 |
Microsoft Visual C#: Data Binding With a Binding Source |
|
|
After specifying the data source, you can use the
binding source because it then holds a list. If you want a singly list-based
control such as a list box, a combo box or a checked list box to use the
list, these controls are equipped with a property named DataSource.
This allows you to apply the list of a binding source directly to the
control. To do this, assign the binding source to the DataSource
property of the control. Here is an example:
|
private void btnBindingSource_Click(object sender, EventArgs e)
{
BindingSource bsNames = new BindingSource();
List<string> strNames = new List<string>();
strNames.Add("Vicky Bisso");
strNames.Add("Amy Warren");
strNames.Add("Chrissie Childs");
strNames.Add("Martial Otto");
bsNames.DataSource = strNames;
lbxNames.DataSource = bsNames;
}
After this assignment, the control can display the list.
The Display Member of a List-Based Control
|
|
In some cases, the list is not single. For example, you
may already know that a data set can contain one or more tables and a table
can contain one or more columns. In this case, you must specify the sub-list
or column that holds the values that you want the control to display. To
support this scenario, the singly list-based controls are equipped with a
property named DisplayMember.
Practical
Learning: Binding Data With Visual Databases Objects
|
|
- Create a new Windows Application named BethesdaCarRental3
- In the Solution Explorer, right-click Form1.cs and click Rename
- Type Central.cs and press Enter
- Double-click the middle of the form and, to create the database
including its tables, implement the event as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace BethesdaCarRental3a
{
public partial class Central : Form
{
public Central()
{
InitializeComponent();
}
void CreateDatabase()
{
using (SqlConnection conBCR =
new SqlConnection("Data Source=(local); " +
"Integrated Security='SSPI';"))
{
string strCreateDatabase = "IF EXISTS ( " +
"SELECT name " +
"FROM sys.databases " +
"WHERE name = N'BethesdaCarRental2' " +
") " +
"DROP DATABASE BethesdaCarRental2; " +
"CREATE DATABASE BethesdaCarRental2";
SqlCommand cmdBCR =
new SqlCommand(strCreateDatabase, conBCR);
conBCR.Open();
cmdBCR.ExecuteNonQuery();
MessageBox.Show("A database named " +
"BethesdaCarRental2 has been created");
}
using (SqlConnection conBCR =
new SqlConnection("Data Source=(local); " +
"Database='BethesdaCarRental2'; " +
"Integrated Security='SSPI';"))
{
string strCreateTable = "CREATE TABLE RentalRates( " +
"RentalRateID int identity(1, 1) NOT NULL, " +
"Category varchar(50), " +
"Daily smallmoney, Weekly smallmoney, " +
"Monthly smallmoney, Weekend smallmoney, " +
"CONSTRAINT PK_RentalRates PRIMARY KEY (RentalRateID));";
SqlCommand cmdBCR = new SqlCommand(strCreateTable, conBCR);
conBCR.Open();
cmdBCR.ExecuteNonQuery();
MessageBox.Show("A table named RentalRates has been " +
"added to the BethesdaCarRental2 database.");
}
using (SqlConnection conBCR =
new SqlConnection("Data Source=(local); " +
"Database='BethesdaCarRental2'; " +
"Integrated Security='SSPI';"))
{
string strCreateTable = "CREATE TABLE Employees( " +
"EmployeeID int identity(1, 1) NOT NULL, " +
"EmployeeNumber nchar(5), " +
"FirstName varchar(32), " +
"LastName varchar(32) NOT NULL, " +
"FullName AS (([LastName]+', ')+[FirstName]), " +
"Title varchar(80), " +
"HourlySalary smallmoney, " +
"CONSTRAINT PK_Employees PRIMARY KEY (EmployeeID));";
SqlCommand cmdBCR = new SqlCommand(strCreateTable, conBCR);
conBCR.Open();
cmdBCR.ExecuteNonQuery();
MessageBox.Show("A table named Employees has been " +
"added to the BethesdaCarRental2 database.");
}
using (SqlConnection conBCR =
new SqlConnection("Data Source=(local); " +
"Database='BethesdaCarRental2'; " +
"Integrated Security='SSPI';"))
{
string strCreateTable = "CREATE TABLE Customers( " +
