 |
GDI+ Examples: Collision Detection I |
|
|
Objects are said to collide when they touch each
other. This is something very important in graphical applications and
games, etc. It used to be particularly difficult but nowadays, it mostly
depends on how you want to implement. In this exercise, we will draw to
circles that keep moving on a form. If the meet (collide), they stop.
|
This example uses shapes of the Microsoft Visual Basic Power
Packs.
Application:
Introducing the Tree View Control
|
|
- Start Microsoft Visual Studio or Microsoft Visual C# Express
- To create an application, on the main menu, click File -> New Project...
- Set the project type to Empty Project
- Change the name to CollisionDetection1
- Click OK
- On the main menu, click Project -> CollisionDetection1 Properties...
- In the Output Type combo box, select Windows Application
- On the main menu, click Project -> Add Reference...
- Click the .NET tab
- In the list, click System
- Press and hold Ctrl
- Click System.Drawing
- Click System.Windows.Forms
- Click Microsoft.VisualBasic.PowerPacks.Vs
- Click OK
- On the main menu, click Project -> Add New Item...
- Click Code File
- Change the name to CollisionDetection
- Click Add
- In the empt document, type the following:
using System;
using System.Drawing;
using System.Windows.Forms;
public class CollisionDetection : Form
{
static int xGreen;
static int xBrown;
static int yGreen;
static int yBrown;
static bool IsMovingDownGreen;
static bool IsMovingDownBrown;
static bool IsMovingRightGreen;
static bool IsMovingRightBrown;
Timer tmrMover;
Microsoft.VisualBasic.PowerPacks.OvalShape osGreen;
Microsoft.VisualBasic.PowerPacks.OvalShape osBrown;
Microsoft.VisualBasic.PowerPacks.ShapeContainer shpContainer;
public CollisionDetection()
{
InitializeComponent();
}
private void InitializeComponent()
{
xGreen = 0;
xBrown = 250;
yGreen = 0;
yBrown = 500;
IsMovingDownGreen = false;
IsMovingDownBrown = false;
IsMovingRightGreen = false;
IsMovingRightBrown = false;
tmrMover = new Timer();
tmrMover.Interval = 2;
tmrMover.Enabled = true;
tmrMover.Tick += new EventHandler(tmrMoverTicked);
osBrown = new Microsoft.VisualBasic.PowerPacks.OvalShape();
osBrown.BackColor = Color.FromArgb(255, 128, 0);
osBrown.BackStyle = Microsoft.VisualBasic.PowerPacks.BackStyle.Opaque;
osBrown.BorderColor = Color.FromArgb(128, 64, 0);
osBrown.BorderWidth = 2;
osBrown.Size = new System.Drawing.Size(80, 80);
osGreen = new Microsoft.VisualBasic.PowerPacks.OvalShape();
osGreen.BackColor = Color.FromArgb(192, 255, 192);
osGreen.BackStyle = Microsoft.VisualBasic.PowerPacks.BackStyle.Opaque;
osGreen.BorderColor = Color.Cyan;
osGreen.BorderWidth = 2;
osGreen.Location = new Point(100, 100);
osGreen.Size = new Size(80, 80);
