 |
Collection-Based Application: Altair Realtors |
|
|
A real estate company or agency is a business that
presents or sells houses (properties) to prospective customers. This is an
application that addresses some of the issues with a real estate company.
|
Practical
Learning: Introducing the Collection Class
|
|
- Start Microsoft Visual Studio
- To create a new application, on the main menu, click File -> New
Project...
- In the middle list, click Windows Forms Application
- Change the Name to AltairRealtors1
- Click OK
- To create a new class, on the main menu, click Project -> Add
Class...
- Set the Name to RealEstateProperty
- Click Add
- Change the file as follows:
<Serializable()>
Public Class RealEstateProperty
Private nbr As String
Private pType As String
Private adrs As String
Private ct As String
Private stt As String
Private zip As String
Private levels As Byte
Private yr As Integer
Private beds As Byte
Private baths As Single
Private cond As String
Private status As String
Private cost As Double
Private pFile As String
Public Property PropertyNumber As String
Get
Return nbr
End Get
Set(ByVal value As String)
nbr = value
End Set
End Property
Public Property PropertyType As String
Get
Return pType
End Get
Set(ByVal value As String)
pType = value
End Set
End Property
Public Property Address As String
Get
Return adrs
End Get
Set(ByVal value As String)
adrs = value
End Set
End Property
Public Property City As String
Get
Return ct
End Get
Set(ByVal value As String)
ct = value
End Set
End Property
Public Property State As String
Get
Return stt
End Get
Set(ByVal value As String)
stt = value
End Set
End Property
Public Property ZIPCode As String
Get
Return zip
End Get
Set(ByVal value As String)
zip = value
End Set
End Property
Public Property Stories As Byte
Get
Stories = levels
End Get
Set(ByVal value As Byte)
levels = value
End Set
End Property
Public Property YearBuilt As Integer
Get
YearBuilt = yr
End Get
Set(ByVal value As Integer)
yr = value
End Set
End Property
Public Property Bedrooms As Byte
Get
Bedrooms = beds
End Get
Set(ByVal value As Byte)
beds = value
End Set
End Property
Public Property Bathrooms As Single
Get
Bathrooms = baths
End Get
Set(ByVal value As Single)
baths = value
End Set
End Property
Public Property Condition As String
Get
Condition = cond
End Get
Set(ByVal value As String)
cond = value
End Set
End Property
Public Property SaleStatus As String
Get
SaleStatus = status
End Get
Set(ByVal value As String)
status = value
End Set
End Property
Public Property MarketValue As Double
Get
MarketValue = cost
End Get
Set(ByVal value As Double)
cost = value
End Set
End Property
Public Property PictureFile As String
Get
PictureFile = pFile
End Get
Set(ByVal value As String)
pFile = value
End Set
End Property
' To determine that two properties are the same,
' we will test only the property number.
' We assume that if two properties have the same number,
' then it is the same property
Public Overrides Function Equals(ByVal obj As Object) As Boolean
Dim rep As RealEstateProperty = CType(obj, RealEstateProperty)
If rep.PropertyNumber = PropertyNumber Then
Equals = True
Else
Equals = False
End If
End Function
End Class
- In the Solution Explorer, right-click Form1.vb and click Rename
- Type AltairRealtors.vb and press Enter twice to
display the form
- From the Toolbox, add a list view to the form
- Right-click that list box on the click Edit Columns...
- Create the columns as follows:
(Name) |
Text |
TextAlign |
Width |
colPropertyNumber |
Property # |
|
65 |
colCity |
City |
|
75 |
colStories |
Stories |
Right |
45 |
colYearBuilt |
Year |
Right |
40 |
colBedrooms |
Beds |
Right |
38 |
colBathrooms |
Baths |
Right |
40 |
colCondition |
Condition |
|
80 |
colSaleStatus |
Status |
|
70 |
colMarketValue |
Value |
Right |
75 |
- Click OK
- Design the form as follows:
 |
Control |
(Name) |
Anchor |
BorderStyle |
SizeMode |
Text |
ListView |
 |
lvwProperties |
Top, Bottom, Left, Right |
|
|
|
Button |
 |
btnNewProperty |
Bottom, Left |
|
|
New Real Estate Property... |
PictureBox |
 |
pbxPicture |
Bottom, Right |
FixedSingle |
Zoom |
|
Button |
 |
btnClose |
Bottom, Right |
|
|
Close |
|
Form |
Text: |
Altair Realtors - Properties Listing |
StartPosition: |
CenterScreen |
- Right-click the form and click Edit Groups...
