 |
Introduction to the LINQ and Class |
|
|
A list used in a LINQ statement can be made of any type
of value (numbers, strings, etc), as long as the values are of the same
type. Here is an example:
|
Imports System.Linq
Module Exercise
Public Function Main() As Integer
Dim Names(4) As String
Names(0) = "Patricia Katts"
Names(1) = "Raymond Kouma"
Names(2) = "Hélène Mukoko"
Names(3) = "Paul Bertrand Yamaguchi"
Names(4) = "Gertrude Monay"
Dim Name = From N
In Names
Select N
For Each Member In Name
Console.WriteLine("Member: " & Member)
Next
Return 0
End Function
End Module
This would produce:
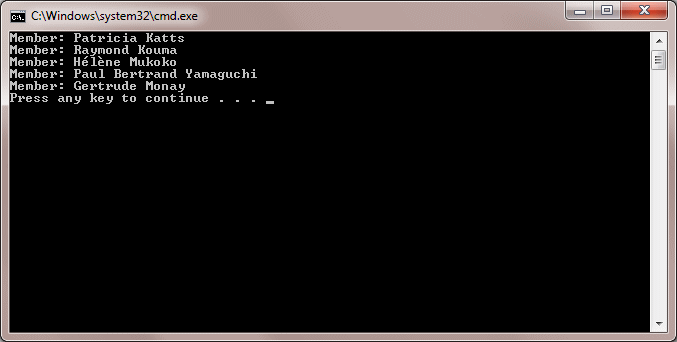
The values used in a LINQ statement can also come from
a class. For example, instead of using one of the primitive types to
create a list, you can use your own class. Here is an example:
Public Class Employee
Public EmployeeNumber As Integer
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
You primarily use the class as you would any other. In
your LINQ statement, you can refer to all members of the collection:
Imports System.Linq
Public Class Employee
Public EmployeeNumber As Integer
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
Module Exercise
Public Function Main() As Integer
Dim Employees(4) As Employee
Employees(0) = New Employee
Employees(0).EmployeeNumber = 971974
Employees(0).FirstName = "Patricia"
Employees(0).LastName = "Katts"
Employees(0).HourlySalary = 24.68
Employees(1) = New Employee
Employees(1).EmployeeNumber = 208411
Employees(1).FirstName = "Raymond"
Employees(1).LastName = "Kouma"
Employees(1).HourlySalary = 20.15
Employees(2) = New Employee
Employees(2).EmployeeNumber = 279374
Employees(2).FirstName = "Hélène"
Employees(2).LastName = "Mukoko"
Employees(2).HourlySalary = 15.55
Employees(3) = New Employee
Employees(3).EmployeeNumber = 707912
Employees(3).FirstName = "Bertrand"
Employees(3).LastName = "Yamaguchi"
Employees(3).HourlySalary = 24.68
Employees(4) = New Employee
Employees(4).EmployeeNumber = 971394
Employees(4).FirstName = "Gertrude"
Employees(4).LastName = "Monay"
Employees(4).HourlySalary = 20.55
Dim Staff = From N In Employees Select N
Return 0
End Function
End Module
In this case, the value of the Select
expression represents the whole variable, which are all members of the
collection. If you want to get a property (or a member) of the class,
apply the period operator to the value of Select and
access the desired member. Here is an example:
Imports System.Linq
Public Class Employee
Public EmployeeNumber As Integer
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
Module Exercise
Public Function Main() As Integer
Dim Employees(4) As Employee
Employees(0) = New Employee
Employees(0).EmployeeNumber = 971974
Employees(0).FirstName = "Patricia"
Employees(0).LastName = "Katts"
Employees(0).HourlySalary = 24.68
Employees(1) = New Employee
Employees(1).EmployeeNumber = 208411
Employees(1).FirstName = "Raymond"
Employees(1).LastName = "Kouma"
Employees(1).HourlySalary = 20.15
Employees(2) = New Employee
Employees(2).EmployeeNumber = 279374
Employees(2).FirstName = "Hélène"
Employees(2).LastName = "Mukoko"
Employees(2).HourlySalary = 15.55
Employees(3) = New Employee
Employees(3).EmployeeNumber = 707912
Employees(3).FirstName = "Bertrand"
Employees(3).LastName = "Yamaguchi"
Employees(3).HourlySalary = 24.68
Employees(4) = New Employee
Employees(4).EmployeeNumber = 971394
Employees(4).FirstName = "Gertrude"
Employees(4).LastName = "Monay"
Employees(4).HourlySalary = 20.55
Dim LastNames = From N
In Employees
Select N.LastName
For Each Member In LastNames
Console.WriteLine("Member: {0}", Member)
Next
Return 0
End Function
End Module
This would produce:
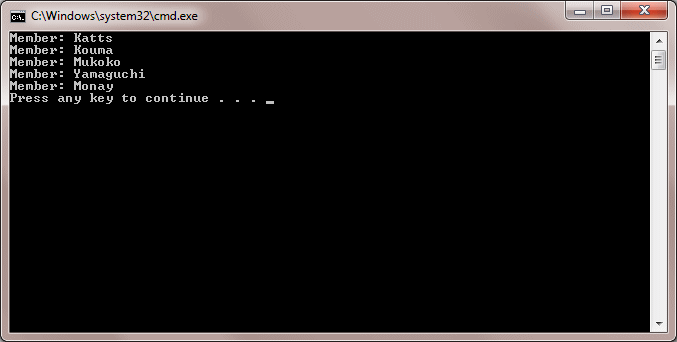
This technique allows you to access only one member of
the class.
As mentioned already, the select
statement primarily produces the whole collection of the values of the
variable. Since this value represents a collection, you can use it in a
list-based such scenario. In this case, to access a member of the class,
use a for or foreach loop to get each
item of the collection variable and apply the period operator on that
value.
