Introducing XML Structure
|
|
File processing consists of:
- Creating values in a document and saving those values in a file
- Opening a file to retrieve some values stored in it
In the same way, you can create XML to be stored in a
file, or you can open an XML document to read its content and use it as you
see fit.
Introduction to XML Elements
|
|
An XML document is made of sections called elements. An
XML file can have as many elements as possible. This means that they can
range in the dozens, hundreds, or thousands. The operating system has the
responsibility of being able to handle the file if it gets exceedingly
large. To rightly play its role, an element must follow some rules.
An XML element is classically made of three parts, two
of which are called tags: a start tag and an end tag. A start tag is made of
three parts: <, followed by a name, and ending with >. An example is
<DEPARTMENT>
The name must follow some rules:
- A name can start with a letter or an underscore (_)
- After the starting letter or underscore, the name can contain
letters, underscores (_), digits (1, 2, 3, 4, 5, 6, 7, 8, 9, or 0),
hyphens (-), and periods (.)
- A name must not include anything that is not in the above two rules.
This means that a name cannot have empty spaces and cannot have special
characters
- A name is case-sensitive. This means that tag, TAG, and Tag are
different
As started above, an element must have a start tag and
an end tag. The end tag appears like the start tag but must include a
forward slash after the <. This also means that the end tag must have the
same name as the start tag. An example is:
</DEPARTMENT>
Between the start tag and the end tag, an element can
have a value. An example is:
<DEPARTMENT>Environmental Protection Agency</DEPARTMENT>
This can be created in file processing as follows:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Departments1.xml" For Output As #1
Print #1, "<DEPARTMENT>Environmental Protection Agency</DEPARTMENT>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
We stated that an element could have a value. This means
that some elements do not have a value. An example is:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Departments2.xml" For Output As #1
Print #1, "<DEPARTMENT></DEPARTMENT>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
This is called an empty element. When an element does
not have a value, you can use one tag that would act as the start and end
tags. In this case, omit the end tag but, between the start tag's name and
>, add a forward slash. An example is:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Departments3.xml" For Output As #1
Print #1, "<DEPARTMENT/>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
It is usually a good idea to add an empty space before
the forward slash to make it easy to read. Here is an example:
<DEPARTMENT />
To resume, an XML element can be made of:
An XML file is made of elements created using regular
text characters. When an application or an environment wants to use that
file, it must be able to recognize the various elements. The program that
must read and analyze the content of the XML document is called a parser.
The values of some of the elements in an XML document
may not be in regular US English, which means that they may use
International characters or symbols, referred to as Unicode. Some other
values may include complex or special characters. One way to assist the
parser is to indicate whether the document uses only regular (US English)
words or regular and Unicode characters. To give such an instruction, you
must add a line in the top section of the document. Such a line is called an
XML declaration. An XML declaration is not required in an XML document,
especially if you know that the parsers that will use your XML file can read
it without a problem. In most cases, although the XML declaration is not
required, you don’t lose anything by adding it.
An XML declaration:
- Must appear as the first line in the XML document
- Is a special element with only a start tag
- Is an element that starts with <?xml and ends with
?>
- Must include a version number. The version number is created as
version="1.0". An example is:
<?xml version="1.0"?>
- As stated already, you can indicate the type of character scheme
applied to the letters in the document. This scheme is called an
encoding. To specify it, assign "UTF-8" or "UTF-16"
to the encoding word. Here is an example:
<?xml version="1.0" encoding="UTF-8"?>
To do this in a file processing code, you can include UTF-8
in single-quotes. Here is an example:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Departments4.xml" For Output As #1
Print #1, "<?xml version='1.0' encoding='UTF-8'?>"
Print #1, "<DEPARTMENT>Environmental Protection Agency</DEPARTMENT>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
You probably know already that, in the Visual Basic
language, to include a double-quote in your code, you can double it. This is
another way to apply the encoding. Other types of encodings can be used.
UTF-8 and UTF-16 are just two examples.
XML Elements and Their Relationships
|
|
Introduction to the Nodes of an XML Document
|
|
As mentioned already, an XML document contains one or a
series of elements. The elements follow one another like a bulleted list of
a text document. The document is organized like a tree (an upside-down
tree). For this reason, each part (or element) is referred to as a node.
An XML document can have just one element. Another XML
document can have as many elements as necessary. Here is an example:
<Employee>Frank Misma 10/04/2008 General Manager</Employee>
<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>
<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>
If an XML document contains more than one element and
those elements are on the same level (in later sections, or little by
little, we will find out what “level” means), the elements must be included
in another element. This is done as follows. Create a start tag. Add the
necessary elements, and close with an end tag that corresponds to the
earlier start tag. Here is an example:
<Identification>
<Employee>Frank Misma 10/04/2008 General Manager</Employee>
<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>
<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>
</Identification>
If an XML document contains many elements, there must be
a special element that starts the document. The start tag of that element is
in the beginning of the document. That element is referred to as the root of
the document. In the above example, <Identification> is the root of the
document. If the document contains an XML declaration, the root is created
just after the declaration. Here is an example:
<?xml version="1.0" encoding=”UTF-8”?>
<Identification>
<Employee>Frank Misma 10/04/2008 General Manager</Employee>
<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>
<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>
</Identification>
This can be created in a file as follows:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Departments5.xml" For Output As #1
Print #1, "<?xml version=""1.0"" encoding=""UTF-8""?>"
Print #1, "<Identification>"
Print #1, "<Employee>Frank Misma 10/04/2008 General Manager</Employee>"
Print #1, "<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>"
Print #1, "<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>"
Print #1, "</Identification>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
The Parent Node of an Element
|
|
An element can be created inside of another element.
