Topic
Applied: Creating an MDI Application
|
|
- Click the form to give it focus
- In the Properties window, change the following characteristics:
IsMdiContainer: True StartPosition: CenterScreen Text:
Notice
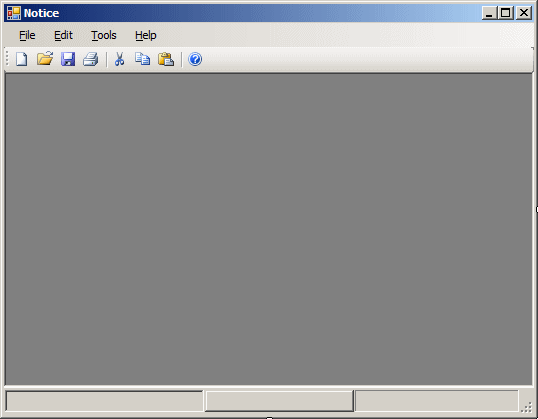
- To add a new form, on the main menu, click Project -> Add Windows
Form...
- In the middle list, make sure Windows Form is selected.
Set the
Name to SingleDocument
- Click Add
- From the Common Controls section of the Toolbox, click RichTextBox
and click the form
- In the Properties window, change the following characteristics:
(Name): RtbNotice Dock: Fill Modifiers: Public
- Display the first form
- On the form, click File and double-click New
- Implement the event as follows:
Public Class Notice
Private Sub MnuFileNew_Click(sender As System.Object,
e As System.EventArgs) Handles MnuFileNew.Click
Dim document As SingleDocument = New SingleDocument
document.Text = "Untitled"
document.MdiParent = Me
document.Show()
MnuFileClose.Enabled = True
End Sub
End Class
- On the Object combo box, select MnuFileOpen
- In the Procedure combo box, select Click
- Implement the event as follows:
Private Sub MnuFileOpen_Click(sender As Object,
e As System.EventArgs) Handles MnuFileOpen.Click
If dlgFileOpen.ShowDialog() = System.Windows.Forms.DialogResult.OK Then
For Each strFile As String In dlgFileOpen.FileNames
Dim document As SingleDocument = New SingleDocument
document.RtbNotice.LoadFile(strFile)
document.MdiParent = Me
document.Show()
Next
End If
End Sub
- Return to the main form
- On its toolbar, click the first button (New)
- Click the Events button
of the Properties window
- Click the Click field and, on the right side, select
MnuFileNew_Click
- On the toolbar of the form, click the second button (Open)
- In the Events section of the Properties window, click the button of
Click and select MnuFileOpen_Click
Introduction to the Children of an MDI
|
|
When you create an MDI application, you must make sure
you provide your users with the ability to create documents. In fact,
probably one of your first assignments is to make sure the user can create
as many documents as necessary. As the documents are created, you need a way
to programmatically keep track of the child forms. For example, you can
store the documents in a collection. To assist you with this, the
Form class is equipped with a property named MdiChildren,
which is a read-only array. Each element of the MdiChildren[]
array is of type Form. Therefore, to access a child form of
an MDI application, you can pass an index to this property.
The Layout of Child Forms
|
|
Based on the standards defined in the operating system,
as child forms are created in an MDI application, they are positioned each
on top of the previous one but below the next one. The arrangement uses a
3-D coordiniate system whose origin is on the lower-left corner of the
parent's frame just under the title bar (or the menu, if any; or the
toolbar, if any), with the Z axis moving from the monitor towards you:
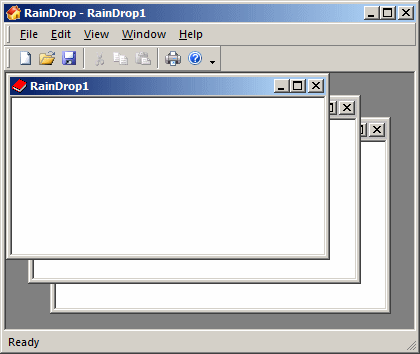
The operating system allows the user to choose among
different arrangements. For example, you can position the documents as
vertical columns, as horizontal rows, or as tiles. To support this, the
Form class is equipped with a method named
LayoutMdi. Its syntax is:
Public Sub LayoutMdi(value As MdiLayout)
The LayoutMdi() method takes an
argument that is a member of the MdiLayout enumeration. The
members of this enumeration are Cascade,
TileHorizontal, TileVertical, and
ArrangeIcons.
Topic
Applied: Laying Out the Documents
|
|
- Make sure the Notice form is displaying.
