When you declare a variable of a class, a special
method must be called to initialize the members of that class. This method
is automatically provided for every class and it is called a constructor.
Whenever you create a new class, a constructor is automatically provided
to it. This particular constructor is called the default
constructor. You have the option of creating it or not. Although a constructor is created for your class, you can
customize its behavior or change it as you see fit.
The constructor of a class is called New and it is
created as a sub procedure. Here is an example:
Public Class Square
Public Sub New()
End Sub
End Class
Like every method, a constructor is equipped with
a body. In this body, you can access any of the member variables (or
method(s)) of the same class. Consider the following program:
Public Module Exercise
Private Class Square
Public Sub New()
MsgBox("Square Builder")
End Sub
End Class
Public Function Main() As Integer
Dim sq As Square = New Square
Return 0
End Function
End Module
When executed, it would produce:

This shows that, when a class has been instantiated,
its constructor is the first method to be called. For this reason, you can
use a constructor to initialize a class, that is, to assign default values
to its member variables. Based on this, instead of initializing the member
variable(s) of a class when initializing it or them, or instead of
creating a special method used to initialize the member variable(s) of a
class, you can use a constructor to do this. The advantage of a
constructor is that it doesn't need to be called: it is automatically
available whenever the class is instantiated.
Practical
Learning: Constructing a Class
|
|
- Create a Console Application named
DepartmentStore4
- In the Solution Explorer, right-click Module1.vb and click Rename
- Set the name to DepartmentStore.vb, press Enter, and accept to
rename the file
- To create a new class, in the Solution Explorer, right-click
DepartmentStore4 -> Add -> Class...
- Set the Name to StoreItem and click Add
- Change the file as follows:
Public Enum ItemCategory
Women
Men
Girls
Boys
Babies
Jewelry
Beauty
Bedroom
Miscellaneous
End Enum
Public Class StoreItem
Friend ItemNumber As Long
Friend Category As ItemCategory
Friend ItemName As String
Friend UnitPrice As Double
Public Sub New()
Me.ItemNumber = 0
Me.Category = ItemCategory.Miscellaneous
Me.ItemName = "Unknown"
Me.UnitPrice = 0D
End Sub
Public Sub ShowItem()
Dim StrCategory As String
Select Case Category
Case ItemCategory.Women
StrCategory = "Women"
Case ItemCategory.Men
StrCategory = "Men"
Case ItemCategory.Girls
StrCategory = "Girls"
Case ItemCategory.Boys
StrCategory = "Boys"
Case ItemCategory.Babies
StrCategory = "Babies"
Case ItemCategory.Jewelry
StrCategory = "Jewelry"
Case ItemCategory.Beauty
StrCategory = "Beauty"
Case ItemCategory.Bedroom
StrCategory = "Bedroom"
Case Else
StrCategory = "Miscellaneous"
End Select
MsgBox("=-= Department Store =-=" & vbCrLf & _
"--- Store Inventory ---" & vbCrLf & _
"Item #:" & vbTab & vbTab & ItemNumber & vbCrLf & _
"Category:" & vbTab & StrCategory & vbCrLf & _
"Item Name:" & vbTab & ItemName & vbCrLf & _
"Unit Price:" & vbTab & FormatCurrency(UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
End Sub
End Class
|
- Access the DepartmentStore.vb file
- To use a StoreItem object, change the file as follows:
Public Module DepartmentStore
Public Function Main() As Integer
Dim Item As StoreItem
Item = New StoreItem
Item.ShowItem()
Return 0
End Function
End Module
|
- Execute the application to see the result
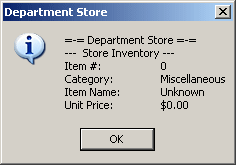
- Close the form and return to your programming environment
A Constructor With Argument(s) |
|
In the previous section, we saw that there was always a
default constructor for a new class that you create; you just havce the option of
explicitly creating one or not. The default constructor as we saw it doesn't
take arguments: this is not a rule, it is simply assumed. Instead of a default
constructor, you may want to create a constructor that takes an argument. Here
is an example:
Private Class Square
Public Sub New(ByVal sd As Double)
End Sub
End Class
With this type of constructor, when you declare an instance
of the class, you can use this new constructor to initialize the class. Here is
an example:
Public Module Exercise
Private Class Square
Public Sub New(ByVal sd As Double)
MsgBox("Square Builder")
End Sub
End Class
Public Function Main() As Integer
Dim sqr As Square = New Square(38.64)
Return 0
End Function
End Module
If you create one constructor for your class and pass at
least one argument to that constructor, the automatic default constructor
created by the compiler disappears. This implies that if you declare an instance
of the class and use the default constructor to initialize it, you would receive
an error when you compile the program. Based on this, the following program will
produce an error:
Public Module Exercise
Private Class Square
Public Sub New(ByVal sd As Double)
MsgBox("Square Builder")
End Sub
End Class
Public Function Main() As Integer
Dim sqr As Square = New Square ' The default constructor is not available
Return 0
End Function
End Module
If you still want to use the default constructor in a class
after creating a constructor that takes at least one argument, you must
explicitly create that default constructor.