Here is an example:
<Identification>
<Employee>Frank Misma</Employee>
</Identification>
In this case, the <Employee> element is created inside
the <Identification> Element. We also say that the <Employee> element is
nested in the <Identification> element. As a result, the <Employee> element
is considered, or referred to as, the parent of the <Identification>
Element. In the same way, a parent of a node can be a parent of another
node. An element can be a parent of a node that itself is a parent.
Indentation consists of aligning the elements of a
document to make it easy to read. To make it possible, the elements are
considered by levels. The root node is the first level. Any node created
inside of it is considered in the second or next level. Subsequently, other
nodes can be created inside of other nodes.
Indentation consists of leaving empty spaces on the left
side of an element with regards to the parent node. The typical number of
empty spaces is 2. Normally, the XML declaration and the root node should be
on the same level. Here is an example:
<?xml version="1.0" encoding=”UTF-8”?>
<Identification>
<Employee>Frank Misma 10/04/2008 General Manager</Employee>
<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>
<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>
</Identification>
If you decide to indent by 4 characters, this is
equivalent to pressing Tab. Here is an example:
<?xml version="1.0" encoding=”UTF-8”?>
<Identification>
<Employee>Frank Misma 10/04/2008 General Manager</Employee>
<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>
<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>
</Identification>
You can use indentation in your text editor. Indentation
is not necessary if you are creating your XML file in Microsoft Access.
The Child Nodes (or Children) of a Parent
|
|
As mentioned already, an element can be created inside
another element. This is also referred to as nesting an element. This means
that the element created inside is said to be nested. The element that
encloses another is referred to as the parent. The element created inside is
referred to as a child element or a child node. Consider the following
example:
<Identification>
<Employee>Frank Misma</Employee>
</Identification>
In this case, the <Employee> node is the child of the
<Identification> node. In the same way, you can create as many child nodes
to a parent as you want. Here is an example we saw already:
<?xml version="1.0" encoding=”UTF-8”?>
<Identification>
<Employee>Frank Misma 10/04/2008 General Manager</Employee>
<Employee>Justine Grand 05/30/2012 Sales Associates</Employee>
<Employee>Arnold Alley 5/2/2010 Webmaster</Employee>
</Identification>
In this case, the parent <Identification> node has three
children that are all named Employee. The child nodes of a parent can have
different names even if they are on the same level. Of course, a child node
can have only one parent, but a parent can have as many children as
necessary.
An element can be created as a child of an element that
itself is a child of another element. In fact, this is a good feature to
organize the elements of an XML document by illustrating their dependencies.
This is also where indentation is important. Here are examples:
<?xml version="1.0" encoding="UTF-8"?>
<Employees>
<Employee>
<FirstName>Frank</FirstName>
<LastName>Misma</LastName>
<DateHired>10/04/2008</DateHired>
<Title>General Manager</Title>
</Employee>
<Employee>
<FirstName>Justine</FirstName>
<LastName>Grand</LastName>
<DateHired>05/30/2012</DateHired>
<Title>Sales Associates</Title>
</Employee>
<Employee>
<FirstName>Arnold</FirstName>
<LastName>Alley</LastName>
<DateHired>5/2/2010</DateHired>
<Title>Webmaster</Title>
</Employee>
</Employees>
The document can be created in file processing as
follows (indentation is used simply to make the document easy to read;
indentation is not required):
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Employees1.xml" For Output As #1
Print #1, "<?xml version='1.0' encoding='UTF-8'?>"
Print #1, "<Employees>"
Print #1, " <Employee>"
Print #1, " <FirstName>Frank</FirstName>"
Print #1, " <LastName>Misma</LastName>"
Print #1, " <DateHired>10/04/2008</DateHired>"
Print #1, " <Title>General Manager</Title>"
Print #1, " </Employee>"
Print #1, " <Employee>"
Print #1, " <FirstName>Justine</FirstName>"
Print #1, " <LastName>Grand</LastName>"
Print #1, " <DateHired>05/30/2012</DateHired>"
Print #1, " <Title>Sales Associates</Title>"
Print #1, " </Employee>"
Print #1, " <Employee>"
Print #1, " <FirstName>Arnold</FirstName>"
Print #1, " <LastName>Alley</LastName>"
Print #1, " <DateHired>5/2/2010</DateHired>"
Print #1, " <Title>Webmaster</Title>"
Print #1, " </Employee>"
Print #1, "</Employees>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
A child node is just another XML element. It must have a
start tag. It can be empty, which means it may not have a value, in which
case it may not have an end tag. Here are examples:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Employees2.xml" For Output As #1
Print #1, "<?xml version='1.0' encoding='UTF-8'?>"
Print #1, "<Employees>"
Print #1, " <Employee>"
Print #1, " <FirstName>Frank</FirstName>"
Print #1, " <LastName>Misma</LastName>"
Print #1, " <DateHired>10/04/2008</DateHired>"
Print #1, " <Title>General Manager</Title>"
Print #1, " </Employee>"
Print #1, " <Employee></Employee>"
Print #1, " <Employee>"
Print #1, " <FirstName>Justine</FirstName>"
Print #1, " <MiddleName></MiddleName>"
Print #1, " <LastName>Grand</LastName>"
Print #1, " <DateHired>05/30/2012</DateHired>"
Print #1, " <Title>Sales Associates</Title>"
Print #1, " </Employee>"
Print #1, " <Employee/>"
Print #1, " <Employee>"
Print #1, " <FirstName>Arnold</FirstName>"
Print #1, " <MiddleName />"
Print #1, " <LastName>Alley</LastName>"
Print #1, " <DateHired>5/2/2010</DateHired>"
Print #1, " <Title>Webmaster</Title>"
Print #1, " <Salary></Salary>"
Print #1, " </Employee>"
Print #1, "</Employees>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
The Value of a Parent Element
|
|
A parent node can have its own value. To specify its
value, type it just after the start tag and before its child node(s). Here
are two examples:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Employees3.xml" For Output As #1
Print #1, "<?xml version='1.0' encoding='UTF-8'?>"
Print #1, "<Employees>"
Print #1, " <Employee>"
Print #1, " <FirstName>Frank</FirstName>"
Print #1, " <LastName>Misma</LastName>"
Print #1, " <DateHired>10/04/2008</DateHired>"
Print #1, " <Title>General Manager</Title>"
Print #1, " </Employee>"
Print #1, " <Employee>First Sales Associate to be hired"
Print #1, " <FirstName>Justine</FirstName>"
Print #1, " <LastName>Grand</LastName>"
Print #1, " <DateHired>05/30/2012</DateHired>"
Print #1, " <Title>Sales Associates</Title>"
Print #1, " </Employee>"
Print #1, " <Employee>For the time being, the webmaster also acts as the application developer."
