In this example, we will calculate the overtime worked by an employee of a
company. To do this, we will consider each week day with 8 regular hours. If an
employee works more than 8 hours in one day, any time over that is considered
overtime. In our payroll simulation, we will cover two weeks but each week has
its separate calculation. After collecting the time for both weeks and
performing the calculation, we will display the number of regular hours, the
number of hours worked overtime, the amount pay for the regular hours, and the
amount pay for overtime, if any. At the end, we will display the total net pay.
Practical
Learning: Introducing the Date Picker
|
|
- Start a new Windows Application named Payroll1
- Set the form's Icon to Drive:\Program Files\Microsoft Visual Studio
.NET 2003\Common7\Graphics\icons\Office\CRDFLE03.ICO
- Design the form as follows:
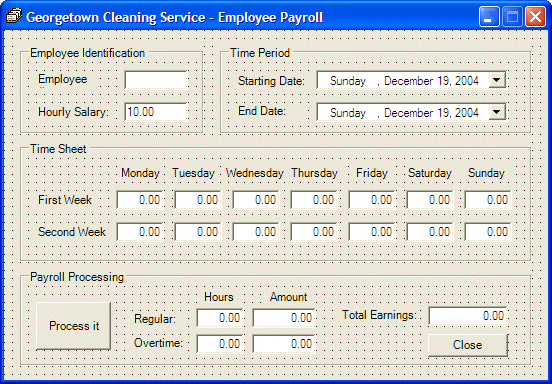 |
Control |
Name |
Text |
Other Properties |
GroupBox |
 |
|
Employee Identification |
|
Label |
 |
|
Employee #: |
|
TextBox |
 |
txtEmployeeNbr |
|
|
Label |
 |
|
Hourly Salary: |
|
TextBox |
 |
txtHourlySalary |
|
|
GroupBox |
 |
|
Time Period |
|
Label |
 |
Starting Date: |
|
|
DateTimePicker |
 |
dtpStartingDate |
|
|
Label |
 |
End Date: |
|
|
DateTimePicker |
 |
dtpEndDate |
|
|
GroupBox |
 |
|
Time Sheet |
|
Label |
 |
|
Monday |
|
Label |
 |
|
Tuesday |
|
Label |
 |
|
Wednesday |
|
Label |
 |
|
Thursday |
|
Label |
 |
|
Friday |
|
Label |
 |
|
Saturday |
|
Label |
 |
|
Sunday |
|
Label |
 |
|
First Week: |
|
TextBox |
 |
txtMonday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtTuesday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtWednesday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtThursday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtFriday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSaturday1 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSunday1 |
0.00 |
TextAlign: Right |
Label |
 |
|
Second Week: |
|
TextBox |
 |
txtMonday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtTuesday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtWednesday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtThursday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtFriday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSaturday2 |
0.00 |
TextAlign: Right |
TextBox |
 |
txtSunday2 |
0.00 |
TextAlign: Right |
GroupBox |
 |
|
Payroll Processing |
|
Label |
 |
|
Hours |
|
Label |
 |
|
Amount |
|
Button |
 |
btnProcessIt |
Process It |
|
Label |
 |
|
Regular |
|
TextBox |
 |
txtRegularHours |
0.00 |
TextAlign: Right |
TextBox |
 |
txtRegularAmount |
0.00 |
TextAlign: Right |
Label |
 |
|
Total Earnings |
|
TextBox |
 |
txtNetPay |
0.00 |
TextAlign: Right |
Label |
 |
|
Overtime |
|
TextBox |
 |
txtOvertimeHours |
0.00 |
TextAlign: Right |
TextBox |
 |
txtOvertiimeAmount |
0.00 |
TextAlign: Right |
Button |
 |
btnClose |
|
|
|
- Double-click the Close button and implement its Click event as follows:
Private Sub btnClose_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) _
Handles btnClose.Click
End
End Sub
|
- While still in the Code Editor, in the Class Name combo box, make sure
(Form1 Events) is selected
Click the arrow of the Method Name combo box and select Load
- Implement the Load event
as follows:
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) _
Handles MyBase.Load
Dim todayDate As DateTime = DateTime.Today
Dim twoWeeksAgo As DateTime = todayDate.AddDays(-14)
Me.dtpStartingDate.Value = twoWeeksAgo
End Sub
|
- In the Class Name combo box, select the dtpStartingDate
- Click the arrow of the Method Name combo box and select CloseUp
- Implement the event as follows:
Private Sub dtpStartingDate_CloseUp(ByVal sender As Object, ByVal e As System.EventArgs) _
Handles dtpStartingDate.CloseUp
' Get the starting date
Dim dteStart As DateTime = Me.dtpStartingDate.Value
' Find out if the user selected a day that is not Monday
If dteStart.DayOfWeek <> DayOfWeek.Monday Then
MsgBox("The date you selected in invalid\n" & _
"The time period should start on a Monday")
End If
Me.dtpStartingDate.Focus()
End Sub
|
- In the Class Name combo box, select the dtpEndDate
- Click the arrow of the Method Name combo box and select CloseUp
