public CustomerZIPCode As String
Public CarCondition As String
Public TankLevel As String
REM This information will be entered when the car is being rented
Public MileageStart As Integer
REM This information will be entered when the car is brought back
Public MileageEnd As Integer
REM This information will be entered when the car is being rented
Public DateStart As DateTime
REM This information will be entered when the car is brought back
Public DateEnd As DateTime
Public Days As Integer
REM This information will be entered when the car is being rented
Public RateApplied As Double
REM This calculation should occur when the car is brought back
Public SubTotal As Double
Public TaxRate As Double
Public TaxAmount As Double
Public OrderTotal As Double
End Class
- To add a new form to the project, in the Solution Explorer, right-click
BethesdaCarRenat1 -> Add -> Windows Form...
- Set the Name to OrderProcessing and press Enter
- Design the form as follows:
 |
Control |
Text |
Name |
Other Properties |
Label |
Processed By: |
|
AutoSize: False
BackColor: Gray
BorderStyle: FixedSingle
ForeColor: White
TextAlign: MiddleLeft |
Label |
Employee #: |
|
|
MaskedTextBox |
|
txtEmployeeNumber |
Mask: 00-000 |
Label |
Employee Name: |
|
|
TextBox |
|
txtEmployeeName |
|
Label |
Processed For |
|
AutoSize: False
BackColor: Gray
BorderStyle: FixedSingle
ForeColor: White
TextAlign: MiddleLeft |
Label |
Driver's Lic #: |
|
|
TextBox |
|
txtDrvLicNumber |
|
Label |
Cust Name: |
|
|
TextBox |
|
txtCustomerName |
|
Label |
Address: |
|
|
TextBox |
|
txtCustomerAddress |
|
Label |
City: |
|
|
TextBox |
|
txtCustomerCity |
|
Label |
State: |
|
|
ComboBox |
|
cbxCustomerStates |
DropDownStyle: DropDownList
Sorted: True
Items: AL, AK, AZ, AR, CA, CO, CT, DE, DC, FL, GA, HI, ID, IL,
IN, IA, KS, KY, LA, ME, MD, MA, MI, MN, MS, MO, MT, NE, NV, NH,
NJ, NM, NY, NC, ND, OH, OK, OR, PA, RI, SC, SD, TN, TX, UT, VT,
VA, WA, WV, WI, WY |
Label |
ZIP Code |
|
|
TextBox |
|
txtCustomerZIPCode |
|
Label |
Car Selected |
|
AutoSize: False
BackColor: Gray
BorderStyle: FixedSingle
ForeColor: White
TextAlign: MiddleLeft |
Label |
Tag Number: |
|
|
TextBox |
|
txtTagNumber |
|
Label |
Car Condition: |
|
|
ComboBox |
|
cbxCarConditions |
Sorted: True
Items:
Needs Repair
Drivable
Excellent |
Label |
Make: |
|
|
TextBox |
|
txtMake |
|
Label |
Model: |
|
|
TextBox |
|
txtModel |
|
Label |
Year: |
|
|
TextBox |
|
txtCarYear |
|
label |
Tank Level: |
|
|
ComboBox |
|
cbxTankLevels |
Empty
1/4 Empty
1/2 Full
3/4 Full
Full |
Label |
Mileage Start: |
|
|
TextBox |
|
txtMileageStart |
TextAlign: Right |
Label |
Mileage End: |
|
|
TextBox |
|
txtMileageEnd |
TextAlign: Right |
Label |
Order Timing |
|
AutoSize: False
BackColor: Gray
BorderStyle: FixedSingle
ForeColor: White
TextAlign: MiddleLeft |
Label |
Start Date: |
|
|
DateTimePicker |
|
dtpStartDate |
|
Label |
End Date: |
|
|
DateTimePicker |
|
dtpEndDate |
|
Label |
Days: |
|
|
TextBox |
0 |
txtDays |
TextAlign: Right |
Label |
Order Status |
|
|
ComboBox |
|
cbxOrderStatus |
Items:
Car On Road
Car Returned
Order Reserved |
Label |
Order Evaluation |
|
AutoSize: False
BackColor: Gray
BorderStyle: FixedSingle
ForeColor: White
TextAlign: MiddleLeft |
Label |
Rate Applied: |
|
|
TextBox |
0.00 |
txtRateApplied |
TextAlign: Right |
Button |
Rental Rates |
btnRentalRates |
|
Label |
Sub-Total: |
|
|
TextBox |
0.00 |
txtSubTotal |
TextAlign: Right |
Button |
Calculate |
btnCalculate |
|
Label |
Tax Rate: |
|
|
TextBox |
7.75 |
txtTaxRate |
TextAlign: Right |
Label |
% |
|
|
Button |
Save |
btnSave |
|
Label |
Tax Amount: |
|
|
TextBox |
0.00 |
txtTaxAmount |
TextAlign: Right |
Button |
Print... |
btnPrint |
|
Label |
Order Total: |
|
|
TextBox |
0.00 |
txtOrderTotal |
TextAlign: Right |
Button |
Print Preview... |
btnPrintPreview |
|
Label |
Receipt #: |
|
|
TextBox |
0 |
txtReceiptNumber |
|
Button |
Open |
btnOpen |
|
Button |
New Rental Order/Reset |
btnNewRentalOrder |
|
Button |
Close |
btnClose |
|
|
- Right-click the form and click View Code
- In the Class Name combo box, select dtpStartDate
- In the Method Name combo box, selectValueChanged and implement the event as follows:
Private Sub dtpStartDateValueChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles dtpStartDate.ValueChanged
dtpEndDate.Value = dtpStartDate.Value
End Sub
- In the Class Name combo box, select dtpEndDate
- In the Method Name combo box, select ValueChanged and implement the event as follows:
REM This event approximately evaluates the number of days as a
REM difference between the end date and the starting date
Private Sub dtpEndDateValueChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles dtpEndDate.ValueChanged
Dim Days As Integer
Dim DateStart As DateTime = dtpStartDate.Value
Dim DateEnd As DateTime = dtpEndDate.Value
REM Let's calculate the difference in days
Dim tme As TimeSpan = DateEnd - DateStart
Days = tme.Days
REM If the customer returns the car the same day,
REM we consider that the car was rented for 1 day
If Days = 0 Then Days = 1
txtDays.Text = Days
REM At any case, we will let the clerk specify the actual number of days
End Sub
- In the Class Name combo box, select btnRentalRates
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnRentalRatesClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRentalRates.Click
Dim RateViewer As RentalRates = New RentalRates
RateViewer.Show()
End Sub
- In the Class Name combo box, select btnCalculate
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCalculateClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCalculate.Click
Dim Days As Integer
Dim RateApplied As Double
Dim SubTotal As Double
Dim TaxRate As Double
Dim TaxAmount As Double
Dim OrderTotal As Double
Try
Days = CInt(txtDays.Text)
Catch ex As FormatException
MsgBox("Invalid Number of Days")
End Try
Try
RateApplied = CDbl(txtRateApplied.Text)
Catch ex As FormatException
MsgBox("Invalid Amount for Rate Applied")
End Try
SubTotal = Days * RateApplied
txtSubTotal.Text = FormatNumber(SubTotal)
Try
TaxRate = CDbl(txtTaxRate.Text)
Catch ex As FormatException
MsgBox("Invalid Tax Rate")
End Try
TaxAmount = SubTotal * TaxRate / 100
