|
The records from a table are
grouped in an object referred to as a set of records also called a record
set. To support record sets, the Microsoft Access Object library is equipped
with a class named Recordset. To use it, declare a variable of type
Object or of type Recordset. Before using it, use the
Set operator to assign it to the object that would use it. Here is an
example:
|
Private Sub cmdRecordset_Click()
' Create a recordset
Dim rstTimeSheet As Recordset
' Specify that the record set points to the records of this form
Set rstTimeSheet = ...
End Sub
Most of the time, you use an Object object or a
Recordset object without being aware because the database engine
handles all or most of the necessary routines behind the scenes. In some
other cases, you must create or initialize an Object object or a
Recordset object in order to use it. To do this, you have various
alternatives. In fact, the way you create a Recordset object may
depend on the library you are using at the time you need to "grab" the set
of records.
Practical
Learning: Introducing Record Sets
|
|
- Start Microsoft Access
- Open the KoloBank1 database from Lesson 20
The Type of Recordset
Objects
|
|
A record set primarily contains records. The records
can be retrieved from a table or gotten directly from a table. When
creating a record set (as we will see in the next few sections), you can
specify whether to use one or more tables and what record(s) would be
included. How the record set is created, the number of tables, the number
of records, and the types of operations that can be performed on the
records lead to various types of record sets:
- If your Recordset object includes only one table, it is
referred to as a Table record set
- If a record set includes one or more tables, it is called a
Dynaset. This type allows adding, editing, updating, or deleting
records that are part of the Recordset object
- A record set is called a Snapshot if it allows you to view
the records of one or more tables, navigating back and forth in the
set, but you cannot make changes to the records
- A Recordset object is referred to as Forward-Only if
you can view its records without changing them but you can only move
forward. This means that, if you get to a record, examine it, and move
to the next record, you cannot refer back to a record you left behind
- If you create a query based on data from one or more tables and
allows the users to navigate back and forth with the ability to make
changes to records, this type of record set is referred to as
Dynamic
The Recordset Object of a Control
|
|
Some controls, such as the combo box or the list box,
are meant to hold a list of values. We also know that a form or a report
is primarily created to show one or more records. Such controls hold their
own record set. If you create a Recordset object and want to
initialize it with the values held in the form where it is called, you can
simply assign it Me.Recordset. Here is an example:
Private Sub cmdRecordset_Click()
' Create a recordset
Dim rstTimeSheet As Recordset
' Specify that the record set points to the records of this form
Set rstTimeSheet = Me.Recordset
End Sub
When a form is equipped to display the values of a
list, that form has a Recordset object that represents its records.
Once again, remember that there are various other ways you can initialize
a Recordset object.
With the Microsoft Access Object Library, to create a
Recordset object using a table or a query that is associated with a
database, you can call the OpenRecordset() method of that database.
The syntax of this method is:
Set Variable = Database.OpenRecordset(Source[, Type [, Options [, Lockedits ]]])
The Variable can be an Object variable
you would have declared as a placeholder for a Recordset object.
Database must represent a valid database. It can be the current
database or another one. The only required argument of this method is the
Source, which is passed as a string. This can be the name of a
table or a query. Here is an example:
Private Sub cmdGetEmployees_Click()
Dim dbKoloBank As Object
Dim rstEmployees As Object
Set dbKoloBank = CurrentDb
Set rstEmployees = dbKoloBank.OpenRecordset("Employees")
End Sub
The above code supposes that you would go through a
database to create a record set. It can be used to create a record set
from the current database or from a closed database. If you are working in
a database that has its own objects and you want to create a record set
using one of these objects, each database object that can act as a data
source, including tables and queries, is equipped with an
OpenRecordset() method. Its syntax is:
Set Variable = object.OpenRecordset([Type [, Options [, Lockedits ]]])
Notice that, this time, you specify neither the
database nor the name of the object.