Under the form, click
MnuMain
- In the Properties window, click Items and click its ellipsis button
- In the Select Item And Add To List Below, make sure MenuItem is
selected and click Add
- Change its Text to &Window
- Change the (Name) to MnuWindow
- Use the up button
to move and position it just above MnuHelp

- While MnuWindow is selected, in the right list, click DropDownItems
and click its button
- Use the Add button to create items with the following
characteristics:
Text |
(Name) |
&Arrange |
MnuWindowArrange |
&Cascade |
MnuWindowCascade |
Tile &Horizontal |
MnuWindowTileHorizontal |
Tile &Vertical |
MnuWindowTileVertical |
- Click OK
- Click OK
- On the form, click Window and double-click Arrange
- Implement the event as follows:
Private Sub MnuWindowArrange_Click(sender As System.Object,
e As System.EventArgs) Handles MnuWindowArrange.Click
LayoutMdi(MdiLayout.ArrangeIcons)
End Sub
- In the Object combo box, select MnuWindowCascade
- In the Procedure combo box, select Click
- Implement the event as follows:
Private Sub MnuWindowCascade_Click(sender As Object,
e As System.EventArgs) Handles MnuWindowCascade.Click
LayoutMdi(MdiLayout.Cascade)
End Sub
- In the Object combo box, select MnuWindowTileHorizontal
- In the Procedure combo box, select Click
- Implement the event as follows:
Private Sub MnuWindowTileHorizontal_Click(sender As Object,
e As System.EventArgs) Handles MnuWindowTileHorizontal.Click
LayoutMdi(MdiLayout.TileHorizontal)
End Sub
- In the Object combo box, select MnuWindowTileVertical
- In the Procedure combo box, select Click
- Implement the event as follows:
Private Sub MnuWindowTileVertical_Click(sender As Object,
e As System.EventArgs) Handles MnuWindowTileVertical.Click
LayoutMdi(MdiLayout.TileVertical)
End Sub
In most MDI applications, a user can create as many
documents as necessary. This also means that the application can hold many
child forms. To access a child form, the user can click its title bar. You
can also provide options on a menu item named Window that would display a
list of open documents.
When a child window is activated, it fires an event
named MdiChildActivate:
Public Event MdiChildActivate As EventHandler
The MdiChildActivate event is of type
EventArgs.
The document that is currently active has a brighter
title bar. To identify this document, the form has a property named
ActiveMdiChild. This read-only property allows you to know what
document is the current active one. This property is of type Form,
which means its produces a Form object. When you enquire
about this property, if its value is null, it means there is no current
active document.
If the value of the ActiveMdiChild
property is not null, a document is active and you can use it. If you want
to access the objects (Windows controls) that are positioned on a child
form, remember that the child form is somehow foreign. Therefore, you should
set the Modifiers property of its hosted controls approppriately. For
example, if you want the parent frame to access a control on the child
frame, set the Modifiers of that control to Public.
Topic
Applied: Getting the Active Document
|
|
- In the Object combo box, select MnuFileClose
- In the Procedure combo box, select Click
- Implement the event as follows:
Private Sub MnuFileClose_Click(sender As Object,
e As System.EventArgs) Handles MnuFileClose.Click
Dim document As SingleDocument = CType(ActiveMdiChild, SingleDocument)
If Not document Is Nothing Then
document.Close()
End If
If MdiChildren.Length = 0 Then
MnuFileClose.Enabled = False
End If
End Sub
- Return to the form
Arranging the Minimized Children
|
|
In an MDI application, if the user doesn't want to
display a document but doesn't want to close it, he can minimise the window.
In the same way, the user can minimize as many child forms as necessary.
When a child form has been minimized, it shows a button in the lower part of
the parent. The buttons of the other minimized child forms are usually
positioned next to each other:
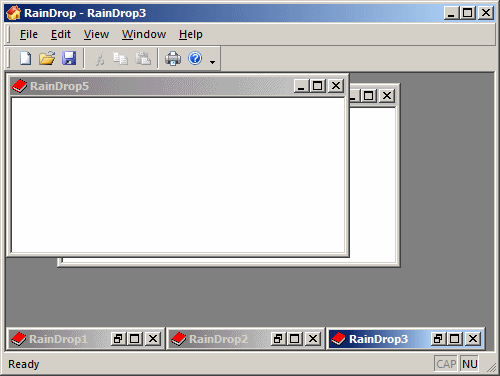
The user can move the buttons at will:
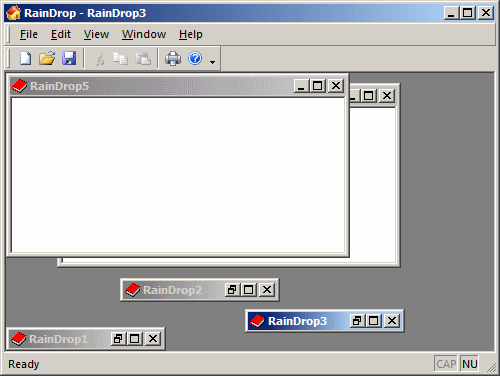
A user can also close the child form using the Close
button of its minimized button. At one time the minimized buttons may
display as a "mess". To let you rearrange them, call the LayoutMdi
method of the Form class and pass the argument as
ArrangeIcons. When you do this, the application will visit all
buttons, if any, that represent minimized child documents, and position them
from the left to the right, adjacently.
|
|