Practical
Learning: Constructing a Class
|
|
- To pass arguments to a constructor, change the file as follows:
Public Enum ItemCategory
Women
Men
Girls
Boys
Babies
Jewelry
Beauty
Bedroom
Miscellaneous
End Enum
Public Class StoreItem
Friend ItemNumber As Long
Friend Category As ItemCategory
Friend ItemName As String
Friend UnitPrice As Double
Public Sub New(ByVal Number As Long, _
ByVal Type As ItemCategory, _
ByVal Name As String, _
ByVal Price As Double)
ItemNumber = Number
Category = Type
ItemName = Name
UnitPrice = Price
End Sub
Public Sub ShowItem()
Dim StrCategory As String
Select Case Category
Case ItemCategory.Women
StrCategory = "Women"
Case ItemCategory.Men
StrCategory = "Men"
Case ItemCategory.Girls
StrCategory = "Girls"
Case ItemCategory.Boys
StrCategory = "Boys"
Case ItemCategory.Babies
StrCategory = "Babies"
Case ItemCategory.Jewelry
StrCategory = "Jewelry"
Case ItemCategory.Beauty
StrCategory = "Beauty"
Case ItemCategory.Bedroom
StrCategory = "Bedroom"
Case Else
StrCategory = "Miscellaneous"
End Select
MsgBox("=-= Department Store =-=" & vbCrLf & _
"--- Store Inventory ---" & vbCrLf & _
"Item #:" & vbTab & vbTab & ItemNumber & vbCrLf & _
"Category:" & vbTab & StrCategory & vbCrLf & _
"Item Name:" & vbTab & ItemName & vbCrLf & _
"Unit Price:" & vbTab & FormatCurrency(UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
End Sub
End Class
|
- Access the DepartmentStore.vb file and change it as follows:
Public Module DepartmentStore
Public Function Main() As Integer
Dim Nbr As Long
Dim Classification As ItemCategory
Dim Description As String
Dim Value As Double
Dim Item As StoreItem
Nbr = 759470
Classification = ItemCategory.Men
Description = "Multistriped Organic Cotton Dress Shirt"
Value = 35.5
Item = New StoreItem(Nbr, Classification, Description, Value)
Item.ShowItem()
Return 0
End Function
End Module
|
- Execute the application to see the result
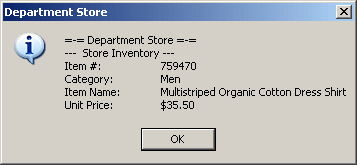
- Close the form and return to your programming environment
A constructor is the primary method of a class. It allows
the programmer to initialize a variable of a class when the class is
instantiated. A constructor that plays this role of initializing an instance of
a class is also called an instance constructor. Most of the time, you don't
need to create a constructor, since one is automatically provided to any class
you create. Sometimes though, as we have seen in some classes, you need to create
your own constructor as you judge it necessary and sometimes, a single constructor
may not be sufficient. For example, when creating a class, you may decide, or
find out, that there must be more than one way for a user to initialize a
variable.
Like any other method, a constructor can be overloaded. In
other words, you can create a class and give it more than one constructor. The
same rules used on overloading regular methods also apply to constructors: the
different constructors must have different number of arguments or different
types of arguments.