After using a Recordset object, you should
(strongly) close it. To close a record set, you can call its Close()
method. Here is an example:
Private Sub cmdTableReference_Click()
Dim rstEmployees As Object
. . . Use the record set here
rstEmployees.Close
End Sub
After closing the record set, you should release the
resources it was using and make them available to other applications that
would need them. To do this, assign the Nothing value to the
Recordset object. Here is an example:
Private Sub cmdTableReference_Click()
Dim rstEmployees As Recordset
. . . Use the record set here
rstEmployees.Close
Set rstEmployees = Nothing
End Sub
If you were using the ActiveConnection property
to connect to the current database, to close it, the Recordset
object is equipped with a property named ActiveConnection. Before
closing the Recordset object, you should first assign Nothing
to its ActiveConnection property.
Opening a Record Set in the Current Database
|
|
The values held by a table are referred to as a record
set. Before performing data entry, you must programmatically open the
table. That is, you must open the record set. To make this possible in the
Microsoft Access Object Library, its Database class is equipped
with a method named OpenRecordset. This method can take as argument
the name of the table on which you want to perform data entry. Based on
this, here is an example of calling the method:
Private Sub cmdDataEntry_Click()
curDatabase.OpenRecordset("Employees")
End Sub
Once you have the record set, you can perform data
entry on it. To support this, the OpenRecordset() method returns an
object named Recordset. If you want to use the table after opening
the record set, get the return value of the method. To do this, declare a
variable of type Object or Recordset and assign it to the
return value of this method call. Here is an example:
Private Sub cmdDataEntry_Click()
Dim curDatabase As Object
Dim rstEmployees As Object
Set curDatabase = CurrentDb
Set rstEmployees = curDatabase.OpenRecordset("Employees")
End Sub
After using the record set, free the memory it was
using by assigning Nothing to it. This would be done as follows:
Private Sub cmdDataEntry_Click()
Dim curDatabase As Object
Dim rstEmployees As Object
Set curDatabase = CurrentDb
Set rstEmployees = curDatabase.OpenRecordset("Employees")
. . . Use the record set here
Set rstEmployees = Nothing
Set curDatabase = Nothing
End Sub
Introduction to the Records of a Record Set
|
|
Introduction to Data Entry
|
|
After opening then getting a record set, you can
create a new record. To support the creation of a record, the Recordset
class is equipped with a method named AddNew. Here is an example of
calling it:
Private Sub cmdDataEntry_Click()
Dim curDatabase As Object
Dim rstEmployees As Object
Set curDatabase = CurrentDb
Set rstEmployees = curDatabase.OpenRecordset("Employees")
rstEmployees.AddNew
Set rstEmployees = Nothing
Set curDatabase = Nothing
End Sub
This method only indicates that you want to create a
new record. To actually create a record, you must specify a value for each
column of the table. To support this, the Recordset class is
equipped with an indexed property. The indexed property of a record set
represents an object of type Field. One of the properties of the
Field class is the Name, which is the
name of a column. Therefore, when accessing the indexed property of the
Recordset class, pass the name of the column as argument (the name of
the column whose value you want to specify).
Another property of the Field class
is named Value. To specify the new value of a column, use
this property to assign the desired value to the column. Here is an
example of specifying a new value for a column named FirstName from a
table named Employees:
Private Sub cmdDataEntry_Click()
Dim curDatabase As Object
Dim rstEmployees As Object
Set curDatabase = CurrentDb
Set rstEmployees = curDatabase.OpenRecordset("Employees")
rstEmployees.AddNew
rstEmployees("FirstName").Value = "Helene"
Set rstEmployees = Nothing
Set curDatabase = Nothing
End Sub
If you had configured the field to automatically
increment its values, make sure you don't provide a value for that field.