txtTaxAmount.Text = FormatNumber(TaxAmount)
OrderTotal = SubTotal + TaxAmount
txtOrderTotal.Text = FormatNumber(OrderTotal)
End Sub
- Return to the Order Processing form
- From the Printing section of the Toolbox, click PrintDocument and click
the form
- In the Properties window, set its (Name) to docPrint and press Enter
- Under the form, double-click docPrint and implement its event as follows:
Private Sub docPrintPrintPage(ByVal sender As Object,
ByVal e As System.Drawing.Printing.PrintPageEventArgs)
Handles docPrint.PrintPage
e.Graphics.DrawLine(New Pen(Color.Black, 2), 80, 90, 750, 90)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 80, 93, 750, 93)
Dim strDisplay As String = "Bethesda Car Rental"
Dim fntString As System.Drawing.Font =
New Font("Times New Roman", 28,
FontStyle.Bold)
e.Graphics.DrawString(strDisplay, fntString,
Brushes.Black, 240, 100)
strDisplay = "Car Rental Order"
fntString = New System.Drawing.Font("Times New Roman", 22,
FontStyle.Regular)
e.Graphics.DrawString(strDisplay, fntString,
Brushes.Black, 320, 150)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 80, 187, 750, 187)
e.Graphics.DrawLine(New Pen(Color.Black, 2), 80, 190, 750, 190)
fntString = New System.Drawing.Font("Times New Roman", 12,
FontStyle.Bold)
e.Graphics.DrawString("Receipt #: ", fntString,
Brushes.Black, 100, 220)
fntString = New System.Drawing.Font("Times New Roman", 12,
FontStyle.Regular)
e.Graphics.DrawString(txtReceiptNumber.Text, fntString,
Brushes.Black, 260, 220)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 100, 240, 380, 240)
fntString = New System.Drawing.Font("Times New Roman", 12,
FontStyle.Bold)
e.Graphics.DrawString("Processed By: ", fntString,
Brushes.Black, 420, 220)
fntString = New System.Drawing.Font("Times New Roman", 12,
FontStyle.Regular)
e.Graphics.DrawString(txtEmployeeName.Text, fntString,
Brushes.Black, 550, 220)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 420, 240, 720, 240)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.FillRectangle(Brushes.Gray,
New Rectangle(100, 260, 620, 20))
e.Graphics.DrawRectangle(Pens.Black,
New Rectangle(100, 260, 620, 20))
e.Graphics.DrawString("Customer", fntString,
Brushes.White, 100, 260)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Driver's License #: ", fntString,
Brushes.Black, 100, 300)
e.Graphics.DrawString("Name: ", fntString,
Brushes.Black, 420, 300)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtDrvLicNumber.Text, fntString,
Brushes.Black, 260, 300)
e.Graphics.DrawString(txtCustomerName.Text, fntString,
Brushes.Black, 540, 300)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 100, 320, 720, 320)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Address: ", fntString,
Brushes.Black, 100, 330)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtCustomerAddress.Text, fntString,
Brushes.Black, 260, 330)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 100, 350, 720, 350)
strDisplay = txtCustomerCity.Text & " " &
cbxCustomerStates.Text & " " &
txtCustomerZIPCode.Text
fntString = New System.Drawing.Font("Times New Roman",
12, FontStyle.Regular)
e.Graphics.DrawString(strDisplay, fntString,
Brushes.Black, 260, 360)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 260, 380, 720, 380)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.FillRectangle(Brushes.Gray,
New Rectangle(100, 410, 620, 20))
e.Graphics.DrawRectangle(Pens.Black,
New Rectangle(100, 410, 620, 20))
e.Graphics.DrawString("Car Information", fntString,
Brushes.White, 100, 410)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Tag #: ", fntString,
Brushes.Black, 100, 450)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtTagNumber.Text, fntString,
Brushes.Black, 260, 450)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 100, 470, 380, 470)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Year: ", fntString,
Brushes.Black, 420, 450)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtCarYear.Text, fntString,
Brushes.Black, 530, 450)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 420, 470, 720, 470)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Make: ", fntString,
Brushes.Black, 100, 480)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtMake.Text, fntString,
Brushes.Black, 260, 480)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 100, 500, 380, 500)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Model: ", fntString,
Brushes.Black, 420, 480)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtModel.Text, fntString,
Brushes.Black, 530, 480)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 420, 500, 720, 500)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Car Condition: ", fntString,
Brushes.Black, 100, 510)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(cbxCarConditions.Text, fntString,
Brushes.Black, 260, 510)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 100, 530, 380, 530)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Tank Level: ", fntString,
Brushes.Black, 420, 510)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(cbxTankLevels.Text, fntString,
Brushes.Black, 530, 510)
e.Graphics.DrawLine(New Pen(Color.Black, 1), 420, 530, 720, 530)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Mileage Start:", fntString,
Brushes.Black, 100, 540)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtMileageStart.Text, fntString,
Brushes.Black, 260, 540)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
100, 560, 380, 560)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Mileage End:", fntString,
Brushes.Black, 420, 540)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtMileageEnd.Text, fntString,
Brushes.Black, 530, 540)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
420, 560, 720, 560)
e.Graphics.FillRectangle(Brushes.Gray,
New Rectangle(100, 590, 620, 20))
e.Graphics.DrawRectangle(Pens.Black,
New Rectangle(100, 590, 620, 20))
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Order Timing Information", fntString,
Brushes.White, 100, 590)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Start Date:", fntString,
