Because a combo box does not (permanently) display its list
like a list box, to show its content, the user can click the arrow button. Here
is an example:
To support combo boxes, the .NET Framework provides a class
named ComboBox. At design time, to add a combo box to your application,
from the Common Controls section of the Toolbox, you can click the ComboBox
button
and click the form or a container. Like ListBox, the ComboBox
class is derived from the ListControl class. Therefore, to
programmatically create a combo box, declare a variable of type ComboBox,
allocate its memory with the new operator and add it to the Controls
collection of its container. Here is an example:
Imports System.Drawing
Imports System.Windows.Forms
Module Exercise
Public Class Starter
Inherits Form
Private lblTitle As Label
Private cbxAcademicDisciplines As ComboBox
Dim components As System.ComponentModel.Container
Public Sub New()
InitializeComponent()
End Sub
Public Sub InitializeComponent()
lblTitle = New Label()
lblTitle.Text = "Academic Disciplines"
lblTitle.Location = New Point(12, 12)
lblTitle.AutoSize = True
Controls.Add(lblTitle)
cbxAcademicDisciplines = New ComboBox()
cbxAcademicDisciplines.Location = New Point(12, 32)
Controls.Add(cbxAcademicDisciplines)
End Sub
End Class
Function Main() As Integer
Dim frmStart As Starter = New Starter
Application.Run(frmStart)
Return 0
End Function
End Module
This would produce:

Practical Learning: Introducing Combo Boxes
|
|
- Start Microsoft Visual Basic and create a new Windows Application named
CollegeParkAutoParts1
- In the Solution Explorer, right-click Form1.vb and click Rename
- Type Central.vb and press Enter
- Design the form as follows:
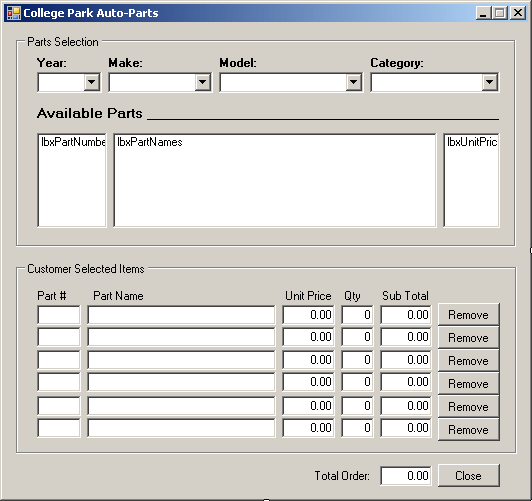 |
Control |
Text |
Name |
Other Properties |
GroupBox |
 |
Parts Selection |
|
|
Label |
 |
Year |
|
Font: Microsoft Sans Serif, 8.25pt,
style=Bold |
Label |
 |
Make |
|
Microsoft Sans Serif, 8.25pt, style=Bold |
Label |
 |
Model |
|
Microsoft Sans Serif, 8.25pt, style=Bold |
Label |
 |
Category |
|
Microsoft Sans Serif, 8.25pt, style=Bold |
ComboBox |
 |
|
cbxCarYears |
|
ComboBox |
 |
|
cbxMakes |
|
ComboBox |
 |
|
cbxModels |
|
ComboBox |
 |
|
cbxCategories |
|
Label |
 |
Available Parts |
|
Font: Microsoft Sans Serif, 9.75pt,
style=Bold |
ListBox |
 |
|
lbxPartNumbers |
|
ListBox |
 |
|
lbxPartNames |
|
ListBox |
 |
|
lbxUnitPrices |
|
GroupBox |
 |
Customer Selected Items |
|
|
Label |
 |
Part # |
|
|
Label |
 |
Part Name |
|
|
Label |
 |
Unit Price |
|
|
Label |
 |
Qty |
|
|
Label |
 |
Sub Total |
|
|
TextBox |
 |
|
txtPartNumber1 |
|
TextBox |
 |
|
txtPartName1 |
|
TextBox |
 |
0.00 |
txtUnitPrice1 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity1 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal1 |
TextAlign: Right |
Button |
 |
Remove |
btnRemove1 |
|
TextBox |
 |
|
txtPartNumber2 |
|
TextBox |
 |
|
txtPartName2 |
|
TextBox |
 |
0.00 |
txtUnitPrice2 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity2 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal2 |
TextAlign: Right |
Button |
 |
Remove |
btnRemove2 |
|
TextBox |
 |
|
txtPartNumber3 |
|
TextBox |
 |
|
txtPartName3 |
|
TextBox |
 |
0.00 |
txtUnitPrice3 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity3 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal3 |
TextAlign: Right |
Button |
 |
Remove |
btnRemove3 |
|
TextBox |
 |
|
txtPartNumber4 |
|
TextBox |
 |
|
txtPartName4 |
|
TextBox |
 |
0.00 |
txtUnitPrice4 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity4 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal4 |
TextAlign: Right |
Button |
 |
Remove |
btnRemove4 |
|
TextBox |
 |
|
txtPartNumber5 |
|
TextBox |
 |
|
txtPartName5 |
|
TextBox |
 |
0.00 |
txtUnitPrice5 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity5 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal5 |
TextAlign: Right |
Button |
 |
Remove |
btnRemove5 |
|
TextBox |
 |
|
txtPartNumber6 |
|
TextBox |
 |
|
txtPartName6 |
|
TextBox |
 |
0.00 |
txtUnitPrice6 |
TextAlign: Right |
TextBox |
 |
0 |
txtQuantity6 |
TextAlign: Right |
TextBox |
 |
0.00 |
txtSubTotal6 |
TextAlign: Right |
Button |
 |
Remove |
btnRemove6 |
|
Label |
 |
Total Order |
|
|
TextBox |
 |
0.00 |
txtTotalOrder |
|
Button |
 |
Close |
btnClose |
|
|
- Execute the application to test it
- Close the form and return to your programming environment
Creating and Selecting Items
|
|
Like all visual controls, a combo box shares all the basic
characteristics of other graphic control: the name, the location, the size, the
ability to be enabled or disabled, the ability to hide or show it, the ability
to dock or anchor, etc.
