The .Net provides a special property used to count a specific number of lapses that have occurred since you started your computer. This information or counter is available through the
Environment.TickCount property.
This property provides the number of milliseconds that have elapsed since you started your computer. Just like the timer control, what you do with the result is up to you and it can be used in various circumstances. For example, computer games and simulations make great use of this function.
After retrieving the value that this property provides, you can display it in a text-based control. |
- Start Microsoft Visual C# and create a Windows Application named TickCounter
- Design the form as follows:

- Add a timer control to the form (it will be positioned in the lower
section of the view). Set its Interval to 20 and its Enabled to
True
- Declare a global private integer named CompTime
- Double-click the form to get its Load event
- Initialize the CompTime variable as follows:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace TickCounter
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.Button btnClose;
private System.Windows.Forms.Timer timer1;
private System.Windows.Forms.GroupBox groupBox1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label1;
private System.ComponentModel.IContainer components;
private System.Int32 CompTime;
public Form1()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
. . .
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Load(object sender, System.EventArgs e)
{
CompTime = Environment.TickCount;
}
}
}
|
- Double-click the timer1 control and implement its Timer event as follows:
private void timer1_Tick(object sender, System.EventArgs e)
{
long CurTickValue = Environment.TickCount;
long Difference = CurTickValue - CompTime;
label1.Text = String.Format("This computer has been ON for {0}", CurTickValue.ToString());
label2.Text = String.Format("This application has been running for {0}", Difference.ToString());
}
|
- Double-click the Close button and implement it as follows:
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
|
- Test the application
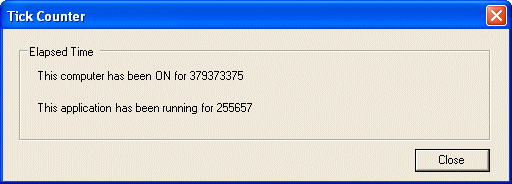
- After testing the application, close it
- To make the values easier to read, change the code of the OnTimer event as follows:
private void timer1_Tick(object sender, System.EventArgs e)
{
long CurTickValue = Environment.TickCount;
long Difference = CurTickValue - CompTime;
long ComputerHours, ComputerMinutes, ComputerSeconds;
long ApplicationHours, ApplicationMinutes, ApplicationSeconds;
ComputerHours = (CurTickValue / (3600 * 999)) % 24;
ComputerMinutes = (CurTickValue / (60 * 999)) % 60;
ComputerSeconds = (CurTickValue / 999) % 60;
ApplicationHours = (Difference / (3600 * 999)) % 24;
ApplicationMinutes = (Difference / (60 * 999)) % 60;
ApplicationSeconds = (Difference / 999) % 60;
label1.Text = String.Format("This computer has been ON for {0} hours, {1} minutes {2} seconds",
ComputerHours.ToString(),
ComputerMinutes.ToString(),
ComputerSeconds.ToString());
label2.Text = String.Format("This application has been running for {0} hours, {1} minutes {2} seconds",
ApplicationHours.ToString(),
ApplicationMinutes.ToString(),
ApplicationSeconds.ToString());
}
|
- Test the application:
- After testing the application, close it
|
|