 |
Example Application: The HTML Body Tag Formatter |
|
|
A scroll bar is used to navigate a bar using a thumb.
While navigating, you can get the current value of the control. This
application explores the properties of scroll bars.
|
Application:
Creating the Application
|
|
- Start a new Windows Application named HTMLBodyTag1
- Design the form as follows:
 |
Control |
Name |
Text |
Other Properties |
GroupBox |
 |
|
Body Attributes |
|
RadioButton |
 |
rdoBackground |
Background |
Checked: True |
TextBox |
 |
txtBackground |
#000000 |
|
RadioButton |
 |
rdoText |
Text |
|
TextBox |
 |
txtText |
#0000FF |
|
RadioButton |
 |
rdoLink |
Link |
|
TextBox |
 |
txtLink |
#FF0000 |
|
RadioButton |
 |
rdoActiveLink |
Active Link |
|
TextBox |
 |
txtActiveLink |
#008000 |
|
RadioButton |
 |
rdoVisitedLink |
Visited Link |
|
TextBox |
 |
txtVisitedLink |
#800000 |
|
Panel |
 |
pnlPreview |
|
BackColor: White BorderStyle: Fixed3D |
VScrollBar |
 |
scrRed |
|
|
VScrollBar |
 |
scrGreen |
|
|
VScrollBar |
 |
scrBlue |
|
|
Label |
 |
|
R |
|
Label |
 |
|
G |
|
Label |
 |
|
B |
|
Panel |
 |
pnlBody |
|
BackColor: White BorderStyle: Fixed3D |
TextBox |
 |
txtTextPreview |
Body tag formatter and colorizer |
BorderStyle: None ForeColor: Blue
TextAlign: Center |
TextBox |
 |
txtLinkPreview |
Sample text as link |
BorderStyle: None ForeColor: Red
TextAlign: Center |
TextBox |
 |
txtALinkPreview |
Current active link |
BorderStyle: None ForeColor: Green
TextAlign: Center |
TextBox |
 |
txtVLinkPreview |
This link has been visited |
BorderStyle: None ForeColor: Maroon
TextAlign: Center |
GroupBox |
 |
|
Color's Values |
|
Label |
 |
|
Red: |
|
TextBox |
 |
txtRed |
0 |
|
Label |
 |
|
Green: |
|
TextBox |
 |
txtGreen |
0 |
|
Label |
 |
|
Blue: |
|
TextBox |
 |
txtBlue |
0 |
|
Button |
 |
btnCopy |
Copy |
|
Button |
 |
btnClose |
Close |
|
TextBox |
 |
txtResult |
<body bgcolor="#FFFFFF"
text="#0000FF" link="#FF0000" alink="#008000"
vlink="#800000"> |
|
- On the form, click one of the scroll bars
- Press and hold Ctrl
- Click the other two scroll bars and release Ctrl
- In the Properties window, click Maximum, type 255, and press
Enter
- Click an unoccupied area of the form to make sure no control is
selected
- On the form, click one of the scroll bars
- Press and hold Ctrl
- Click the other two scroll bars and release Ctrl
- In the Properties window, click LargeChange, type 1, and
press Enter
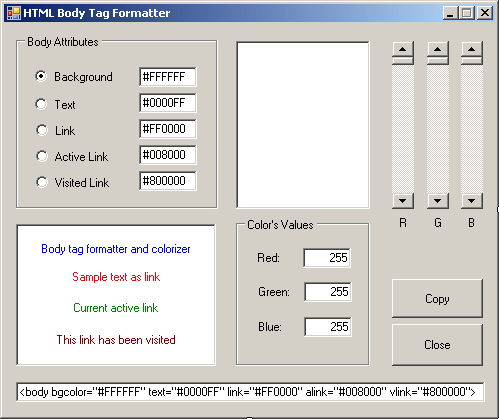
- Right-click the form and click View Code
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace HTMLBodyTag1
{
public partial class Form1 : Form
{
private string HexBG, HexText, HexLink, HexALink, HexVLink;
public Form1()
{
InitializeComponent();
}
internal void ApplyColor()
{
// Retrieve the current hexadecimal colors from their Edit controls
HexBG = txtBackground.Text;
HexText = txtText.Text;
HexLink = txtLink.Text;
HexALink = txtActiveLink.Text;
HexVLink = txtVisitedLink.Text;
// Get the integral position of each ScrollBar control
string strRed = Convert.ToString(255 - scrRed.Value);
string strGreen = Convert.ToString(255 - scrGreen.Value);
string strBlue = Convert.ToString(255 - scrBlue.Value);
// Display the position of each ScrollBar
// in its corresponding Edit control
txtRed.Text = strRed;
txtGreen.Text = strGreen;
txtBlue.Text = strBlue;
// Change the color of the Preview panel
// according to the values of the ScrollBar positions
pnlPreview.BackColor = Color.FromArgb(255 - scrRed.Value,
255 - scrGreen.Value,
255 - scrBlue.Value);
string FmtRed = (255 - scrRed.Value).ToString("X");
if (FmtRed.Length == 1)
FmtRed = String.Concat("0", FmtRed);
string FmtGreen = (255 - scrGreen.Value).ToString("X");
if (FmtGreen.Length == 1)