After adding a new record, you must ask the record set
to receive the new value. To support this, the Recordset class is
equipped with a method named Update. Call this method after
specifying a value for each column. Here is example of calling this
method:
Private Sub cmdCreateTable_Click()
Dim curDatabase As Object
Dim tblEmployees As Object
Dim fldFirstName As Object, fldLastName As Object
' Get a reference to the current database
Set curDatabase = CurrentDb
' Create a new table named Employees
Set tblEmployees = curDatabase.CreateTableDef("Employees")
Set fldFirstName = tblEmployees.CreateField("FirstName", dbText)
tblEmployees.Fields.Append fldFirstName
Set fldLastName = tblEmployees.CreateField("LastName", dbText)
tblEmployees.Fields.Append fldLastName
' Add the Employees table to the current database
curDatabase.TableDefs.Append tblEmployees
End Sub
Private Sub cmdDataEntry_Click()
Dim curDatabase As Object
Dim rstEmployees As Object
Set curDatabase = CurrentDb
Set rstEmployees = curDatabase.OpenRecordset("Employees")
rstEmployees.AddNew
rstEmployees("FirstName").Value = "Helene"
rstEmployees("LastName").Value = "Mukoko"
rstEmployees.Update
Set rstEmployees = Nothing
Set curDatabase = Nothing
End Sub
Practical
Learning: Introducing Data Entry in a Record Set
|
|
- In the Navigation Pane, right-click NewTimeSheet and click Design
View
- On the form, right-click the Submit Time Sheet button and click
Build Event
- In the Choose Builder dialog box, click Code Builder and click OK
- Implement the event as follows:
Private Sub cmdSubmitTimeSheet_Click()
On Error GoTo cmdSubmitTimeSheet_Error
Dim curDatabase As Database
Dim rstTimeSheets As Recordset
Dim fldTimeSheet As Field
If IsNull(txtEmployeeNumber) Then
MsgBox "You must enter the employee number to proceed.", _
vbOKOnly Or vbInformation, "Kolo Bank"
Exit Sub
End If
If IsNull(txtStartDate) Then
MsgBox "You must enter the starting date to proceed.", _
vbOKOnly Or vbInformation, "Kolo Bank"
Exit Sub
End If
Set curDatabase = CurrentDb
Set rstTimeSheets = curDatabase.OpenRecordset("TimeSheets")
With rstTimeSheets
.AddNew
.Fields("EmployeeNumber").Value = txtEmployeeNumber
.Fields("StartDate").Value = CDate(txtStartDate)
.Fields("EndDate").Value = CDate(txtEndDate)
.Fields("TimeSheetCode").Value = txtTimeSheetCode
.Fields("Week1Monday").Value = txtWeek1Monday
.Fields("Week1Tuesday").Value = txtWeek1Tuesday
.Fields("Week1Wednesday").Value = txtWeek1Wednesday
.Fields("Week1Thursday").Value = txtWeek1Thursday
.Fields("Week1Friday").Value = txtWeek1Friday
.Fields("Week1Saturday").Value = txtWeek1Saturday
.Fields("Week1Sunday").Value = txtWeek1Sunday
.Fields("Week2Monday").Value = txtWeek2Monday
.Fields("Week2Tuesday").Value = txtWeek2Tuesday
.Fields("Week2Wednesday").Value = txtWeek2Wednesday
.Fields("Week2Thursday").Value = txtWeek2Thursday
.Fields("Week2Friday").Value = txtWeek2Friday
.Fields("Week2Saturday").Value = txtWeek2Saturday
.Fields("Week2Sunday").Value = txtWeek2Sunday
.Fields("Notes").Value = txtNotes
.Update
End With
Set rstTimeSheets = Nothing
Set curDatabase = Nothing
MsgBox "The time sheet has been submitted.", _
vbOKOnly Or vbInformation, "Kolo Bank"