Creating the List of Items
|
|
Probably the most important aspect of a combo box is the
list of items it holds. Like the list box and all other list-based controls, the
list of a combo box is held by the Items property. You create this list using
the exact same techniques we reviewed for the list box. This means that you can
use the String Collection Editor.
The Items property of the ComboBox class is created
from the nested ObjectCollection class. This class has the same
functionality and features as its counterpart of the list box. This means that,
to programmatically create of items, you can (continuously) call the
ObjectCollection.Add() method. Here is an example:
Public Sub InitializeComponent()
lblTitle = New Label()
lblTitle.Text = "Academic Disciplines"
lblTitle.Location = New Point(12, 12)
lblTitle.AutoSize = True
Controls.Add(lblTitle)
cbxAcademicDisciplines = New ComboBox()
cbxAcademicDisciplines.Location = New Point(12, 32)
cbxAcademicDisciplines.Items.Add("Natural sciences")
cbxAcademicDisciplines.Items.Add("Mathematics and Computer sciences")
cbxAcademicDisciplines.Items.Add("Social sciences")
cbxAcademicDisciplines.Items.Add("Humanities")
cbxAcademicDisciplines.Items.Add("Professions and Applied sciences")
Controls.Add(cbxAcademicDisciplines)
End Sub
This would produce:
To add an array of items, you can call the AddRange()
method. To insert an item somewhere inside the list, you can call the
Insert() method.
If you want the list of items to be sorted, you can change
the value of the Sorted property in the Properties window from False
(the default) to True. To sort a list programmatically, you can assign a
true value to the Sorted property. You can un-sort the list by changing
the value of the Sorted property. This property works exactly like its
equivalent in the ListBox control.
Practical Learning: Using the ObjectCollection Class
|
|
- Right-click the form and click View Code
- In the Class Name combo box, select (Central Events)
- In the Method Name combo box, select Load
- To create a list of items using the Add() method, implement its
Load event as follows:
Private Sub CentralLoad(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles Me.Load
For i As Integer = Date.Today.Year To 1960 Step -1
cbxCarYears.Items.Add(i)
Next
End Sub
|
- Save the form
To select an item from the list, the user can click it. To
programmatically select an item, you can assign a string to the Text property of
a DropDown or a Simple combo box. Probably the best way to select
an item is to specify its index. The items of a combo box are stored in a
zero-based list. To select an item, you can assign its position to the
SelectedIndex property. In the same way, to find out what item is selected,
you can get the value of the SelectedIndex property.
Instead of using of using the index of an item, to select an
item using its identity or name, you can use the SelectedItem property.
To select an item by its name, assign it to the SelectedItem property.
Practical Learning: Using the ObjectCollection Class
|
|
- On the main menu, click Project -> Add Class...
- Set the name to PartDescription and press Enter
- To create a class that can holds a structured item of a list, change the
class as follows:
Public Class PartDescription
Private ID As Long
Private yr As Integer
Private mk As String
Private mdl As String
Private cat As String
Private name As String
Private price As Double
Public Sub New(ByVal code As Long, ByVal year As Integer,
ByVal make As String, ByVal model As String,
ByVal type As String, ByVal desc As String,
ByVal UPrice As Double)
ID = code
yr = year
mk = make
mdl = model
cat = type
name = desc
price = UPrice
End Sub
Public Property PartNumber() As Long
Get
Return ID
End Get
Set(ByVal value As Long)
ID = value
End Set
End Property
Public Property CarYear() As Integer
Get
Return yr
End Get
Set(ByVal value As Integer)
yr = value
End Set
End Property
Public Property Make() As String
Get
Return mk
End Get
Set(ByVal value As String)
mk = value
End Set
End Property
Public Property Model() As String
Get
Return mdl
End Get
Set(ByVal value As String)
mdl = value
End Set
End Property
Public Property Category() As String
Get
Return cat
End Get
Set(ByVal value As String)
cat = value
End Set
End Property
Public Property PartName() As String
Get
Return name
End Get
Set(ByVal value As String)
name = value
End Set
End Property
Public Property UnitPrice() As Double
Get
Return price
End Get
Set(ByVal value As Double)
price = value
End Set
End Property
Public Overrides Function ToString() As String
Return PartNumber & " " &
CarYear.ToString() & " " &
Make & " " &
Model & " " &
Category & " " &
PartName & " " &
UnitPrice
End Function
End Class
|
- Access the Central.vb file and change the Load event as follows:
Private parts(55) As PartDescription
Private Sub CentralLoad(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles Me.Load
For i As Integer = Date.Today.Year To 1960 Step -1
cbxCarYears.Items.Add(i)
Next
parts(0) = New PartDescription(447093, 2002, "Ford",
"Escort SE L4 2.0", "Engine Electrical",
"Alternator 75amp Remanufactured w/ 75 Amp",
205.05)
parts(1) = New PartDescription(203815, 2006, "Dodge",
"Caravan SE L4 2.4", "Cooling System",
"Radiator Cap", 6.65)
parts(2) = New PartDescription(293047, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Thermostat Gasket", 4.95)
parts(3) = New PartDescription(990468, 2002, "Honda",
"Civic 1.7 EX 4DR", "Exhaust",
"Bolt & Spring Kit (Manifold outlet, Muffler Inlet)",
85.75)