Practical
Learning: Overloading a Constructor
|
|
- Access the StoreItem.vb file
- To overload the constructor, change the file as follows:
Public Enum ItemCategory
Women
Men
Girls
Boys
Babies
Jewelry
Beauty
Bedroom
Miscellaneous
End Enum
Public Class StoreItem
Friend ItemNumber As Long
Friend Category As ItemCategory
Friend ItemName As String
Friend ItemSize As String
Friend UnitPrice As Double
REM Default Constructor, used to initialize an unidentified object
Public Sub New()
Me.ItemNumber = 0
Me.Category = ItemCategory.Miscellaneous
Me.ItemName = "Unknown"
Me.ItemSize = "Unknown or Fits All"
Me.UnitPrice = 0D
End Sub
REM This constructor is for items without size (jewelry, beauty, etc)
Public Sub New(ByVal Number As Long, _
ByVal Type As ItemCategory, _
ByVal Name As String, _
ByVal Price As Double)
Me.ItemNumber = Number
Me.Category = Type
Me.ItemName = Name
Me.UnitPrice = Price
End Sub
REM This constructor is used to identify an item to wear
Public Sub New(ByVal Number As Long, _
ByVal Type As ItemCategory, _
ByVal Name As String, _
ByVal Size As String, _
ByVal Price As Double)
Me.ItemNumber = Number
Me.Category = Type
Me.ItemName = Name
Me.ItemSize = Size
Me.UnitPrice = Price
End Sub
REM This method is used to display a description of an item
REM The caller will determine whether the item has a size or not
Public Sub ShowItem(ByVal HasSize As Boolean)
Dim StrCategory As String
Select Case Category
Case ItemCategory.Women
StrCategory = "Women"
Case ItemCategory.Men
StrCategory = "Men"
Case ItemCategory.Girls
StrCategory = "Girls"
Case ItemCategory.Boys
StrCategory = "Boys"
Case ItemCategory.Babies
StrCategory = "Babies"
Case ItemCategory.Jewelry
StrCategory = "Jewelry"
Case ItemCategory.Beauty
StrCategory = "Beauty"
Case ItemCategory.Bedroom
StrCategory = "Bedroom"
Case Else
StrCategory = "Miscellaneous"
End Select
If HasSize = True Then
MsgBox("=-= Department Store =-=" & vbCrLf & _
"--- Store Inventory ---" & vbCrLf & _
"Item #:" & vbTab & vbTab & ItemNumber & vbCrLf & _
"Category:" & vbTab & StrCategory & vbCrLf & _
"Item Name:" & vbTab & ItemName & vbCrLf & _
"Size:" & vbTab & vbTab & ItemSize & vbCrLf & _
"Unit Price:" & vbTab & FormatCurrency(UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
Else
MsgBox("=-= Department Store =-=" & vbCrLf & _
"--- Store Inventory ---" & vbCrLf & _
"Item #:" & vbTab & vbTab & ItemNumber & vbCrLf & _
"Category:" & vbTab & StrCategory & vbCrLf & _
"Item Name:" & vbTab & ItemName & vbCrLf & _
"Unit Price:" & vbTab & FormatCurrency(UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
End If
End Sub
End Class
|
- Access the DepartmentStore.vb file and change it as follow:
Public Module DepartmentStore
Public Function Main() As Integer
Dim Nbr As Long
Dim Classification As ItemCategory
Dim Description As String
Dim Size As String
Dim Value As Double
Dim Item As StoreItem
REM Using the default constructor
Item = New StoreItem
Item.ShowItem(True)
Nbr = 608432
Classification = ItemCategory.Jewelry
Description = "Silver 1/10-ct. T.W. Diamond Heart-Link Bracelet"
Value = 95.85
REM Using the constructor that takes 4 arguments
Item = New StoreItem(Nbr, Classification, Description, Value)
Item.ShowItem(False)
Nbr = 437876
Classification = ItemCategory.Women
Description = "Crinkled Georgette Dress"
Size = "Medium"
Value = 42.95
REM Using the constructor that takes 5 arguments
REM after initialing a Women object
Item = New StoreItem(Nbr, ItemCategory.Women, _
Description, Size, Value)
Item.ShowItem(True)
REM Using the constructor that takes 5 arguments
REM Directly initialing the constructor
Item = New StoreItem(790475, ItemCategory.Men, _
"New Wool Comfort Pants", "36x33", 38.75)
Item.ShowItem(True)
REM Using the constructor that takes 5 arguments
REM Initialing the values in random order
Item = New StoreItem(Type:=ItemCategory.Women, _
Size:="9 1/2 W", _
Price:=35.5, _
Name:="Modest Dress Heels", _
Number:=487046)
Item.ShowItem(True)
Return 0
End Function
End Module
|
- Execute the application to see the result:
- Close the form and return to your programming environment
When you initialize a variable using the New operator, you
are in fact asking the compiler to provide you some memory space in the heap
memory. The compiler is said to "allocate" memory for your variable.
When that variable is no longer needed, for example when your program closes, it
(the variable) must be removed from memory and the space it was using can be
made available to other variables or other programs. This is referred to as
garbage collection.
The .NET Framework solves the problem of garbage collection
by letting the compiler "clean" memory after you. This is done
automatically when the compiler judges it necessary so that the programmer
doesn't need to worry about this issue.
When you declare a variable based on a class, the
compiler allocates memory for it. This portion of memory would be
available and used while the program is running and as long as the
compiler judges this necessary. While the program is running, the instance
of the class uses or consumes the computer's resources as necessary. When
the object is not needed anymore, for example when the program terminates,
the object must be destroyed, the memory it was using must be emptied to
be made available to other programs on the computer, and the resources
that the object was using should (must) be freed to be restored to the
computer so they can be used by other programs. To make this possible, the
Object class is equipped with a method called Finalize that is
protected and therefore made available to all descendant classes of the
.NET Framework.
The syntax of the Object.Finalize() method is:
Overrides Protected Sub Finalize()
The Finalize() method is automatically called
when an instance of a class is not needed anymore. In all of the
classes we have used so far, this method was transparently called when the
compiler judged that the instance of the class was not used anymore. If
you don't want this method to be called, call the
Public Shared Sub SuppressFinalize(ByVal obj As Object)
In most cases, you can let the compiler call the
Finalize() method when the time comes.
|
|