 |
Microsoft Visual C# Example: The z Score |
|
|
The z score, also called a standardized value, is a
value a member of a (numeric) collection is above or below the mean.
Obviously before calculating it, you must have a series of values. You
must also calculate the mean of all those values. You must also have the
standard deviation.
|
The formula to calculate the z score of an element of
a sample is:

The factors in this equation are:
Factor |
Description |
x |
An element of the series |
x |
The mean of the sample |
s |
The standard deviation of the sample |
|
|
The formula to calculate the z score of an element of
a population is:

The factors in this equation are:
Factor |
Description |
x |
An element of the series |
µ |
The mean of the population |
σ |
The standard deviation of the population |
Application:
Starting the Application
|
|
- Start Microsoft Visual Studio
- To create a new application, on the main menu, click File -> New
Project...
- In the middle list, click Windows Forms Application
- Change the Name to ZScore1
- Click OK
- In the Solution Explorer, right-click Form1.cs and click Rename
- Type Exercise.cs and press Enter
- Design the form as follows:
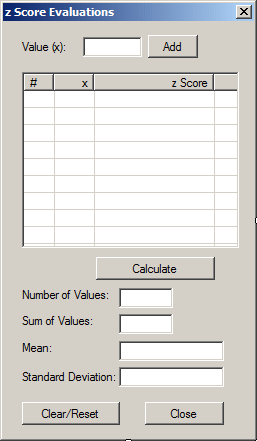 |
Control |
Name |
Text |
Label |
 |
|
Value (x): |
TextBox |
 |
txtValue |
|
Button |
 |
btnAdd |
Add |
ListView |
 |
lvwValues |
FullRowSelect: True GridLines: True View:
Details |
Columns |
|
(Name) |
Text |
TextAlign |
Width |
colIndex |
# |
|
30 |
colX |
x |
|
40 |
colZScore |
z Score |
Right |
120 |
|
Button |
 |
btnCalculate |
Calculate |
Label |
 |
|
Number of Values: |
TextBox |
 |
txtCount |
|
Label |
 |
|
Sum of Values: |
TextBox |
 |
txtSum |
|
Label |
 |
|
Mean: |
TextBox |
 |
txtMean |
|
Label |
 |
|
Standard Deviation |
TextBox |
 |
txtStandardDeviation |
|
Button |
 |
btnClear |
Clear/Reset |
Button |
 |
btnClose |
Close |
|
- Double-click an unoccupied area of the form
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ZScore1
{
public partial class Exercise : Form
{
List<double> values;
public Exercise()
{
InitializeComponent();
}
private void Exercise_Load(object sender, EventArgs e)
{
values = new List<double>();
}
void ShowValues()
{
int i = 0;
lvwValues.Items.Clear();
foreach (double value in values)
{
ListViewItem lviValue = new ListViewItem(i.ToString());
lviValue.SubItems.Add(value.ToString());
lvwValues.Items.Add(lviValue);
}
}
}
}
- Return to the form and double-click the Add button
- Implement the event as follows:
private void btnAdd_Click(object sender, EventArgs e)
{
double x = 0.00D;
double sum = 0.00D, mean = 0.00D;
double bigSum = 0.00, stdDev;
// Check that the user entered a value for x
if (txtValue.Text.Length == 0)
{
MessageBox.Show("You must enter the x value.",
"Statistics");
return;
}
// Get the value for x
try
{
x = double.Parse(txtValue.Text);
}
catch (FormatException)
{
MessageBox.Show("The value you entered is invalid.",
"Statistics");
}
// Add the value to the list
values.Add(x);
ShowValues();
// Calculate the sum of the values
foreach (double value in values)
sum += value;
mean = sum / values.Count;
// Calculate the total for the standard deviation
for (int i = 0; i < values.Count; i++)
bigSum += Math.Pow(values[i] - mean, 2);
// Now we can calculate the standard deviation
stdDev = Math.Sqrt(bigSum / (values.Count - 1));
txtCount.Text = values.Count.ToString();
txtSum.Text = sum.ToString();
txtMean.Text = mean.ToString();
txtStandardDeviation.Text = stdDev.ToString();
txtValue.Text = "";
txtValue.Focus();
}
- Return to the form
- Double-click the Calculate button
- Implement the event as follows:
private void btnCalculate_Click(object sender, EventArgs e)
{
double sum = 0.00, mean = 0.00;
double bigSum = 0.00, stdDev;
lvwValues.Items.Clear();
// Example Values: To use these values, remove comment
/*
values.Add(70.8); values.Add(66.2); values.Add(71.7);
values.Add(68.7); values.Add(67.6); values.Add(69.2);
values.Add(66.5); values.Add(67.2); values.Add(68.3);
values.Add(65.6); values.Add(63); values.Add(68.3);
values.Add(73.1); values.Add(67.6); values.Add(68);
values.Add(71); values.Add(61.3); values.Add(76.2);
values.Add(66.3); values.Add(69.7); values.Add(65.4);
values.Add(70); values.Add(62.9); values.Add(68.5);
values.Add(68.3); values.Add(69.4); values.Add(69.2);
values.Add(68); values.Add(71.9); values.Add(66.1);
values.Add(72.4); values.Add(73); values.Add(68);
values.Add(68.7); values.Add(70.3); values.Add(63.7);
values.Add(71.1); values.Add(65.6); values.Add(68.3);
values.Add(66.3); */
foreach (double value in values)
sum += value;
mean = sum / values.Count;
// Calculate the total for the standard deviation
for (int i = 0; i < values.Count; i++)
bigSum += Math.Pow(values[i] - mean, 2);
// Now we can calculate the standard deviation
stdDev = Math.Sqrt(bigSum / (values.Count - 1));
int j = 1;
foreach (double value in values)
{
ListViewItem lviValue = new ListViewItem(j.ToString());
lviValue.SubItems.Add(value.ToString());
lviValue.SubItems.Add(((value - mean) / stdDev).ToString());
lvwValues.Items.Add(lviValue);
j++;
}
txtCount.Text = values.Count.ToString();
txtSum.Text = sum.ToString();
txtMean.Text = mean.ToString();
txtStandardDeviation.Text = stdDev.ToString();
}
- Return to the form
- Double-click the Clear/Reset button
- Implement the event as follows:
private void btnClear_Click(object sender, EventArgs e)
{
txtValue.Text = "";
lvwValues.Items.Clear();
txtCount.Text = "";
txtSum.Text = "";
txtMean.Text = "";
txtStandardDeviation.Text = "";
txtValue.Focus();
}
- Return to the form
- Double-click the Close button
- Implement the event as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- To execute, press F5
- Enter values and click Add each time
- After adding the values, click Calculate
- Close the form and return to your programming environment
Application
|
|