parts(4) = New PartDescription(304158, 1996, "Buick",
"Regal Custom V6 3.8", "Fuel Injection",
"Fuel Injector", 82.75)
parts(5) = New PartDescription(807245, 2004, "Acura",
"MDX 3.5 4WD", "Driveshaft & Axle",
"CV Boot Clamp 7 x 750mm 1 Large + 1 Small Clamp",
1.6)
parts(6) = New PartDescription(203485, 2001, "Ford",
"Taurus LX V6 3.0", "Fuel Injection",
"Oxygen Sensor OE Style 4Wire Front 2 Required",
52.65)
parts(7) = New PartDescription(248759, 1999, "Jeep",
"Wrangler Sahara", "Air Intake",
"Air Filter AirSoft Panel", 7.95)
parts(8) = New PartDescription(202848, 1998, "Honda",
"Accord 2.3 LX 4DR", "Air Intake",
"Air Filter", 12.55)
parts(10) = New PartDescription(932759, 2006, "Kia",
"Rio 1.6DOHC16V 4-DR", "Cooling System",
"Thermostat", 14.45)
parts(11) = New PartDescription(304975, 2000, "Honda",
"Civic 1.6 EX 4DR", "Suspension",
"Ball Joint Front Lower 2 per car", 40.55)
parts(12) = New PartDescription(208450, 2003, "Chevrolet",
"Monte Carlo LS V6 3.4", "Fuel Injection",
"Oxygen Sensor OE connector Rear", 65.55)
parts(13) = New PartDescription(209480, 2002, "Ford",
"Focus SE DOHC L4 2.0", "Steering",
"Steering Rack Remanufactured", 170.85)
parts(9) = New PartDescription(203495, 2004, "Honda",
"Civic 1.7 EX 4DR", "Climate Control",
"A/C Clutch OE compressor = Sanden", 184.95)
parts(14) = New PartDescription(203480, 2007, "Toyota",
"Corolla", "Air Intake", "Air Filter", 12.65)
parts(15) = New PartDescription(109379, 2005, "Volvo",
"S40 2.5L T5 AWD", "Fuel Delivery",
"Fuel Filter Early Design Outer Diameter = 55mm",
30.95)
parts(16) = New PartDescription(935794, 2002, "Ford",
"Escape XLS 4WD", "Brake",
"Brake Caliper Remanufactured Front Right",
65.55)
parts(17) = New PartDescription(203485, 2006, "BMW",
"325i", "Climate Control",
"AC High Pressure Side Switch", 49.95)
parts(18) = New PartDescription(204875, 1996, "Chevrolet",
"Monte Carlo Z34 V6 3.4", "Fuel Delivery",
"Fuel Filter", 8.05)
parts(19) = New PartDescription(937485, 2007, "Toyota",
"Camry V6", "Air Intake", "Air Filter", 12.95)
parts(20) = New PartDescription(294759, 2001, "Ford",
"Escape XLT 4WD", "Air Intake",
"Air Filter Panel", 7.25)
parts(21) = New PartDescription(297495, 2003, "Honda",
"Civic 1.7 EX 4DR", "Brake",
"Brake Caliper Reman w/ ProAct Pads Front Right",
82.55)
parts(22) = New PartDescription(794735, 2006, "BMW",
"325i", "Climate Control",
"Cabin Air/Pollen Filter With Activated Carbon",
28.05)
parts(23) = New PartDescription(937485, 2007, "Toyota",
"Corolla", "Body Electrical",
"Halogen SilverStar 12V 65W inner-high beam",
22.85)
parts(24) = New PartDescription(492745, 2005, "Ford",
"Focus ZX3 L4 2.0", "Air Intake",
"Fuel Injection Perf Kit", 342.95)
parts(25) = New PartDescription(937005, 2004, "Acura",
"MDX 3.5 4WD", "Driveshaft & Axle",
"CV Boot Clamp 7 x 750mm For Large End of Boot inner boot",
1.6)
parts(26) = New PartDescription(293749, 2004, "Acura",
"MDX 3.5 4WD", "Driveshaft & Axle",
"Axle Nut 24mm x 15 rear ",
2.35)
parts(27) = New PartDescription(920495, 2006, "BMW",
"325i", "Climate Control",
"Adjustable Telescoping Mirror", 7.95)
parts(28) = New PartDescription(204075, 2004, "Acura",
"MDX 3.5 4WD", "Driveshaft & Axle",
"Wheel Bearing Rear 1 per wheel", 70.15)
parts(29) = New PartDescription(979304, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Thermostat Housing", 20.95)
parts(30) = New PartDescription(300456, 2004, "Acura",
"MDX 3.5 4WD", "Driveshaft & Axle",
"Wheel Bearing Front 1 per wheel", 66.65)
parts(31) = New PartDescription(404860, 2001, "Ford",
"Taurus LX V6 3.0", "Suspension",
"Shock Absorber GR2 Rear Wagon only", 39.4)
parts(32) = New PartDescription(585688, 2007, "Buick",
"Lacrosse CXS V6 3.6", "Brake",
"Climate Control", 10.65)
parts(33) = New PartDescription(739759, 2001, "Ford",
"Taurus LX V6 3.0", "Suspension",
"Shock Absorber GasaJust Rear Wagon only", 30.95)
parts(34) = New PartDescription(927495, 2005, "Volvo",
"S40 2.5L T5 AWD", "Engine Mechanical",
"Timing Belt Idler Pulley Original Equipment INA",
65.55)
parts(40) = New PartDescription(979374, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Thermostat Gasket", 4.95)
parts(35) = New PartDescription(542347, 2007, "Buick",
"Lacrosse CXS V6 3.6", "Brake",
"Brake Pad Set ProACT Ceramic w/Shims Front", 80.05)
parts(36) = New PartDescription(683064, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Radiator Hose Upper", 103.75)
parts(37) = New PartDescription(248759, 1999, "Jeep",
"Wrangler Sahara", "Air Intake",
"Air Filter", 50.95)
parts(38) = New PartDescription(973974, 2007, "Toyota",
"Corolla", "Air Intake",
"Air Mass Meter W/o Housing Meter/sensor only",
134.95)
parts(39) = New PartDescription(285800, 2001, "Ford",
"Escape XLT 4WD", "Transmission", "AT Filter", 34.95)
parts(41) = New PartDescription(207495, 2007, "Toyota",
"Corolla", "Body Electrical",
"Headlight Bulb 12V 65W inner-high beam", 9.35)
parts(42) = New PartDescription(566676, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Auxiliary Fan Switch", 42.95)
parts(43) = New PartDescription(304950, 2007, "Toyota",
"Corolla", "Body Electrical",
"Headlight Bulb 12V 51W outer", 7.85)
parts(44) = New PartDescription(797394, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Water Flange Gasket", 0.85)
parts(45) = New PartDescription(910203, 2007, "Buick",
"Lacrosse CXS V6 3.6", "Suspension",
"Strut Mount Inc Sleeve Rear Right", 80.85)
parts(46) = New PartDescription(790794, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Radiator Hose Lower", 9.45)
