 |
GDI+ Shapes: Rectangles and Squares |
|
|
A rectangle is a geometric figure made of four sides
that compose four right angles. To draw a rectangle, you can either specify
the Rectangle value that encloses it, or you can define its location and its
dimensions. To draw a rectangle that is enclosed in a Rectangle
value, you can use the following version of the Graphics.DrawRectangle()
method:
|
public void DrawRectangle(Pen pen, Rectangle rect);
Remember that such a rectangle object can be illustrated
as follows:
After defining a Rectangle variable, you can pass it to
the method. Here is an example:
using System;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : Form
{
public Exercise()
{
InitializeComponent();
}
void InitializeComponent()
{
Paint += new PaintEventHandler(Exercise_Paint);
}
private void Exercise_Paint(object sender, PaintEventArgs e)
{
Pen penCurrent = new Pen(Color.Red);
Rectangle Rect = new Rectangle(20, 20, 248, 162);
e.Graphics.DrawRectangle(penCurrent, Rect);
}
}
public class Program
{
public static int Main()
{
Application.Run(new Exercise());
return 0;
}
}
Remember that you can also define a Pen and/or a
Rectangle objects in the parentheses of the method:
private void Exercise_Paint(object sender, PaintEventArgs e)
{
e.Graphics.DrawRectangle(new Pen(Color.Red),
new Rectangle(20, 20, 248, 162));
}
This would produce:
It is (very) important to remember that the third
argument of the Rectangle represents its width (and not its right)
value and the fourth argument represents its height (and not its bottom)
value. This is a confusing fact for those who have programmed in GDI: GDI+
defines a Rectangle differently than GDI. In fact, to determine the
location and dimensions of a rectangle to draw, the Graphics class
provides the following versions of the DrawRectangle() method:
public
void DrawRectangle(Pen pen,
int x,
int y,
int width,
int height);
void DrawRectangle(Pen pen,
float x,
float y,
float width,
float height);
This time, the rectangle is represented by its location
with a point at (x, y) and its dimensions with the width
and height argument. This can be illustrated in a Windows coordinate
system as follows:
Based on this, the earlier rectangle can also be drawn
with the following:
private void Exercise_Paint(object sender, PaintEventArgs e)
{
e.Graphics.DrawRectangle(new Pen(Color.Red), 20, 20, 248, 162);
}
A square is a rectangle whose four sides are equal.
Application:
Drawing a Rectangle
|
|
- Start Microsoft Visual C# and create a new Windows Application named
WeeklySales1
- Design the form as follows:
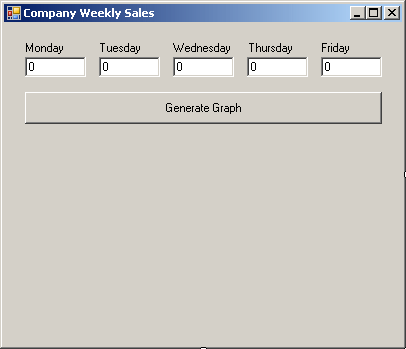 |
Control |
Name |
Text |
Label |
 |
|
Monday |
Label |
 |
|
Tuesday |
Label |
 |
|
Wednesday |
Label |
 |
|
Thursday |
Label |
 |
|
Friday |
TextBox |
 |
txtMonday |
0 |
TextBox |
 |
txtTuesday |
0 |
TextBox |
 |
txtWednesday |
0 |
TextBox |
 |
txtThursday |
0 |
TextBox |
 |
txtFriday |
0 |
Button |
 |
Generate |
btnGenerate |
|
- Double-click an unoccupied area of the form and change the file as
follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WeeklySales1
{
public partial class Form1 : Form