cmdReset_Click
cmdSubmitTimeSheet_Exit:
Exit Sub
cmdSubmitTimeSheet_Error:
MsgBox "There was a problem when submitting the time sheet.", _
vbOKOnly Or vbInformation, "Kolo Bank"
Resume cmdSubmitTimeSheet_Exit
End Sub
- In the Object combo box, select cmdClose
- Implement the event as follows:
Private Sub cmdClose_Click()
DoCmd.Close
End Sub
- Return to Microsoft Access
- Save and close the form
- In the Navigation Pane, right-click NewPayroll and click Design
View
- On the form, right-click Find Time Sheet and click Build Event
- In the Choose Builder dialog box, click Code Builder and click OK
- Implement the event as follows:
Private Sub cmdFindTimeSheet_Click()
On Error GoTo cmdFindTimeSheet_Error
Dim dWeek1Monday As Double, dWeek1Tuesday As Double
Dim dWeek1Wednesday As Double, dWeek1Thursday As Double
Dim dWeek1Friday As Double, dWeek1Saturday As Double
Dim dWeek1Sunday As Double
Dim dWeek2Monday As Double, dWeek2Tuesday As Double
Dim dWeek2Wednesday As Double, dWeek2Thursday As Double
Dim dWeek2Friday As Double, dWeek2Saturday As Double
Dim dWeek2Sunday As Double
Dim TotalWeek1Time As Double
Dim TotalWeek2Time As Double
Dim Week1RegularTime As Double
Dim Week2RegularTime As Double
Dim Week1OvertimeTime As Double
Dim Week2OvertimeTime As Double
Dim Week1RegularPay As Currency
Dim Week2RegularPay As Currency
Dim Week1OvertimePay As Currency
Dim Week2OvertimePay As Currency
Dim dRegularTime As Double
Dim dOvertimeTime As Double
Dim RegularPay As Double
Dim OvertimePay As Double
Dim TotalEarnings As Double
Dim NetEarnings As Double
Dim dHourlySalary As Double
Dim OvertimeSalary As Double
Dim FederalTax As Double
Dim SocialSecurityTax As Double
Dim MedTax As Double
Dim StTax As Double
If IsNull(txtTimeSheetCode) Then
MsgBox "You must enter a time sheet code.", _
vbOKOnly Or vbInformation, "Kolo Bank", _
vbOKOnly Or vbInformation, "Kolo Bank"
Exit Sub
End If
If Not IsNull(DLookup("TimeSheetCode", "TimeSheets", _
"TimeSheetCode = '" & txtTimeSheetCode & "'")) Then
txtEmployeeNumber = DLookup("EmployeeNumber", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'")
txtEmployeeName = DLookup("LastName", "Employees", _
"EmployeeNumber = '" & _
txtEmployeeNumber & "'") & _
", " & _
DLookup("FirstName", "Employees", _
"EmployeeNumber = '" & _
txtEmployeeNumber & "'")
txtHourlySalary = DLookup("HourlySalary", "Employees", _
"EmployeeNumber = '" & _
txtEmployeeNumber & "'")
txtStartDate = CDate(DLookup("StartDate", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
txtEndDate = CDate(DLookup("EndDate", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
txtPayDate = FormatDateTime(DateAdd("d", 18, _
CDate(txtStartDate)), vbLongDate)
' Retrieve the hourly salary
dHourlySalary = CDbl(txtHourlySalary)
' Retrieve the time for each day
' First Week
dWeek1Monday = CDbl(DLookup("Week1Monday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek1Tuesday = CDbl(DLookup("Week1Tuesday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek1Wednesday = CDbl(DLookup("Week1Wednesday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek1Thursday = CDbl(DLookup("Week1Thursday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek1Friday = CDbl(DLookup("Week1Friday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek1Saturday = CDbl(DLookup("Week1Saturday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek1Sunday = CDbl(DLookup("Week1Sunday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