- Create the groups as follows:
Header |
Name |
Condominium |
lvgCondominium |
Townhouse |
lvgTownhouse |
Single Family |
lvgSingleFamily |
- Click OK
- To create a new form, on the main menu, click Projects -> Add
Windows Form...
- Set the Name to PropertyEditor
- Click Add
- In the Dialogs section of the Toolbox, click OpenFileDialog
- Click the form
- In the Properties window, change its characteristics as follows:
(Name): dlgPicture DefaultExt: jpg Filter: JPEG
Files (*.jpg,*.jpeg)|*.jpg Title: Select House Picture
- Design the form as follows:
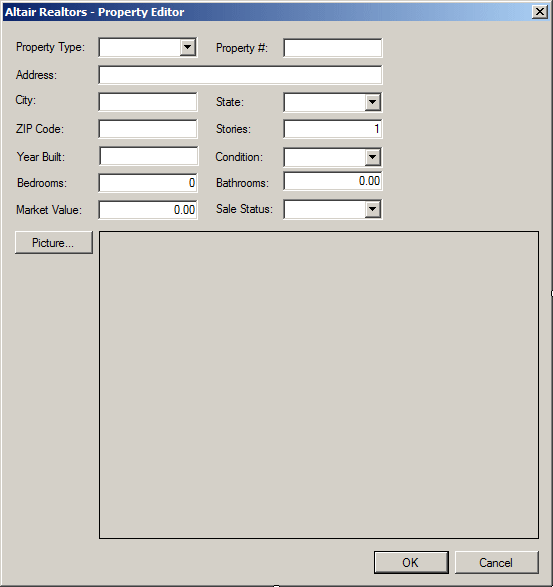 |
Control |
(Name) |
DropDownStyle |
Text |
Items |
Modifiers |
Other Properties |
Label |
 |
|
|
Property Type: |
|
|
|
ComboBox |
 |
cbxPropertyTypes |
DropDownList |
|
Condominium Townhouse Single Family
Unknown |
Public |
|
Label |
 |
|
|
Property #: |
|
|
|
TextBox |
 |
txtPropertyNumber |
|
|
|
Public |
|
Label |
 |
|
|
Address: |
|
|
|
TextBox |
 |
txtAddress |
|
|
|
Public |
|
Label |
 |
|
|
City: |
|
|
|
TextBox |
 |
txtCity |
|
|
|
Public |
|
Label |
 |
|
|
State: |
|
|
|
ComboBox |
 |
cbxStates |
DropDownList |
|
AL, AK, AZ, AR, CA, CO, CT, DE, DC, FL, GA, HI,
ID, IL, IN, IA, KS, KY, LA, ME, MD, MA, MI, MN, MS,
MO, MT, NE, NV, NH, NJ, NM, NY, NC, ND, OH, OK, OR,
PA, RI, SC, SD, TN, TX, UT, VT, VA, WA, WV, WI, WY |
Public |
|
Label |
 |
|
|
ZIP Code: |
|
|
|
TextBox |
 |
txtZIPCode |
|
|
|
Public |
|
Label |
 |
|
|
Stories: |
|
|
|
TextBox |
 |
txtStories |
|
|
|
Public |
|
Label |
 |
|
|
Year Built: |
|
|
|
TextBox |
 |
txtYearBuilt |
|
|
|
Public |
|
Label |
 |
|
|
Condition: |
|
|
|
ComboBox |
 |
cbxConditions |
DropDownList |
|
Excellent Good Shape Needs Fixing |
Public |
|
Label |
 |
|
|
Bedrooms: |
|
|
|
TextBox |
 |
txtBedrooms |
|
0 |
|
Public |
|
Label |
 |
|
|
Bathrooms: |
|
|
|
TextBox |
 |
txtBathrooms |
|
0.00 |
|
Public |
|
Label |
 |
|
|
Market Value: |
|
|
|
TextBox |
 |
txtMarketValue |
|
0.00 |
|
Public |
|
Label |
 |
|
|
Sale Status: |
|
|
|
ComboBox |
 |
cbxSaleStatus |
DropDownList |
|
Unspecified Available Sold |
Public |
|
Button |
 |
btnPicture |
|
Picture... |
|
|
|
PictureBox |
 |
pbxProperty |
|
|
|
|
BorderStyle: FixedSingle SizeMode: Zoom |
Button |
 |
btnOK |
|
OK |
|
|
DialogResult: OK |
Button |
 |
btnCancel |
|
Cancel |
|
|
DialogResult: Cancel |
|
Form |
FormBorderStyle: |
FixedDialog |
Text: |
Altair Realtors - Property Editor |
StartPosition: |
CenterScreen |
AcceptButton: |
btnOK |
CancelButton: |
btnCancel |
MaximizeBox: |
False |
MinimizeBox: |
False |
ShowInTaskBar: |
False |