Brushes.Black, 100, 620)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(dtpStartDate.Value.ToString("D"),
fntString, Brushes.Black, 260, 620)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
100, 640, 720, 640)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("End Date:", fntString,
Brushes.Black, 100, 650)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(dtpEndDate.Value.ToString("D"),
fntString, Brushes.Black, 260, 650)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
100, 670, 520, 670)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Days:", fntString,
Brushes.Black, 550, 650)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtDays.Text, fntString,
Brushes.Black, 640, 650)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
550, 670, 720, 670)
e.Graphics.FillRectangle(Brushes.Gray,
New Rectangle(100, 700, 620, 20))
e.Graphics.DrawRectangle(Pens.Black,
New Rectangle(100, 700, 620, 20))
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Order Evaluation", fntString,
Brushes.White, 100, 700)
Dim fmtString As StringFormat = New StringFormat
fmtString.Alignment = StringAlignment.Far
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Rate Applied:", fntString,
Brushes.Black, 100, 740)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtRateApplied.Text, fntString,
Brushes.Black, 300, 740, fmtString)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
100, 760, 380, 760)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Tax Rate:", fntString,
Brushes.Black, 420, 740)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtTaxRate.Text, fntString,
Brushes.Black, 640, 740, fmtString)
e.Graphics.DrawString("%", fntString,
Brushes.Black, 640, 740)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
420, 760, 720, 760)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Sub-Total:", fntString,
Brushes.Black, 100, 770)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtSubTotal.Text, fntString,
Brushes.Black, 300, 770, fmtString)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
100, 790, 380, 790)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Tax Amount:", fntString,
Brushes.Black, 420, 770)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtTaxAmount.Text, fntString,
Brushes.Black, 640, 770, fmtString)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
420, 790, 720, 790)
fntString = New Font("Times New Roman", 12, FontStyle.Bold)
e.Graphics.DrawString("Order Total:", fntString,
Brushes.Black, 420, 800)
fntString = New Font("Times New Roman", 12, FontStyle.Regular)
e.Graphics.DrawString(txtOrderTotal.Text, fntString,
Brushes.Black, 640, 800, fmtString)
e.Graphics.DrawLine(New Pen(Color.Black, 1),
420, 820, 720, 820)
End Sub
- Return to the Order Processing form
- From the Printing section of the Toolbox, click PrintDialog and click the
form
- In the Properties window, change its Name to dlgPrint
- Still in the Properties windows, set its Document property to docPrint
- On the Order Processing form, double-click the Print button and implement its event as
follows:
Private Sub btnPrintClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnPrint.Click
If dlgPrint.ShowDialog() = DialogResult.OK Then
docPrint.Print()
End If
End Sub
- Return to the Order Processing form
- From the Printing section of the Toolbox, click PrintPreviewDialog and
click the form
- In the Properties window, change its (Name) to dlgPrintPreview
- Still in the Properties windows, set its Document property to docPrint
- On the Order Processing form, double-click the Print Preview button
- Implement the event as follows:
Private Sub btnPrintPreviewClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnPrintPreview.Click
dlgPrintPreview.ShowDialog()
End Sub
- In the Solution Explorer, right-click Form1.vb and click Rename
- Type Central.vb and press Enter twice
- Design the form as follows:
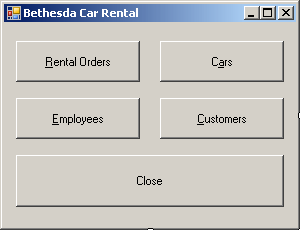 |
Control |
Text |
Name |
Button |
Customers &Rental Orders |
btnRentalOrders |
Button |
C&ars |
btnCars |
Button |
&Employees |
btnEmployees |
Button |
&Customers |
btnCustomers |
Button |
C&lose |
btnClose |
|
- Double-click the Rental Orders button and implement its event as follows:
Private Sub btnRentalOrdersClick(ByVal sender As System.Object,
ByVal e As System.EventArgs)
Handles btnRentalOrders.Click
Dim dlgOrder As OrderProcessing = New OrderProcessing
dlgOrder.ShowDialog()
End Sub
- In the Class Name combo box, select btnCars
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnCarsClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCars.Click
Dim dlgCars As CarEditor = New CarEditor
dlgCars.ShowDialog()
End Sub
- In the Class Name combo box, select btnEmployees
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnEmployeesClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnEmployees.Click
Dim dlgEmployees As Employees = New Employees
dlgEmployees.ShowDialog()
End Sub
- In the Class Name combo box, select btnCustomers
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCustomersClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCustomers.Click
Dim dlgCustomers As Customers = New Customers
dlgCustomers.ShowDialog()
End Sub
- In the Class Name combo box, select btnClose
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCloseClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnClose.Click
Close()
End Sub
- Save all
- In the Solution Explorer, right-click CarEditor.vb and click View Code
- In the top section of the file, import the IO and the Binary namespace
then declare a variable as follows:
Imports System.IO
Imports System.Runtime.Serialization.Formatters.Binary
Public Class CarEditor
Private ListOfCars As Dictionary(Of String, Car)
- In the Class Name combo box, select (CarEditor Events)
- In the Method Name combo box, select Load and implement the event as follows:
Private Sub CarEditorLoad(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles Me.Load
Dim vehicle As Car = New Car
Dim StreamCars As FileStream
Dim FormatterCars As BinaryFormatter = New BinaryFormatter
REM This is the file that holds the list of items
Dim Filename As String = "C:\Bethesda Car Rental\Cars.crs"
lblPictureName.Text = "."
