|
The variables of a program cannot perform assignments. In the same way, the
member variables of a class cannot perform actions. So far, we were
using procedures to do that. In the same way, to create a class as complete as
possible, you can equip it with its own procedures. Such a procedure is created
as a member of the class.
|
A procedure that is made a member of a class is
called a method.
Practical
Learning: Introducing Methods
|
|
- Start Microsoft Visual Basic and create a Console Application named
DepartmentStore1
- In the Solution Explorer, right-click Module1.vb and click Rename
- Set the name to DepartmentStore.vb, press Enter, and accept to
rename the file
- Change the document as follows:
Public Module DepartmentStore
Public Function Main() As Integer
Return 0
End Function
End Module
|
- Save the file
- To create a new class, on the main menu, click Project -> Add Class...
- In the Templates section of the Add New Item dialog box, make sure the
Class item is selected.
Set the Name to StoreItem
- Click Add
- Change the document as follows:
Public Class StoreItem
Friend ItemNumber As Long
Friend ItemName As String
Friend UnitPrice As Double
End Class
|
- Save the file
- To use a DepartmentStore object,
change the Program.vb file as follows:
Public Module DepartmentStore
Public Function Main() As Integer
Dim dptStore As StoreItem
dptStore = New StoreItem
With dptStore
.ItemNumber = 437876
.ItemName = "Crinkled Georgette Dress"
.UnitPrice = 42.95
MsgBox("=-= Department Store =-=" & vbCrLf & _
"Item #:" & vbTab & vbTab & .ItemNumber & vbCrLf & _
"Item Name:" & vbTab & .ItemName & vbCrLf & _
"Unit Price: " & vbTab & FormatCurrency(.UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
End With
Return 0
End Function
End Module
|
- Execute the application to test it
- Click OK and return to your programming environment
To create a method, in the body of a class, use the
same formula we learned in Lesson 5. For example, to create a sub procedure, you
use the following formula:
Class Class Name
Sub ProcedureName()
End Sub
End Class
Here is an example:
Private Class Rectangle
Sub Show()
End Sub
End Class
In the body of the method, do whatever you want. For
example, you can call the MsgBox() function to display a message. Here is an
example:
Private Class Rectangle
Sub Show()
MsgBox("Whatever")
End Sub
End Class
Practical
Learning: Creating a Method
|
|
- Access the StoreItem.vb file
- Create two methods as follows:
Public Class StoreItem
Friend ItemNumber As Long
Friend ItemName As String
Friend UnitPrice As Double
Sub Create()
ItemNumber = 790475
ItemName = "New Wool Comfort Pants"
UnitPrice = 38.75
End Sub
Sub Display()
MsgBox("=-= Department Store =-=" & vbCrLf & _
"Item #:" & vbTab & vbTab & ItemNumber & vbCrLf & _
"Item Name:" & vbTab & ItemName & vbCrLf & _
"Unit Price: " & vbTab & FormatCurrency(UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
End Sub
End Class
|
- Save the file
To access a method outside of its class, you can use the
period operator as we saw for the member variables. Here is an example
Public Module Exercise
Private Class Rectangle
Sub Show()
MsgBox("Whatever")
End Sub
End Class
Public Function Main() As Integer
Dim Recto As Rectangle
Recto = New Rectangle
Recto.Show()
Return 0
End Function
End Module
In the same way, you can use a With statement to access
a method of a class.
Practical
Learning: Calling the Methods of a Class
|
|
- Access the DepartmentStore.vb file
- To use the methods of the class,
change the code as follows:
Public Module DepartmentStore
Public Function Main() As Integer
Dim dptStore As StoreItem
dptStore = New StoreItem
With dptStore
.Create()
.Display()
End With
Return 0
End Function
End Module
|
- Execute the application to test it
- Click OK and return to your programming environment
Using the Member Variables of a Class |
|
In the previous sections, we saw how to create member
variables inside of a class. Here is an example:
Class Rectangle
Length As Double
Height As Double
Sub Show()
End Sub
End Class
When you have created a member variable in a class, it is
available to all methods of the same class. This means that, to use it from a
method of the same class, you do not have to declare a variable for the class.
Here is an example:
Public Module Exercise
Private Class Rectangle
Public Length As Double
Friend Height As Double
Sub Show()
MsgBox("=-= Rectangle Characteristics =-=" & vbCrLf & _
"Length: " & vbTab & Length & vbCrLf & _
"Height: " & vbTab & Height)
End Sub
End Class
Public Function Main() As Integer
Dim Recto As Rectangle
Recto = New Rectangle
Recto.Length = 24.55 : Recto.Height = 20.75
Recto.Show()
Return 0
End Function
End Module
Notice that the Length and the Height member variables are
accessed inside of the Show() method without an instance of the Rectangle class.