- Right-click the form and click View Code
- Declare two variables as follows:
Public Class PropertyEditor
Public PictureChanged As Boolean
Public PictureFile As String
End Class
- In the top left combo box, select (PropertyEditor Events)
- In the right combo box, selec Load
- Implement the event as follows:
Public Class PropertyEditor
Public PictureChanged As Boolean
Public PictureFile As String
Private Sub PropertyEditor_Load(ByVal sender As Object,
ByVal e As System.EventArgs) Handles Me.Load
PictureChanged = False
PictureFile = "C:\Altair Realtors1\000-000.jpg"
End Sub
End Class
- In the top left combo box, select btnPicture
- In the right combo box, select Click
- Implement the event as follows:
Public Class PropertyEditor
Public PictureChanged As Boolean
Public PictureFile As String
Private Sub PropertyEditor_Load(ByVal sender As Object,
ByVal e As System.EventArgs) Handles Me.Load
PictureChanged = False
PictureFile = "C:\Altair Realtors1\000-000.jpg"
End Sub
Private Sub btnPicture_Click(ByVal sender As Object,
ByVal e As System.EventArgs) Handles btnPicture.Click
If dlgPicture.ShowDialog() = DialogResult.OK Then
pbxProperty.Image = Image.FromFile(dlgPicture.FileName)
PictureFile = dlgPicture.FileName
PictureChanged = True
End If
End Sub
End Class
Practical
Learning: Starting a Linked List Class
|
|
- Click the AltairRealtors.vb [Design] tab
- Right-click the form and click View Code
- Change the file as follows:
Imports System.IO
Imports System.Collections.Generic
Imports System.Runtime.Serialization.Formatters.Binary
Public Class AltairRealtors
Private Properties As LinkedList(Of RealEstateProperty)
Private Sub ShowProperties()
' Get a reference to the file that holds the records of properties
Dim Filename As String = "C:\Altair Realtors\properties.atr"
' Make sure the file exists
If File.Exists(Filename) = True Then
' if so, create a file stream
Dim stmProperties As FileStream = New FileStream(Filename,
FileMode.Open,
FileAccess.Read)
' Create a binary formatter
Dim bfmProperty As BinaryFormatter = New BinaryFormatter
' If some properties were created already,
' get them and store them in the collection
Properties = CType(bfmProperty.Deserialize(stmProperties), LinkedList(Of RealEstateProperty))
' First, empty the list view
lvwProperties.Items.Clear()
Dim lviProperty As ListViewItem = Nothing
' Visit each property in the collection and add it to the list view
For Each House As RealEstateProperty In Properties
If house.PropertyType.Equals("Condominium") Then
lviProperty = New ListViewItem(House.PropertyNumber, lvwProperties.Groups(0))