' Second Week
dWeek2Monday = CDbl(DLookup("Week2Monday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek2Tuesday = CDbl(DLookup("Week2Tuesday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek2Wednesday = CDbl(DLookup("Week2Wednesday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek2Thursday = CDbl(DLookup("Week2Thursday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek2Friday = CDbl(DLookup("Week2Friday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek2Saturday = CDbl(DLookup("Week2Saturday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
dWeek2Sunday = CDbl(DLookup("Week2Sunday", "TimeSheets", _
"TimeSheetCode = '" & _
txtTimeSheetCode & "'"))
' Calculate the total time for first week
TotalWeek1Time = dWeek1Monday + dWeek1Tuesday + _
dWeek1Wednesday + dWeek1Thursday + _
dWeek1Friday + dWeek1Saturday + dWeek1Sunday
' Calculate the total time for second week
TotalWeek2Time = dWeek2Monday + dWeek2Tuesday + _
dWeek2Wednesday + dWeek2Thursday + _
dWeek2Friday + dWeek2Saturday + dWeek2Sunday
' The overtime is paid time and half
OvertimeSalary = dHourlySalary * 1.5
' If the employee worked under 40 hours, there is no overtime
If TotalWeek1Time <= 40 Then
Week1RegularTime = TotalWeek1Time
Week1RegularPay = dHourlySalary * Week1RegularTime
Week1OvertimeTime = 0
Week1OvertimePay = 0
' If the employee worked over 40 hours, calculate the overtime
ElseIf TotalWeek1Time > 40 Then
Week1RegularTime = 40
Week1RegularPay = dHourlySalary * 40
Week1OvertimeTime = TotalWeek1Time - 40
Week1OvertimePay = Week1OvertimeTime * OvertimeSalary
End If
If TotalWeek2Time <= 40 Then
Week2RegularTime = TotalWeek2Time
Week2RegularPay = dHourlySalary * Week2RegularTime
Week2OvertimeTime = 0
Week2OvertimePay = 0
ElseIf TotalWeek2Time > 40 Then
Week2RegularTime = 40
Week2RegularPay = dHourlySalary * 40
Week2OvertimeTime = TotalWeek2Time - 40
Week2OvertimePay = Week2OvertimeTime * OvertimeSalary
End If
dRegularTime = Week1RegularTime + Week2RegularTime
dOvertimeTime = Week1OvertimeTime + Week2OvertimeTime
RegularPay = Week1RegularPay + Week2RegularPay
OvertimePay = Week1OvertimePay + Week2OvertimePay
TotalEarnings = RegularPay + OvertimePay
' The following calculations are for demonstration purpose only
' Consult the brochure for federal tax table
' FederalTax = ???
SocialSecurityTax = TotalEarnings * 6.2 / 100
MedTax = TotalEarnings * 1.45 / 100
StTax = TotalEarnings * 5.5 / 100
NetEarnings = TotalEarnings - SocialSecurityTax - MedTax - StTax
txtRegularTime = dRegularTime
txtOvertimeTime = dOvertimeTime
txtRegularPay = CCur(RegularPay)
txtOvertimePay = CCur(OvertimePay)
txtGrossPay = CDbl(TotalEarnings)
txtSocialSecurityTax = CDbl(SocialSecurityTax)
txtMedicareTax = CDbl(MedTax)
txtStateTax = CDbl(StTax)
txtNetPay = CDbl(NetEarnings)
Else
MsgBox "No time sheet was found in that time frame for the indicated employee."
