Introduction to the Items of a Toolbar
By default, just after you have added a toolbar to your
form, it is empty, waiting for you to add objects to it. To hold its
collection of objects, the ToolStrip class is equipped with
a property named Items. The ToolStrip.Items
property is an object of type ToolStripItemCollection,
which itself is a collection class. Each member of the
ToolStripItemCollection class is of type ToolStripItem.
During desgin, to add the desired items:
- Click the arrowed button on its top-right side and click Edit Items:
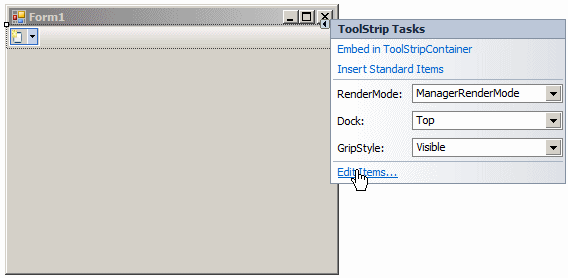
- Right-click the toolbar and click Edit Items...
- Click the toolbar on the form to select it. Then, under the
Properties window, click Edit Items
Any of these actions would open the Items Collection
Editor where you can individually select items, click Add, and configure
them:

Instead of adding objects one by one, Microsoft Visual
Studio provides a default or standard design for a toolbar. To use it, after
visually adding the toolbar to a form:
- Click the arrowed button on it top-right side and click Insert
Standard Items
- Right-click the toolbar and click Insert Standard Items
- Click the toolbar on the form to select it. Then, under the
Properties window, click Insert Standard Items
Any of these actions would equip the toolbar with the
most common buttons used on a standard toolbar.
The Buttons of a Toolbar
The most common object of a toolbar is a button. If you
are working visually, to add a button to a toolbar, click the toolbar. An
icon would display. Click that button:
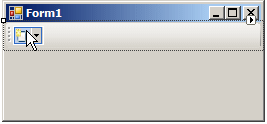
A button of a toolbar is based on a class named
ToolStripButton. The ToolStripButton clas is
derived from the ToolStripItem class which, as mentioned
before, is represented by the Items property that is a member of the
ToolStrip class.
To programmatically create a toolbar button, declare a
variable of this class. The ToolStripButton class is
equipped with 6 constructors. You can use the default constructor if you are
not ready to provide the other pieces of information a button may need.
After declaring the variable, add it to the Items property of its container.
Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripButton btnCreate;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
btnCreate = new ToolStripButton();
toolbar.Items.Add(btnCreate);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
By default, a button of a toolbar displays an icon on
top. If you click the toolstrip to create a button, the new button receives
a default icon:
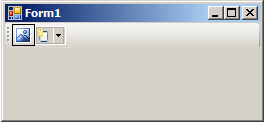
To specify or change the icon of a button, after
clicking it, in the Properties window, click Image, then click its browse
button. In the Select Resource dialog box, click Import, locate the desired
icon, select it, and click OK. To programmatically specify the icon of a
button, you have many options. The ToolStripButton class is
equipped with a property named Image. Assign an icon to this property. Here
is an example:
void InitializeComponent()
{
toolbar = new ToolStrip();
btnCreate = new ToolStripButton();
btnCreate.Image = Image.FromFile(@"C:\Icons Collection\create.ico");
toolbar.Items.Add(btnCreate);
Controls.Add(toolbar);
}
The ToolStripButton class provides
another constructor whose syntax is:
public ToolStripButton(Image image);
When creating a button, you can use this constructor to
directly specify its icon. Here is an example:
void InitializeComponent()
{
toolbar = new ToolStrip();
btnCreate = new ToolStripButton(Image.FromFile(@"C:\Icons Collection\create.ico"));
toolbar.Items.Add(btnCreate);
Controls.Add(toolbar);
}
If an icon is not explicit enough, you can make an icon
display text or to display a combination of an icon and text. To support
this, the ToolStripItem class provides a property named
DisplayStyle. This property is of type
ToolStripItemDisplayStyle, which is an enumeration. The members of
the ToolStripItemDisplayStyle are:
- None: The button will display neither an icon nor
text
- Text: The button will display text only
- Image: The button will display an icon only
- ImageAndText: The button will display an icon and
text
To visually configure this behavior, access the
Properties window for the button and, in the DisplayStyle
field, select the desired member. To do this programmatically, access the
DisplayStyle property and assign the desired value to it.