Print #1, " <FirstName>Arnold</FirstName>"
Print #1, " <LastName>Alley</LastName>"
Print #1, " <DateHired>5/2/2010</DateHired>"
Print #1, " <Title>Webmaster</Title>"
Print #1, " </Employee>"
Print #1, "</Employees>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
The Siblings of an Element
|
|
The elements on the same level are referred to as
siblings. Consider the following elements:
Private Sub cmdXMLElement_Click()
On Error GoTo cmdElement_Error
Open "Employees4.xml" For Output As #1
Print #1, "<?xml version="1.0" encoding=”UTF-8”?>"
Print #1, "<Employees>"
Print #1, " <Employee>"
Print #1, " <FirstName>Frank</FirstName>"
Print #1, " <LastName>Misma</LastName>"
Print #1, " <DateHired>10/04/2008</DateHired>"
Print #1, " <Title>General Manager</Title>"
Print #1, " </Employee>"
Print #1, " <Employee>"
Print #1, " <FirstName>Justine</FirstName>"
Print #1, " <LastName>Grand</LastName>"
Print #1, " <DateHired>05/30/2012</DateHired>"
Print #1, " <Title>Sales Associates</Title>"
Print #1, " <HourlySalary>22.15</HourlySalary>"
Print #1, " </Employee>"
Print #1, " <Contractor>"
Print #1, " <FullName>Arnold Alley</LastName>"
Print #1, " <Job>Webmaster</Job>"
Print #1, " </Contractor>"
Print #1, "</Employees>"
Close #1
Exit Sub
cmdElement_Error:
MsgBox "There was a problem when trying to create the XML file.", _
vbOKOnly Or vbInformation, _
"Extensible Markup Language"
Resume Next
End Sub
In this case, the <Employee> and the <Contractor>
elements are siblings. The <FirstName>, the <LastName, the <DateHired>, and
the <Title> elements of the first <Employee> node are siblings. In the same
way, the <FullName> and the <Job> nodes are sibling elements.
Practical
Learning: Creating an XML File
|
|
- In the Navigation pane, right-click the NewRepairOrder form and
click Design View
- On the form, right-click Save Repair Order and click Build Event
- In the Choose Builder dialog box, double-click Code Builder
- Implement the event as follows:
Private Sub cmdSaveRepairOder_Click()
On Error GoTo cmdSaveRepairOder_Error
Dim Action As Integer
Dim dlgSaveFile As FileDialog
Dim strProblemDescription As String
Dim strJobName1 As String, strPartName2 As String, strJobName2 As String
Dim strJobName3 As String, strJobName4 As String, strJobName5 As String
Set dlgSaveFile = Application.FileDialog(msoFileDialogSaveAs)
Action = dlgSaveFile.Show
If Action <> 0 Then
Open dlgSaveFile.SelectedItems(1) & ".xml" For Output As #1
Print #1, "<?xml version=""1.0"" encoding=""UTF-8""?>"
Print #1, "<RepairOrder>"
Print #1, "<CustomerName>" & txtCustomerName & "</CustomerName>"
Print #1, "<Address>" & txtAddress & "</Address>"
Print #1, "<City>" & txtCity & "</City>"
Print #1, "<State>" & txtState & "</State>"
Print #1, "<ZIPCode>" & txtZIPCode & "</ZIPCode>"
Print #1, "<Make>" & txtMake & "</Make>"
Print #1, "<Model>" & txtModel & "</Model>"
Print #1, "<CarYear>" & txtCarYear & "</CarYear>"
If IsNull(txtProblemDescription) Then
Print #1, "<ProblemDescription></ProblemDescription>"
Else
Print #1, "<ProblemDescription>" & Replace(txtProblemDescription, ",", ",") & "</ProblemDescription>"
End If
If IsNull(txtPartName1) Then
Print #1, "<PartName1></PartName1>"
Else
Print #1, "<PartName1>" & Replace(txtPartName1, ",", ",") & "</PartName1>"
End If
Print #1, "<UnitPrice1>" & txtUnitPrice1 & "</UnitPrice1>"