If File.Exists(Filename) Then
StreamCars = New FileStream(Filename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
REM Retrieve the list of cars
ListOfCars = CType(FormatterCars.Deserialize(StreamCars),
Dictionary(Of String, Car))
Finally
StreamCars.Close()
End Try
Else
ListOfCars = New Dictionary(Of String, Car)
End If
End Sub
- In the Solution Explorer, right-click OrderProcessing.vb and click View
Code
- Make the following changes:
Imports System.IO
Imports System.Runtime.Serialization.Formatters.Binary
Public Class OrderProcessing
Private ReceiptNumber As Integer
Private ListOfRentalOrders As SortedList(Of Integer, RentalOrder)
- Save all
- In the Solution Explorer, right-click CarEditor.vb and click View Code
- In the Class Name combo box, select btnSubmit
- In the Method Name combo box, select Click and implement the Click event as follows:
Private Sub btnSubmitClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnSubmit.Click
Dim Vehicle As Car = New Car
Dim StreamCars As FileStream
Dim FormatterCars As BinaryFormatter = New BinaryFormatter
Directory.CreateDirectory("C:\Bethesda Car Rental")
Dim strFilename As String = "C:\Bethesda Car Rental\Cars.crs"
If txtTagNumber.Text.Length = 0 Then
MsgBox("You must enter the car's tag number")
Exit Sub
End If
If txtMake.Text.Length = 0 Then
MsgBox("You must specify the car's manufacturer")
Exit Sub
End If
If txtModel.Text.Length = 0 Then
MsgBox("You must enter the model of the car")
Exit Sub
End If
If txtYear.Text.Length = 0 Then
MsgBox("You must enter the year of the car")
Exit Sub
End If
REM Create a car
Vehicle.Make = txtMake.Text
Vehicle.Model = txtModel.Text
Vehicle.Year = CInt(txtYear.Text)
Vehicle.Category = cbxCategories.Text
Vehicle.HasCDPlayer = chkCDPlayer.Checked
Vehicle.HasDVDPlayer = chkDVDPlayer.Checked
Vehicle.IsAvailable = chkAvailable.Checked
REM Call the Add method of our collection class to add the car
ListOfCars.Add(txtTagNumber.Text, Vehicle)
REM Save the list
StreamCars = New FileStream(strFilename,
FileMode.Create,
FileAccess.Write,
FileShare.Write)
Try
FormatterCars.Serialize(StreamCars, ListOfCars)
If lblPictureName.Text.Length <> 0 Then
Dim PictureFile As FileInfo = New FileInfo(lblPictureName.Text)
PictureFile.CopyTo("C:\Bethesda Car Rental\" &
txtTagNumber.Text &
PictureFile.Extension)
End If
txtTagNumber.Text = ""
txtMake.Text = ""
txtModel.Text = ""
txtYear.Text = ""
cbxCategories.Text = "Economy"
chkCDPlayer.Checked = False
chkDVDPlayer.Checked = False
chkAvailable.Checked = False
lblPictureName.Text = "."
pbxCar.Image = Nothing
Finally
StreamCars.Close()
End Try
End Sub
- In the Solution Explorer, right-click Customers.vb and click View Code
- Make the following changes:
Imports System.IO
Imports System.Runtime.Serialization.Formatters.Binary
Public Class Customers
Private ListOfCustomers As Dictionary(Of String, Customer)
Private Sub ShowCustomers()
End Sub
Private Sub CustomersLoad(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles Me.Load
Dim StreamCustomers As FileStream
ListOfCustomers = New Dictionary(Of String, Customer)
Dim FormatterCustomers As BinaryFormatter = New BinaryFormatter
REM This is the file that holds the list of parts
Dim strFilename As String = "C:\Bethesda Car Rental\Customers.crc"
If File.Exists(strFilename) Then
StreamCustomers = New FileStream(strFilename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
REM Retrieve the list of employees from file
ListOfCustomers =
CType(FormatterCustomers.Deserialize(StreamCustomers),
Dictionary(Of String, Customer))
Finally
StreamCustomers.Close()
End Try
End If
ShowCustomers()
End Sub
End Class
- In the Class Name combo box, select btnNewCustomer
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnNewCustomerClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnNewCustomer.Click
Dim bcrStream As FileStream
Dim bcrBinary As BinaryFormatter = New BinaryFormatter
Dim Editor As CustomerEditor = New CustomerEditor
Directory.CreateDirectory("C:\Bethesda Car Rental")
If Editor.ShowDialog() = DialogResult.OK Then
If Editor.txtDrvLicNbr.Text = "" Then
MsgBox("You must provide the driver's " &
"license number of the customer")
Exit Sub
End If
If Editor.txtFullName.Text = "" Then
MsgBox("You must provide the employee's full name")
Exit Sub
End If
Dim strFilename As String = "C:\Bethesda Car Rental\Customers.crc"
Dim Cust As Customer = New Customer
Cust.FullName = Editor.txtFullName.Text
Cust.Address = Editor.txtAddress.Text
Cust.City = Editor.txtCity.Text
Cust.State = Editor.cbxStates.Text
Cust.ZIPCode = Editor.txtZIPCode.Text
ListOfCustomers.Add(Editor.txtDrvLicNbr.Text, Cust)
bcrStream = New FileStream(strFilename,
FileMode.Create,
FileAccess.Write,
FileShare.Write)
bcrBinary.Serialize(bcrStream, ListOfCustomers)
bcrStream.Close()
ShowCustomers()
