This exercise applies the concepts reviewed for drawing shapes. In this
case, we will draw some rectangles to product a (vertical) column chart.
Practical
Learning: Drawing a Rectangle
|
|
- Start a new Windows Forms Application named WeeklySales1
- Set the form's Icon to Drive:\Program Files\Microsoft Visual Studio .NET 2003\Common7\Graphics\icons\Office\GRAPH07.ICO
- Design the form as follows:
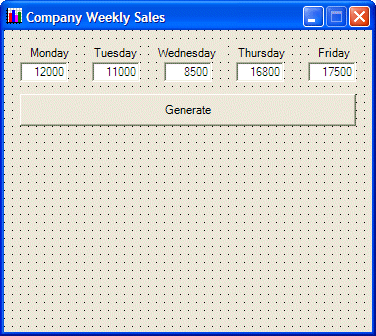 |
Control |
Name |
Text |
Other Properties |
Label |
 |
|
Monday |
|
Label |
 |
|
Tuesday |
|
Label |
 |
|
Wednesday |
|
Label |
 |
|
Thursday |
|
Label |
 |
|
Friday |
|
TextBox |
 |
txtMonday |
12000 |
TextAlign: Right |
TextBox |
 |
txtTuesday |
11000 |
TextAlign: Right |
TextBox |
 |
txtWednesday |
8500 |
TextAlign: Right |
TextBox |
 |
txtThursday |
16800 |
TextAlign: Right |
TextBox |
 |
txtFriday |
17500 |
TextAlign: Right |
Button |
 |
Generate |
btnGenerate |
|
|
- Double-click an unoccupied area on the form and implement its Load event
as follows:
. . . No Change
namespace WeeklySales1a
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
. . . No Change
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
Graphics graphDrawingArea;
Bitmap bmpDrawingArea;
. . . No Change
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
. . . No Change
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Load(object sender, System.EventArgs e)
{
bmpDrawingArea = new Bitmap(Width, Height);
graphDrawingArea = Graphics.FromImage(bmpDrawingArea);
}
}
}
|
- Return to the form and click an empty area on it. In the Properties
window, click the Events button

- Double-click the Paint field and implement its event as follows:
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
e.Graphics.DrawImage(bmpDrawingArea, 0, 0);
}
|
- Double-click the Generate button and implement its Click event as follows:
private void btnGenerate_Click(object sender, System.EventArgs e)
{
int monday = int.Parse(this.txtMonday.Text) / 100;
int tuesday = int.Parse(this.txtTuesday.Text) / 100;
int wednesday = int.Parse(this.txtWednesday.Text) / 100;
int thursday = int.Parse(this.txtThursday.Text) / 100;
int friday = int.Parse(this.txtFriday.Text) / 100;
graphDrawingArea.Clear(this.BackColor);
graphDrawingArea.DrawRectangle(new Pen(Color.Red),
this.txtMonday.Left+5, 280-monday, 40, monday);
graphDrawingArea.DrawRectangle(new Pen(Color.Blue),
this.txtTuesday.Left+5, 280-tuesday, 40, tuesday);
graphDrawingArea.DrawRectangle(new Pen(Color.Fuchsia),
this.txtWednesday.Left+5, 280-wednesday, 40, wednesday);
graphDrawingArea.DrawRectangle(new Pen(Color.Brown),
this.txtThursday.Left+5, 280-thursday, 40, thursday);
graphDrawingArea.DrawRectangle(new Pen(Color.Turquoise),
this.txtFriday.Left+5, 280-friday, 40, friday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black), 10, 280, Width - 30, 1);
Invalidate();
}
|
- Execute the application and test the form
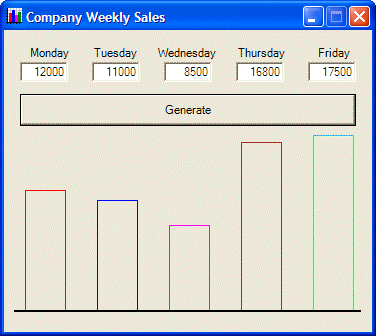
- After using it, close the form
- Change the implementation of the Click event of the Generate button as follows:
private void btnGenerate_Click(object sender, System.EventArgs e)
{
int monday = int.Parse(this.txtMonday.Text) / 100;
int tuesday = int.Parse(this.txtTuesday.Text) / 100;
int wednesday = int.Parse(this.txtWednesday.Text) / 100;
int thursday = int.Parse(this.txtThursday.Text) / 100;
int friday = int.Parse(this.txtFriday.Text) / 100;
graphDrawingArea.Clear(this.BackColor);
graphDrawingArea.FillRectangle(new SolidBrush(Color.Red),
this.txtMonday.Left+5, 280-monday, 40, monday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black),
this.txtMonday.Left+5, 280-monday, 40, monday);
graphDrawingArea.FillRectangle(new SolidBrush(Color.Blue),
this.txtTuesday.Left+5, 280-tuesday, 40, tuesday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black),
this.txtTuesday.Left+5, 280-tuesday, 40, tuesday);
graphDrawingArea.FillRectangle(new SolidBrush(Color.Fuchsia),
this.txtWednesday.Left+5, 280-wednesday, 40, wednesday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black),
this.txtWednesday.Left+5, 280-wednesday, 40, wednesday);
graphDrawingArea.FillRectangle(new SolidBrush(Color.Brown),
this.txtThursday.Left+5, 280-thursday, 40, thursday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black),
this.txtThursday.Left+5, 280-thursday, 40, thursday);
graphDrawingArea.FillRectangle(new SolidBrush(Color.Turquoise),
this.txtFriday.Left+5, 280-friday, 40, friday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black),
this.txtFriday.Left+5, 280-friday, 40, friday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black), 10, 280, Width - 30, 1);
Invalidate();
}
|
- Execute the application and test the form

- After using it, close the form
|
|