Print #1, "<Quantity1>" & txtQuantity1 & "</Quantity1>"
Print #1, "<SubTotal1>" & txtSubTotal1 & "</SubTotal1>"
If IsNull(txtPartName2) Then
Print #1, "<PartName2></PartName2>"
Else
Print #1, "<PartName2>" & Replace(txtPartName2, ",", ",") & "</PartName2>"
End If
Print #1, "<UnitPrice2>" & txtUnitPrice2 & "</UnitPrice2>"
Print #1, "<Quantity2>" & txtQuantity2 & "</Quantity2>"
Print #1, "<SubTotal2>" & txtSubTotal2 & "</SubTotal2>"
If IsNull(txtPartName3) Then
Print #1, "<PartName3></PartName3>"
Else
Print #1, "<PartName3>" & Replace(txtPartName3, ",", ",") & "</PartName3>"
End If
Print #1, "<UnitPrice3>" & txtUnitPrice3 & "</UnitPrice3>"
Print #1, "<Quantity3>" & txtQuantity3 & "</Quantity3>"
Print #1, "<SubTotal3>" & txtSubTotal3 & "</SubTotal3>"
If IsNull(txtPartName4) Then
Print #1, "<PartName4></PartName4>"
Else
Print #1, "<PartName4>" & Replace(txtPartName4, ",", ",") & "</PartName4>"
End If
Print #1, "<UnitPrice4>" & txtUnitPrice4 & "</UnitPrice4>"
Print #1, "<Quantity4>" & txtQuantity4 & "</Quantity4>"
Print #1, "<SubTotal4>" & txtSubTotal4 & "</SubTotal4>"
If IsNull(txtPartName5) Then
Print #1, "<PartName5></PartName5>"
Else
Print #1, "<PartName5>" & Replace(txtPartName5, ",", ",") & "</PartName5>"
End If
Print #1, "<UnitPrice5>" & txtUnitPrice5 & "</UnitPrice5>"
Print #1, "<Quantity5>" & txtQuantity5 & "</Quantity5>"
Print #1, "<SubTotal5>" & txtSubTotal5 & "</SubTotal5>"
If IsNull(txtJobName1) Then
Print #1, "<JobName1></JobName1>"
Else
Print #1, "<JobName1>" & Replace(txtJobName1, ",", ",") & "</JobName1>"
End If
Print #1, "<JobCost1>" & txtJobCost1 & "</JobCost1>"
If IsNull(txtJobName2) Then
Print #1, "<JobName2></JobName2>"
Else
Print #1, "<JobName2>" & Replace(txtJobName2, ",", ",") & "</JobName2>"
End If
Print #1, "<JobCost2>" & txtJobCost2 & "</JobCost2>"
If IsNull(txtJobName3) Then
Print #1, "<JobName3></JobName3>"
Else
Print #1, "<JobName3>" & Replace(txtJobName3, ",", ",") & "</JobName3>"
End If
Print #1, "<JobCost3>" & txtJobCost3 & "</JobCost3>"
If IsNull(txtJobName4) Then
Print #1, "<JobName4></JobName4>"
Else
Print #1, "<JobName4>" & Replace(txtJobName4, ",", ",") & "</JobName4>"
End If
Print #1, "<JobCost4>" & txtJobCost4 & "</JobCost4>"
If IsNull(txtJobName5) Then
Print #1, "<JobName5></JobName5>"
Else
Print #1, "<JobName5>" & Replace(txtJobName5, ",", ",") & "</JobName5>"
End If
Print #1, "<JobCost5>" & txtJobCost5 & "</JobCost5>"
Print #1, "<TotalParts>" & txtTotalParts & "</TotalParts>"
Print #1, "<TotalLabor>" & txtTotalLabor & "</TotalLabor>"
Print #1, "<TotalRepair>" & txtTotalRepair & "</TotalRepair>"
If IsNull(txtRecommendations) Then
Print #1, "<Recommendations></Recommendations>"
Else
Print #1, "<Recommendations>" & Replace(txtRecommendations, ",", ",") & "</Recommendations>"
End If
Print #1, "</RepairOrder>"
Close #1
End If
Set dlgSaveFile = Nothing
cmdResetForm_Click
Exit Sub
cmdSaveRepairOder_Error:
MsgBox "There is a problem with the repair order. It cannot be saved.", _
vbOKOnly Or vbInformation, _
"College Park Auto Repair"
Resume Next
End Sub
- Close the NewRepairOrder form
- When asked whether you want to save, click Yes
- In the Navigation pane, right-click the OpenRepairOrder form and
click Design View
- On the form, right-click Open Repair Order and click Build Event...