Details on Implementing Methods |
|
The Case of Function Methods |
|
So far, we have seen how to create sub procedure in a class, which is a method
that does not return a value. Like a normal procedure, a method can be made to return a value, in which case
it would be a function. To create a method as a function, use the same
techniques we have used so far to create a function. Here is an example:
Friend Class Rectangle
Public Length As Double
Public Height As Double
Function Assign() As Double
End Function
End Class
After declaring a procedure, in its body, you can
implement the expected behavior. As seen for a sub procedure, when a method has been created, it has
access to all of the other members of the same class. This means that you
don't have to re-declare a member of a class to access it in a method.
Based on this, you can manipulate any member variable of the same class as
you wish. This means that, naturally, you do not have to create a function
method to change the value of a member variable. A normal sub procedure can take
care of this. Instead, you would create a method if you need to perform a
calculation and get a value from it instead of storing that value in one of the
member variables. Here is an example:
Friend Class Rectangle
Public Length As Double
Public Height As Double
Function Assign() As Double
End Function
Function Perimeter() As Double
End Function
End Class
Therefore, in the body of the function, you can access a
member variable, you can call another method of the same class, or you can use
an external value as you see fit. When the function exits, make sure it returns
the appropriate value based on its type. Like the member variables, the methods can be accessed outside
of the class using the period operator. Here is an example:
Public Module Exercise
Friend Class Rectangle
Public Length As Double
Public Height As Double
Function Perimeter() As Double
Return (Length + Height) * 2
End Function
Function Area#()
Return Length * Height
End Function
End Class
Public Function Main() As Integer
Dim Recto As Rectangle
Recto = New Rectangle
Recto.Length = 42.58 : Recto.Height = 28.08
MsgBox("=-= Rectangle Characteristics =-=" & vbCrLf & _
"Length: " & vbTab & vbTab & Recto.Length & vbCrLf & _
"Height: " & vbTab & vbTab & Recto.Height & vbCrLf & _
"Perimeter: " & vbTab & Recto.Perimeter() & vbCrLf & _
"Area: " & vbTab & vbTab & Recto.Area())
Return 0
End Function
End Module
This would produce:

Like a sub procedure that is created as a method, a member
function of a class can be made private, public, or friendly. It follows the
exact same rules we reviewed early.
Practical
Learning: Implementing the Methods of a Class
|
|
- Start Microsoft Visual Basic and create a Console Application named
DepartmentStore2
- In the Solution Explorer, right-click Module1.vb and click Rename
- Set the name to DepartmentStore.vb, press Enter, and accept to
rename the file
- To add two methods of the class, change the file as follows:
Module DepartmentStore
Public Class StoreItem
Public ItemNumber As Long
Public ItemName As String
Public UnitPrice As Double
Public Sub RegisterStoreItem()
ItemNumber = CLng(InputBox("=#= Department Store =#=" & vbCrLf & _
" --- Item Registration ---" & vbCrLf & _
vbCrLf & vbCrLf & vbCrLf & _
"Enter Item #", "Department Store"))
ItemName = InputBox("=#= Department Store =#=" & vbCrLf & _
" --- Item Registration ---" & vbCrLf & _
"Enter Item Name", "Department Store")
UnitPrice = CDbl(InputBox("=#= Department Store =#=" & vbCrLf & _
" --- Item Registration ---" & vbCrLf & _
vbCrLf & vbCrLf & vbCrLf & _
"Enter Unit Price", "Department Store"))
End Sub
Public Sub ShowItem()
MsgBox("=-= Department Store =-=" & vbCrLf & _
"--- Store Inventory ---" & vbCrLf & _
"Item #:" & vbTab & vbTab & ItemNumber & vbCrLf & _
"Item Name:" & vbTab & ItemName & vbCrLf & _
"Unit Price:" & vbTab & FormatCurrency(UnitPrice), _
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information, _
"Department Store")
End Sub
End Class
Public Function Main() As Integer
Dim dptStore As StoreItem = New StoreItem
dptStore.RegisterStoreItem()
dptStore.ShowItem()
Return 0
End Function
End Module
|
- Execute the program to test it
- Enter the item # as 740797
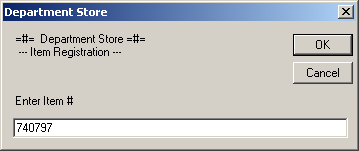
- Enter the item name as Pencil Skirt

- Enter the price as 25.75
- Close the form and return to your programming environment