Practical
Learning: Creating the Application
|
|
- Create a new Window Application named ElementaryOperations1
- In the Solution Explorer, right-click Form1.vb and click Rename
- Set the name to Exercise.vb
- Design the form as follows:
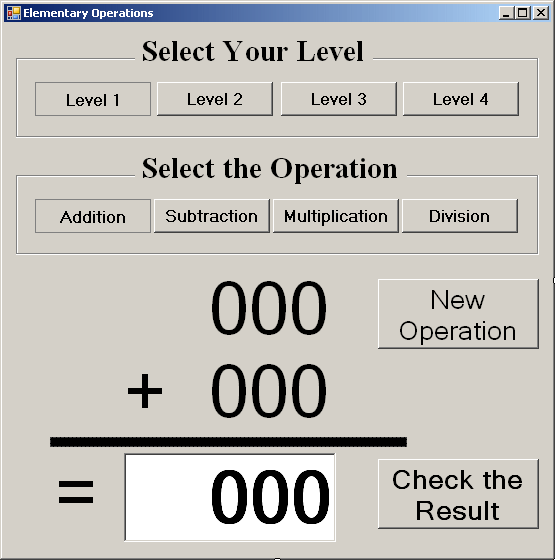 |
Control |
Text |
Name |
TextAlign |
Font |
Additional Properties |
Label |
Select Your Level |
|
|
|
|
GroupBox |
|
|
|
|
|
RadioButton |
Level 1 |
rdoLevel1 |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button
Checked: TRue |
RadioButton |
Level 2 |
rdoLevel2 |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button |
RadioButton |
Level 3 |
rdoLevel3 |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button |
RadioButton |
Level 4 |
rdoLevel4 |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button |
Label |
Select the OPeration |
|
|
|
|
GroupBox |
|
|
|
|
|
RadioButton |
Addition |
rdoAddition |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button
Checked: TRue |
RadioButton |
Subtraction |
rdoSubtraction |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button |
RadioButton |
Multiplication |
rdoMultiplication |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button |
RadioButton |
Division |
rdoDivision |
MiddleCenter |
Microsoft Sans Serif, 12pt, style=Bold |
Appearance: Button |
Label |
000 |
lblOperand1 |
MiddleRight |
Microsoft Sans Serif, 54pt |
|
Button |
New Operation |
btnNewOperation |
|
Microsoft Sans Serif, 20pt |
|
Label |
+ |
lblOperation |
MiddleRight |
Microsoft Sans Serif, 54pt |
|
Label |
00 |
lblOperand2 |
MiddleRight |
Microsoft Sans Serif, 54pt |
|
Panel |
|
|
|
|
Size: 317, 10 |
Label |
= |
|
Center |
Microsoft Sans Serif, 54pt |
AutoSize: True
ForeColor: Green |
TextBox |
000 |
txtResult |
Right |
Microsoft Sans Serif, 54pt, style=Bold |
|
Button |
Check Result |
btnCheckResult |
|
|
|
|
- Double-click the New Operation button and implement its event as
follows:
Private Sub btnNewOPerationClick(ByVal sender As System.Object,
ByVal e As System.EventArgs)
Handles btnNewOPeration.Click
Dim Number1 As Integer
Dim Number2 As Integer
Dim rndNumber As Random
rndNumber = New Random
' If the user clicked the Level 1 button,
' the operation will be performed on numbers from 1 to 9
If rdoLevel1.Checked = True Then
Number1 = rndNumber.Next(1, 10)
Number2 = rndNumber.Next(1, 10)
ElseIf rdoLevel2.Checked = True Then
' If the user clicked the Level 2 button,
' the operation will be performed on numbers from 10 to 19
Number1 = rndNumber.Next(10, 30)
Number2 = rndNumber.Next(10, 30)
ElseIf rdoLevel3.Checked = True Then
' If the user clicked the Level 3 button,
' the operation will be performed on numbers from 21 to 49
Number1 = rndNumber.Next(30, 50)
Number2 = rndNumber.Next(30, 50)
ElseIf rdoLevel4.Checked = True Then
' If the user clicked the Level 4 button,
' the operation will be performed on numbers from 51 to 99
Number1 = rndNumber.Next(50, 101)
Number2 = rndNumber.Next(50, 101)
End If
' Display the numbers to the user
lblOperand1.Text = CStr(Number1)
lblOperand2.Text = CStr(Number2)
' Just in case, empty the Result text box
txtResult.Text = ""
' Give focus to the Result text box
txtResult.Focus()
End Sub
|
- In the Class Name combo box, select rdoLevel1
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoLevel1Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoLevel1.Click
' When the user clicks the Level 1 button,
' behave as if the user had click the New Operation button
' That is, behave as if the user wants a new operation
btnNewOPerationClick(sender, e)
End Sub
|
- In the Class Name combo box, select rdoLevel2
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoLevel2Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoLevel1.Click
btnNewOPerationClick(sender, e)
End Sub
|
- In the Class Name combo box, select rdoLevel3
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoLevel3Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoLevel1.Click