parts(47) = New PartDescription(970394, 2007, "Buick",
"Lacrosse CXS V6 3.6", "Suspension",
"Coil Spring Insulator Front Lower", 14.55)
parts(48) = New PartDescription(290840, 2005, "Volvo",
"S40 2.5L T5 AWD", "Engine Mechanical",
"Rod Bearing Set 1 per Rod Standard Reqs. 5-per Engine",
26.95)
parts(49) = New PartDescription(209704, 2007, "Toyota",
"Corolla", "Body Electrical",
"Wiper Blade Excel+ Front Right", 7.25)
parts(50) = New PartDescription(200368, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Radiator Drain Plug incl gasket", 3.15)
parts(51) = New PartDescription(200970, 2005, "Volvo",
"S40 2.5L T5 AWD", "Engine Mechanical",
"Reference Sensor Flywheel Engine Speed", 62.05)
parts(52) = New PartDescription(542347, 2007, "Buick",
"Lacrosse CXS V6 3.6", "Air Intake",
"Air Filter", 50.25)
parts(53) = New PartDescription(927045, 2001, "Ford",
"Escape XLT 4WD", "Air Intake",
"Air Filter", 62.95)
parts(54) = New PartDescription(990659, 2000, "Toyota",
"RAV4 2WD/4-DOOR", "Cooling System",
"Radiator OE Plastic tank", 136.85)
parts(55) = New PartDescription(440574, 2007, "Buick",
"Lacrosse CXS V6 3.6", "Suspension",
"Strut Mount Inc Sleeve Rear Left", 80.8)
End Sub
|
- In the Class Name combo box, select cbxCarYears
- In the Method Name combo box, select SelectedIndexChanged and implement
the event as follows:
Private Sub cbxCarYearsSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles cbxCarYears.SelectedIndexChanged
cbxMakes.Text = ""
cbxMakes.Items.Clear()
cbxModels.Text = ""
cbxModels.Items.Clear()
cbxCategories.Text = ""
cbxCategories.Items.Clear()
lbxPartNumbers.Items.Clear()
lbxPartNames.Items.Clear()
lbxUnitPrices.Items.Clear()
For Each part As PartDescription In parts
If part.CarYear = CInt(cbxCarYears.Text) Then
If cbxMakes.FindString(part.Make) = -1 Then
cbxMakes.Items.Add(part.Make)
End If
End If
Next
End Sub
|
- In the Class Name combo box, select cbxMakes
- In the Method Name combo box, select SelectedIndexChanged and implement
the event as follows:
Private Sub cbxMakesSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles cbxMakes.SelectedIndexChanged
cbxModels.Text = ""
cbxModels.Items.Clear()
cbxCategories.Text = ""
cbxCategories.Items.Clear()
lbxPartNumbers.Items.Clear()
lbxPartNames.Items.Clear()
lbxUnitPrices.Items.Clear()
For Each part As PartDescription In parts
If (part.CarYear = CInt(cbxCarYears.Text) And
(part.Make = cbxMakes.Text)) Then
If cbxModels.FindString(part.Model) = -1 Then
cbxModels.Items.Add(part.Model)
End If
End If
Next
End Sub
|
- In the Class Name combo box, select cbxModels
- In the Method Name combo box, select SelectedIndexChanged and implement
the event as follows:
Private Sub cbxModelsSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles cbxModels.SelectedIndexChanged
lbxPartNumbers.Items.Clear()
lbxPartNames.Items.Clear()
lbxUnitPrices.Items.Clear()
For Each part As PartDescription In parts
If (part.CarYear = CInt(cbxCarYears.Text)) And
(part.Make = cbxMakes.Text) And
(part.Model = cbxModels.Text) Then
If cbxCategories.FindString(part.Category) = -1 Then
cbxCategories.Items.Add(part.Category)
End If
End If
Next
End Sub
|
- In the Class Name combo box, select cbxCategories
- In the Method Name combo box, select SelectedIndexChanged and implement
the event as follows:
Private Sub cbxCategoriesSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles cbxCategories.SelectedIndexChanged
lbxPartNumbers.Items.Clear()
lbxPartNames.Items.Clear()
lbxUnitPrices.Items.Clear()
For Each part As PartDescription In parts
If (part.CarYear = CInt(cbxCarYears.Text) And
(part.Make = cbxMakes.Text) And
(part.Model = cbxModels.Text) And
(part.Category = cbxCategories.Text)) Then
lbxPartNumbers.Items.Add(CStr(part.PartNumber))
lbxPartNames.Items.Add(part.PartName)
lbxUnitPrices.Items.Add(CStr(part.UnitPrice))
End If
Next
End Sub
|
- In the Class Name combo box, select lbxPartNumbers
- In the Method Name combo box, select SelectedIndexChanged
- When the user clicks an item from the Part Numbers list box, to make
sure the corresponding item is selected in the other list boxes, implement
the event as follows:
Private Sub lbxPartNumbersSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles lbxPartNumbers.SelectedIndexChanged
lbxUnitPrices.SelectedIndex = lbxPartNumbers.SelectedIndex
lbxPartNames.SelectedIndex = lbxUnitPrices.SelectedIndex
End Sub
|
- In the Class Name combo box, select lbxPartNames
- In the Method Name combo box, select SelectedIndexChanged and implement
the event as follows:
Private Sub lbxPartNamesSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles lbxPartNames.SelectedIndexChanged
lbxPartNumbers.SelectedIndex = lbxPartNames.SelectedIndex
lbxUnitPrices.SelectedIndex = lbxPartNames.SelectedIndex
End Sub
|
- In the Class Name combo box, select lbxUnitPrices
- In the Method Name combo box, select SelectedIndexChanged and implement
the event as follows:
Private Sub lbxUnitPricesSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles lbxUnitPrices.SelectedIndexChanged
lbxPartNames.SelectedIndex = lbxUnitPrices.SelectedIndex
lbxPartNumbers.SelectedIndex = lbxUnitPrices.SelectedIndex
End Sub
|
- Under the above event, create a procedure as follows:
Private Sub CalculateOrder()
' Calculate the current total order and update the order
Dim SubTotal1 As Double
Dim SubTotal2 As Double
Dim SubTotal3 As Double
Dim SubTotal4 As Double
Dim SubTotal5 As Double
Dim SubTotal6 As Double
Dim OrderTotal As Double
' Retrieve the value of each sub total
Try
