This exercise is meant to apply the concepts of a Button
control. It is used to process simple orders for a cleaning store. |
Practical Learning: Introducing Buttons
|
|
- Start a new Windows Application named GCS1
- Set the form's icon to Drive:\Program Files\Microsoft Visual Studio .NET 2003\Common7\Graphics\icons\Misc\BULLSEYE.ICO
- Design the form as follows:
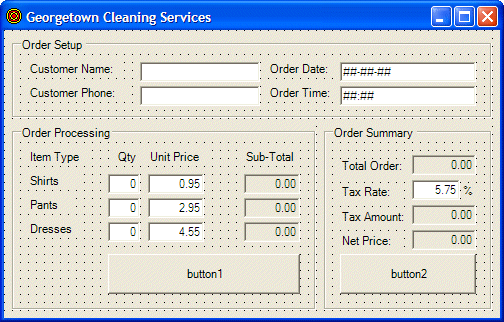 |
Control |
Name |
Text |
Additional Properties |
GroupBox |
 |
|
Order Setup |
|
Label |
 |
|
Customer Name: |
|
TextBox |
 |
txtCustName |
|
|
Label |
 |
|
Order Date: |
|
MaskedEdBox |
 |
txtOrderDate |
|
Format: dddd dd mmmm yyyy
Mask: ##-##-## |
Label |
 |
|
Order Time: |
|
MaskedEdBox |
 |
txtOrderTime |
|
Format: hh:mm AM/PM
Mask: ##:## |
GroupBox |
 |
|
Order Processing |
|
Label |
 |
|
Item Type |
|
Label |
 |
|
Qty |
|
Label |
 |
|
Unit Price |
|
Label |
 |
|
Sub Total |
|
Label |
 |
|
Shirts |
|
TextBox |
 |
txtQtyShirts |
0 |
TextAlign: Right |
TextBox |
 |
txtPriceShirts |
0.95 |
TextAlign: Right |
TextBox |
 |
txtTotalShirts |
0.00 |
TextAlign: Right
ReadOnly: True |
Label |
 |
|
Pants |
|
TextBox |
 |
txtQtyPants |
0 |
TextAlign: Right |
TextBox |
 |
txtPricePants |
2.95 |
TextAlign: Right |
TextBox |
 |
txtTotalPants |
0.00 |
TextAlign: Right
ReadOnly: True |
Label |
 |
|
Dresses |
|
TextBox |
 |
txtQtyDresses |
0 |
TextAlign: Right |
TextBox |
 |
txtPriceDresses |
2.95 |
TextAlign: Right |
TextBox |
 |
txtTotaldresses |
4.55 |
TextAlign: Right
ReadOnly: True |
Button |
 |
btnCalculate |
Calculate |
|
GroupBox |
 |
|
Order Summary |
|
Label |
 |
|
Total Order |
|
TextBox |
 |
txtTotalOrder |
0.00 |
TextAlign: Right
ReadOnly: True |
Label |
 |
|
TaxRate |
|
TextBox |
 |
txtTaxRate |
5.75 |
TextAlign: Right |
Label |
 |
|
% |
|
Label |
 |
|
Tax Amount |
|
TextBox |
 |
txtTaxAmount |
0.00 |
TextAlign: Right
ReadOnly: True |
Label |
 |
|
Net Price |
|
TextBox |
 |
txtNetPrice |
0.00 |
TextAlign: Right
ReadOnly: True |
Button |
 |
btnClose |
Close |
|
|
- Save All
- On the main menu, click File -> New -> File...
- In the New File dialog box, double-click Icon File
- Right-click a white area in the drawing section, position the mouse on
Current Icon Image Types, and click 16x16, 16 Colors
- Save it as Calculate in the main folder of the current project
- Change its design as follows:

- Right-click a white area in the drawing section, position the mouse on
Current Icon Image Types, and click 32x32, 16 Colors
- Right-click a white area in the drawing section and click Delete Image
Type
- Save the icon
- On the main menu, click Project -> Add New Item...