"CustomerID int identity(1, 1) NOT NULL, " +
"DrvLicNumber varchar(50), " +
"FullName varchar(80), " +
"Address varchar(100) NOT NULL, " +
"City varchar(50), " +
"State varchar(50), " +
"ZIPCode varchar(20), " +
"CONSTRAINT PK_Customer PRIMARY KEY (CustomerID));";
SqlCommand cmdBCR = new SqlCommand(strCreateTable, conBCR);
conBCR.Open();
cmdBCR.ExecuteNonQuery();
MessageBox.Show("A table named Customers has been " +
"added to the BethesdaCarRental2 database.");
}
using (SqlConnection conBCR =
new SqlConnection("Data Source=(local); " +
"Database='BethesdaCarRental2'; " +
"Integrated Security='SSPI';"))
{
string strCreateTable = "CREATE TABLE Cars( " +
"CarID int identity(1, 1) NOT NULL, " +
"TagNumber varchar(20), " +
"Make varchar(50), " +
"Model varchar(50) NOT NULL, " +
"CarYear smallint, " +
"Category varchar(50), " +
"CDPlayer bit, " +
"DVDPlayer bit, " +
"Available bit, " +
"CONSTRAINT PK_Car PRIMARY KEY (CarID));";
SqlCommand cmdBCR =
new SqlCommand(strCreateTable, conBCR);
conBCR.Open();
cmdBCR.ExecuteNonQuery();
MessageBox.Show("A table named Cars has been " +
"added to the BethesdaCarRental2 database.");
}
using (SqlConnection conBCR =
new SqlConnection("Data Source=(local); " +
"Database='BethesdaCarRental2'; " +
"Integrated Security='SSPI';"))
{
string strCreateTable = "CREATE TABLE RentalOrders( " +
"RentalOrderID int identity(1, 1) NOT NULL, " +
"DateProcessed datetime, " +
"EmployeeID int Constraint " +
"FK_Employees References " +
"Employees(EmployeeID) NOT NULL, " +
"EmployeeName varchar(80), " +
"CustomerID int Constraint " +
"FK_Customers References " +
"Customers(CustomerID) NOT NULL, " +
"CustomerName varchar(80), " +
"CustomerAddress varchar(100), " +
"CustomerCity varchar(50), " +
"CustomerState varchar(50), " +
"CustomerZIPCode varchar(20), " +
"CarID int Constraint " +
"FK_Cars References Cars(CarID) NOT NULL, " +
"CarMake varchar(50), " +
"CarModel varchar(50), " +
"CarYear smallint, " +
"CarCondition varchar(50), " +
"TankLevel varchar(40), " +
"MileageStart int, " +
"MileageEnd int, " +
"RentStartDate datetime, " +
"RentEndDate datetime, " +
"Days int, " +
"RateApplied money, " +
"SubTotal money, " +
"TaxRate decimal(6, 2), " +
"TaxAmount money, " +
"OrderTotal money, " +
"OrderStatus varchar(50), " +
"CONSTRAINT PK_RentalOrder " +
"PRIMARY KEY (RentalOrderID));";
SqlCommand cmdBCR = new SqlCommand(strCreateTable, conBCR);
conBCR.Open();
cmdBCR.ExecuteNonQuery();
MessageBox.Show("A table named RentalOrder has been " +
"added to the BethesdaCarRental2 database.");
}
}
private void Central_Load(object sender, EventArgs e)
{
CreateDatabase();
}
}
}
- Execute the application to actually create the database and its
tables
- Close the form and return to your programming environment
- To create a new form, on the main menu, click Project -> Add Windows
Form...
- Set the name to Employees and click Add
- To create a data source, on the main menu, click Data -> Add New
Data Source...
- In the first page of the wizard, make sure Database is selected and
click Next
- In the combo box
- If you see a BethesdaCarRental2, select it
- If you do not have BethesdaCarRental2, click New Connection...
In the Server combo box, select the server or type (local).
In the Select Or Enter A Database Name combo box, select
BethesdaCarRental2. Click Test Connection. Click OK twice. In the
Data Source Configuration Wizard, make sure the new connection is
selected and click Next. Change the Connection String to
csBethesdaCarRental and click Next. Expand the Tables node
and expand the Employees node. Click the check boxes of EmployeeID,
EmployeeNumber, FirstName, LastName, Title, and HourlySalary. Change
the DataSet Name to dsBethesdaCarRental

- Click Finish
- In the Data Sources window, click Employees and click the arrow of
its combo box to select DataGridView (it should be selected already as
the default).