{
private Graphics graphDrawingArea;
private Bitmap bmpDrawingArea;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
bmpDrawingArea = new Bitmap(Width, Height);
graphDrawingArea = Graphics.FromImage(bmpDrawingArea);
}
}
}
- Return to the form and click an empty area on it. In the Properties
window, click the Events button

- Double-click the Paint field and implement its event as follows:
private void Form1_Paint(object sender, PaintEventArgs e)
{
e.Graphics.DrawImage(bmpDrawingArea, 0, 0);
}
- Return to the form and double-click the Generate button
- Implement the event as follows:
private void btnGenerate_Click(object sender, EventArgs e)
{
int monday= 0,
tuesday = 0,
wednesday = 0,
thursday = 0,
friday = 0;
try
{
monday = int.Parse(txtMonday.Text) / 100;
}
catch (FormatException)
{
MessageBox.Show("Invalid sales on Monday");
txtMonday.Text = "0";
}
try
{
tuesday = int.Parse(txtTuesday.Text) / 100;
}
catch (FormatException)
{
MessageBox.Show("Invalid sales on Tuesday");
txtTuesday.Text = "0";
}
try
{
wednesday = int.Parse(txtWednesday.Text) / 100;
}
catch (FormatException)
{
MessageBox.Show("Invalid sales on Wednesday");
txtWednesday.Text = "0";
}
try
{
thursday = int.Parse(txtThursday.Text) / 100;
}
catch (FormatException)
{
MessageBox.Show("Invalid sales on Thursday");
txtThursday.Text = "0";
}
try
{
friday = int.Parse(txtFriday.Text) / 100;
}
catch (FormatException)
{
MessageBox.Show("Invalid sales on Friday");
txtFriday.Text = "0";
}
graphDrawingArea.Clear(this.BackColor);
graphDrawingArea.DrawRectangle(new Pen(Color.Red),
this.txtMonday.Left + 10,
300 - monday,
40, monday);
graphDrawingArea.DrawRectangle(new Pen(Color.Blue),
this.txtTuesday.Left + 10,
300 - tuesday,
40, tuesday);
graphDrawingArea.DrawRectangle(new Pen(Color.Fuchsia),
this.txtWednesday.Left + 5,
300 - wednesday,
40, wednesday);
graphDrawingArea.DrawRectangle(new Pen(Color.Brown),
this.txtThursday.Left + 5,
300 - thursday,
40, thursday);
graphDrawingArea.DrawRectangle(new Pen(Color.Turquoise),
this.txtFriday.Left + 5,
300 - friday, 40, friday);
graphDrawingArea.DrawRectangle(new Pen(Color.Black),
10, 300, Width - 30, 1);
Invalidate();
}
- Execute the application and test the form
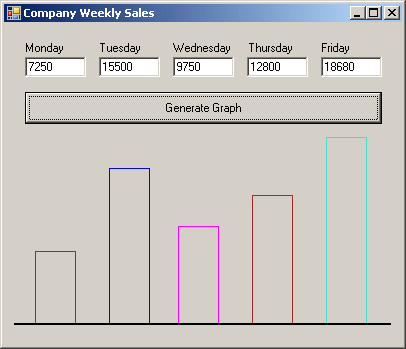
- After using it, close the form
The DrawRectangle() method is used to draw one
rectangle. If you plan to draw many rectangles, you can proceed in one step
by using the Graphics.DrawRectangles() method. It comes in two
versions whose syntaxes are:
public void DrawRectangles(Pen pen, Rectangle[] rects);
public void DrawRectangles(Pen pen, RectangleF[] rects);
This method requires an array of Rectangle or
RectangleF values. When executed, it draws individual rectangles using
each member of the array as its own rectangle. Here is an example:
private void Exercise_Paint(object sender, PaintEventArgs e)
{
Pen penCurrent = new Pen(Color.Red);
Rectangle[] Rect = { new Rectangle(20, 20, 120, 20),
new Rectangle(20, 50, 120, 30),
new Rectangle(20, 90, 120, 40),
new Rectangle(20, 140, 120, 60) };
e.Graphics.DrawRectangles(penCurrent, Rect);
}
This would produce:
|
|