shpContainer = new Microsoft.VisualBasic.PowerPacks.ShapeContainer();
shpContainer.Location = new System.Drawing.Point(0, 0);
shpContainer.Margin = new System.Windows.Forms.Padding(0);
shpContainer.Shapes.Add(osBrown);
shpContainer.Shapes.Add(osGreen);
shpContainer.Size = new System.Drawing.Size(778, 576);
BackColor = System.Drawing.Color.Black;
ClientSize = new System.Drawing.Size(778, 576);
Controls.Add(shpContainer);
StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.DoubleBuffer, true);
}
private void tmrMoverTicked(object sender, EventArgs e)
{
// If the status of the origin indicates that it is moving right,
// then increase the horizontal axis
if (IsMovingRightGreen == true)
xGreen++;
else // If it's moving left, then decrease the horizontal movement
xGreen--;
// If the status of the origin indicates that it is moving down,
// then increase the vertical axis
if (IsMovingDownGreen == true)
yGreen++;
else // Otherwise, decrease it
yGreen--;
// Collision: if the axis hits the right side of the screen,
// then set the horizontal moving status to "Right", which will be used
// by the above code
if ((xGreen + osGreen.Width) >= ClientSize.Width)
IsMovingRightGreen = false;
if ((xGreen + osGreen.Width) <= 40)
IsMovingRightGreen = true; ;
if ((yGreen + osGreen.Height) > ClientSize.Height)
IsMovingDownGreen = false;
if ((yGreen + osGreen.Height) <= 40)
IsMovingDownGreen = true;
BackColor = Color.Black;
osGreen.Location = new Point(xGreen, yGreen);
// -----------------------------------------------------------
if (IsMovingRightBrown == true)
xBrown++;
else // If it's moving left, then decrease the horizontal movement
xBrown--;
// If the status of the origin indicates that it is moving down,
// then increase the vertical axis
if (IsMovingDownBrown == true)
yBrown++;
else // Otherwise, decrease it
yBrown--;
// Collision: if the axis hits the right side of the screen,
// then set the horizontal moving status to "Right", which will be used
// by the above code
if ((xBrown + osBrown.Width) >= ClientSize.Width)
IsMovingRightBrown = false;
if ((xBrown + osBrown.Width) <= 40)
IsMovingRightBrown = true; ;
if ((yBrown + osBrown.Height) > ClientSize.Height)
IsMovingDownBrown = false;
if ((yBrown + osBrown.Height) <= 40)
IsMovingDownBrown = true;
osBrown.Location = new Point(xBrown, yBrown);
if (osBrown.Bounds.IntersectsWith(osGreen.Bounds))
{
tmrMover.Enabled = false;
/*
* Random rndNumber = new Random();
xGreen = rndNumber.Next(Width);
xBrown = rndNumber.Next(Width);
yGreen = rndNumber.Next(Height);
yBrown = rndNumber.Next(Height);
*/
}
}
}
public class Exercise
{
public static int Main()
{
Application.Run(new CollisionDetection());
return 0;
}
}
- To execute, press F5
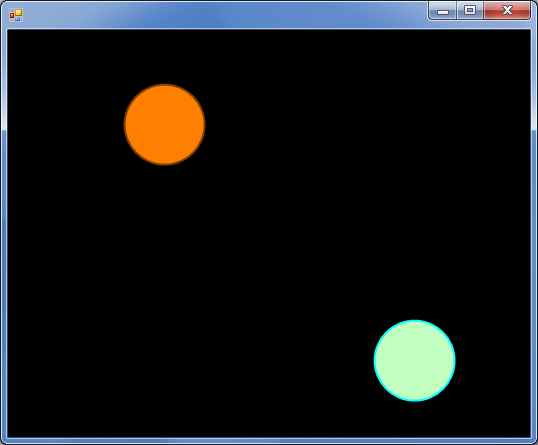
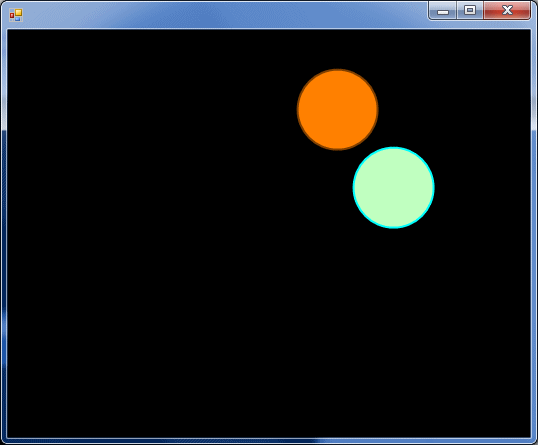
- Close the form
|
|