 |
Windows Controls: The Label |
|
|
A label is a control that serves as a guide to the user.
It provides static text that the user cannot change but can read to get
information on a form. The programmer can also use it to display simple
information to the user. Most controls on the form are not explicit at first
glance and the user may not know what they are used for. Therefore, you can
assign a label to a control as a help to the user.
|
To add a label to a container, click the Label button
from the Toolbox and click the object that would host it.
To programmatically create a label, declare a handle to
Label, initialize it using its default constructor, and add it to the
Controls property of the form. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : System.Windows.Forms.Form
{
Label lblMessage;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblMessage = new Label();
Controls.Add(lblMessage);
}
}
public class Program
{
static int Main()
{
System.Windows.Forms.Application.Run(new Exercise());
return 0;
}
}
Application:
Introducing Labels
|
|
- Start Microsoft Visual Studio and create a Windows Application named
ElementaryAddition1
- From the Common Control section of the Toolbox, click Label and
click the form
- From the Common Control section of the Toolbox, click the Label
again and click the form
- From the Common Control section of the Toolbox, click the Label
again and click the form
Characteristics of a Label
|
|
The most important characteristic of a label control is
the text it displays. That text is also referred to as its caption and this
is what the user would read. The text of a label is its Text property
and is its default. To set a label’s caption, after adding the
control to a container, click Text in the Properties window and type
the desired value. As we mentioned when studying controls characteristics,
at design time, the text you type in the Text field is considered
“as isâ€. If you want to create a more elaborate and formatted
string, you would have to do it programmatically. Here is an example:
public class Exercise : System.Windows.Forms.Form
{
Label lblMessage;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblMessage = new Label();
lblMessage.Text = DateTime.Now.ToString();
Controls.Add(lblMessage);
}
}
Here is an example of what this would produce:

When it comes to its caption, one of the most valuable
characteristics of the text of a label is the variance of the font. When
designing a caption. you can change the default font to make it more
attractive.
Application:
Captioning the Labels
|
|
- On the form, click the first label
- In the Properties window, click Text and type 00
- Click (Name) and type lblOperand1
- Click TextAlign and the arrow of its combo box to select Center
- Click the + button of the Font field and change the characteristics
as follows:
Name: Tahoma Size: 48 Bold: True
- On the form, click the second label and, in the Properties window,
change its characteristics as follows:
Text: + TextAlign:
Center (Name): lblOPeration Font -> Name: Arial Font ->
Size: 50 Font -> Bold: True
- On the form, click the third label and, in the Properties window,
change its characteristics as follows:
Text: 00 TextAlign:
Center (Name): lblOperand2 Font -> Name: Arial Font ->
Size: 50 Font -> Bold: True
Automatically Sizing a Label
|
|
After adding a label to a form, by default, it receives
a fixed size. If you type its caption and press Enter, the text you provided
would be confined to the allocated dimensions of the control. If the text is
too long, part of it may disappear. You can then resize the label to provide
more area. Another solution is to automatically resize the label to
accommodate the length of the string you typed. This is aspects is
controlled by the Boolean AutoSize property. Its default value is
False. If you set this property to True, at design time, a
rectangular border appears around it. If you type a string in the Text
field and press Enter, the control would be resized to show the whole string
but using only the necessary space.
If you programmatically create a label, it assumes a
default size. If you assign it a string that is too long for that default
size, part of the string may appear on a subsequent line. If you want the
whole string to appear on the same line, you can set the AutoSize to
true. Here is an example:
public class Exercise : System.Windows.Forms.Form
{
Label lblMessage;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
lblMessage = new Label();
lblMessage.Text = DateTime.Now.ToString();
lblMessage.AutoSize = true;
Controls.Add(lblMessage);
}
}
Here is an example of what this would produce:

Application:
Using AutoSize
|
|
- Click an unoccupied area of the form and press Ctrl + A to select
all three labels
- In the Properties window, double-click AutoSize to set the value to
False
- Save all
After typing the caption of a label whose AutoSize
property is set to False, you can resize its allocated space to your liking.
This is because a string occupies a rectangular area. Here is an example:
By default, the caption of a
label is positioned starting on the middle-left side of its allocated
rectangle. Alternatively, you can position it to one of the nine available
positions. The position of the caption of a label is controlled by the TextAlign
property which is based on the ContentAlignment enumerator:
It can have the following values:
TopLeft |
TopCenter |
TopRight |
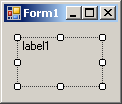 |
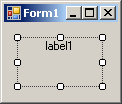 |
 |
MiddleLeft |
MiddleCenter |
MiddleRight |
 |
 |
 |
BottomLeft |
BottomCenter |
BottomRight |
 |
 |
 |
Other fancy characteristics you can
apply to a label include its font and color. For example, a label is
primarily meant to display a string. To make it fancier, you can display a
(small) picture next to it. To do this, at design time, use the Image
field of the Properties window to select a picture. You can also specify the
picture at run time by assigning an Image value to the Label.Image
property. After adding a picture that would accompany the label, you can
specify what position the picture would hold with regards to the label. To
do this, select the desired position of the ImageAlign field in the
Properties window.
Instead of using a picture from the Image
property, you can create a list of images using an ImageList control and
assign it to the label. In fact, the advantage of an ImageList is
that, unlike the Image property, it allows you to use more than one
picture.
After assigning an ImageList to the label control
using the Properties window or code, you can use the ImageIndex to
specify what picture to display next to the label.
A label provides another property called UseMnemonic.
This property is valuable only when the label accompanies another control.
Application:
Using a Text Box
|
|
- Complete the design of the form as follows:
 |
Control |
Text |
Name |
TextAlign |
Font |
Additional Properties |
Label |
 |
00 |
lblOperand1 |
Center |
Name: Tahoma Size: 48 Bold: True |
ForeColor: Blue |
Label |
 |
+ |
|
Center |
Name: Arial Size: 50 Bold: True |
ForeColor: Maroon |
Label |
 |
00 |
lblOperand2 |
Center |
Name: Tahoma Size: 48 Bold: True |
ForeColor: Blue |
Label |
 |
= |
|
Center |
Name: Arial Size: 50 Bold: True |
ForeColor: Green |
Label |
 |
00 |
lblResult |
Center |
Name: Tahoma Size: 48 Bold: True |
|
Label |
 |
New Operation |
lblNewOperation |
Center |
Name: Tahoma Size: 28 Bold: True |
BorderStyle: Fixed3D ForeColor: White
BackColor:Maroon |
Label |
 |
Quit |
lblQuit |
Center |
Name: Tahoma Size: 28 Bold: True |
BorderStyle: FixedSingle |
|
- To be able to use the Visual Basic library, in the Solution
Explorer, right-click References -> Add References...
- In the .NET property page, click Microsoft.VisualBasic

- Click OK and OK
- Double-click New Operation and implement its event as follows:
private void lblNewOperation_Click(object sender, EventArgs e)
{
int operand1;
int operand2;
int answer = 0;
Random rnd = new Random();
operand1 = rnd.Next(99);
operand2 = rnd.Next(99);
int result = operand1 + operand2;
lblOperand1.Text = operand1.ToString();
lblOperand2.Text = operand2.ToString();
lblResult.Text = "0";
string strPrompt = lblOperand1.Text + " + " +
lblOperand2.Text + " =";
try
{
answer =
int.Parse(Microsoft.VisualBasic.Interaction.InputBox(
strPrompt,
"Elementary Addition",
"000",
100,
100));
lblResult.Text = answer.ToString();
if (answer == result)
MessageBox.Show("WOW - Good Answer");
else
MessageBox.Show("PSSST - Wrong Answer");
}
catch (FormatException)
{
}
}
- Return to the form and double-click Quit
- Implement its event as follows:
private void lblQuit_Click(object sender, EventArgs e)
{
Close();
}
- Execute the application and test it
- Click the Close button to close the form and return to your
programming environment
|
|