If you want a button to display text, first select
either Text or ImageAndText for the
DisplayStyle property. To visually specify the text to
display, type it in the Text property. To do it programmatically, you have
various options. You can assign a string to the Text property of the button.
Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripButton btnCreate;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
btnCreate = new ToolStripButton();
btnCreate.DisplayStyle = ToolStripItemDisplayStyle.Text;
btnCreate.Text = "Create";
toolbar.Items.Add(btnCreate);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
This would produce:
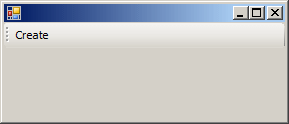
The ToolStripButton class is equipped with another
constructor whose syntax is:
public ToolStripButton(string text);
You can use this constructor to create a toolbar and
specify its text. Of course, you must specify an appropriate value for the
DisplayStyle property. Here is an example:
void InitializeComponent()
{
toolbar = new ToolStrip();
btnCreate = new ToolStripButton("Create");
btnCreate.DisplayStyle = ToolStripItemDisplayStyle.Text;
toolbar.Items.Add(btnCreate);
Controls.Add(toolbar);
}
To display both an icon and text, select the appropriate
value of the DisplayStyle property. Then, in the Properties
window of the button, specify the icon and enter the desired text.
Otherwise, the ToolStripButton class provides another
constructor whose syntax is:
public ToolStripButton(string text, Image image);
If you decide to use this version, pass the appropriate
arguments.
To use a button, the user can click it. Therefore, you
should create an event handler for the button. You can use the Events
section of the Properties window or programmatically write it. Here is an
example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripButton btnCreate;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
btnCreate = new ToolStripButton("Create");
btnCreate.DisplayStyle = ToolStripItemDisplayStyle.Text;
btnCreate.Click += new EventHandler(btnCreateClick);
toolbar.Items.Add(btnCreate);
Controls.Add(toolbar);
}
private void btnCreateClick(object sender, EventArgs e)
{
MessageBox.Show("The document will be created");
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
Otherwise, the ToolStripButton class
supports all the normal events of a button.
Menu Items on a Toolbar
Instead of a classic button, you can create a type of
menu on a toolbar so that, when the user clicks the button, a menu would
come up. To get it:
- Click an empty area on the toolbar to create a new button. Click the
arrow on the right side of the new button and click DropDownButton
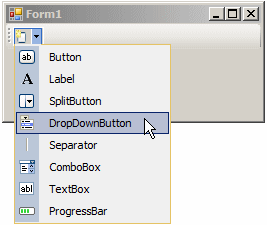
- Display or Access the Properties window for the toolbar. In Select
Item And Add To List Below, select DropDownButton and click Add
The button that is equipped with a menu is available
through a class named ToolStripDropDownButton. This class
inherits from a class named ToolStripDropDownItem.
The ToolStripDropDownButton class has
seven constructors. To programmatically create a drop down button, you can
declare a variable of type ToolStripDropDownButton using
its default constructor, allocate memory for it using the new
operator, and add it to the Items collection of the ToolStrip
variable. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripDropDownButton ddRecentlyUsed;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
ddRecentlyUsed = new ToolStripDropDownButton();
toolbar.Items.Add(ddRecentlyUsed);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
The primary characteristic of a drop down button is that
it is equipped with a menu. To visually create the menu, after adding the
button, it displays a text box labeled Type Here, just like we saw for the
menu items. Alternatively, click the drop down button on the toolbar. In the
Properties window, click DropDownItems, then click its browse button to open
the Items Collection Editor.