- In the Choose Builder dialog box, double-click Code Builder
- Implement the event as follows:
Option Compare Database
Option Explicit
Private strFileName As String
Private Sub cmdResetForm_Click()
. . . No Change
strFileName = ""
End Sub
Private Sub cmdOpenRepairOder_Click()
On Error GoTo cmdOpenRepairOder_Error
Dim Action As Integer
Dim dlgOpenFile As FileDialog
Dim strDeclaration As String, strRoot As String
Dim CustomerName As String, Address As String, City As String
Dim State As String, ZIPCode As String, Make As String, Model As String
Dim CarYear As String, ProblemDescription As String
Dim PartName1 As String, UnitPrice1 As String, Quantity1 As String
Dim SubTotal1 As String, PartName2 As String, UnitPrice2 As String
Dim Quantity2 As String, SubTotal2 As String, PartName3 As String
Dim UnitPrice3 As String, Quantity3 As String, SubTotal3 As String
Dim PartName4 As String, UnitPrice4 As String, Quantity4 As String
Dim SubTotal4 As String, PartName5 As String, UnitPrice5 As String
Dim Quantity5 As String, SubTotal5 As String
Dim JobName1 As String, JobCost1 As String
Dim JobName2 As String, JobCost2 As String
Dim JobName3 As String, JobCost3 As String
Dim JobName4 As String, JobCost4 As String
Dim JobName5 As String, JobCost5 As String
Dim TotalParts As String, TotalLabor As String
Dim TotalRepair As String, Recommendations As String
Set dlgOpenFile = Application.FileDialog(msoFileDialogOpen)
Action = dlgOpenFile.Show
If Action <> 0 Then
cmdResetForm_Click
Open dlgOpenFile.SelectedItems(1) For Input As #1
strFileName = dlgOpenFile.SelectedItems(1)
Input #1, strDeclaration
Input #1, strRoot
Input #1, CustomerName
Input #1, Address
Input #1, City
Input #1, State
Input #1, ZIPCode
Input #1, Make
Input #1, Model
Input #1, CarYear
Input #1, ProblemDescription
Input #1, PartName1
Input #1, UnitPrice1
Input #1, Quantity1
Input #1, SubTotal1
Input #1, PartName2
Input #1, UnitPrice2
Input #1, Quantity2
Input #1, SubTotal2
Input #1, PartName3
Input #1, UnitPrice3
Input #1, Quantity3
Input #1, SubTotal3
Input #1, PartName4
Input #1, UnitPrice4
Input #1, Quantity4
Input #1, SubTotal4
Input #1, PartName5
Input #1, UnitPrice5
Input #1, Quantity5
Input #1, SubTotal5
Input #1, JobName1
Input #1, JobCost1
Input #1, JobName2
Input #1, JobCost2
Input #1, JobName3
Input #1, JobCost3
Input #1, JobName4
Input #1, JobCost4
Input #1, JobName5
Input #1, JobCost5
Input #1, TotalParts
Input #1, TotalLabor
Input #1, TotalRepair
Input #1, Recommendations
txtCustomerName = Replace(Replace(Replace(CustomerName, "<CustomerName>", ""), "</CustomerName>", ""), ",", ",")
txtAddress = Replace(Replace(Replace(Address, "<Address>", ""), "</Address>", ""), ",", ",")
txtCity = Replace(Replace(Replace(City, "<City>", ""), "</City>", ""), ",", ",")
