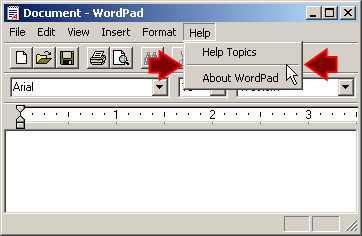
There are two reasons you would use a separator. You can use
a separator just for aesthetic reasons, to make your menu look good. Another,
more valuable reason, is to create groups of menu items and show their belonging
together by showing a line separating one group from another.
To visually specify a separator, when creating the menu
item, set its string to a simple -.
To support menu separators, the .NET Framework provides the
ToolStripSeparator class, which is derived from ToolStripItem. To
programmatically create a separator, declare a handle to ToolStripSeparator,
initialize it using the new operator, add it to the Items property of the
ToolStripItem menu category that will hold the separator. Here is an
example:
Imports System.Drawing
Imports System.Windows.Forms
Module Exercise
Public Class Starter
Inherits Form
Private mnuMain As MenuStrip
Private mnuFile As ToolStripMenuItem
Private mnuFileNew As ToolStripMenuItem
Private mnuSeparator As ToolStripSeparator
Friend WithEvents mnuFileExit As ToolStripMenuItem
Dim components As System.ComponentModel.Container
Public Sub New()
InitializeComponent()
End Sub
Public Sub InitializeComponent()
mnuMain = New MenuStrip
Controls.Add(mnuMain)
mnuFile = New ToolStripMenuItem("&File")
mnuFileNew = New ToolStripMenuItem("&New")
mnuFileNew.ShortcutKeys = Keys.Control Or Keys.N
mnuSeparator = New ToolStripSeparator
mnuFileExit = New ToolStripMenuItem("E&xit")
mnuFile.DropDownItems.Add(mnuFileNew)
mnuFile.DropDownItems.Add(mnuSeparator)
mnuFile.DropDownItems.Add(mnuFileExit)
mnuMain.Items.Add(mnuFile)
End Sub
Private Sub FileExiting(ByVal sender As Object, _
ByVal e As EventArgs) _
Handles mnuFileExit.Click
End
End Sub
End Class
Function Main() As Integer
Dim frmStart As Starter = New Starter
Application.Run(frmStart)
Return 0
End Function
End Module
This would produce:
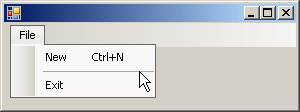
Practical
Learning: Creating a Menu Separator
|
|
- On the (Central) form, click File and, under New Property, click Type
Here
- Type - and press Enter
- Under the new separator, click Type Here, type E&xit and press
Enter
- On the form, click File and click Exit
- In the Properties window, change the (Name) to mnuFileExit
- On the form, click File and double-click Exit
- Implement the event as follows:
Private Sub mnuFileExit_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles mnuFileExit.Click
End
End Sub
|
- Execute the application to test it
- Close the form using its main menu and return to your programming
environment
- Under the form, click cmsProperties
- In the Properties window, click Items and click the ellipsis button
- In the Select Item And Add To List Below combo box, select Separator and
click Add
- In the Select Item And Add To List Below combo box, select MenuItem and
click Add
- On the side, change the properties as follows:
Text: Show
(Name): mnuShow
- Click OK
If you have menu items that perform similar tasks, you can
put them in a group, which you can do using line separators. Another option is
to create the menu items in their own group. The group of menu items that are
created as children of a parent menu is referred to as a sub-menu. Here is an
example of a sub-menu in Microsoft Paint:

To visually create a sub-menu, under the form, click the
menu control that will hold the items. In the menu designer
- If the sub-menu will be created for a main menu item, first click the
menu category, then click the menu item that will hold the sub-menu
- If the sub-menu will be created for a contextual menu, click the menu
item that will hold the sub-menu
After selecting the eventual parent of the intended
sub-menu, click the right Type Here box, type the desired caption and optionally
give it a name.
To create another item for the sub-menu, you can click the
Type Here box under the previous one. In the same way, you can add as many items
as you judge necessary. Here is an example:
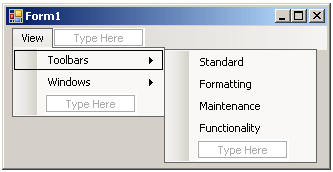
You can also create a sub-menu for a menu item that itself
is a sub-menu. Here is an example:
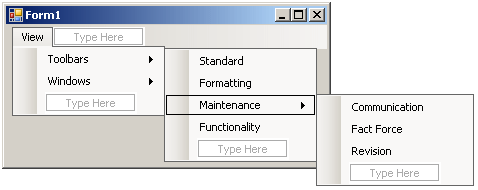
To create a sub-menu for an item A that itself is a
sub-menu, click that menu item A, click the Type Here box on the right side, and
type its caption.