ElseIf House.PropertyType = "Townhouse" Then
lviProperty = New ListViewItem(House.PropertyNumber, lvwProperties.Groups(1))
Else ' If house.PropertyType.Equals("Single Family"))
lviProperty = New ListViewItem(House.PropertyNumber, lvwProperties.Groups(2))
lviProperty.SubItems.Add(house.City)
lviProperty.SubItems.Add(CStr(house.Stories))
lviProperty.SubItems.Add(CStr(house.YearBuilt))
lviProperty.SubItems.Add(CStr(house.Bedrooms))
lviProperty.SubItems.Add(FormatNumber(House.Bathrooms))
lviProperty.SubItems.Add(house.Condition)
lviProperty.SubItems.Add(house.SaleStatus)
lviProperty.SubItems.Add(CStr(House.MarketValue))
lvwProperties.Items.Add(lviProperty)
End If
Next
' Close the file stream
stmProperties.Close()
End If
End Sub
End Class
- In the Names combo box, select AltairRealtors events
- In the Procedures combo box, select Load
- Implement the event as follows:
Private Sub AltairRealtors_Load(ByVal sender As Object,
ByVal e As System.EventArgs) Handles Me.Load
Properties = New LinkedList(Of RealEstateProperty)
ShowProperties()
End Sub
Practical
Learning: Adding the First Node
|
|
- In the left combo box of the Code Editor, selec btnNewProperty
- In the right combo box, select Click
- Implement the event as follows:
Private Sub btnNewProperty_Click(ByVal sender As Object,
ByVal e As System.EventArgs) Handles btnNewProperty.Click
Dim Editor As PropertyEditor = New PropertyEditor
Dim RndNumber As Random = New Random(DateTime.Now.Millisecond)
Dim Number1 As Integer = RndNumber.Next(100, 999)
Dim Number2 As Integer = RndNumber.Next(100, 999)
Dim propNumber As String = Number1 & "-" & Number2
editor.txtPropertyNumber.Text = propNumber
' Check that the directory that contains the list of properties exists.
' If it doesn't exist, create it
Dim DirInfo As DirectoryInfo = Directory.CreateDirectory("C:\\Altair Realtors1")
' Get a reference to the file that holds the properties
Dim Filename As String = "C:\Altair Realtors1\properties.atr"
' First check if the file was previously created
If File.Exists(Filename) = True Then
' If the list of properties exists already,
' get it and store it in a file stream
Dim stmProperties As FileStream = New FileStream(Filename,
FileMode.Open,
FileAccess.Read)
Dim bfmProperty As BinaryFormatter = New BinaryFormatter
' Store the list of properties in the collection
Properties = CType(bfmProperty.Deserialize(stmProperties), LinkedList(Of RealEstateProperty))
' Close the file stream
stmProperties.Close()
End If
If editor.ShowDialog() = DialogResult.OK Then