txtState = Replace(Replace(Replace(State, "<State>", ""), "</State>", ""), ",", ",")
txtZIPCode = Replace(Replace(ZIPCode, "<ZIPCode>", ""), "</ZIPCode>", "")
txtMake = Replace(Replace(Make, "<Make>", ""), "</Make>", "")
txtModel = Replace(Replace(Model, "<Model>", ""), "</Model>", "")
txtCarYear = Replace(Replace(CarYear, "<CarYear>", ""), "</CarYear>", "")
txtProblemDescription = Replace(Replace(Replace(ProblemDescription, "<ProblemDescription>", ""), "</ProblemDescription>", ""), ",", ",")
txtPartName1 = Replace(Replace(Replace(PartName1, "<PartName1>", ""), "</PartName1>", ""), ",", ",")
txtUnitPrice1 = Replace(Replace(UnitPrice1, "<UnitPrice1>", ""), "</UnitPrice1>", "")
txtQuantity1 = Replace(Replace(Quantity1, "<Quantity1>", ""), "</Quantity1>", "")
txtSubTotal1 = Replace(Replace(SubTotal1, "<SubTotal1>", ""), "</SubTotal1>", "")
txtPartName2 = Replace(Replace(Replace(PartName2, "<PartName2>", ""), "</PartName2>", ""), ",", ",")
txtUnitPrice2 = Replace(Replace(UnitPrice2, "<UnitPrice2>", ""), "</UnitPrice2>", "")
txtQuantity2 = Replace(Replace(Quantity2, "<Quantity2>", ""), "</Quantity2>", "")
txtSubTotal2 = Replace(Replace(SubTotal2, "<SubTotal2>", ""), "</SubTotal2>", "")
txtPartName3 = Replace(Replace(Replace(PartName3, "<PartName3>", ""), "</PartName3>", ""), ",", ",")
txtUnitPrice3 = Replace(Replace(UnitPrice3, "<UnitPrice3>", ""), "</UnitPrice3>", "")
txtQuantity3 = Replace(Replace(Quantity3, "<Quantity3>", ""), "</Quantity3>", "")
txtSubTotal3 = Replace(Replace(SubTotal3, "<SubTotal3>", ""), "</SubTotal3>", "")
txtPartName4 = Replace(Replace(Replace(PartName4, "<PartName4>", ""), "</PartName4>", ""), ",", ",")
txtUnitPrice4 = Replace(Replace(UnitPrice4, "<UnitPrice4>", ""), "</UnitPrice4>", "")
txtQuantity4 = Replace(Replace(Quantity4, "<Quantity4>", ""), "</Quantity4>", "")
txtSubTotal4 = Replace(Replace(SubTotal4, "<SubTotal4>", ""), "</SubTotal4>", "")
txtPartName5 = Replace(Replace(Replace(PartName5, "<PartName5>", ""), "</PartName5>", ""), ",", ",")
txtUnitPrice5 = Replace(Replace(UnitPrice5, "<UnitPrice5>", ""), "</UnitPrice5>", "")
txtQuantity5 = Replace(Replace(Quantity5, "<Quantity5>", ""), "</Quantity5>", "")
txtSubTotal5 = Replace(Replace(SubTotal5, "<SubTotal5>", ""), "</SubTotal5>", "")
txtJobName1 = Replace(Replace(Replace(JobName1, "<JobName1>", ""), "</JobName1>", ""), ",", ",")
txtJobCost1 = Replace(Replace(JobCost1, "<JobCost1>", ""), "</JobCost1>", "")
txtJobName2 = Replace(Replace(Replace(JobName2, "<JobName2>", ""), "</JobName2>", ""), ",", ",")
txtJobCost2 = Replace(Replace(JobCost2, "<JobCost2>", ""), "</JobCost2>", "")
txtJobName3 = Replace(Replace(Replace(JobName3, "<JobName3>", ""), "</JobName3>", ""), ",", ",")
txtJobCost3 = Replace(Replace(JobCost3, "<JobCost3>", ""), "</JobCost3>", "")