Drag the Employees node and drop it on the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
employeesBindingSource |
bsEmployees |
employeesTableAdapter |
taEmployees |
employeesBindingNavigator |
bnEmployees |
- On the form, click the data grid view control and, in the Properties
window, change its (Name) to dgvEmployees
- Click the ellipsis of the Columns field and make the following
changes:
Selected Columns |
HeaderText |
Width |
EmployeeID |
Empl ID |
50 |
EmployeeNumber |
Empl # |
50 |
FirstName |
First Name |
65 |
LastName |
Last Name |
65 |
Title |
|
120 |
HourlySalary |
Hourly Salary |
75 |
- Click OK
- Set DataGridView's Anchor property to Top, Bottom, Left, Right
- Under the DataGridView control, add a button and set its properties
as follows:
Text: Close (Name): btnClose Anchor:
Bottom, Right
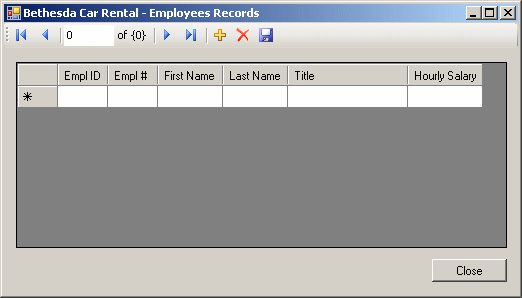
- Double-click the Close button and implement its even as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Access the Central.cs [Design] tab
- Add a button to it and set its properties as follows:
Text:
Employees (Name): btnEmployees
- Double-click the Employees button and implement its even as follows:
private void Central_Load(object sender, EventArgs e)
{
// CreateDatabase();
}
private void btnEmployees_Click(object sender, EventArgs e)
{
Employees clerks = new Employees();
clerks.ShowDialog();
}
- Execute the application to test it
- Click the Employees button and create the following records:
Empl # |
First Name |
Last Name |
Title |
Hourly Salary |
20725 |
Julie |
Flanell |
Regional Manager |
36.55 |
92705 |
Paulette |
Simms |
Sales Manager |
26.65 |
84002 |
Alexandra |
Ulm |
Sales Representative |
12.85 |
47295 |
Ellie |
Tchenko |
Sales Representative |
11.95 |

- Close the forms and return to your programming environment
- To create a new form, on the main menu, click Project -> Add Windows
Form...
- Set the name to Customers and click Add
- In the Data Sources window, right-click dsBethesdaCaRental and click
Configure Dataset with Wizard...
- Expand the Tables node and click the check box of Customers
- Click Finish
- In the Data Sources window, drag Customers and drop it on the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
customersBindingSource |
bsCustomers |
customersTableAdapter |
taCustomers |
customersBindingNavigator |
bnCustomers |
- On the form, click the data grid view control and, in the Properties
window, change its (Name) to dgvCustomers
- Click the ellipsis of the Columns field and make the following
changes:
Selected Columns |
HeaderText |
Width |
CustomerID |
Cust ID |
50 |
DrvLicNumber |
Driver's Lic # |
80 |
FullName |
Full Name |
120 |
Address |
|
120 |
City |
|
120 |
State |
|
40 |
ZIPCode |
ZIP Code |
60 |
- Click OK
- Set DataGridView's Anchor property to Top, Bottom, Left, Right
- Under the DataGridView control, add a button and set its properties
as follows:
Text: Close (Name): btnClose Anchor:
Bottom, Right
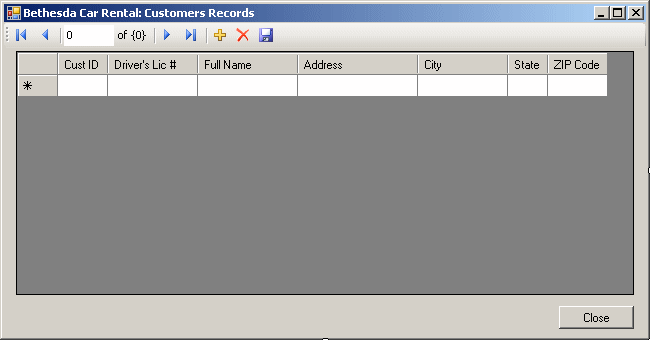
- Double-click the Close button and implement its even as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Access the Central.cs [Design] tab
- Add a button to it and set its properties as follows:
Text:
Customers (Name): btnCustomers
- Double-click the Employees button and implement its even as follows:
private void btnCustomers_Click(object sender, EventArgs e)
{
Customers clients = new Customers();
clients.ShowDialog();
}
- Execute the application to test it
- Click the Customers button and create
Create a few records
- Close the forms and return to your programming environment
- To create a new form, on the main menu, click Project -> Add Windows
Form...