SubTotal1 = CDbl(txtSubTotal1.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
subTotal2 = CDbl(txtSubTotal2.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
SubTotal3 = CDbl(txtSubTotal3.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
SubTotal4 = CDbl(txtSubTotal4.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
SubTotal5 = CDbl(txtSubTotal5.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
SubTotal6 = CDbl(txtSubTotal6.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
' Calculate the total value of the sub totals
OrderTotal = SubTotal1 + subTotal2 + SubTotal3 +
SubTotal4 + SubTotal5 + SubTotal6
' Display the total order in the appropriate text box
txtTotalOrder.Text = CStr(OrderTotal)
End Sub
|
- In the Class Name combo box, select lbxPartNumbers
- In the Method Name combo box, select DoubleClick and implement the event
as follows:
Private Sub lbxPartNumbersDoubleClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles lbxPartNumbers.DoubleClick
If txtPartNumber1.Text = "" Then
' Display the item number in the Part # text box
txtPartNumber1.Text = CType(lbxPartNumbers.SelectedItem, String)
' Display the name of the selected item in
' the current Description text box
txtPartName1.Text = CType(lbxPartNames.SelectedItem, String)
' Display the unit price of this item in
' the corresponding Unit Price text box
txtUnitPrice1.Text = CType(lbxUnitPrices.SelectedItem, String)
' Since an item was selected, set its quantity to 1
txtQuantity1.Text = "1"
' Calculate the sub total of the current item item
txtSubTotal1.Text = txtUnitPrice1.Text
' Give focus to the Qty text box of the current item
txtQuantity1.Focus()
' If the previous Part # text box is not empty, then use the next one
ElseIf txtPartNumber2.Text = "" Then
txtPartNumber2.Text = CType(lbxPartNumbers.SelectedItem, String)
txtPartName2.Text = CType(lbxPartNames.SelectedItem, String)
txtUnitPrice2.Text = CType(lbxUnitPrices.SelectedItem, String)
txtQuantity2.Text = "1"
txtSubTotal2.Text = txtUnitPrice2.Text
txtQuantity2.Focus()
ElseIf txtPartNumber3.Text = "" Then
txtPartNumber3.Text = CType(lbxPartNumbers.SelectedItem, String)
txtPartName3.Text = CType(lbxPartNames.SelectedItem, String)
txtUnitPrice3.Text = CType(lbxUnitPrices.SelectedItem, String)
txtQuantity3.Text = "1"
txtSubTotal3.Text = txtUnitPrice3.Text
txtQuantity3.Focus()
ElseIf txtPartNumber4.Text = "" Then
txtPartNumber4.Text = CType(lbxPartNumbers.SelectedItem, String)
txtPartName4.Text = CType(lbxPartNames.SelectedItem, String)
txtUnitPrice4.Text = CType(lbxUnitPrices.SelectedItem, String)
txtQuantity4.Text = "1"
txtSubTotal4.Text = txtUnitPrice4.Text
txtQuantity4.Focus()
ElseIf txtPartNumber5.Text = "" Then
txtPartNumber5.Text = CType(lbxPartNumbers.SelectedItem, String)
txtPartName5.Text = CType(lbxPartNames.SelectedItem, String)
txtUnitPrice5.Text = CType(lbxUnitPrices.SelectedItem, String)
txtQuantity5.Text = "1"
txtSubTotal5.Text = txtUnitPrice5.Text
txtQuantity5.Focus()
ElseIf txtPartNumber6.Text = "" Then
txtPartNumber6.Text = CType(lbxPartNumbers.SelectedItem, String)
txtPartName6.Text = CType(lbxPartNames.SelectedItem, String)
txtUnitPrice6.Text = CType(lbxUnitPrices.SelectedItem, String)
txtQuantity6.Text = "1"
txtSubTotal6.Text = txtUnitPrice6.Text
txtQuantity6.Focus()
' If all Part # text boxes are filled, don't do anything
Else
Exit Sub
End If
CalculateOrder()
End Sub
|
- In the Class Name combo box, select lbxPartNames
- In the Method Name combo box, select DoubleClick and implement the event
as follows:
Private Sub lbxPartNamesDoubleClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles lbxPartNames.DoubleClick
' This event should have been fired already
' But we call it just to be on the safe side
lbxPartNamesSelectedIndexChanged(sender, e)
' Now behave as if the Part Numbers list box was double-clicked
lbxPartNumbersDoubleClick(sender, e)
End Sub
|
- In the Class Name combo box, select lbxUnitPrices
- In the Method Name combo box, select Double-Click and implement the
event as follows:
Private Sub lbxUnitPricesSelectedIndexChanged(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles lbxUnitPrices.SelectedIndexChanged
lbxPartNames.SelectedIndex = lbxUnitPrices.SelectedIndex
lbxPartNumbers.SelectedIndex = lbxUnitPrices.SelectedIndex
End Sub
|
- In the Class Name combo box, select txtQuantity1
- In the Method Name combo box, select Leave and implement the event as
follows:
Private Sub txtQuantity1Leave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtQuantity1.Leave
Dim Quantity As Integer
Dim UnitPrice As Double, SubTotal As Double
' Get the quantity of the current item
Try
Quantity = CInt(txtQuantity1.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
' Get the unit price of the current item
Try
UnitPrice = CDbl(txtUnitPrice1.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
' Calculate the current sub total
SubTotal = Quantity * UnitPrice
' Display the new sub total in the corresponding text box
txtSubTotal1.Text = CStr(SubTotal)
' Update the order
CalculateOrder()
End Sub
|
- In the Class Name combo box, select txtQuantity2
- In the Method Name combo box, select Leave and implement the event as
follows:
Private Sub txtQuantity2Leave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtQuantity2.Leave
Dim Quantity As Integer
Dim UnitPrice As Double, SubTotal As Double
Try
Quantity = CInt(txtQuantity2.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
UnitPrice = CDbl(txtUnitPrice2.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
SubTotal = Quantity * UnitPrice
