 |
GDI+ Shapes: Ellipses and Circles |
|
|
An ellipse is a closed continuous line whose points are
positioned so that two points exactly opposite each other have the exact
same distant from a central point. It can be illustrated as follows:
|

Because an ellipse can fit in a rectangle, in GDI+
programming, an ellipse is defined with regards to a rectangle it would fit
in. To draw an ellipse, you can use the Graphics.DrawEllipse() method
that comes in four versions whose syntaxes are:
public void DrawEllipse(Pen pen,
Rectangle rect);
public void DrawEllipse(Pen pen,
RectangleF rect);
public void DrawEllipse(Pen pen,
int x,
int y,
int width,
int height);
public void DrawEllipse(Pen pen,
float x,
float y,
float width,
float height);
The arguments of this method play the same roll as those
of the Graphics.DrawRectangle() method:
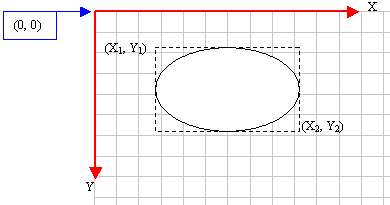
Here is an example:
private void Exercise_Paint(object sender, PaintEventArgs e)
{
Pen penCurrent = new Pen(Color.Red);
e.Graphics.DrawEllipse(penCurrent, new Rectangle(20, 20, 226, 144));
}

Application:
Drawing a Circle
|
|
- Start a new Windows Application named RotatingCircles1
- Double-click middle of the form and change the file as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace RotatingCircles1
{
public partial class Form1 : Form
{
Graphics graphDrawingArea;
Bitmap bmpDrawingArea;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
bmpDrawingArea = new Bitmap(Width, Height);
graphDrawingArea = Graphics.FromImage(bmpDrawingArea);
}
}
}
- From the Components section of the Toolbox, click Timer and click
the form
- In the Properties window, change the following values:
Enabled: True Interval: 20
- Under the form, double-click the timer1 object and make the
following changes:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace RotatingCircles1
{
public partial class Form1 : Form
{
Graphics graphDrawingArea;
Bitmap bmpDrawingArea;
static int mainRadius = 10;
static int smallRadius = 5;
static bool isMax;
static bool smallRadiusMax;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
bmpDrawingArea = new Bitmap(Width, Height);
graphDrawingArea = Graphics.FromImage(bmpDrawingArea);
}
void DrawCentralCircle(int CenterX, int CenterY, int Radius)
{
int start = CenterX - Radius;
int end = CenterY - Radius;
int diam = Radius * 2;
graphDrawingArea.DrawEllipse(new Pen(Color.Blue),
start, end, diam, diam);
}
void DrawCornerCircle(int CenterX, int CenterY, int Radius)
{
int start = CenterX - Radius;
int end = CenterY - Radius;
int diam = Radius * 2;
graphDrawingArea.DrawEllipse(new Pen(Color.Red),
start, end, diam, diam);
}
private void timer1_Tick(object sender, EventArgs e)
{
Graphics graph = Graphics.FromHwnd(this.Handle);
int centerX = ClientRectangle.Width / 2;
int centerY = ClientRectangle.Height / 2;
if (isMax == true)
mainRadius--;
else
mainRadius++;
if (mainRadius > (ClientRectangle.Height / 2))
isMax = true;
if (mainRadius < 10)
isMax = false;
if (smallRadiusMax == true)
smallRadius--;
else
smallRadius++;
if (smallRadius > 240)
smallRadiusMax = true;
if (smallRadius < 5)
smallRadiusMax = false;
// Central
DrawCentralCircle(centerX, centerY, mainRadius);
// Top-Left
DrawCornerCircle(centerX / 2, centerY / 2, smallRadius);
// Top-Right
DrawCornerCircle(centerX + (centerX / 2), centerY / 2, smallRadius);
// Bottom-Left
DrawCornerCircle(centerX / 2, centerY + (centerY / 2), smallRadius);
// BottomRight
DrawCornerCircle(centerX + (centerX / 2),
centerY + (centerY / 2), smallRadius);
graph.DrawImage(bmpDrawingArea, 0, 0);
}
}
}
- Execute the application to see the result
- Close the form
|
|