- Set the name to Cars and click Add
- In the Data Sources window, right-click dsBethesdaCaRental and click
Configure Dataset with Wizard...
- Expand the Tables node and click the check box of Cars
- Click Finish
- In the Data Sources window, drag Cars and drop it on the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
carsBindingSource |
bsCars |
carsTableAdapter |
taCars |
carsBindingNavigator |
bnCars |
- On the form, click the data grid view control and, in the Properties
window, change its (Name) to dgvCars
- Click the ellipsis of the Columns field and make the following
changes:
Selected Columns |
HeaderText |
Width |
CarID |
Car ID |
50 |
TagNumber |
Tag # |
70 |
Make |
|
80 |
Model |
|
80 |
CarYear |
Year |
50 |
Category |
|
80 |
CDPlayer |
CD Player |
65 |
DVDPlayer |
DVD Player |
70 |
Available |
|
65 |
- Click OK
- Set DataGridView's Anchor property to Top, Bottom, Left, Right
- Under the DataGridView control, add a button and set its properties
as follows:
Text: Close (Name): btnClose Anchor:
Bottom, Right

- Double-click the Close button and implement its even as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Access the Central.cs [Design] tab
- Add a button to it and set its properties as follows:
Text:
Cars (Name): btnCars
- Double-click the Employees button and implement its even as follows:
private void btnCars_Click(object sender, EventArgs e)
{
Cars vehicles = new Cars();
vehicles.ShowDialog();
}
- Execute the application to test it
- Click the Cars button and create
Create a few records

- Close the forms and return to your programming environment
- To add a new form to the project, on the main menu, click Project ->
Add -> Windows Form...
- Set the Name to RentalRates and press Enter
- In the Data Sources window, right-click dsBethesdaCaRental and click
Configure Dataset with Wizard...
- Expand the Tables node and click the check box of RentalRates
- Click Finish
- In the Data Sources window, drag RentalRates and drop it on the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
rentalRatesBindingSource |
bsRentalRates |
rentalRatesTableAdapter |
taRentalRates |
rentalRatesBindingNavigator |
bnRentalRates |
- On the form, click the data grid view control and, in the Properties
window, change its (Name) to dgvRentalRates
- Click the ellipsis of the Columns field and make the following
changes:
Selected Columns |
HeaderText |
Width |
RentalRateID |
Rate ID |
50 |
Category |
|
75 |
Daily |
|
50 |
Weekly |
|
50 |
Monthly |
|
50 |
Weekend |
|
50 |
- Click OK
- To create a new form, on the main menu, click Project -> Add Windows
Form...
- Set the name to OrderProcessing and click Add
- In the Data Source window, right-click dsBethesdaCarRental and click
Configure DataSet With Wizard...