cmdReset_Click
End If
cmdFindTimeSheet_Exit:
Exit Sub
cmdFindTimeSheet_Error:
MsgBox "An error occured when retrieving the time sheet information" & vbCrLf & _
"Please call the program vendor and report the error as follows:" & vbCrLf & _
"Error #: " & Err.Number & vbCrLf & _
"Reason: " & Err.Description, _
vbOKOnly Or vbInformation, "Kolo Bank"
Resume Next
End Sub
- In the Object combo box, select txtFederalTax
- In the Procedure combo box, select LostFocus
- Implement the event as follows:
Private Sub txtFederalTax_LostFocus()
Dim GrossPay As Double
Dim FederalWithholding As Double
Dim SocialSecurity As Double
Dim Medicare As Double
Dim State As Double
Dim NetPay As Double
GrossPay = CDbl(txtGrossPay)
FederalWithholding = CDbl(txtFederalTax)
SocialSecurity = CDbl(txtSocialSecurityTax)
Medicare = CDbl(txtMedicareTax)
State = CDbl(txtStateTax)
NetPay = GrossPay - FederalWithholding - SocialSecurity - Medicare - State
txtNetPay = NetPay
End Sub
- In the Object combo box, select cmdApproveSubmitPayroll
- Implement the event as follows:
Private Sub cmdApproveSubmitPayroll_Click()
On Error GoTo cmdApproveSubmitPayroll_Error
Dim curDatabase As Object
Dim rstPayrolls As Object
Dim fldPayroll As Object
If IsNull(txtTimeSheetCode) Then
MsgBox "You must enter the time sheet code.", _
vbOKOnly Or vbInformation, "Kolo Bank"
Exit Sub
End If
Set curDatabase = CurrentDb
Set rstPayrolls = curDatabase.OpenRecordset("Payrolls")
With rstPayrolls
.AddNew
.Fields("StartDate").Value = txtStartDate
.Fields("EndDate").Value = txtEndDate
.Fields("TimeSheetCode").Value = txtTimeSheetCode
.Fields("EmployeeNumber").Value = txtEmployeeNumber
.Fields("EmployeeName").Value = txtEmployeeName
.Fields("HourlySalary").Value = txtHourlySalary
.Fields("RegularTime").Value = txtRegularTime
.Fields("RegularPay").Value = txtRegularPay
.Fields("OvertimeTime").Value = txtOvertimeTime
.Fields("OvertimePay").Value = txtOvertimePay
.Fields("GrossPay").Value = txtGrossPay
.Fields("FederalTax").Value = txtFederalTax