txtJobName4 = Replace(Replace(Replace(JobName4, "<JobName4>", ""), "</JobName4>", ""), ",", ",")
txtJobCost4 = Replace(Replace(JobCost4, "<JobCost4>", ""), "</JobCost4>", "")
txtJobName5 = Replace(Replace(Replace(JobName5, "<JobName5>", ""), "</JobName5>", ""), ",", ",")
txtJobCost5 = Replace(Replace(JobCost5, "<JobCost5>", ""), "</JobCost5>", "")
txtTotalParts = Replace(Replace(TotalParts, "<TotalParts>", ""), "</TotalParts>", "")
txtTotalLabor = Replace(Replace(TotalLabor, "<TotalLabor>", ""), "</TotalLabor>", "")
txtTotalRepair = Replace(Replace(TotalRepair, "<TotalRepair>", ""), "</TotalRepair>", "")
txtRecommendations = Replace(Replace(Replace(Recommendations, "<Recommendations>", ""), "</Recommendations>", ""), ",", ",")
Close #1
End If
Set dlgOpenFile = Nothing
Exit Sub
cmdOpenRepairOder_Error:
MsgBox "There is a problem with the repair order. It cannot be opened.", _
vbOKOnly Or vbInformation, _
"College Park Auto Repair"
Resume Next
End Sub
Private Sub cmdResetForm_Click()
. . . No Change
strFileName = ""
End Sub
- In the Object combo box, select cmdUpdateRepairOrder
- Implement the event as follows:
Private Sub cmdUpdateRepairOrder_Click()
On Error GoTo cmdUpdateRepairOder_Error
If strFileName <> "" Then
Open strFileName For Output As #1
Print #1, "<?xml version=""1.0"" encoding=""UTF-8""?>"
Print #1, "<RepairOrder>"
Print #1, "<CustomerName>" & txtCustomerName & "</CustomerName>"
Print #1, "<Address>" & txtAddress & "</Address>"
Print #1, "<City>" & txtCity & "</City>"
Print #1, "<State>" & txtState & "</State>"
Print #1, "<ZIPCode>" & txtZIPCode & "</ZIPCode>"
Print #1, "<Make>" & txtMake & "</Make>"
Print #1, "<Model>" & txtModel & "</Model>"
Print #1, "<CarYear>" & txtCarYear & "</CarYear>"
Print #1, "<ProblemDescription>" & Replace(txtProblemDescription, ",", ",") & "</ProblemDescription>"
Print #1, "<PartName1>" & Replace(txtPartName1, ",", ",") & "</PartName1>"
Print #1, "<UnitPrice1>" & txtUnitPrice1 & "</UnitPrice1>"
Print #1, "<Quantity1>" & txtQuantity1 & "</Quantity1>"
Print #1, "<SubTotal1>" & txtSubTotal1 & "</SubTotal1>"
Print #1, "<PartName2>" & Replace(txtPartName2, ",", ",") & "</PartName2>"
Print #1, "<UnitPrice2>" & txtUnitPrice2 & "</UnitPrice2>"
Print #1, "<Quantity2>" & txtQuantity2 & "</Quantity2>"
Print #1, "<SubTotal2>" & txtSubTotal2 & "</SubTotal2>"
Print #1, "<PartName3>" & Replace(txtPartName3, ",", ",") & "</PartName3>"
Print #1, "<UnitPrice3>" & txtUnitPrice3 & "</UnitPrice3>"
Print #1, "<Quantity3>" & txtQuantity3 & "</Quantity3>"
Print #1, "<SubTotal3>" & txtSubTotal3 & "</SubTotal3>"
Print #1, "<PartName4>" & Replace(txtPartName4, ",", ",") & "</PartName4>"
Print #1, "<UnitPrice4>" & txtUnitPrice4 & "</UnitPrice4>"
Print #1, "<Quantity4>" & txtQuantity4 & "</Quantity4>"