- Expand the Tables node and click the check box of RentalOrders and
click Finish
- In the Data Sources window, click RentalOrders and click the arrow
of its combo box
- Select Details
- From the Data Sources window, drag RentalOrders and drop it on the
form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
rentalOrdersBindingSource |
bsRentalOrders |
rentalOrdersTableAdapter |
taRentalOrders |
rentalOrdersBindingNavigator |
bnRentalOrders |
- Again, from the Data Sources window, drag Employees and drop it on
the form
- While the DataGridView control is still selected on the form, press
Delete to remove it from the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
employeesBindingSource |
bsEmployees |
employeesTableAdapter |
taEmployees |
- From the Data Sources window, drag Customers and drop it in an empty
area of the form
- While the DataGridView control is still selected on the form, press
Delete to remove it from the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
customersBindingSource |
bsCustomers |
customersTableAdapter |
taCustomers |
- Once again, from the Data Sources window, drag Cars and drop it on
the form
- While the DataGridView control is still selected on the form, press
Delete to remove it from the form
- Under the form, click the objects and, using the Properties window,
change their names as follows:
Object |
Name |
carsBindingSource |
bsCars |
carsTableAdapter |
taCars |
- On the form, delete the text boxes on the right side of EmployeeID,
CustomerID, CarID, CarCondition, TankLevel, and OrderStatus
- Design the form as follows (you will add new controls to replace
those that were deleted):
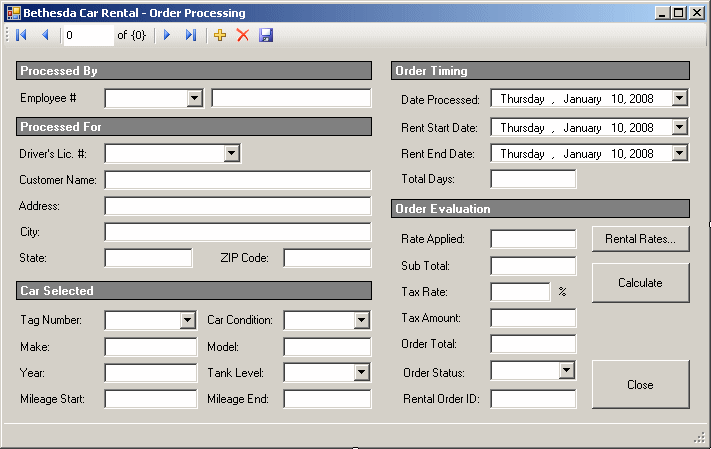 |
Control |
Old Name |
Text |
Name |
Additional Properties |
Label |
New |
Processed By: |
|
BackColor: Gray BorderStyle:
FixedSingle |
Label |
Old |
Employee #: |
|
|
ComboBox |
New |
|
cboEmployeeID |
|
TextBox |
employeeNameTextBox |
|
txtEmployeeName |
|
Label |
|
Processing For |
|
BackColor: Gray BorderStyle:
FixedSingle |
Label |
Old |
Driver's Lic. #: |
|
|
ComboBox |
New |
|
cbxCustomerID |
|
Label |
Old |
Customer Name: |
|
|
TextBox |
customerNameTextBox |
|
txtCustomerName |
|
Label |
Old |
Address: |
|
|
TextBox |
customerAddressTextBox |
|
txtCustomerAddress |
|
Label |
Old |
City: |
|
|
TextBox |
customerCityTextBox |
|
txtCustomerCity |
|
Label |
Old |
State: |
|
|
TextBox |
customerStateTextBox |
|
txtCustomerState |
|
Label |
Old |
ZIP Code: |
|
|
TextBox |
customerZIPCodeTextBox |
|
txtCustomerZIPCode |
|
Label |
New |
Car Selected |
|
BackColor: Gray BorderStyle:
FixedSingle |
Label |
Old |
Tag Number: |
|
|
ComboBox |
New |
|
cbxCarID |
|
Label |
Old |
Car Condition: |
|
|
ComboBox |
New |
|
cbxCarConditions |
Items: Needs Repair Drivable
Excellent |
Label |
Old |
Make: |
|
|
TextBox |
carMakeTextBox |
|
txtCarMake |
|
Label |
|
Model: |
|
|
TextBox |
carModelTextBox |
|
txtCarModel |
|
Label |
Old |
Year: |
|
|
TextBox |
Old |
|
txtCarYear |
|
Label |
Old |
Tank Level: |
|
|
ComboBox |
New |
|
cbxTankLevels |
Items: Empty 1/4 Empty 1/2 Full
3/4 Full Full |
Label |
Old |
Mileage Start: |
|
|
TextBox |
mileageStartTextBox |
|
txtMileageStart |
TextAlign: Right |
Label |
|
Mileage End: |
|
|
TextBox |
mileageEndTextBox |
|
txtMileageEnd |
TextAlign: Right |
Label |
|
Order Timing |
|
BackColor: Gray BorderStyle:
FixedSingle |
Label |
Old |
Date Processed |
|
|
DateTimePicker |
dateProcessedDateTimePicker |
|
dtpDateProcessed |
|
Label |
Old |
Rent Start Date: |
|
|
DateTimePicker |
rentStartDateDateTimePicker |
|
dtpRentStartDate |
|
Label |
|