You programmatically create the menu items of a drop
down button like we saw for the menu items. After creating them, add them to
the DropDownItems collection of the drop down button. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripDropDownButton ddRecentlyUsed;
ToolStripMenuItem ddiFirst;
ToolStripMenuItem ddiSecond;
ToolStripMenuItem ddiThird;
ToolStripMenuItem ddiFourth;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
ddRecentlyUsed = new ToolStripDropDownButton();
ddRecentlyUsed.Image = Image.FromFile(@"C:\Exercises\recent.ico");
ddRecentlyUsed.DisplayStyle = ToolStripItemDisplayStyle.Image;
ddiFirst = new ToolStripMenuItem("First");
ddiSecond = new ToolStripMenuItem("Second");
ddiThird = new ToolStripMenuItem("Third");
ddiFourth = new ToolStripMenuItem("Fourth");
ddRecentlyUsed.DropDownItems.Add(ddiFirst);
ddRecentlyUsed.DropDownItems.Add(ddiSecond);
ddRecentlyUsed.DropDownItems.Add(ddiThird);
ddRecentlyUsed.DropDownItems.Add(ddiFourth);
toolbar.Items.Add(ddRecentlyUsed);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
This would produce:
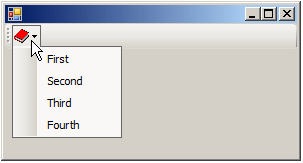
The menu items of a drop down button behave like the
menu items we saw in Lessons 10-12. Based on this, you can configure the
items of the button the same way we reviewed for menu items.
Separators on a Tool Bar
A separator is a vertical bar that is used to create
sections or groups of items on a toolbar. There is no strict rule as to
where to put a separator. Only your experience and needs will guide you.
To create a separator:
- Click an empty area on the toolbar to create a new button. Click the
arrow on the right side of the new button and click Separator
- Display the Properties window for the toolbar. In Select Item And
Add To List Below, select Separator and click Add
Practical Learning:
Adding Buttons to a Toolbar
- On the form, right-click the tool strip and click Insert Standard
Items
- Under the form, click tbrStandard. Under the Properties window,
click Edit Items
- Change the names of the items as follows:
Old Name |
New Name |
newToolStripButton |
tbrStandardNew |
openToolStripButton |
tbrStandardOpen |
saveToolStripButton |
tbrStandardSave |
printToolStripButton |
tbrStandardPrint |
Separator |
|
cutToolStripButton |
tbrStandardCut |
copyToolStripButton |
tbrStandardCopy |
pasteToolStripButton |
tbrStandardPaste |
Separator |
|
helpToolStripButton |
tbrStandardHelp |
- Click OK
The Split Button on a Toolbar
When the user clicks a drop down button, its menu
automatically displays. As an alternative, you can create a button split by
a bar, showing a normal button on the left and a menu on the right. To
support this, the .NET Framework provides the ToolStripSplitButton
class. This class is represented by a control named split button. To
visually add a split button to a toolbar:
- Click an empty area on the toolbar to create a new button. Click the
arrow on the right side of the new button and click SplitButton
- Display the Properties window for the toolbar. In Select Item And
Add To List Below, select SplitButton and click Add
To programmatically create a split button, declare a
variable of type ToolStripSplitButton and add it to the
Items property of the toolbar. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripSplitButton btnTypesOfApplications;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
btnTypesOfApplications = new ToolStripSplitButton();
toolbar.Items.Add(btnTypesOfApplications);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
There are two ways a user can use a split button, by
clicking the button itself on the left or the arrow on the right. When the
arrow button is clicked, a menu displays. The menu items that display are of
type ToolStripItem. This means that you create them using
the same descriptions we saw for menus.
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripSplitButton btnTypesOfApplications;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
btnTypesOfApplications = new ToolStripSplitButton();
btnTypesOfApplications.Image = Image.FromFile(@"C:\Exercise\apps.ico");
btnTypesOfApplications.DisplayStyle = ToolStripItemDisplayStyle.Image;
btnTypesOfApplications.DropDownItems.Add(new ToolStripMenuItem("Work Processing"));
btnTypesOfApplications.DropDownItems.Add(new ToolStripMenuItem("Spreadsheet"));
btnTypesOfApplications.DropDownItems.Add(new ToolStripMenuItem("Databases"));
btnTypesOfApplications.DropDownItems.Add(new ToolStripMenuItem("Presentation"));
toolbar.Items.Add(btnTypesOfApplications);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
Labels on a Toolbar
You can create a section on a toolbar to display text.
To support this, the .NET Framework provides the ToolStripLabel
class, which is derived from the ToolStripItem class.
To visually create a label:
- Click an empty area on the toolbar to create a new button. Then
click the arrow of the new button and click Label
- Access the Properties window for the toolbar. In Select Item And Add
To List Below, select Label and click Add
As its name indicates, a label is meant to display text.