Print #1, "<SubTotal4>" & txtSubTotal4 & "</SubTotal4>"
Print #1, "<PartName5>" & Replace(txtPartName5, ",", ",") & "</PartName5>"
Print #1, "<UnitPrice5>" & txtUnitPrice5 & "</UnitPrice5>"
Print #1, "<Quantity5>" & txtQuantity5 & "</Quantity5>"
Print #1, "<SubTotal5>" & txtSubTotal5 & "</SubTotal5>"
Print #1, "<JobName1>" & Replace(txtJobName1, ",", ",") & "</JobName1>"
Print #1, "<JobCost1>" & txtJobCost1 & "</JobCost1>"
Print #1, "<JobName2>" & Replace(txtJobName2, ",", ",") & "</JobName2>"
Print #1, "<JobCost2>" & txtJobCost2 & "</JobCost2>"
Print #1, "<JobName3>" & Replace(txtJobName3, ",", ",") & "</JobName3>"
Print #1, "<JobCost3>" & txtJobCost3 & "</JobCost3>"
Print #1, "<JobName4>" & Replace(txtJobName4, ",", ",") & "</JobName4>"
Print #1, "<JobCost4>" & txtJobCost4 & "</JobCost4>"
Print #1, "<JobName5>" & Replace(txtJobName5, ",", ",") & "</JobName5>"
Print #1, "<JobCost5>" & txtJobCost5 & "</JobCost5>"
Print #1, "<TotalParts>" & txtTotalParts & "</TotalParts>"
Print #1, "<TotalLabor>" & txtTotalLabor & "</TotalLabor>"
Print #1, "<TotalRepair>" & txtTotalRepair & "</TotalRepair>"
Print #1, "<Recommendations>" & Replace(txtRecommendations, ",", ",") & "</Recommendations>"
Print #1, "</RepairOrder>"
Close #1
End If
Exit Sub
cmdUpdateRepairOder_Error:
MsgBox "There is a problem with the repair order. It cannot be updated.", _
vbOKOnly Or vbInformation, _
"College Park Auto Repair"
Resume Next
End Sub
- Close Microsoft Visual Basic and return to Microsoft Access
- Close the form
- When asked whether you want to save, click Yes
- In the Navigation pane, double-click the the NewRepairOrder form
- Complete it with a repair order. Here is an example:
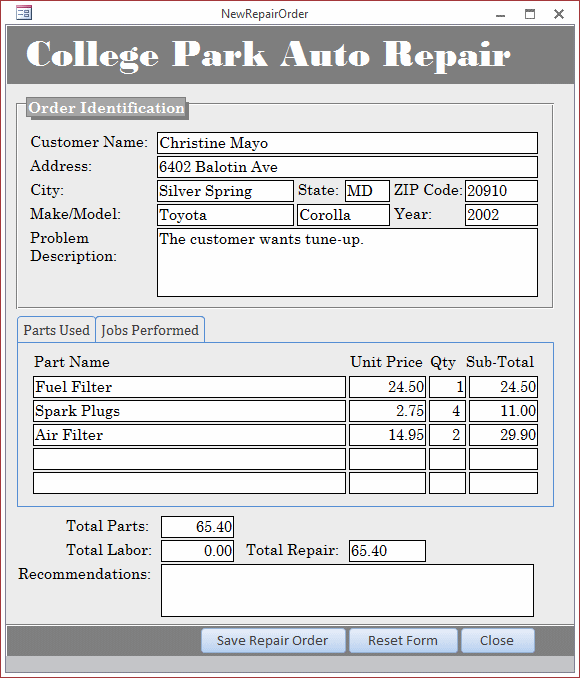
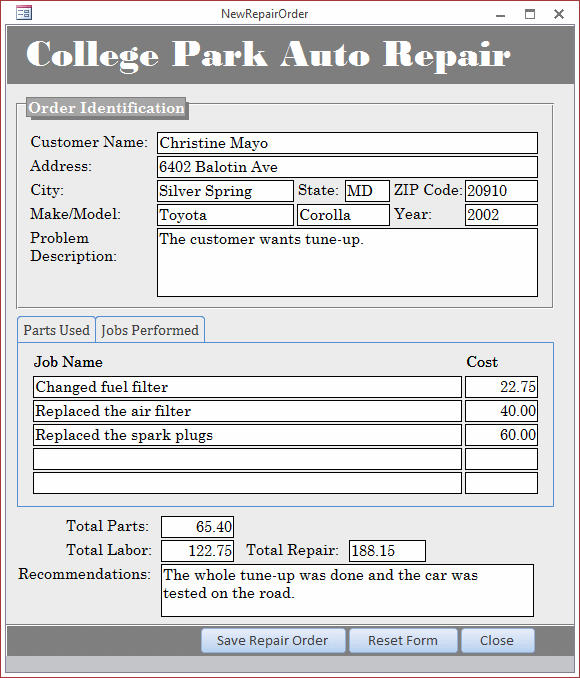
- Click Save Repair Order
- In the dialog box, set the file name using the initials of the
customer name (our example would be cm)
- Click Save
- Create another repair order. Here is an example:
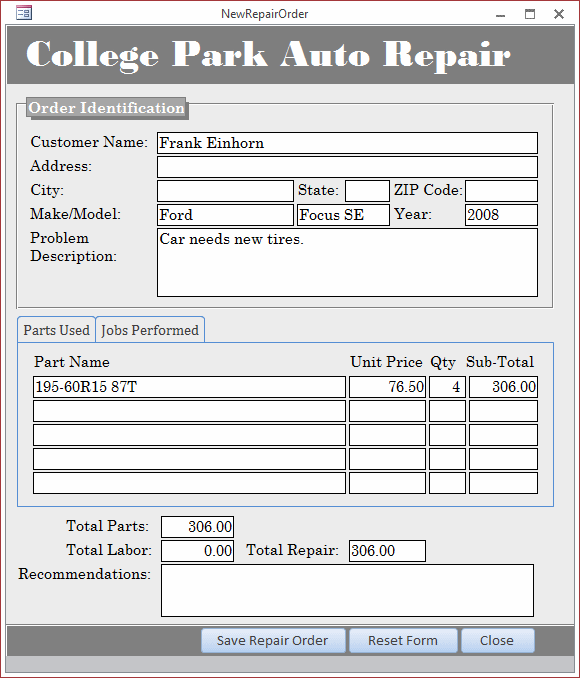
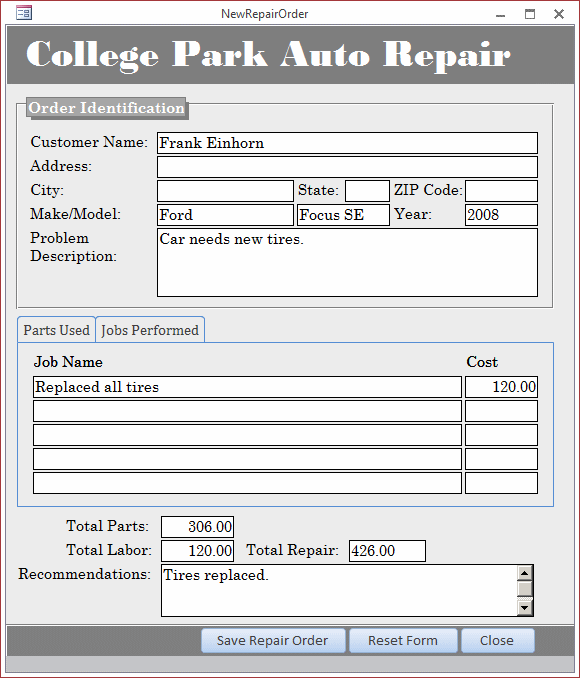
- Click Save Repair Order
- In the dialog box, set the file name using the initials of the
customer name (our example would be fe)
- Close the NewRepairOrder
- In the Navigation pane, double-click the OpenRepairOrder form
- Click the Open Repair Order button
- In the dialog box, select a file name you had saved (such as cm.xml)
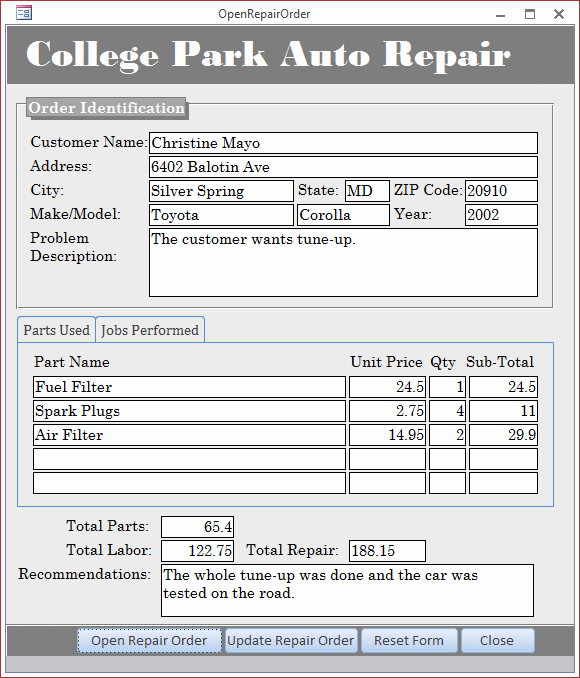
- Change some values in the form. Here is an example:
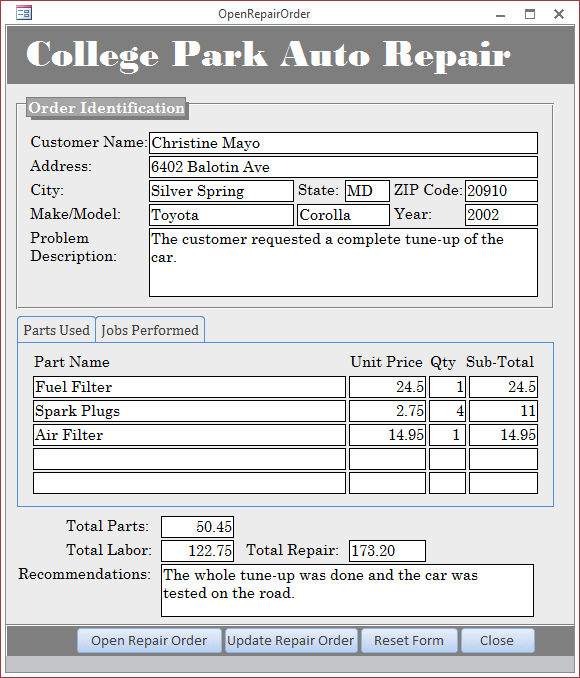
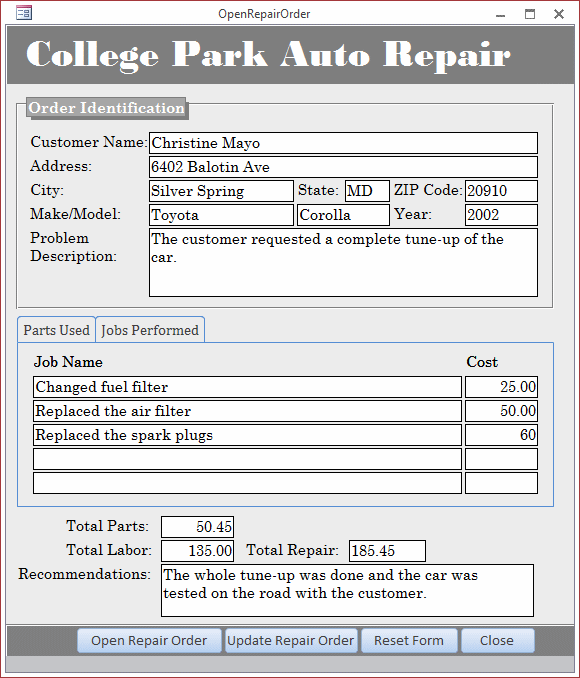
- Click Update Repair Order
- Click Open Repair Oder
- Select the other repair order
- Open the first repair order and make sure the changes you had made
were saved
- Close the form
|
|