Rent End Date: |
|
|
DateTimePicker |
rentEndDateDateTimePicker |
|
dtpRentEndDate |
|
Label |
Old |
Total Days: |
|
|
TextBox |
daysTextBox |
|
txtTotalDays |
|
Label |
|
Order Evaluation |
|
BackColor: Gray BorderStyle:
FixedSingle |
Label |
Old |
Rate Applied |
|
|
TextBox |
rateAppliedTextBox |
|
txtRateApplied |
TextAlign: Right |
Button |
New |
Rental Rates |
btnRentalRates |
|
Label |
Old |
Sub Total: |
|
|
TextBox |
subTotalTextBox |
|
txtSubTotal |
TextAlign: Right |
Button |
New |
Calculate |
btnCalculate |
|
Label |
Old |
Tax Rate: |
|
|
TextBox |
taxRateTextBox |
|
txtTaxRate |
TextAlign: Right |
Label |
|
% |
|
|
Label |
|
Tax Amount: |
|
|
TextBox |
taxAmountTextBox |
|
txtTaxAmount |
TextAlign: Right |
Label |
|
Order Total: |
|
|
TextBox |
orderTotalTextBox |
|
txtOrderTotal |
TextAlign: Right |
Label |
Old |
Order Status: |
|
|
ComboBox |
New |
|
cbxOrderStatus |
Items: Car On Road Car Returned
Order Reserved |
Label |
Old |
Rental Order ID: |
|
|
TextBox |
rentalOrderIDTextBox |
|
txtRentalOrderID |
TextAlign: Right |
Button |
|
Close |
btnClose |
|
StatusStrip |
|
|
|
|
|
- Click each control that was added and
change
its data bindings
- On the form, double-click the Employee # combo box and implement its
Click event as follows:
private void cbxEmployeeID_SelectedIndexChanged(object sender, EventArgs e)
{
using (System.Data.SqlClient.SqlConnection conEmployees =
new System.Data.SqlClient.SqlConnection("Data Source=(local);" +
"Database=BethesdaCarRental2;" +
"Integrated Security=yes"))
{
string strEmployee = "SELECT FullName FROM Employees " +
"WHERE EmployeeNumber = '" +
cbxEmployeeID.Text + "';";
System.Data.SqlClient.SqlCommand cmdEmployees =
new System.Data.SqlClient.SqlCommand(strEmployee, conEmployees);
conEmployees.Open();
System.Data.SqlClient.SqlDataReader rdrEmployees =
cmdEmployees.ExecuteReader();
while (rdrEmployees.Read())
txtEmployeeName.Text = rdrEmployees.GetString(0);
}
}
- Return to the OrderProcessing form
- On the form, double-click the Driver's Lic # combo box and implement
its Click event as follows:
private void cbxCustomerID_SelectedIndexChanged(object sender, EventArgs e)
{
using (System.Data.SqlClient.SqlConnection conCustomers =
new System.Data.SqlClient.SqlConnection("Data Source=(local);" +
"Database=BethesdaCarRental2;" +
"Integrated Security=yes"))
{
string strCustomer = "SELECT * FROM Customers " +
"WHERE DrvLicNumber = '" +
cbxCustomerID.Text + "';";
System.Data.SqlClient.SqlCommand cmdCustomers =
new System.Data.SqlClient.SqlCommand(strCustomer, conCustomers);
conCustomers.Open();
System.Data.SqlClient.SqlDataReader rdrCustomers =
cmdCustomers.ExecuteReader();
while (rdrCustomers.Read())
{
txtCustomerName.Text = rdrCustomers.GetString(2);
txtCustomerAddress.Text = rdrCustomers.GetString(3);
txtCustomerCity.Text = rdrCustomers.GetString(4);
txtCustomerState.Text = rdrCustomers.GetString(5);
txtCustomerZIPCode.Text = rdrCustomers.GetString(6);
}
}
}
- Return to the OrderProcessing form
- On the form, double-click the Tag Number combo box and implement its
Click event as follows:
private void cbxCarID_SelectedIndexChanged(object sender, EventArgs e)
{
using (System.Data.SqlClient.SqlConnection conCars =
new System.Data.SqlClient.SqlConnection("Data Source=(local);" +
"Database=BethesdaCarRental2;" +
"Integrated Security=yes"))
{
string strCar = "SELECT * FROM Cars " +
"WHERE TagNumber = '" +
cbxCarID.Text + "';";
System.Data.SqlClient.SqlCommand cmdCars =
new System.Data.SqlClient.SqlCommand(strCar, conCars);
conCars.Open();
System.Data.SqlClient.SqlDataReader rdrCars =