FmtGreen = String.Concat("0", FmtGreen);
string FmtBlue = (255 - scrBlue.Value).ToString("X");
if (FmtBlue.Length == 1)
FmtBlue = String.Concat("0", FmtBlue);
// Get the position of each ScrollBar control
// Create a hexadecimal color starting with #
// And display the color in the appropriate Edit control
if (rdoBackground.Checked == true)
{
string BG = "#";
BG = String.Concat(BG, FmtRed);
BG = String.Concat(BG, FmtGreen);
BG = String.Concat(BG, FmtBlue);
txtBackground.Text = BG;
pnlBody.BackColor = pnlPreview.BackColor;
txtTextPreview.BackColor = pnlPreview.BackColor;
txtLinkPreview.BackColor = pnlPreview.BackColor;
txtALinkPreview.BackColor = pnlPreview.BackColor;
txtVLinkPreview.BackColor = pnlPreview.BackColor;
HexBG = txtBackground.Text;
}
else if (rdoText.Checked == true)
{
string Txt = "#";
Txt = String.Concat(Txt, FmtRed);
Txt = String.Concat(Txt, FmtGreen);
Txt = String.Concat(Txt, FmtBlue);
txtText.Text = Txt;
txtTextPreview.ForeColor = Color.FromArgb(255 - scrRed.Value,
255 - scrGreen.Value,
255 - scrBlue.Value);
HexText = txtText.Text;
}
else if (rdoLink.Checked == true)
{
string TL = "#";
TL = String.Concat(TL, FmtRed);
TL = String.Concat(TL, FmtGreen);
TL = String.Concat(TL, FmtBlue);
txtLink.Text = TL;
txtLinkPreview.ForeColor = Color.FromArgb(255 - scrRed.Value,
255 - scrGreen.Value,
255 - scrBlue.Value);
HexLink = txtLink.Text;
}
else if (rdoActiveLink.Checked == true)
{
string AL = "#";
AL = String.Concat(AL, FmtRed);
AL = String.Concat(AL, FmtGreen);
AL = String.Concat(AL, FmtBlue);
txtActiveLink.Text = AL;
txtALinkPreview.ForeColor = Color.FromArgb(255 - scrRed.Value,
255 - scrGreen.Value,
255 - scrBlue.Value);
HexALink = txtActiveLink.Text;
}
else if (rdoVisitedLink.Checked == true)
{
string VL = "#";
VL = String.Concat(VL, FmtRed);
VL = String.Concat(VL, FmtGreen);
VL = String.Concat(VL, FmtBlue);
txtVisitedLink.Text = VL;
txtVLinkPreview.ForeColor = Color.FromArgb(255 - scrRed.Value,
255 - scrGreen.Value,
255 - scrBlue.Value);
HexVLink = txtVisitedLink.Text;
}
// Update the contents of the bottom text box
string BD = "<body bgcolor=\"";
BD = String.Concat(BD, HexBG);
BD = String.Concat(BD, "\" text=\"");
BD = String.Concat(BD, HexText);
BD = String.Concat(BD, "\" link=\"");
BD = String.Concat(BD, HexLink);
BD = String.Concat(BD, "\" alink=\"");
BD = String.Concat(BD, HexALink);
BD = String.Concat(BD, "\" vlink=\"");
BD = String.Concat(BD, HexVLink);
BD = String.Concat(BD, "\">");
txtResult.Text = BD;
}
}
}
- Return to the form and double-click the left scroll bar
- Implement its event as follows:
private void scrRed_Scroll(object sender, ScrollEventArgs e)
{
ApplyColor();
}
- Return to the form and double-click the middle scroll bar
- Implement its event as follows:
private void scrGreen_Scroll(object sender, ScrollEventArgs e)
{
ApplyColor();
}
- Return to the form and double-click the right scroll bar
- Implement its event as follows:
private void scrBlue_Scroll(object sender, ScrollEventArgs e)
{
ApplyColor();
}
- Execute the application to test the form
- Close the form and return to your programming environment
- When the user clicks a radio button from the Body Attributes group
box, we need to display its color on the Preview panel. When a
particular button is clicked, we will retrieve the color of its font
from the Body text box, translate that color into red, green, and blue
values, and then use those values to automatically update the scroll
bars and the edit boxes. While we are at it, we also need to update the
corresponding text box in the Body Attributes group box. Since this
functionality will be used by all radio buttons in the group, we will
use a global function to which we can pass two variables.