As another technique, after selecting the menu item that
will act as the parent of the sub-menu, in the Properties window, click the
ellipsis button of the DropDownItems field to open the Items Collection Editor
dialog box. To create an item for the sub-menu, in the top combo box, select
MenuItem and click Add. Then configure the menu item as see fit (Text, (Name),
etc).
Like any menu item, each sub-menu item is an object of type
ToolStripMenuItem. Therefore, to programmatically create a sub-menu,
create each ToolStripMenuItem item and add it to the ToolStripMenuItem
menu item that will act as its parent.
Practical
Learning: Creating and Using Sub-Menus
|
|
- Under the form, click cmsProperties and, on the form, click Show
- On the right side of Show, click Type Here, type All and press
Enter
- Under All, click Type Here and type Apartments
- Press the down arrow key and type Townhouses
- Press the down arrow key and type Single Families
- On the form, click All and, in the Properties window, change its Name to
mnuShowAll
- On the form, click Apartments and, in the Properties window, change its
Name to mnuShowApartments
- On the form, click Townhouses and, in the Properties window, change its
Name to mnuShowTownhouses
- On the form, click Single Families and, in the Properties window, change
its Name to mnuShowSingleFamilies
- Right-click the form and click View Code
- In the Class Name combo box, select mnuShowAll
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub mnuShowAll_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles mnuShowAll.Click
lvwRentalProperties.Items.Clear()
If lstRentalProperties.Count > 0 Then
For Each prop As RentalProperty In lstRentalProperties
Dim itmProperty As ListViewItem = _
New ListViewItem(prop.PropertyCode)
itmProperty.SubItems.Add(prop.PropertyType)
itmProperty.SubItems.Add(CStr(prop.Bedrooms))
itmProperty.SubItems.Add(FormatNumber(prop.Bathrooms))
itmProperty.SubItems.Add(FormatNumber(prop.MonthlyRent))
itmProperty.SubItems.Add(prop.OccupancyStatus)
lvwRentalProperties.Items.Add(itmProperty)
Next
End If
End Sub
|
- In the Class Name combo box, select mnuShowApartments
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub mnuShowApartments_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles mnuShowApartments.Click
lvwRentalProperties.Items.Clear()
If lstRentalProperties.Count > 0 Then
For Each prop As RentalProperty In lstRentalProperties
If prop.PropertyType = "Apartment" Then
Dim itmProperty As ListViewItem = _
New ListViewItem(prop.PropertyCode)
itmProperty.SubItems.Add(prop.PropertyType)
itmProperty.SubItems.Add(CStr(prop.Bedrooms))
itmProperty.SubItems.Add(FormatNumber(prop.Bathrooms))
itmProperty.SubItems.Add(FormatNumber(prop.MonthlyRent))
itmProperty.SubItems.Add(prop.OccupancyStatus)
lvwRentalProperties.Items.Add(itmProperty)
End If
Next
End If
End Sub
|
- In the Class Name combo box, select mnuShowTownhouses
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub mnuShowTownhouses_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles mnuShowTownhouses.Click
lvwRentalProperties.Items.Clear()
If lstRentalProperties.Count > 0 Then
For Each prop As RentalProperty In lstRentalProperties
If prop.PropertyType = "Townhouse" Then
Dim itmProperty As ListViewItem = _
New ListViewItem(prop.PropertyCode)
itmProperty.SubItems.Add(prop.PropertyType)
itmProperty.SubItems.Add(CStr(prop.Bedrooms))
itmProperty.SubItems.Add(FormatNumber(prop.Bathrooms))
itmProperty.SubItems.Add(FormatNumber(prop.MonthlyRent))
itmProperty.SubItems.Add(prop.OccupancyStatus)
lvwRentalProperties.Items.Add(itmProperty)
End If
Next
End If
End Sub
|
- In the Class Name combo box, select mnuShowSingleFamilies
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub mnuShowSingleFamilies_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles mnuShowSingleFamilies.Click
lvwRentalProperties.Items.Clear()
If lstRentalProperties.Count > 0 Then
For Each prop As RentalProperty In lstRentalProperties
If prop.PropertyType = "Single Family" Then
Dim itmProperty As ListViewItem = _
New ListViewItem(prop.PropertyCode)
itmProperty.SubItems.Add(prop.PropertyType)
itmProperty.SubItems.Add(CStr(prop.Bedrooms))
itmProperty.SubItems.Add(FormatNumber(prop.Bathrooms))
itmProperty.SubItems.Add(FormatNumber(prop.MonthlyRent))
itmProperty.SubItems.Add(prop.OccupancyStatus)
lvwRentalProperties.Items.Add(itmProperty)
End If
Next
End If
End Sub
|
- Execute the application to test it
- Create a few properties as earlier then change the types of properties
to display
- Close the form and return to your programming environment
|
|