End If
End Sub
- In the Solution Explorer, right-click Employees.vb and click View Code
- Change the file as follows:
Imports System.IO
Imports System.Runtime.Serialization.Formatters.Binary
Public Class Employees
Private ListOfEmployees As SortedDictionary(Of String, Employee)
Private Sub ShowEmployees()
End Sub
Private Sub EmployeesLoad(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles Me.Load
Dim StreamEmployees As FileStream
ListOfEmployees = New SortedDictionary(Of String, Employee)
Dim FormatterEmployees As BinaryFormatter = New BinaryFormatter
REM This is the file that holds the list of parts
Dim strFilename As String = "C:\Bethesda Car Rental\Employees.cre"
If File.Exists(strFilename) Then
StreamEmployees = New FileStream(strFilename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
REM Retrieve the list of employees from file
ListOfEmployees =
CType(FormatterEmployees.Deserialize(StreamEmployees),
SortedDictionary(Of String, Employee))
Finally
StreamEmployees.Close()
End Try
End If
ShowEmployees()
End Sub
End Class
- In the Class Name combo box, select btnNewEmployee
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnNewEmployeeClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnNewEmployee.Click
Dim Editor As EmployeeEditor = New EmployeeEditor
Dim bcrBinary As BinaryFormatter = New BinaryFormatter
REM If this folder doesn't exist, create it
Directory.CreateDirectory("C:\Bethesda Car Rental")
REM Display the Employee Editor dialog box
REM and find out if the user clicked OK after using it
If Editor.ShowDialog() = DialogResult.OK Then
REM If the user didn't provide an employee number
REM don't do anything
If Editor.txtEmployeeNumber.Text = "" Then
MsgBox("You must provide an employee number")
Exit Sub
End If
REM If the user didn't provide a last name,
REM don't do anything
If Editor.txtLastName.Text = "" Then
MsgBox("You must provide the employee's last name")
Exit Sub
End If
REM Ger a reference to the Employees file if it exists
Dim strFilename As String = "C:\Bethesda Car Rental\Employees.cre"
REM Get the values that the user entered in the dialog box
Dim Empl As Employee = New Employee
REM Create an employee from those values
Empl.FirstName = Editor.txtFirstName.Text
Empl.LastName = Editor.txtLastName.Text
Empl.Title = Editor.txtTitle.Text
Empl.HourlySalary = CDbl(Editor.txtHourlySalary.Text)
REM Add the employee to the main list
ListOfEmployees.Add(Editor.txtEmployeeNumber.Text, Empl)
REM Save the current list of employees
Dim bcrStream As FileStream = New FileStream(strFilename,
FileMode.Create,
FileAccess.Write,
FileShare.Write)
bcrBinary.Serialize(bcrStream, ListOfEmployees)
bcrStream.Close()
REM Display the list of employees
ShowEmployees()
End If
End Sub
- In the Solution Explorer, right-click OrderProcessing.vb and click View
Code
- In the Class Name combo box, select txtDrvLicNumber
- In the Method Name combo box, select Leave and implement the event as follows:
Private Sub txtDrvLicNumberLeave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtDrvLicNumber.Leave
Dim Renter As Customer
Dim strDrvLicNumber As String = txtDrvLicNumber.Text
Dim FormatterCustomers As BinaryFormatter = New BinaryFormatter
Dim ListOfCustomers As Dictionary(Of String, Customer) =
New Dictionary(Of String, Customer)
REM Make sure the user entered a driver's license number
If strDrvLicNumber.Length = 0 Then
MsgBox("You must enter the renter's " &
"driver's license number")
txtDrvLicNumber.Focus()
Exit Sub
End If
REM Get a reference to the file that holds the list of customers
Dim strFilename As String = "C:\Bethesda Car Rental\Customers.crc"
REM Find out if that file exists
If File.Exists(strFilename) Then
REM If there is a file of customers, open it
Dim StreamCustomers As FileStream = New FileStream(strFilename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
REM Retrieve the customers from the file and store
REM them in the ListOfCustomers variable
ListOfCustomers =
CType(FormatterCustomers.Deserialize(StreamCustomers),
Dictionary(Of String, Customer))
REM Check the list of customers to
REM find out if there is a customer
REM who has the driver's license number that the user entered
If ListOfCustomers.ContainsKey(strDrvLicNumber) = True Then
REM If so, get the information about that customer
REM and display that information on
REM the Order Processing form
For Each Cust As KeyValuePair(Of String, Customer)
In ListOfCustomers
If Cust.Key = strDrvLicNumber Then
Renter = Cust.Value
txtCustomerName.Text = Renter.FullName
txtCustomerAddress.Text = Renter.Address
txtCustomerCity.Text = Renter.City
cbxCustomerStates.Text = Renter.State
txtCustomerZIPCode.Text = Renter.ZIPCode
End If
Next
Else
REM If there is no customer with
REM that driver's license number
REM there is nothing to display...