cmdCars.ExecuteReader();
while (rdrCars.Read())
{
txtCarMake.Text = rdrCars.GetString(2);
txtCarModel.Text = rdrCars.GetString(3);
txtCarYear.Text = rdrCars.GetSqlInt16(4).ToString();
}
}
}
- Return to the OrderProcessing form
- On the form, double-click the Rent End Date control and implement
the event as follows:
private void dtpRentEndDate_ValueChanged(object sender, EventArgs e)
{
if( dtpRentStartDate.Value > dtpRentEndDate.Value )
txtTotalDays.Text = "0";
else if (dtpRentStartDate.Value == dtpRendependDate.Value)
txtTotalDays.Text = "1";
else
{
int days;
DateTime dteStart = dtpRentStartDate.Value;
DateTime dteEnd = dtpRentEndDate.Value;
// Let's calculate the difference in days
TimeSpan tme = dteEnd - dteStart;
days = tme.Days;
// If the customer returns the car the same day,
// we consider that the car was rented for 1 day
if (days == 0)
days = 1;
txtTotalDays.Text = days.ToString();
// At any case, we will let the clerk specify the actual number of days
}
}
- Return to the OrderProcessing form and double-click the Rental Rates
button
- Implement the event as follows:
private void btnRentalRates_Click(object sender, EventArgs e)
{
RentalRates rates = new RentalRates();
rates.ShowDialog();
}
- Return to the OrderProcessing form and doouble-click the Calculate
button
- Implement the events as follows:
private void btnCalculate_Click(object sender, EventArgs e)
{
int Days = 0;
double RateApplied = 0.00;
double TaxRate = 0.00;
double SubTotal, TaxAmount, OrderTotal;
try
{
Days = int.Parse(txtTotalDays.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid number of days.");
}
try
{
RateApplied = double.Parse(txtRateApplied.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid rate applied.");
}
try
{
TaxRate = double.Parse(txtTaxRate.Text);
}
catch (FormatException)
{
MessageBox.Show("Invalid tax rate.");
}
SubTotal = Days * RateApplied;
TaxAmount = SubTotal * TaxRate / 100;
OrderTotal = SubTotal + TaxAmount;
txtSubTotal.Text = SubTotal.ToString("F");
txtTaxAmount.Text = TaxAmount.ToString("F");
txtOrderTotal.Text = OrderTotal.ToString("F");
}
- Return to OrderProcessing the form and double-click the Close button
- Implement its even as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Access the Central form
- Add a button to it and set its properties as follows:
Text:
OrderProcessing (Name): btnOrderProcessing
- Double-click the OrderProcessing button and implement the
even as follows:
private void btnOrderProcessing_Click(object sender, EventArgs e)
{
OrderProcessing frmOrder = new OrderProcessing();
frmOrder.ShowDialog();
}
- Return to the Central form and double-click the Close button
- Implement the event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Execute the application and display the OrderProcessing form
- To create an order, click the + button, complete the form, and click
the Save button. Here are examples:
- Close the forms and return to your programming environment
- Execute the application again and display the Order Processing form
again
- Click the Rental Rates button and create the values as follows:
Category |
Daily |
Weekly |
Monthly |
Weekend |
Economy |
35.95 |
32.75 |
28.95 |
24.95 |
Compact |
39.95 |
35.75 |
32.95 |
28.95 |
Standard |
45.95 |
39.75 |
35.95 |
32.95 |
Full Size |
49.95 |
42.75 |
38.95 |
35.95 |
Mini Van |
55.95 |
50.75 |
45.95 |
42.95 |
SUV |
55.95 |
50.75 |
45.95 |
42.95 |
Truck |
42.75 |
38.75 |
35.95 |
32.95 |
Van |
69.95 |
62.75 |
55.95 |
52.95 |
- Update the orders you previously processed and save them
- Close the forms and return to your programming environment
|
|