txtSubTotal2.Text = CStr(SubTotal)
CalculateOrder()
End Sub
|
- In the Class Name combo box, select txtQuantity3
- In the Method Name combo box, Leave Click and implement the event as
follows:
Private Sub txtQuantity3Leave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtQuantity3.Leave
Dim Quantity As Integer
Dim UnitPrice As Double, SubTotal As Double
Try
Quantity = CInt(txtQuantity3.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
UnitPrice = CDbl(txtUnitPrice3.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
SubTotal = Quantity * UnitPrice
txtSubTotal3.Text = CStr(SubTotal)
CalculateOrder()
End Sub
|
- In the Class Name combo box, select txtQuantity4
- In the Method Name combo box, Leave Click and implement the event as
follows:
Private Sub txtQuantity4Leave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtQuantity4.Leave
Dim Quantity As Integer
Dim UnitPrice As Double, SubTotal As Double
Try
Quantity = CInt(txtQuantity4.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
UnitPrice = CDbl(txtUnitPrice4.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
SubTotal = Quantity * UnitPrice
txtSubTotal4.Text = CStr(SubTotal)
CalculateOrder()
End Sub
|
- In the Class Name combo box, select txtQuantity5
- In the Method Name combo box, select Leave and implement the event as
follows:
Private Sub txtQuantity5Leave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtQuantity5.Leave
Dim Quantity As Integer
Dim UnitPrice As Double, SubTotal As Double
Try
Quantity = CInt(txtQuantity5.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
UnitPrice = CDbl(txtUnitPrice5.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
SubTotal = Quantity * UnitPrice
txtSubTotal5.Text = CStr(SubTotal)
CalculateOrder()
End Sub
|
- In the Class Name combo box, select txtQuantity6
- In the Method Name combo box, select Leave and implement the event as
follows:
Private Sub txtQuantity6Leave(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles txtQuantity6.Leave
Dim Quantity As Integer
Dim UnitPrice As Double, SubTotal As Double
Try
Quantity = CInt(txtQuantity6.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
Try
UnitPrice = CDbl(txtUnitPrice6.Text)
Catch ex As FormatException
MsgBox("Invalid Value")
End Try
SubTotal = Quantity * UnitPrice
txtSubTotal6.Text = CStr(SubTotal)
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnRemove1
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnRemove1Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRemove1.Click
txtPartNumber1.Text = ""
txtPartName1.Text = ""
txtUnitPrice1.Text = "0.00"
txtQuantity1.Text = "0"
txtSubTotal1.Text = "0.00"
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnRemove2
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnRemove2Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRemove2.Click
txtPartNumber2.Text = ""
txtPartName2.Text = ""
txtUnitPrice2.Text = "0.00"
txtQuantity2.Text = "0"
txtSubTotal2.Text = "0.00"
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnRemove3
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnRemove3Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRemove3.Click
txtPartNumber3.Text = ""
txtPartName3.Text = ""
txtUnitPrice3.Text = "0.00"
txtQuantity3.Text = "0"
txtSubTotal3.Text = "0.00"
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnRemove4
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnRemove4Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRemove4.Click
txtPartNumber4.Text = ""
txtPartName4.Text = ""
txtUnitPrice4.Text = "0.00"
txtQuantity4.Text = "0"
txtSubTotal4.Text = "0.00"
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnRemove5
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnRemove5Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRemove5.Click
txtPartNumber5.Text = ""
txtPartName5.Text = ""
txtUnitPrice5.Text = "0.00"
txtQuantity5.Text = "0"
txtSubTotal5.Text = "0.00"
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnRemove6
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnRemove6Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnRemove6.Click
txtPartNumber6.Text = ""
txtPartName6.Text = ""
txtUnitPrice6.Text = "0.00"
txtQuantity6.Text = "0"
txtSubTotal6.Text = "0.00"
CalculateOrder()
End Sub
|
- In the Class Name combo box, select btnClose
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCloseClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnClose.Click
End
End Sub
|
- Execute the application to test it

- After using it, close the form
Finding a String in the Combo Box
|
|
Instead of simply selecting an item from a combo box, the
user may want to find out if a certain string exists in the list. To
support this operation, the ComboBox class is equipped with a method
named FindString that is overloaded with two versions. One of the
syntaxes of this method is:
Public Function FindString(s As String) As Integer
This method takes as argument the string to find in the
combo box. If the item is found in the list, the method returns its position. If
the list does not have that string, the method return -1. The above syntax of
the method would look through the whole list. If you want the search to start at
a specific index, you can use the following version of the FindString()
method:
Public Function FindString(s As String, startIndex As Integer) As Integer
This version takes as the first argument a string. Instead
of start looking for it from the beginning of the list, this method starts at
the index specified by the startIndex value.