txtCustomerName.Text = ""
txtCustomerAddress.Text = ""
txtCustomerCity.Text = ""
cbxCustomerStates.Text = ""
txtCustomerZIPCode.Text = ""
REM ... and let the user know
MsgBox("There is no customer with that driver's " &
"license number in our database")
Exit Sub
End If
Finally
StreamCustomers.Close()
End Try
End If
End Sub
- Access the Employees.vb file and implement the ShowEmployees() procedure as follows:
Private Sub ShowEmployees()
If ListOfEmployees.Count = 0 Then
Exit Sub
End If
REM Before displaying the employees, empty the list view
lvwEmployees.Items.Clear()
REM This variable will allow us to identify the odd and even indexes
Dim Counter As Integer = 1
REM Use the KeyValuePair class to visit each key/value item
For Each kvp As KeyValuePair(Of String, Employee) In ListOfEmployees
Dim lviEmployee As ListViewItem = New ListViewItem(kvp.Key)
Dim empl As Employee = kvp.Value
lviEmployee.SubItems.Add(empl.FirstName)
lviEmployee.SubItems.Add(empl.LastName)
lviEmployee.SubItems.Add(empl.FullName)
lviEmployee.SubItems.Add(empl.Title)
lviEmployee.SubItems.Add(FormatNumber(empl.HourlySalary))
If Counter Mod 2 = 0 Then
lviEmployee.BackColor = Color.FromArgb(255, 128, 0)
lviEmployee.ForeColor = Color.White
Else
lviEmployee.BackColor = Color.FromArgb(128, 64, 64)
lviEmployee.ForeColor = Color.White
End If
lvwEmployees.Items.Add(lviEmployee)
Counter = Counter + 1
Next
End Sub
- Access the Customers.vb file and implement the ShowCustomers() method as
follows:
Private Sub ShowCustomers()
Dim Counter As Integer
If ListOfCustomers.Count = 0 Then
Exit Sub
End If
lvwCustomers.Items.Clear()
Counter = 1
For Each kvp As KeyValuePair(Of String, Customer) In ListOfCustomers
Dim lviCustomer As ListViewItem = New ListViewItem(kvp.Key)
Dim Cust As Customer = kvp.Value
lviCustomer.SubItems.Add(Cust.FullName)
lviCustomer.SubItems.Add(Cust.Address)
lviCustomer.SubItems.Add(Cust.City)
lviCustomer.SubItems.Add(Cust.State)
lviCustomer.SubItems.Add(Cust.ZIPCode)
If Counter Mod 2 = 0 Then
lviCustomer.BackColor = Color.Navy
lviCustomer.ForeColor = Color.White
Else
lviCustomer.BackColor = Color.Blue
lviCustomer.ForeColor = Color.White
End If
lvwCustomers.Items.Add(lviCustomer)
Counter = Counter + 1
Next
End Sub
- Execute the application
- Click the Cars button and create the
cars
- Close the Car Editor form
- Click the Customers button then click the New Customer button continually
and create a few customers as follows:
Driver's Lic. # |
State |
Full Name |
Address |
City |
ZIP Code |
M-505-862-575 |
MD |
Lynda Melman |
4277 Jamison Avenue |
Silver Spring |
20904 |
379-82-7397 |
DC |
John Villard |
108 Hacken Rd NE |
Washington |
20012 |
J-938-928-274 |
MD |
Chris Young |
8522 Aulage Street |
Rockville |
20852 |
497-22-0614 |
PA |
Pamela Ulmreck |
12075 Famina Rd |
Blain |
17006 |
922-71-8395 |
VA |
Helene Kapsco |
806 Hyena Drive |
Alexandria |
22231 |
C-374-830-422 |
MD |
Hermine Crasson |
6255 Old Georgia Ave |
Silver Spring |
20910 |
836-55-2279 |
NY |
Alan Pastore |
4228 Talion Street |
Amherst |
14228 |
397-59-7487 |
TN |
Phillis Buster |
724 Cranston Circle |
Knoxville |
37919 |
115-80-2957 |
FL |
Elmus Krazucki |
808 Rasters Ave |
Orlando |
32810 |
294-90-7744 |
VA |
Helena Weniack |
10448 Great Pollard Hwy |
Arlington |
22232 |
|
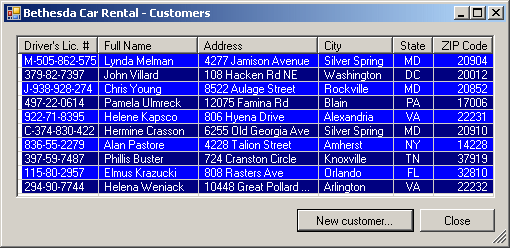 |
- Close the Customers form
- On the Central form, click the Employees button
- Create a few employees as follows:
Employee # |
First Name |
Last Name |
Title |
Hourly Salary |
62-845 |
Patricia |
Katts |
General Manager |
42.25 |
92-303 |
Henry |
Larson |
Sales Representative |
12.50 |
25-947 |
Gertrude |
Monay |
Sales Representative |
14.05 |
73-947 |
Helene |
Sandt |
Intern |
8.85 |
40-508 |
Melanie |
Karron |
Sales Representative |
12.75 |
22-580 |
Ernest |
Chisen |
Sales Manager |
22.95 |
20-308 |
Melissa |
Roberts |
Administrative Assistant |
15.45 |
|
 |
- Notice that the list of employees is automatically sorted based on the
employees numbers.