After adding it to a toolbar, assign a string to its Text
property.
Instead of simple text, you can make a label behave like
a link. To support this, the ToolStripLabel class is
equipped with a Boolean property named IsLink. If you set
this property to true, the label would follow the standard description of a
web link (without the link itself; you must find a way to make it active the
desired link).
A Text Box on a Toolbar
You can add a text box to a toolbar so the user can type
something in it or read text from it. To make this possible, you can use a
class named ToolStripTextBox. To add a text box to a
toolbar:
- Click an empty area on the toolbar to create a new button. Then
click the arrow of the new button and click TextBox
- Display the Access the Properties window for the toolbar. In Select
Item And Add To List Below, select TextBox and click Add
To programmatically add a textbox to a toolbar, declare
a variable of type ToolStripTextBox. The
ToolStripTextBox class is equipped with 3 constructors. The default
constructor is used to create a normal and simple text box. After declaring
and initializing the variable, add it to the Items property
of the toolstrip. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripTextBox txtFullName;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
txtFullName = new ToolStripTextBox();
toolbar.Items.Add(txtFullName);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
This would produce:
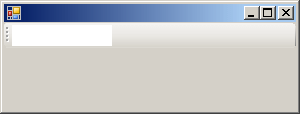
Most of the time, you create an empty text box in which
a user can enter text. At any time, if you want to display text in the text
box, assign a string to the Text property of the button. This can be done as
follows:
void InitializeComponent()
{
toolbar = new ToolStrip();
txtFullName = new ToolStripTextBox();
txtFullName.Text = "John Doe";
toolbar.Items.Add(txtFullName);
Controls.Add(toolbar);
}
The text box of a toolbar uses the normal features of a
Microsoft Windows text box. At the risk of repeating ourselves, we reviewed
it in Lesson 13.
A Combo Box on a Toolbar
You can add a combo box to a toolbar to provide a list
from which the user can select an item. The combo box is handled by the
ToolStripComboBox class. To add visually add a combo box to
a toolbar:
- Click an empty area on the toolbar to create a new button. Then
click the arrow of the new button and click ComboBox:
- Display the Properties window for the toolbar. In Select Item And
Add To List Below, select ComboBox and click Add
To programmatically create a combo box, declare a
variable of type ToolStripComboBox. The
ToolStripComboBox class has 3 constructors. Use the default
constructor to create a combo box. Initialize the variable and add it to the
Items property of the toolbar. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripComboBox cbxCategories;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
cbxCategories = new ToolStripComboBox();
toolbar.Items.Add(cbxCategories);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
This would produce:
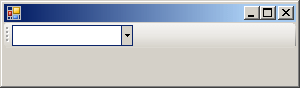
One of the properties of the combo box of a toolbar is
named ComboBox. This property is of type ComboBox. This property can be
assigned a predefined object that has all the desired and necessary
characteristics of a Microsoft Windows combo box. At the risk of repeating
ourselves, we will review the characteristics of a combo box in another
lesson.
A Progress Bar on a Tool Bar
A progress bar is used to show the evolution of
something by drawing continuous rectangles, usually blue. To support them on
a toolbar, the .NET Framework provides the ToolStripProgressBar
class. This class inherits from the ToolStripControlHost
class.
To visuall add a progress bar on a toolbar:
- Click an empty area on the toolbar to create a new button. Then
click the arrow of the new button and click ProgressBar
- Display the Properties window for the toolbar. In Select Item And
Add To List Below, select ProgressBar and click Add
To programmatically create a progress bar to be hosted
by a toolbar, declare a variable of type ToolStripProgressBar.
The ToolStripProgressBar class has two constructors. You
can use the default constructor to declare the variable, initialize it using
the new operator, and add it to the Items property of a
ToolStrip variable. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
ToolStrip toolbar;
ToolStripProgressBar pgrEvolution;
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
toolbar = new ToolStrip();
pgrEvolution = new ToolStripProgressBar();
toolbar.Items.Add(pgrEvolution);
Controls.Add(toolbar);
}
[STAThread]
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
This would produce:
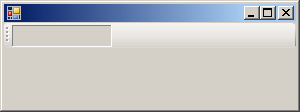
After creating a progress bar, you must find a way to
make it display its rectangle (and why).
|