.Fields("SocialSecurityTax").Value = txtSocialSecurityTax
.Fields("MedicareTax").Value = txtMedicareTax
.Fields("StateTax").Value = txtStateTax
.Fields("NetPay").Value = txtNetPay
.Fields("Notes").Value = txtNotes
.Update
End With
Set rstPayrolls = Nothing
Set curDatabase = Nothing
MsgBox "The payroll has been submitted.", _
vbOKOnly Or vbInformation, "Kolo Bank"
cmdReset_Click
cmdApproveSubmitPayroll_Exit:
Exit Sub
cmdApproveSubmitPayroll_Error:
MsgBox "There was a problem when submitting the payroll.", _
vbOKOnly Or vbInformation, "Kolo Bank"
Resume cmdApproveSubmitPayroll_Exit
End Sub
- Close Microsoft Visual Basic and return to Microsoft Access
- Save and close the NewPayroll form
- In the Navigation Pane, double-click NewTimeSheet
- Enter the following values:
Employee # |
503-938 |
|
Start Date |
02-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
8 |
8.5 |
8 |
9.5 |
8.5 |
0 |
0 |
Week 2 |
8 |
8 |
6.5 |
6 |
6 |
0 |
0 |
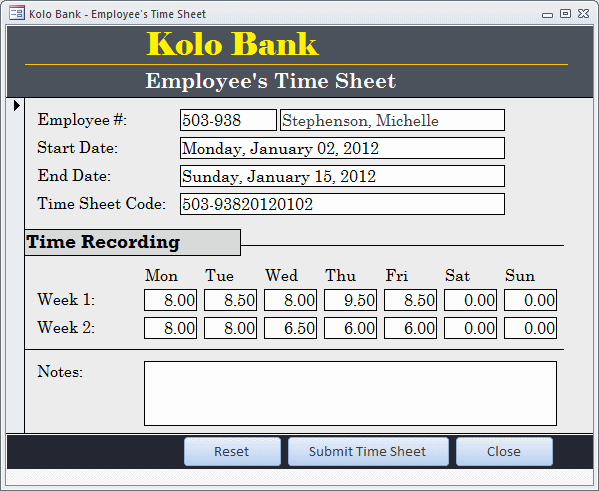
- Click Submit Time Sheet
- Click OK
- Enter the following values
Employee # |
552-884 |
|
Start Date |
02-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
8 |
7.5 |
7 |
7.5 |
6 |
0 |
0 |
Week 2 |
6 |
6.5 |
6 |
7.5 |
6 |
0 |
0 |
- Click Submit Time Sheet and click OK
- Enter the following values:
Employee # |
660-026 |
|
Start Date |
02-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
0 |
0 |
0 |
0 |
0 |
8 |
8 |
Week 2 |
0 |
0 |
0 |
0 |
0 |
8 |
8 |
- Click Submit Time Sheet and click OK
- Enter the following values
Employee # |
552-884 |
|
Start Date |
16-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
8 |
6.5 |
8.5 |
8 |
8.5 |
0 |
0 |
Week 2 |
8 |
7 |
7.5 |
8 |
8.5 |
0 |
0 |
- Click Submit Time Sheet and click OK
- Enter the following values:
Employee # |
503-938 |
|
Start Date |
16-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
10 |
9.5 |
8.5 |
9 |
9.5 |
0 |
0 |
Week 2 |
9 |
8.5 |
8.5 |
9.5 |
10.5 |
0 |
0 |
- Click Submit Time Sheet and click OK
- Enter the following values:
Employee # |
952-846 |
|
