An ellipse is a closed continuous line whose points are
positioned so that two points exactly opposite each other have the exact same
distant from a central point. It can be illustrated as follows:
Because an ellipse can fit in a rectangle, in GDI+
programming, an ellipse is defined with regards to a rectangle it would fit in.
To draw an ellipse, you can use the Graphics.DrawEllipse() method that comes in
four versions whose syntaxes are:
public void DrawEllipse(Pen pen, Rectangle rect);
public void DrawEllipse(Pen pen, RectangleF rect);
public void DrawEllipse(Pen pen, int x, int y, int width, int height);
public void DrawEllipse(Pen pen, float x, float y, float width, float height);
The arguments of this function play the same roll as those of
the Graphics.DrawRectangle() method:
Here is an example:
|
private void button1_Click(object sender, System.EventArgs e)
{
Graphics graph = this.CreateGraphics();
Pen penCurrent = new Pen(Color.Red);
graph.DrawEllipse(penCurrent, new Rectangle(20, 20, 226, 144));
}
|
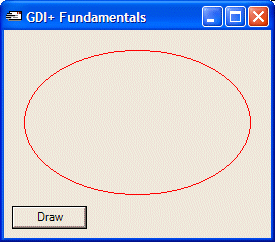 |
A pie is a fraction of an ellipse delimited by two lines
that span from the center of the ellipse to one side each. It can be illustrated
as follows:
Like an ellipse (and like an arc), a pie is meant to fit in
a rectangle. Based on this, a pie is delimited by a rectangular shaped that
defines its location and the dimensions it would fit in.
To draw a pie, you can use the Graphics.DrawPie()
method that comes in various versions as follows:
public void DrawPie(Pen pen,
Rectangle rect,
float startAngle,
float sweepAngle);
public void DrawPie(Pen pen,
RectangleF rect,
float startAngle,
float sweepAngle);
public void DrawPie(Pen pen,
int x,
int y,
int width,
int height,
int startAngle,
int sweepAngle);
public void DrawPie(Pen pen,
float x,
float y,
float width,
float height,
float startAngle,
float sweepAngle);
The first two versions allow you to specify a
rectangle that would enclose the pie. The other two versions allow you to
specify the location (with x and y) and dimensions of the pie. Besides the borders of the rectangle in which the
pie would fit, a pie must specify its starting angle, startAngle, measured
clockwise from the x-axis its starting point. A pie must also determine its
sweep angle measured clockwise from the startAngle parameter to the end
of the pie.
Here is an example:
|
private void button1_Click(object sender, System.EventArgs e)
{
Graphics graph = this.CreateGraphics();
Pen penCurrent = new Pen(Color.Red);
graph.DrawPie(penCurrent, 20, 20, 200, 100, 45, 255);
}
This would produce:
|
|