- Implement the event as follows:
Private Sub dtpEndDate_CloseUp(ByVal sender As Object, ByVal e As System.EventArgs) _
Handles dtpEndDate.CloseUp
' Get the starting date
Dim dteStart As DateTime = Me.dtpStartingDate.Value
' Get the ending date
Dim dteEnd As DateTime = Me.dtpEndDate.Value
' Make sure the first day of the period is Monday
If dteStart.DayOfWeek <> DayOfWeek.Monday Then
MsgBox("The starting date you selected in invalid\n" & _
"The time period should start on a Monday")
End If
Me.dtpStartingDate.Focus()
' Find the number of days that separate the start and end
Dim timeDifference As TimeSpan = dteEnd.Subtract(dteStart)
Dim fourteenDaysLater As Double = timeDifference.Days
If (dteEnd.DayOfWeek <> DayOfWeek.Sunday) Or (fourteenDaysLater <> 13) Then
MsgBox("The ending date you selected in invalid\n" & _
"The time period should span 2 weeks and end on a Sunday")
End If
Me.dtpEndDate.Focus()
End Sub
|
- In the Class Name combo box, select the btnProcessIt
- Click the arrow of the Method Name combo box and select Click
- Implement the Click event as follows:
Private Sub btnProcessIt_Click(ByVal sender As Object, ByVal e As System.EventArgs) _
Handles btnProcessIt.Click
Dim monday1 As Double
Dim tuesday1 As Double
Dim wednesday1 As Double
Dim thursday1 As Double
Dim friday1 As Double
Dim saturday1 As Double
Dim sunday1 As Double
Dim monday2 As Double
Dim tuesday2 As Double
Dim wednesday2 As Double
Dim thursday2 As Double
Dim friday2 As Double
Dim saturday2 As Double
Dim sunday2 As Double
Dim totalHoursWeek1 As Double
Dim totalHoursWeek2 As Double
Dim regHours1 As Double
Dim regHours2 As Double
Dim ovtHours1 As Double
Dim ovtHours2 As Double
Dim regAmount1 As Double
Dim regAmount2 As Double
Dim ovtAmount1 As Double
Dim ovtAmount2 As Double
Dim regularHours As Double
Dim overtimeHours As Double
Dim regularAmount As Double
Dim overtimeAmount As Double
Dim totalEarnings As Double
Dim hourlySalary As Double
' Retrieve the hourly salary
hourlySalary = CDbl(Me.txtHourlySalary.Text)
' Retrieve the time for each day
' First Week
monday1 = CDbl(Me.txtMonday1.Text)
tuesday1 = CDbl(Me.txtTuesday1.Text)
wednesday1 = CDbl(Me.txtWednesday1.Text)
thursday1 = CDbl(Me.txtThursday1.Text)
friday1 = CDbl(Me.txtFriday1.Text)
saturday1 = CDbl(Me.txtSaturday1.Text)
sunday1 = CDbl(Me.txtSunday1.Text)
' Second Week
monday2 = CDbl(Me.txtMonday2.Text)
tuesday2 = CDbl(Me.txtTuesday2.Text)
wednesday2 = CDbl(Me.txtWednesday2.Text)
thursday2 = CDbl(Me.txtThursday2.Text)
friday2 = CDbl(Me.txtFriday2.Text)
saturday2 = CDbl(Me.txtSaturday2.Text)
sunday2 = CDbl(Me.txtSunday2.Text)
' Calculate the total number of hours for each week
totalHoursWeek1 = monday1 + tuesday1 + wednesday1 + thursday1 + _
friday1 + saturday1 + sunday1
totalHoursWeek2 = monday2 + tuesday2 + wednesday2 + thursday2 + _
friday2 + saturday2 + sunday2
' The overtime is paid time and half
Dim ovtSalary As Double
ovtSalary = hourlySalary * 1.5
' If the employee worked under 40 hours, there is no overtime
If totalHoursWeek1 < 40 Then
regHours1 = totalHoursWeek1
regAmount1 = hourlySalary * regHours1
ovtHours1 = 0
ovtAmount1 = 0
' If the employee worked over 40 hours, calculate the overtime
ElseIf totalHoursWeek1 >= 40 Then
regHours1 = 40
regAmount1 = hourlySalary * 40
ovtHours1 = totalHoursWeek1 - 40
ovtAmount1 = ovtHours1 * ovtSalary
End If
If totalHoursWeek2 < 40 Then
regHours2 = totalHoursWeek2
regAmount2 = hourlySalary * regHours2
ovtHours2 = 0
ovtAmount2 = 0
ElseIf totalHoursWeek2 >= 40 Then
regHours2 = 40
regAmount2 = hourlySalary * 40
ovtHours2 = totalHoursWeek2 - 40
ovtAmount2 = ovtHours2 * ovtSalary
End If
regularHours = regHours1 + regHours2
overtimeHours = ovtHours1 + ovtHours2
regularAmount = regAmount1 + regAmount2
overtimeAmount = ovtAmount1 + ovtAmount2
totalEarnings = regularAmount + overtimeAmount
Me.txtRegularHours.Text = regularHours.ToString("F")
Me.txtOvertimeHours.Text = overtimeHours.ToString("F")
Me.txtRegularAmount.Text = regularAmount.ToString("C")
Me.txtOvertimeAmount.Text = overtimeAmount.ToString("C")
Me.txtNetPay.Text = totalEarnings.ToString("C")
End Sub
|
- Execute the application to test it
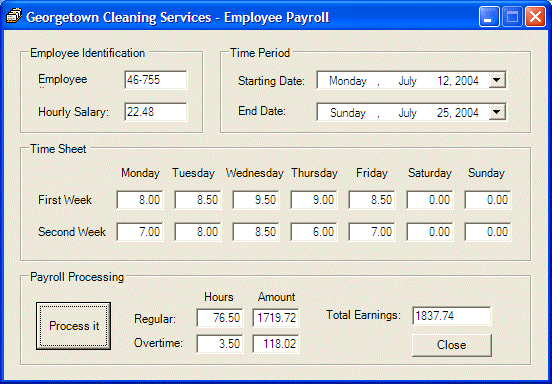
- After using it, close the form and return to your programming environment
|
|