Close the forms and return to your programming environment
- In the Solution Explorer, right-click OrderProcessing.vb and click View
Code
- In the Class Name combo box, select btnNewRentalOrder
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnNewRentalOrderClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnNewRentalOrder.Click
Dim StreamRentalOrders As FileStream
txtEmployeeNumber.Text = ""
txtEmployeeName.Text = ""
txtDrvLicNumber.Text = ""
txtCustomerName.Text = ""
txtCustomerAddress.Text = ""
txtCustomerCity.Text = ""
cbxCustomerStates.Text = ""
txtCustomerZIPCode.Text = ""
txtTagNumber.Text = ""
cbxCarConditions.Text = ""
txtMake.Text = ""
txtModel.Text = ""
txtCarYear.Text = ""
cbxTankLevels.Text = "Empty"
txtMileageStart.Text = "0"
txtMileageEnd.Text = "0"
dtpStartDate.Value = DateTime.Today
dtpEndDate.Value = DateTime.Today
txtDays.Text = ""
cbxOrderStatus.Text = ""
txtRateApplied.Text = "0.00"
txtSubTotal.Text = "0.00"
txtTaxRate.Text = "7.75"
txtTaxAmount.Text = "0.00"
txtOrderTotal.Text = "0.00"
Dim FormatterRentalOrders As BinaryFormatter = New BinaryFormatter()
Dim Filename As String = "C:\Bethesda Car Rental\RentalOrders.cro"
If File.Exists(Filename) Then
StreamRentalOrders = New FileStream(Filename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
ListOfRentalOrders =
CType(FormatterRentalOrders.Deserialize(StreamRentalOrders),
SortedList(Of Integer, RentalOrder))
ReceiptNumber =
ListOfRentalOrders.Keys(ListOfRentalOrders.Count - 1) + 1
Finally
StreamRentalOrders.Close()
End Try
Else
ReceiptNumber = 100001
ListOfRentalOrders = New SortedList(Of Integer, RentalOrder)
End If
txtReceiptNumber.Text = ReceiptNumber
End Sub
- In the Class name combo box, select (OrderProcessing Events)
- In the Method Name combo box, select Load and implement the event as follows:
Private Sub OrderProcessingLoad(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles Me.Load
btnNewRentalOrderClick(sender, e)
End Sub
- Return to the Order Processing form
- In the Class Name combo box, select btnSave
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnSaveClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnSave.Click
If txtReceiptNumber.Text = "" Then
MsgBox("The receipt number is missing")
Exit Sub
End If
REM Don't save this rental order if we don't
REM know who processed it
If txtEmployeeNumber.Text = "" Then
MsgBox("You must enter the employee number or the " &
"clerk who processed this order.")
Exit Sub
End If
REM Don't save this rental order if we don't
REM know who is renting the car
If txtDrvLicNumber.Text = "" Then
MsgBox("You must specify the driver's license number " &
"of the customer who is renting the car")
Exit Sub
End If
REM Don't save the rental order if we don't
REM know what car is being rented
If txtTagNumber.Text = "" Then
MsgBox("You must enter the tag number " &
"of the car that is being rented")
Exit Sub
End If
REM Create a rental order based on the information on the form
Dim CurrentOrder As RentalOrder = New RentalOrder
CurrentOrder.EmployeeNumber = txtEmployeeNumber.Text
CurrentOrder.OrderStatus = cbxOrderStatus.Text
CurrentOrder.CarTagNumber = txtTagNumber.Text
CurrentOrder.CustomerDrvLicNbr = txtDrvLicNumber.Text
CurrentOrder.CustomerName = txtCustomerName.Text
CurrentOrder.CustomerAddress = txtCustomerAddress.Text
CurrentOrder.CustomerCity = txtCustomerCity.Text
CurrentOrder.CustomerState = cbxCustomerStates.Text
CurrentOrder.CustomerZIPCode = txtCustomerZIPCode.Text
CurrentOrder.CarCondition = cbxCarConditions.Text
CurrentOrder.TankLevel = cbxTankLevels.Text
Try
CurrentOrder.MileageStart = CInt(txtMileageStart.Text)
Catch ex As FormatException
MsgBox("Invalid mileage start value")
End Try
Try
CurrentOrder.MileageEnd = CInt(txtMileageEnd.Text)
Catch ex As FormatException
MsgBox("Invalid mileage End value")
End Try
Try
CurrentOrder.DateStart = dtpStartDate.Value;
Catch ex As FormatException
MsgBox("Invalid start date")
End Try
Try
CurrentOrder.DateEnd = dtpEndDate.Value;
Catch ex As FormatException
MsgBox("Invalid End date")
End Try
Try
CurrentOrder.Days = CInt(txtDays.Text)
Catch ex As FormatException
MsgBox("Invalid number of days")
End Try
Try
CurrentOrder.RateApplied = CDbl(txtRateApplied.Text)
Catch ex As FormatException
MsgBox("Invalid rate value")
End Try
CurrentOrder.SubTotal = CDbl(txtSubTotal.Text)
Try
CurrentOrder.TaxRate = CDbl(txtTaxRate.Text)
Catch ex As FormatException
MsgBox("Inavlid tax rate")
End Try
CurrentOrder.TaxAmount = CDbl(txtTaxAmount.Text)
CurrentOrder.OrderTotal = CDbl(txtOrderTotal.Text)
REM The rental order is ready
REM Get the receipt number from its text box
Try
ReceiptNumber = CInt(txtReceiptNumber.Text)
Catch ex As FormatException
MsgBox("You must provide a receipt number")
End Try
REM Get the list of rental orders and
REM check if there is already one with the current receipt number
REM If there is already a receipt number like that...