The FindString() method performs its operation
without regards to case. This means that it would perform the same search for
BlindMan, Blindman, blindMan, or BLINDMAN and would produce the same result for
them. If you want the case of the characters to be taken into consideration, use
the FindStringExact() method that also is overloaded with two versions.
The syntax of the first version is:
Public Function FindStringExact(s As String) As Integer
This method proceeds like the FindString() method by
starting to look for the string from the beginning of the list. If you want to
specify from where to start looking for the string, you should use the following
version:
Public Function FindStringExact(s As String, startIndex As Integer) As Integer
The Styles of a Combo Box
|
|
Like most graphical controls, a combo box appears as a 3-D
object with raised borders. As an alternative, you can display it as a flat
object. To assist you with this choice, the ComboBox class provides the
FlatStyle property. The FlatStyle property is based on the FlatStyle
enumeration. Its members are:
- Standard: This is the default value of the property. It makes the
control appear with raised borders:
Public Sub InitializeComponent()
lblTitle = New Label()
lblTitle.Text = "Academic Disciplines"
lblTitle.Location = New Point(12, 12)
lblTitle.AutoSize = True
Controls.Add(lblTitle)
cbxAcademicDisciplines = New ComboBox()
cbxAcademicDisciplines.Location = New Point(12, 32)
cbxAcademicDisciplines.Items.Add("Natural sciences")
cbxAcademicDisciplines.Items.Add("Mathematics and Computer sciences")
cbxAcademicDisciplines.Items.Add("Social sciences")
cbxAcademicDisciplines.Items.Add("Humanities")
cbxAcademicDisciplines.Items.Add("Professions and Applied sciences")
cbxAcademicDisciplines.FlatStyle = FlatStyle.Standard
Controls.Add(cbxAcademicDisciplines)
End Sub
|
 |
- Popup: The control will appear flat with a surrounding gray line:
cbxAcademicDisciplines.FlatStyle = FlatStyle.Popup
|
 |
- Flat: The control appears flat with a white surroundiong border:
cbxAcademicDisciplines.FlatStyle = FlatStyle.Flat
|
 |
- System: The user's operating system (and theme, if any) will
determine how the control must appear
In our introduction to the combo box, we saw that it
appeared like a text box with a down-pointing button on its right side. In
reality, that was the description of just one type of combo box. There are three
styles of combo boxes, although all allow the user to make only one selection.
These styles are controlled by the DropDownStyle property, which is based
on the ComboBoxStyle enumeration.
One of the types of combo boxes is referred to as Drop Down
and is created by setting the DropDownStyle property to DropDown.
Here is an example:
Public Sub InitializeComponent()
lblTitle = New Label()
lblTitle.Text = "Academic Disciplines"
lblTitle.Location = New Point(12, 12)
lblTitle.AutoSize = True
Controls.Add(lblTitle)
cbxAcademicDisciplines = New ComboBox()
cbxAcademicDisciplines.Location = New Point(12, 32)
cbxAcademicDisciplines.Items.Add("Natural sciences")
cbxAcademicDisciplines.Items.Add("Mathematics and Computer sciences")
cbxAcademicDisciplines.Items.Add("Social sciences")
cbxAcademicDisciplines.Items.Add("Humanities")
cbxAcademicDisciplines.Items.Add("Professions and Applied sciences")
cbxAcademicDisciplines.DropDownStyle = ComboBoxStyle.DropDown
Controls.Add(cbxAcademicDisciplines)
End Sub
This type is made of a text box on the left side and a
down-pointing arrowed button on the right side. Depending on how the control was
created, when it comes up, it may not display anything:
Normally, if you want a DropDown style of combo box
to display a string when the control comes up, you can either enter a value in
the Text property or assign a string to the ComboBox.Text
property. Here is an example:
Public Sub InitializeComponent()
lblTitle = New Label()
lblTitle.Text = "Academic Disciplines"
lblTitle.Location = New Point(12, 12)
lblTitle.AutoSize = True
Controls.Add(lblTitle)
cbxAcademicDisciplines = New ComboBox()
cbxAcademicDisciplines.Location = New Point(12, 32)
cbxAcademicDisciplines.Items.Add("Natural sciences")
cbxAcademicDisciplines.Items.Add("Mathematics and Computer sciences")
cbxAcademicDisciplines.Items.Add("Social sciences")
cbxAcademicDisciplines.Items.Add("Humanities")
cbxAcademicDisciplines.Items.Add("Professions and Applied sciences")
cbxAcademicDisciplines.DropDownStyle = ComboBoxStyle.DropDown
cbxAcademicDisciplines.Text = "Social sciences"
Controls.Add(cbxAcademicDisciplines)
End Sub
This would produce:

The string you give to the Text property does not
have to be one of the items of the list.
To use the combo box, the user can click its down pointing
arrow. At any time, to find out whether the list is displaying, you can check
the value of the DroppedDown Boolean property. In the same way, to drop
the list, you can programmatically set the combo box' DroppedDown
property to true.
Once the list is displaying, if the user clicks that arrow,
a list would appear (or expand). If the string assigned to the Text
property is one of the items in the list, it would display in the text box side
of the control and it would be selected in the list. Here is an example:
If the string assigned to the Text property is not
one of the items in the list, it would still appear selected in the text box
side of the control:
Here is an example:

When the list displays, either because the user clicked the
arrow button, pressed Alt + the down arrow key or because you decided to display
it, the control fires a DropDown event, which is of type EventArgs.
