Providing help is one of the most neglected areas of development, especially
for beginning programmers. There are various reasons to this. Some programmers
tend to think that help is such a small job that it should be left to
non-programmers. This has never been a valid argument since the person who
creates an application knows it better than anybody. This means that asking
someone else to create help for an application would only slow the process because
the other person would need to have new and more information about the
application. Another reason is that, most programmers who want to take care of
programming when creating an application think that they are working double to
create a single program. This drives them to set this aspect aside and
continue only with the application, thinking that they can come back to taking
care of help when the application is complete. What makes this unrealistic is
that, sometimes when they finish the application, they are "tired".
There are various small but effective techniques you can use to provide help in your
application: never neglect help.
Practical
Learning: Introducing Help
|
|
- Start a Windows Application named CIC1
- In Solution Explorer, double-click App.ico and change its design as
follows:

- Right-click an empty area in the design section, position the mouse
on Current Icon Image Types, and click 16x16, 16 colors
- Design the 16x16 version of the icon as follows:

- Close the App.ico tab
- In the Properties window, change the form's Text to Clarksville Ice
Cream
- Still in the Properties window, click the Icon field and click its
ellipsis button. Then select the App.ico
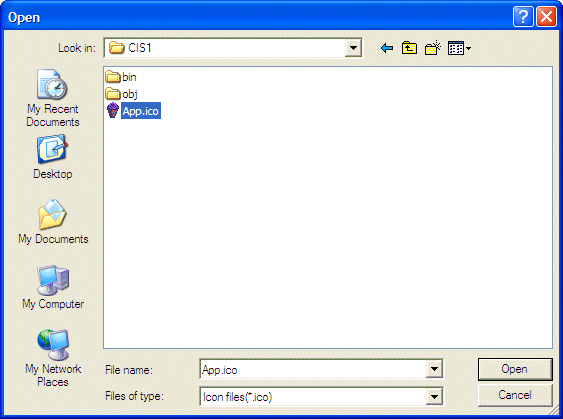
- Click Open
- Design the form as follows:
 |
Control |
Name |
Text |
Additional Properties |
GroupBox |
|
|
|
Label |
|
Order Date: |
|
DateTimePicker |
dtpOrderDate |
|
Format: Short |
Label |
|
Order Time: |
|
DateTimePicker |
dtpOrderTime |
|
Format: Time
ShowUpDown: True |
Label |
|
Flavor: |
|
ComboBox |
cboFlavors |
|
DropDownStyle: DropDownList |
Label |
|
Container: |
|
ComboBox |
cboContainers |
|
DropDownStyle: DropDownList |
Label |
|
Ingredient: |
|
ComboBox |
cboIngredients |
|
DropDownStyle: DropDownList |
Label |
|
Scoops: |
|
TextBox |
txtScoops |
1 |
TextAlign: Right |
Label |
|
Order Total: |
|
TextBox |
txtOrderTotal |
0.00 |
TextAlign: Right |
Button |
btnCalcTotal |
Calculate Total |
|
Button |
btnNewOrder |
New Order |
|
Button |
btnClose |
Close |
|
|
- Click the combo box to the right of the Flavor label. Then, in the
Properties, click the ellipsis button
of Items property and create the list with:
Vanilla
Cream of Cocoa
Chocolate Chip
Cherry Coke
Butter Pecan
Chocolate Cookie
Chunky Butter
Organic Strawberry
Chocolate Brownies
Caramel Au Lait |
- Click OK
- Click the combo box to the right of the Container label. Then, in
the Properties, click the ellipsis button
of Items property and create the list with:
- Click OK
- Click the combo box to the right of the Ingredient label. Then, in
the Properties, click the ellipsis button
of Items property and create the list with:
None
Peanuts
Mixed Nuts
M & M
Cookies |
- Click OK
- Double-click the Calculate Total button to access its Click event
- Implement it as follows:
private void btnCalcTotal_Click(object sender, System.EventArgs e)
{
string Container;
decimal PriceContainer = 0.00M,
PriceIngredient = 0.00M,
PriceScoops = 0.00M,
OrderTotal = 0.00M;
int SelectedIngredient = -1,
NumberOfScoops = 1;
// First find out what container the customer requested
Container = this.cboContainers.Text;
// The price of a container depends on which one the customer selected
if( Container == "Cone" )
PriceContainer = 0.55M;
else if( Container == "Cup" )
PriceContainer = 0.75M;
else
PriceContainer = 1.15M;
// Find out if the customer wants any ingredient at all
SelectedIngredient = this.cboIngredients.SelectedIndex;
// If the customer selected an ingredient, which is not "None", add $.95
if( SelectedIngredient > 1 )
PriceIngredient = 0.95M;
try
{
// Get the number of scoops
NumberOfScoops = int.Parse(this.txtScoops.Text);
if( NumberOfScoops == 2 )
PriceScoops = 2.55M;
else if( NumberOfScoops == 3 )
PriceScoops = 3.25M;
else
PriceScoops = 1.85M;
}
catch(FormatException)
{
MessageBox.Show("The value you entered for the scoops is not valid" +
"\nOnly natural numbers such as 1, 2, or 3 are allowed" +
"\nPlease try again");
}
// Make sure the user selected a flavor, otherwise, there is no reason to process an order
if( this.cboFlavors.SelectedIndex > 0 )
OrderTotal = PriceScoops + PriceContainer + PriceIngredient;
this.txtOrderTotal.Text = OrderTotal.ToString("C");
}
|
- Double-click the New Order button to access its Click event and
implement it as follows:
private void btnNewOrder_Click(object sender, System.EventArgs e)
{
// If the user clicks New Order, we reset the form with the default values
this.dtpOrderDate.Value = DateTime.Today;
this.dtpOrderTime.Value = DateTime.Now;
this.cboFlavors.SelectedIndex = -1;
this.cboContainers.SelectedIndex = -1;
this.cboIngredients.SelectedIndex = 0;
this.txtScoops.Text = "1";
this.txtOrderTotal.Text = "0.00";
}
|
- Double-click the Close button and implement its Click event as
follows:
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
|
- Test the application. Here is an example:
 |
OOPS!!! |
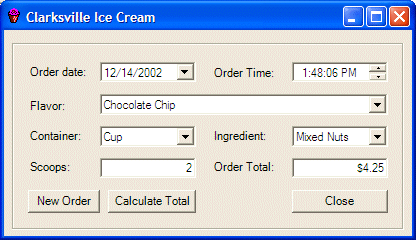 |
- Close the form and return to Visual Studio
One way you can provide simple help consists
of displaying short indicative messages on a status bar. To do this, you
can first create sections, called panels, on a status bar and then display
the necessary messages in the section of your choice. The message can be
anything but it should consist of just a few words to fit in its section
without going over board.
Practical
Learning: Helping Through a Status Bar
|
|
- Display the from and expand its bottom border a little bit
- On the Toolbox, click StatusBar and click the bottom section of the
form
- In the Properties window, set the ShowPanels property to True
- To create the sections of the status bar, click the ellipsis button
of the Panels field
- In the StatusBarPanel Collection Editor, click Add and change the
following values:
Width: 340
Text: Ready
- Click Add and change the following value:
Width: 60
Text: Delete the text
- Click OK