Start Date |
16-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
8 |
8 |
8 |
8 |
8 |
0 |
0 |
Week 2 |
8 |
8 |
8 |
8 |
8 |
0 |
0 |
- Click Submit Time Sheet and click OK
- Enter the following values
Employee # |
503-938 |
|
Start Date |
30-Jan-2012 |
|
|
|
|
Mon |
Tue |
Web |
Thu |
Fri |
Sat |
Sun |
Week 1 |
8.5 |
9.5 |
8.5 |
9 |
8 |
6 |
6 |
Week 2 |
8 |
9 |
9.5 |
10 |
8.5 |
6 |
5.5 |
- Click Submit Time Sheet and click OK
- Close the form
- In the Navigation Pane, double-click NewPayrol
- Enter the Time Sheet Code as 552-88420120102
- Click the Find Time Sheet button
- In the Federal Tax text box, type 602.82 (we
multiplied the gross pay by 30 and divided it by 100; of course, this
is not how it's done, this is just for our lesson) and press Tab
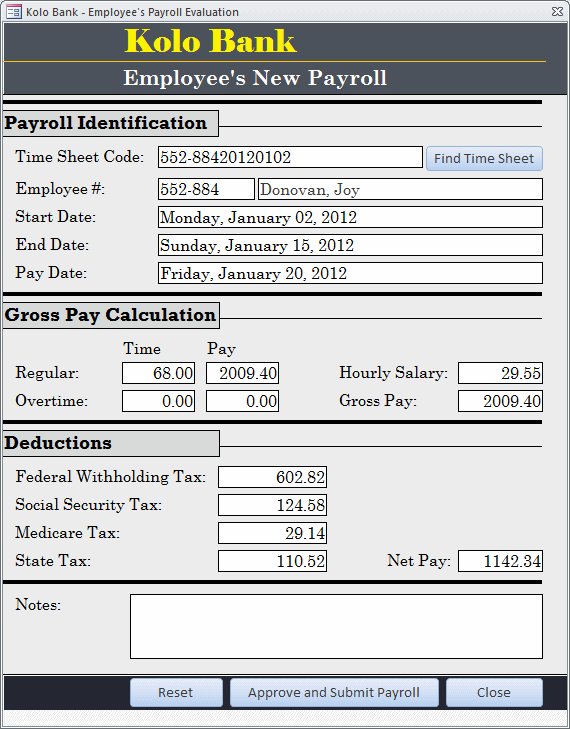
- Click Approve and Submit Payroll
- Click OK
- Enter the Time Sheet Code as 503-93820120102
- Click the Find Time Sheet button
- In the Federal Tax text box, type 510.58 and
press Tab
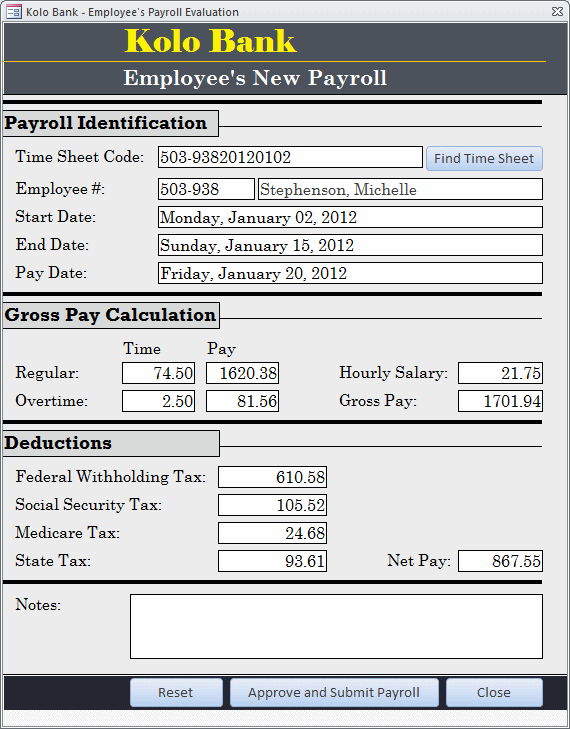
- Click Approve and Submit Payroll
- Click OK
- Enter the Time Sheet Code as 660-02620120102
- Click the Find Time Sheet button
- In the Federal Tax text box, type 155.90
- Click Approve and Submit Payroll
- Enter the Time Sheet Code as 503-93820120116
- Click the Find Time Sheet button
- In the Federal Tax text box, type 708.78 and
press Tab:
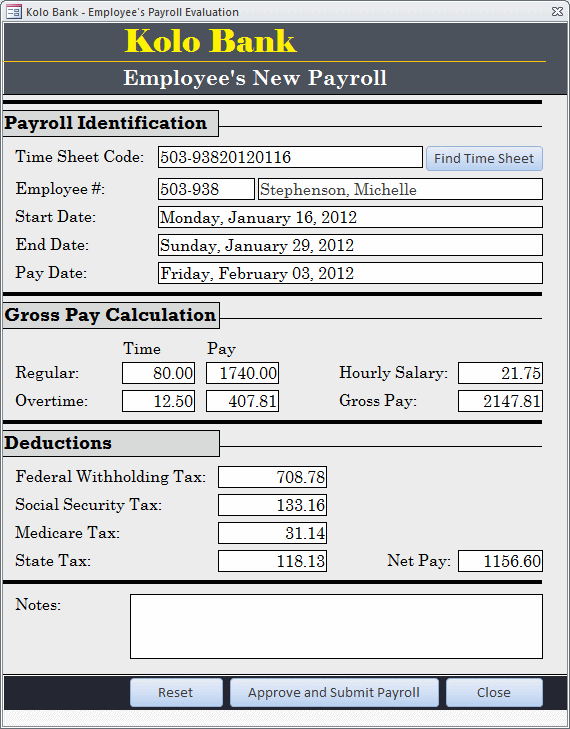
- Click Approve and Submit Payroll
- Click OK
- Enter the Time Sheet Code as 552-88420120116
- Click the Find Time Sheet button
- In the Federal Tax text box, type 695.90 and
press Tab
- Click Approve and Submit Payroll
- Click OK
- Enter the Time Sheet Code as 952-84620120116
- Click the Find Time Sheet button
- In the Federal Tax text box, type 978.96 and
press Tab
- Click Approve and Submit Payroll
- Enter the Time Sheet Code as 503-93820120130
- Click the Find Time Sheet button
- In the Federal Tax text box, type 835.20 and
press Tab
- Click Approve and Submit Payroll
- Click OK
- Close the form