btnNewOPerationClick(sender, e)
End Sub
|
- In the Class Name combo box, select rdoLevel4
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoLevel4Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoLevel1.Click
btnNewOPerationClick(sender, e)
End Sub
|
- In the Class Name combo box, select rdoAddition
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoAdditionClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoAddition.Click
lblOperation.Text = "+"
End Sub
|
- In the Class Name combo box, select rdoSubtraction
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoSubtractionClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoSubtraction.Click
lblOperation.Text = "-"
End Sub
|
- In the Class Name combo box, select rdoMultiplication
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoMultiplicationClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoMultiplication.Click
lblOperation.Text = "*"
End Sub
|
- In the Class Name combo box, select rdoDivision
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub rdoDivisionClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles rdoDivision.Click
lblOperation.Text = "/"
End Sub
|
- In the Class Name combo box, select btnCheckResult
- In the Method Name combo box, select Click and implement its event as
follows:
Private Sub btnCheckResultClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCheckResult.Click
Dim Number1 As Double
Dim Number2 As Double
Dim UserResult As Double
Dim OurResult As Double
Dim RandomNumber As Random = New Random
' It is hard to perform a comparison on a division
' So we will have to do some gymastic here to get something
' We will use this variable to format the number
' to appear as 0.00
' That will allow us to perform the comparision
' on a decimal number with a precision of 2
Dim strFixedResult As String
strFixedResult = ""
Dim Congratulations As String() = {
"Right :) - WOW - Good Answer!",
"Good Answer :) - You are Impressive",
"Right Answer :) - What a Good Job!",
"Good :) - You Are Greaaaaaaaaaaaat",
"Wonderful Answer :) - You Know It"
}
Dim WrongAnswers As String() = {
"Uhhhhhhhhhh - Bad Answer",
"Wrong - You will do better next time",
"Nop",
"Common - You can do Better Than That!",
"No - You are probably getting tired"
}
' Make sure the user provides a result
If txtResult.Text = "" Then
MsgBox("You must provide a result before clicking the button")
Exit Sub
End If
' Use exception handling to get the result
Try
UserResult = CDbl(txtResult.Text)
Number1 = CDbl(lblOperand1.Text)
Number2 = CDbl(lblOperand2.Text)
' Get the user's answer
If rdoAddition.Checked = True Then
OurResult = Number1 + Number2
' Format the result to appear with 2 decimal numbers
strFixedResult = String.Format("{0:F}", Number1 + Number2)
End If
If rdoSubtraction.Checked = True Then
OurResult = Number1 - Number2
strFixedResult = String.Format("{0:F}", Number1 - Number2)
End If
If rdoMultiplication.Checked = True Then
OurResult = Number1 * Number2
strFixedResult = String.Format("{0:F}", Number1 * Number2)
End If
If rdoDivision.Checked = True Then
OurResult = Number1 / Number2
strFixedResult = String.Format("{0:F}", Number1 / Number2)
End If
' Check if the user's answer is the right one
' Because of the division, we will format the result as 0.00
' then perform the comparison
If strFixedResult = CDbl(txtResult.Text).ToString("F") Then
MsgBox(Congratulations(RandomNumber.Next(0, 4)),
MsgBoxStyle.OkOnly Or MsgBoxStyle.Question,
"Elementary Operations")
Else
MsgBox(WrongAnswers(RandomNumber.Next(0, 4)),
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information,
"Elementary Operations")
End If
' After checking the user's answer, generate a new operation
btnNewOPerationClick(sender, e)
Catch ex As Exception
MsgBox("Invalid Numeric Value.")
End Try
End Sub
|
- Execute the application and test it
- Close the form and return to your programming environment
Download
|
|