Dim Prop As RealEstateProperty = New RealEstateProperty
Prop.PropertyNumber = Editor.txtPropertyNumber.Text
Prop.PropertyType = Editor.cbxPropertyTypes.Text
Prop.Address = Editor.txtAddress.Text
Prop.City = Editor.txtCity.Text
Prop.State = Editor.cbxStates.Text
Prop.ZIPCode = Editor.txtZIPCode.Text
Prop.Stories = CByte(Editor.txtStories.Text)
Prop.YearBuilt = CInt(Editor.txtYearBuilt.Text)
Prop.Bedrooms = CByte(Editor.txtBedrooms.Text)
Prop.Bathrooms = CSng(Editor.txtBathrooms.Text)
Prop.Condition = Editor.cbxConditions.Text
Prop.SaleStatus = Editor.cbxSaleStatus.Text
Prop.MarketValue = CDbl(Editor.txtMarketValue.Text)
If Editor.PictureFile <> "" Then
Dim PictureFile As FileInfo = New FileInfo(Editor.PictureFile)
PictureFile.CopyTo("C:\Altair Realtors1\" &
Editor.txtPropertyNumber.Text &
PictureFile.Extension)
Prop.PictureFile = "C:\Altair Realtors1\" &
Editor.txtPropertyNumber.Text &
PictureFile.Extension
Else
Prop.PictureFile = "C:\Altair Realtors1\000-000.jpg"
End If
' Add the property in the collection
Properties.AddFirst(Prop)
' Get a reference to the properties file
Dim strFilename As String = DirInfo.FullName & "\properties.atr"
' Create a file stream to hold the list of properties
Dim stmProperties As FileStream = New FileStream(strFilename,
FileMode.Create,
FileAccess.Write)
Dim bfmProperty As BinaryFormatter = New BinaryFormatter
' Serialize the list of properties
bfmProperty.Serialize(stmProperties, Properties)
' Close the file stream
stmProperties.Close()
' Show the list of properties
ShowProperties()
End If
End Sub
- In the left combo box of the Code Editor, select lvwProperties
- In the Events (right) combo box, select ItemSelectionChanged
- Implement the event as follows:
Private Sub lvwProperties_ItemSelectionChanged(ByVal sender As Object,
ByVal e As System.Windows.Forms.ListViewItemSelectionChangedEventArgs) Handles lvwProperties.ItemSelectionChanged
Dim CurrentProperty As RealEstateProperty = New RealEstateProperty
For Each Prop As RealEstateProperty In Properties
If Prop.PropertyNumber = e.Item.SubItems(0).Text Then
pbxProperty.Image = Image.FromFile(Prop.PictureFile)
End If
Next
End Sub
- To execute, press F5
- Click the New Real Estate Property button and
create a property

- Click OK
- Copy the following picture and paste (or save it) in the Altair
Realtors folder on the C: drive (or the folder that contains the file
properties of this project):
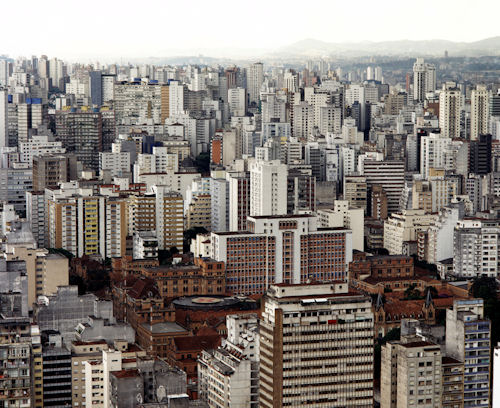
- Create some properties
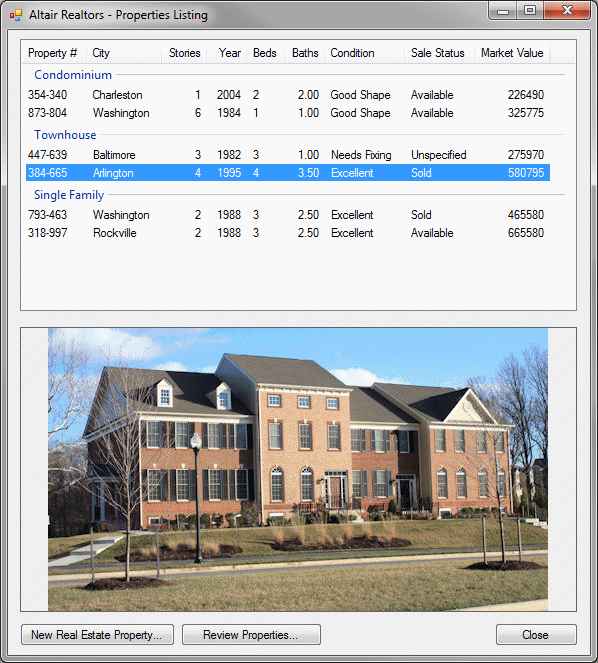
- Close the form and return to your programming environment
|
|