- In the form, click the dtpOrderDate control, that is, the combo box
on the right side of the Order Date label
- In the Properties window, click the Events button
and double-click MouseMove
- Return to the form
- In the same way, initiate the MouseMove event of the dtpOrderTime
date time picker, the cboFlavors combo box, the cboContainers combo
box, the cboIngredients combo box, the txtScoops text box, and the
txtOrderTotal text box
- Implement the events as follows:
private void cboFlavors_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "Select the customer's desired flavor";
}
private void cboContainers_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "Select the type of object that will contain the ice cream";
}
private void cboIngredients_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "Select an ingredient to spice the ice cream";
}
private void txtScoops_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "Select the number of scoops to fill the container";
}
private void txtOrderTotal_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "This displays the total of the order";
}
private void dtpOrderDate_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "Specify the date this order was processed";
}
private void dtpOrderTime_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
{
this.statusBar1.Panels[0].Text = "Specify the time this order was processed";
}
|
- Test the application
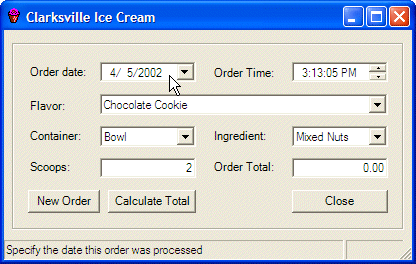
- After using it, close the form and return to Visual Studio
A tool tip is a small yellow box that displays a
word or a group of words when the user positions the mouse on top of a
control:
To create a tool tip system in a Visual Studio
application, first add a ToolTip control to a form. After adding a ToolTip
control, the form and all controls on it receive a new field in
the Properties window. If the new ToolTip control is called ToolTip1, the
new field in the Properties window for each control is ToolTip on
ToolTip1. To display a tool tip for a control, first click it on the form.
Then, in the Properties window, click ToolTip on ToolTip1, and type the
desired tool tip.
Practical
Learning: Adding Tool Tips
|
|
- To prepare for tool tips, on the Toolbox, click ToolTip and click
the form
- On the form, click each control and, in the Properties window, set
its ToolTip On ToolTip1 property as follows:
Control |
ToolTip On ToolTip1 |
dtpOrderDate |
Click the arrow to select a date |
dtpOrderTime |
Click each section, then click one of the arrows
to change its value |
cboFlavors |
Click the arrow to display a list, then select a
flavor from the list |
cboContainers |
Click to display the list of containers and select
one |
cboIngedients |
Display the list of ingredients and make the
customer's choice |
txtScoops |
Enter the number of scoops (1, 2, or 3) to fill
the container |
txtOrderTotal |
This displays the total amount of this order |
btnCalcTotal |
Click here to calculate the total of the order |
btnNewOrder |
Click here to reset the form |
btnClose |
Click here to Close the form |
- Test the application

- Close it and return to Visual Studio
Online help is the system of providing help files with an application. Online
help is usually available from the main menu through a menu group created under
a Help category.
In the past, the techniques to provide or program online help
were not always easy. The early implementations of help were created in a
system called WinHelp. This required using a Rich Text Format (rtf) file and
appropriately formatting it. It is possible that, when folks at Microsoft
developed WinHelp, they had only Microsoft Word in mind. It was difficult to
get the required file ready if you were using another application such as
WordPad or WordPerfect... Creating the help file was not enough. Once the
file was ready, it had to be added to the application, and appropriately. This back and forth
gymnastic was a great motivation for neglect. As if these difficulties were not
enough, or because of these difficulties, Microsoft created another system
called HTML Help. This neither solved most problems nor created an easier
solution. At this time, this HTML Help is widely used and many
companies, such as AutoDesk (AutoCAD) and Macromedia to name just two,
have adopted it . Many companies such as Borland, Jasc, just to name two, are still using
WinHelp. This indicates that HTML Help didn't solve all problems and was not
anonymously adopted.
Because HTML Help is the most supported help system by Microsoft, we will use it. Also, it
is easier to use HTML Help in a Microsoft Visual Studio application than to use
WinHelp.
|
|