- In the Add New Item dialog box, click Icon File
- Change the name to SubTotal and click Open
- Right-click a white area in the drawing section, position the mouse on
Current Icon Image Types, and click 16x16, 16 Colors
- Change its design as follows:
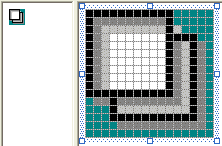
- Right-click a white area in the drawing section, position the mouse on
Current Icon Image Types, and click 32x32, 16 Colors
- Right-click a white area in the drawing section and click Delete Image
Type
- Save the icon
- Add three buttons inside the lower-left group box and delete the content
of their Text property
- Complete the design of the form as follows:
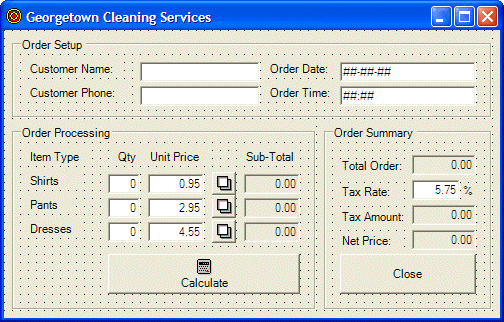 |
Button Name |
Image |
ImageAlign |
TextAlign |
btnShirts |
SubTotal |
|
|
btnPants |
SubTotal |
|
|
btnDresses |
SubTotal |
|
|
btnCalculate |
Calculate |
TopCenter |
BottomCenter |
|
- On the main menu, click View Tab Order and click the controls in the
following order (observe the sequence in the Order Processing group box)
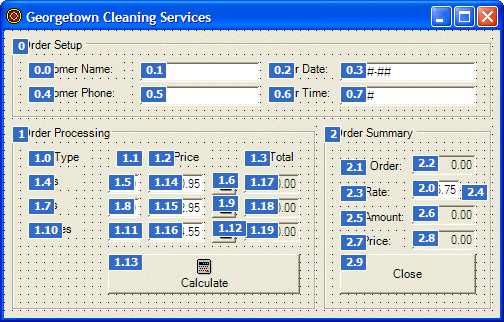
- Save all
- Double-click the button for the shirts and implement its Click event as
follows:
private void btnShirts_Click(object sender, System.EventArgs e)
{
int quantity = 1;
double unitPrice = 0.00, subTotal;
// Retrieve the number of this item
// Just in case the user types an invalid value, we are using a try...catch
try
{
quantity = int.Parse(this->txtQtyShirts->Text);
}
catch(FormatException)
{
MessageBox::Show("The value you entered for the number of shirts is not valid" +
"\nPlease try again");
}
// Retrieve the unit price of this item
// Just in case the user types an invalid value, we are using a try...catch
try
{
unitPrice = double.Parse(this->txtPriceShirts->Text);
}
catch(FormatException)
{
MessageBox::Show("The value you entered for the price of shirts is not valid" +
"\nPlease try again");
}
// Calculate the sub-total for this item
subTotal = quantity * unitPrice;
// Display the sub-total in the corresponding text box
this->txtTotalShirts->Text = subTotal.ToString("F");
}
|
- Double-click the button for the pants and implement its Click event as
follows:
private void btnPants_Click(object sender, System.EventArgs e)
{
int quantity = 1;
double unitPrice = 0.00, subTotal;
try
{
quantity = int.Parse(this->txtQtyPants->Text);
}
catch(FormatException)
{
MessageBox::Show("The value you entered for the number of pants is not valid" +
"\nPlease try again");
}
try
{
unitPrice = double.Parse(this->txtPricePants->Text);
}
catch(FormatException)
{
MessageBox::Show("The value you entered for the price of pants is not valid" +
"\nPlease try again");
}
subTotal = quantity * unitPrice;
this->txtTotalPants->Text = subTotal.ToString("F");
}
|
- Double-click the button for the dresses and implement its Click event as
follows:
private void btnDresses_Click(object sender, System.EventArgs e)
{
int quantity = 1;
double unitPrice = 0.00, subTotal;
try
{
quantity = int.Parse(this->txtQtyDresses->Text);
}
catch(FormatException)
{
MessageBox::Show("The value you entered for the number of dresses is not valid" +
"\nPlease try again");
}
try
{
unitPrice = double.Parse(this->txtPriceDresses->Text);
}
catch(FormatException)
{
MessageBox::Show("The value you entered for the price of dresses is not valid" +
"\nPlease try again");
}
subTotal = quantity * unitPrice;
this->txtTotalDresses->Text = subTotal.ToString("F");
}
|
- Double-click the Calculate button and implement its Click event as
follows:
private void btnCalculate_Click(object sender, System.EventArgs e)
{
double priceShirts, pricePants, priceDresses, totalOrder;
double taxRate = 0.00, taxAmount;
double netPrice;
// Retrieve the value of the sub-total for each category of items
priceShirts = double.Parse(this->txtTotalShirts->Text);
pricePants = double.Parse(this->txtTotalPants->Text);
priceDresses = double.Parse(this->txtTotalDresses->Text);
// Calculate the total
totalOrder = priceShirts + pricePants + priceDresses;
// Retrieve the value of the tax rate
try
{
taxRate = double.Parse(this->txtTaxRate->Text);
}
catch(FormatException)
{
MessageBox::Show("The tax rate you entered is invalid" +
"\nPlease try again");
}
// Calculate the amount owed for the taxes
taxAmount = totalOrder * taxRate / 100;
// Add the tax amount to the total order
netPrice = totalOrder + taxAmount;
// Display the values of the order summary
this->txtTotalOrder->Text = totalOrder.ToString("C");
this->txtTaxAmount->Text = taxAmount.ToString("C");
this->txtNetPrice->Text = netPrice.ToString("C");
}
|
- Double-click the Close button and implement its Click event as follows:
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
|
- Execute the application to test the form. In the Order Date text box, type
081103 and in the Order Time, type 0728 then complete the rest of the order
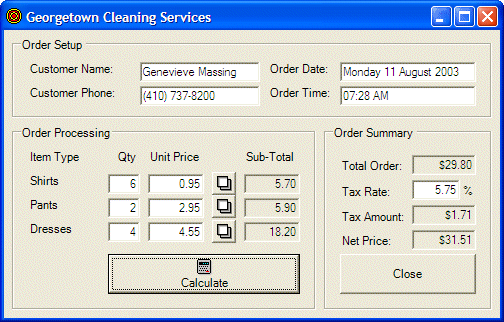
- Close the form and return to your programming environment
|
|