If the user sees an item that he or she wants or was asked
to select, he or she can click it. After an item has been clicked, two things
happen: 1. the list retracts (or collapses) like a plastic 2. the item that was
clicked fills the text part and becomes the new selection:
On the other hand, after displaying the list, if the user
doesn't want to select anything from the list, he or she can click the arrow
again or click anywhere away from the list. The list would collapse and the text
part would get back to the previous text.
One of the major characteristics of a DropDown style
of combo box, as compared to the type we will see next, is that, if the user
knows for sure that the item he or she is looking for is in the list, he can
first delete the string in the text box part of the control, then start typing.
For example, if the list contains a string such as Social Sciences , the user
can delete the text part, and start typing so. If there is only one item that
starts with s, the user can then click the arrow twice and the item would be
selected. Imagine the list contains such items as Jules and Julienne, if the
user types the first common letters of these item and double-click the arrow,
the first item that has these letters would be selected. This means that, if the
user wants to other item to be selected, he or she should type the letters
beyond the common ones. In the case of Jules and Julienne, if the user wants
Julienne to be selected from an incomplete string, he or she can type juli and
click the arrowed button twice.
Another style of combo box is gotten by setting the
DropDownStyle to DropDownList. This type also is made of a text box
on the left and a down-pointing arrowed button on the right side. It also may
appear empty when it comes up, depending on how it was created. The biggest
difference between a DropDown combo box and a DropDownList combo
box is that, with the drop down list, the user can only select from the list: he
or she cannot type anything in the text box part of the control.
Once again, to use the control, the user can click its
arrow, which causes the list to display. The user can also display the list
using the keyboard by pressing Alt + down arrow key after giving focus to the
control.
The last type of combo box is called a simple combo box and
is gotten by setting the DropDownStyle to Simple. After setting
this value, you must heighten the control to get the desired size. This type of
combo box is also made of two parts but they are distinct. The top section of
the combo box displays a text box. Immediately under the text box, there is a
list box. The following is the Character dialog box of OpenOffice.org. Its Font
property page is equipped with the Font, the Typeface, and the Size combo boxes
that are of a Simple style:
Notice that the control doesn't display a down-arrow
pointing button on the right side of the selected item since the list is
available already. To use this combo box, the user can examine the list part. If
he or she sees the desired item, he can click it. When an item is clicked, it
becomes the string of the top text part. If the user clicks a different item, it
would become the new selection, replacing the one that was in the text part.
Although this appears as a list box, the user cannot select more than one item.
The most regularly used combo boxes are made of text items.
You can also create a combo box that displays colors or pictures. To create such
a combo box, you start by changing the value of the DrawMode property
that is set to Normal by default. If you want to display items that are not just
regular text, you can set this property to either OwnerDrawFixed, which
would make all items have the same height, or OwnerDrawVariable, which
allows different items to have different sizes.
If the combo box has a DropDownStyle other than
Simple, there is typically a fixed number of items that display when the
user clicks the control’s arrow. You can control the number of items that
displays using the MaxDropDownItems property. By default, this is set to
8. If the list contains a number of items less than the MaxDropDownItems
integer value, all of the items would display fine. If the list contains more
than the MaxDropDownItems number of items, when the user clicks the
arrow, a vertical scroll box would appear. The control would display
MaxDropDownItems number of items to reveal more, the user would have to
scroll in the list.
In the next previous sections, we saw how to create a list
of items. The .NET Framework provides an alternative. Instead of creating a list
from scratch, you can use one that exists already. For example, you can use a
list of recently accessed web sites or custom list of your own. To assist you
with this, the ComboBox class provides with three techniques.
To specify an external list of items to use for the combo
box, you have two options. You can use the AutoCompleteSource property,
that is based on the AutoCompleteSource enumeration. The members of this
enumeration are: None, RecentlyUsedList, FileSystem,
FileSystemDirectories, HistoryList, ListItems,
AllSystemSources, AllUrl, and CustomSource. Imagine that you
want to use the list of web pages you had visited lately. To use that list, you
can specify the AutoCompleteSource as HistoryList.
After specifying the source of the list, use the
AutoCompleteMode property to specify how the combo box (or rather the text
box side of the control) will assist the user. This property is based on the
AutoCompleteMode enumeration that has four members. None is the
default value. Imagine you had set the value of the AutoCompleteSource
property as HistoryList. If you specify AutoCompleteMode as:
- Suggest: In the text box part of the combo box, the user can
click and start typing. A list of closely-matched items would display:

In this case, as soon as the user types h, a list of URLs that start with h
(for http) would come up. Once the user sees the desired item, he or she can
then click that item to select it. Since there are many items, to
continuously narrow the list, the user can keep typing until the desired
item comes up
- Append: In the text box part, the user can start typing. The
control would then start looking for the closest matches and try to complete
the user's entry with those available. Here is an example:
First the user types h and http:' comes up as the first closest match.
Then, the user specifies that the address starts with m and the compiler
suggests, in alphabetical order, the closest URL with that. Then, the user
types ms and finds out that msdn2 is available
- SuggestAppend: This is a combination of the previous two options.
When the control comes up, the user can start typing. The control would then
display the list of items that start with what the user typed and it would
display the starting closest match
The user can continue typing. If the desired item appears in the list,
the user can select it. Otherwise, as the user is typing, the closest match
displays in the text box part of the control
Instead of using an external list, you can create your own.
To do this, use the AutoCompleteCustomSource property. At design time, to
create a list of strings, access the Properties window for the text box. In the
Properties window, click the ellipsis button of the AutoCompleteCustomSource
field to open the String Collection Editor. Enter the strings separated by a
hard Return, and click OK. You can also programmatically create the list. To
assist you, the .NET Framework provides a class named
AutoCompleteStringCollection. The AutoCompleteStringCollection class
implements the IList, the ICollection, and the IEnumerable
interfaces.
After creating the custom list, to let the combo box use it,
set the AutoCompleteMode property to CustomSource.
Download |
|