When the
user clicks a particular radio button, that button is represented by a
text box in the lower-left Body section. We need to get the color of
that edit box and pass it to our function. Since the clicked radio
button has a corresponding text box in the Body Attributes group box, we
need to change/update that value with the hexadecimal value of the first
argument. Therefore, we will pass a string argument to our function. In the Code Editor, just after the closing curly bracket of the
scrBlue_Scroll event, create the following method:
internal void ClickOption(System.Drawing.Color Clr, String Result)
{
// These variables will hold the red, green, and blue
// values of the passed color
int red, green, blue;
// Colorize the Preview panel with the passed color
pnlPreview.BackColor = Clr;
// Get the red value of the color of the Preview panel
red = 255 - pnlPreview.BackColor.R;
// Get the green value of the color of the Preview panel
green = 255 - pnlPreview.BackColor.G;
// Get the blue value of the color of the Preview panel
blue = 255 - pnlPreview.BackColor.B;
// Now that we have the red, green, and blue values of the color,
// Update the scroll bars with the new values
scrRed.Value = red;
scrGreen.Value = green;
scrBlue.Value = blue;
// Update the red, green, and blue values
// of the Numeric Values group box
txtRed.Text = red.ToString();
txtGreen.Text = green.ToString();
txtBlue.Text = blue.ToString();
// Update the string that was passed using
// the retrieved red, green, and blue values
Result = String.Concat(Result, "#");
Result = String.Concat(Result, red.ToString("X"));
Result = String.Concat(Result, green.ToString("X"));
Result = String.Concat(Result, blue.ToString("X"));
}
- Return to the form
- Double-click the Background radio button and implement its event as
follows:
private void rdoBackground_CheckedChanged(object sender, EventArgs e)
{
// If the user clicks Background radio button
// set color of the panel to that of the radio button
Color BGColor = pnlBody.BackColor;
pnlBody.BackColor = BGColor;
// Call the ClickOption() method to calculate
// the hexadecimal value of the color
ClickOption(pnlBody.BackColor, txtBackground.Text);
HexBG = txtBackground.Text;
}
- Return to the form
- Double-click the Text radio button and implement its event as
follows:
private void rdoText_CheckedChanged(object sender, EventArgs e)
{
Color BGColor = pnlBody.BackColor;
txtTextPreview.BackColor = BGColor;
ClickOption(txtTextPreview.ForeColor, txtText.Text);
HexText = txtText.Text;
}
- Return to the form
- Double-click the Link radio button and implement its event as
follows:
private void rdoLink_CheckedChanged(object sender, EventArgs e)
{
Color BGColor = pnlBody.BackColor;
txtLinkPreview.BackColor = BGColor;
ClickOption(txtLinkPreview.ForeColor, txtLink.Text);
HexLink = txtLink.Text;
}
- Return to the form
- Double-click the Active Link radio button and implement its event as
follows:
private void rdoActiveLink_CheckedChanged(object sender, EventArgs e)
{
Color BGColor = pnlBody.BackColor;
txtALinkPreview.BackColor = BGColor;
ClickOption(txtALinkPreview.ForeColor, txtActiveLink.Text);
HexALink = txtActiveLink.Text;
}
- Return to the form
- Double-click the Visited Link radio button and implement its event
as follows:
private void rdoVisitedLink_CheckedChanged(object sender, EventArgs e)
{
Color BGColor = pnlBody.BackColor;
txtVLinkPreview.BackColor = BGColor;
ClickOption(txtVLinkPreview.ForeColor, txtVisitedLink.Text);
HexVLink = txtVisitedLink.Text;
}
- Return to the form
- Double-click the Copy button and implement it as follows:
private void btnCopy_Click(object sender, EventArgs e)
{
txtResult.SelectAll();
txtResult.Copy();
}
- Return to the form
- Double-click the Close button and implement it as follows:
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
- Execute the application

- Close the form
|
|