If ListOfRentalOrders.ContainsKey(ReceiptNumber) = True Then
REM Simply update its value
ListOfRentalOrders(ReceiptNumber) = CurrentOrder
Else
REM If there is no order with that receipt,
REM then create a new rental order
ListOfRentalOrders.Add(ReceiptNumber, CurrentOrder)
End If
REM The list of rental orders
Dim strFilename As String = "C:\Bethesda Car Rental\RentalOrders.cro"
Dim bcrStream As FileStream = New FileStream(strFilename,
FileMode.Create,
FileAccess.Write,
FileShare.Write)
Dim bcrBinary As BinaryFormatter = New BinaryFormatter
Try
bcrBinary.Serialize(bcrStream, ListOfRentalOrders)
Finally
bcrStream.Close()
End Try
End Sub
- In the Class Name combo box, select txtEmployeeNumber
- In the Method Name combo box, select Leave and
implement the event as follows:
Private Sub txtEmployeeNumberLeave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtEmployeeNumber.Leave
Dim Clerk As Employee = Nothing
Dim StreamEmployees As FileStream
Dim strEmployeeNumber As String = txtEmployeeNumber.Text
If strEmployeeNumber.Length = 0 Then
MsgBox("You must enter the employee's number")
txtEmployeeNumber.Focus()
Exit Sub
End If
Dim ListOfEmployees As SortedDictionary(Of String, Employee) =
New SortedDictionary(Of String, Employee)
Dim FormatterEmployees As BinaryFormatter = New BinaryFormatter
Dim strFilename As String = "C:\Bethesda Car Rental\Employees.cre"
If File.Exists(strFilename) Then
StreamEmployees = New FileStream(strFilename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
REM Retrieve the list of employees
ListOfEmployees =
CType(FormatterEmployees.Deserialize(StreamEmployees),
SortedDictionary(Of String, Employee))
If ListOfEmployees.TryGetValue(strEmployeeNumber, Clerk) Then
txtEmployeeName.Text = Clerk.FullName
Else
txtEmployeeName.Text = ""
MsgBox("There is no employee with that number")
Exit Sub
End If
Finally
StreamEmployees.Close()
End Try
End If
End Sub
- In the Class Name combo box, select txtTagNumber
- In the Method Name combo box, select Leave and
implement the event as follows:
Private Sub txtTagNumberLeave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtTagNumber.Leave
Dim SelectedCar As Car
Dim StreamCars As FileStream
Dim strTagNumber As String = txtTagNumber.Text
Dim ListOfCars As Dictionary(Of String, Car) =
New Dictionary(Of String, Car)
Dim FormatterCars As BinaryFormatter = New BinaryFormatter()
If strTagNumber.Length = 0 Then
MsgBox("You must enter the car's tag number")
txtTagNumber.Focus()
Exit Sub
End If
Dim strFilename As String = "C:\Bethesda Car Rental\Cars.crs"
If File.Exists(strFilename) Then
StreamCars = New FileStream(strFilename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
REM Retrieve the list of employees from file
ListOfCars = CType(FormatterCars.Deserialize(StreamCars),
Dictionary(Of String, Car))
If ListOfCars.TryGetValue(strTagNumber, SelectedCar) Then
txtMake.Text = SelectedCar.Make
txtModel.Text = SelectedCar.Model
txtCarYear.Text = SelectedCar.Year
Else
txtMake.Text = ""
txtModel.Text = ""
txtCarYear.Text = ""
MsgBox("There is no car with that tag " &
"number in our database")
Exit Sub
End If
Finally
StreamCars.Close()
End Try
End If
End Sub
- In the Class Name combo box, select btnOpen
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnOpenClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnOpen.Click
Dim Order As RentalOrder = Nothing
If txtReceiptNumber.Text = "" Then
MsgBox("You must enter a receipt number")
Exit Sub
End If
ReceiptNumber = CInt(txtReceiptNumber.Text)
If ListOfRentalOrders.TryGetValue(ReceiptNumber, Order) Then
txtEmployeeNumber.Text = Order.EmployeeNumber
txtEmployeeNumberLeave(sender, e)
cbxOrderStatus.Text = Order.OrderStatus
txtTagNumber.Text = Order.CarTagNumber
txtTagNumberLeave(sender, e)
txtDrvLicNumber.Text = Order.CustomerDrvLicNbr
txtCustomerName.Text = Order.CustomerName
txtCustomerAddress.Text = Order.CustomerAddress
txtCustomerCity.Text = Order.CustomerCity
cbxCustomerStates.Text = Order.CustomerState
txtCustomerZIPCode.Text = Order.CustomerZIPCode
cbxCarConditions.Text = Order.CarCondition
cbxTankLevels.Text = Order.TankLevel
txtMileageStart.Text = Order.MileageStart
txtMileageEnd.Text = Order.MileageEnd
dtpStartDate.Value = Order.DateStart
dtpEndDate.Value = Order.DateEnd
txtDays.Text = Order.Days
txtRateApplied.Text = FormatNumber(Order.RateApplied)
txtSubTotal.Text = FormatNumber(Order.SubTotal)
txtTaxRate.Text = FormatNumber(Order.TaxRate)
txtTaxAmount.Text = FormatNumber(Order.TaxAmount)
txtOrderTotal.Text = FormatNumber(Order.OrderTotal)
Else
MsgBox("There is no rental order with that receipt number")
Exit Sub
End If
End Sub
- In the Class Name combo box, select btnClose
- In the Method Name combo box, select Click and implement the event as follows:
Private Sub btnCloseClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnClose.Click
Close()
End Sub
- Execute the application
- Create a few rental orders and print them
- Close the forms and return to your programming environment
- Execute the application again
- Open a previously created order to simulate a customer returning a car and
change some values on the form such as the return date, the mileage end, and
the order status
- Also click the Calculate button to get the final evaluation
- Print the rental orders
- Close the forms and return to your programming environment
- Create a Windows Application named BethesdaCarRental1a
When writing any code that has to do with lists, use a class from the System.Collections
namespace
- Create a class named Employee that will hold the following pieces of
information about each employee: the employee number, the date hired, the
first name, the middle name, the last name, the home address, the city, the
state, the ZIP (or postal) code, the marital status, the title, the email
address, the home telephone number, the cell phone number, the work
telephone number, the extension, and the hourly salary
- Create a form named EmployeeEditor and design it so that it includes:
- A text box for the employee number, the date hired, the first name,
the middle name, the last name, the home address, the city, the ZIP (or
postal) code, the title, the email address, the home telephone number,
the cell phone number, the work telephone number, the extension, and the
hourly salary
- A combo box for the state (the items must include all US states and
all Canadian provinces in 2-letter abbreviation each), the marital
status (the items will include Single no Children, Single Parent,
Married no Children, Married With Children, Separated, Widow, Other)
- Create a form named Employees and that will be used to show some
information about each employee of the company, including the employee number,
the date hired, the full name (it will consist of the last name, followed by a
comma, followed by the first name), the marital status, the title, and the
hourly salary
|
|