Practical
Learning: Creating a Query
|
|
- Change the Load event as follows:
Imports System.IO
Imports System.Runtime.Serialization.Formatters.Binary
Module AltairRealtors
Private lstProperties() As House
Function Main() As Integer
Dim stmProperties As FileStream = Nothing
Dim bfmProperties As BinaryFormatter = New BinaryFormatter
' This is the file that holds the list of properties
Dim Filename As String = "C:\Altair Realtors\Properties.atr"
' Find out if there is already a file that contains a list of properties.
' If that file exists, open it.
If File.Exists(Filename) Then
stmProperties = New FileStream(Filename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
' Retrieve the list of items from file
lstProperties = CType(bfmProperties.Deserialize(stmProperties), House())
Dim Numbers = From iNumbers
In lstProperties
Select iNumbers.PropertyNumber
Dim i As Integer = 1
Console.WriteLine(" # Prop #")
Console.WriteLine("-----------")
For Each PropNbr In Numbers
Console.WriteLine("{0,2}. {1}", i, PropNbr)
i = i + 1
Next
Console.WriteLine("==========")
Finally
stmProperties.Close()
End Try
End If
Return 0
End Function
End Module
- Execute the application to see the result
# Prop #
-----------
1. 524880
2. 688364
3. 611464
4. 749562
5. 420115
6. 200417
7. 927474
8. 682630
9. 288540
10. 247472
11. 297446
12. 924792
13. 294796
14. 811155
15. 447597
16. 297415
17. 475974
18. 927409
19. 304750
20. 207850
==========
Press any key to continue . . .
- Close the DOS window and return to your programming environment
- Change the file as follows:
Imports System.IO
Imports System.Runtime.Serialization.Formatters.Binary
Module AltairRealtors
Private lstProperties() As House
Function Main() As Integer
Dim stmProperties As FileStream = Nothing
Dim bfmProperties As BinaryFormatter = New BinaryFormatter
' This is the file that holds the list of properties
Dim Filename As String = "C:\Altair Realtors\Properties.atr"
' Find out if there is already a file that contains a list of properties.
' If that file exists, open it.
If File.Exists(Filename) Then
stmProperties = New FileStream(Filename,
FileMode.Open,
FileAccess.Read,
FileShare.Read)
Try
' Retrieve the list of items from file
lstProperties = CType(bfmProperties.Deserialize(stmProperties), House())
Dim properties = From props
In lstProperties
Select props
Dim i As Integer = 1
Console.WriteLine("+===+========+==============+===============+=======+===========+======+=======+=======+======+===========+")
Console.WriteLine("| # | Prop # | Type | City | State | Condition | Beds | Baths |Stories| Year | Value |")
Console.WriteLine("+===+========+==============+===============+=======+===========+======+=======+=======+======+===========+")
For Each prop In properties
Console.WriteLine("| {0,2}| {1,-6} | {2,-12} | {3,-13} | {4,-4} | {5,-9} | {6,-3} | {7,-5} | {8,-4}| {9,-4} | {10,6} |",
i, prop.PropertyNumber, prop.Type, prop.City, prop.State, prop.Condition, prop.Bedrooms,
prop.Bathrooms.ToString("F"), prop.Stories, prop.YearBuilt, prop.MarketValue.ToString("F"))
Console.WriteLine("+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+")
i = i + 1
Next
Finally
stmProperties.Close()
End Try
End If
Return 0
End Function
End Module
- Execute the application to see the result
+===+========+==============+===============+=======+===========+======+=======+=======+======+===========+
| # | Prop # | Type | City | State | Condition | Beds | Baths |Stories| Year | Value |
+===+========+==============+===============+=======+===========+======+=======+=======+======+===========+
| 1| 524880 | SingleFamily | Silver Spring | MD | Good | 4 | 2.50 | 3 | 1995 | 495880.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 2| 688364 | SingleFamily | Alexandria | VA | Excellent | 4 | 3.50 | 2 | 2000 | 620724.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 3| 611464 | SingleFamily | Laurel | MD | Good | 1 | 0.00 | 2 | 0 | 422625.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 4| 749562 | Townhouse | Gettysburg | WV | Good | 3 | 2.50 | 3 | 2002 | 425400.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 5| 420115 | Unknown | Washington | DC | Unknown | 2 | 0.00 | 0 | 1982 | 312555.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 6| 200417 | Condominium | Germantown | MD | Excellent | 2 | 1.00 | 0 | 0 | 215495.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 7| 927474 | Townhouse | Arlington | VA | BadShape | 4 | 2.50 | 3 | 1992 | 415665.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 8| 682630 | SingleFamily | Martinsburg | WV | Good | 4 | 3.50 | 3 | 2005 | 325000.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 9| 288540 | Condominium | Silver Spring | MD | Good | 1 | 1.00 | 0 | 2000 | 242775.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 10| 247472 | SingleFamily | Silver Spring | MD | Excellent | 3 | 3.00 | 3 | 1996 | 625450.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 11| 297446 | Townhouse | Laurel | MD | Unknown | 4 | 1.50 | 2 | 2002 | 412885.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 12| 924792 | SingleFamily | Washington | DC | Good | 5 | 3.50 | 3 | 2000 | 555885.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 13| 294796 | SingleFamily | Falls Church | VA | Excellent | 5 | 2.50 | 2 | 1995 | 485995.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 14| 811155 | Condominium | Alexandria | VA | Good | 1 | 1.00 | 0 | 2000 | 352775.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 15| 447597 | Townhouse | Hyattsville | MD | Excellent | 3 | 2.00 | 3 | 1992 | 365880.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 16| 297415 | Townhouse | ashington | DC | Good | 4 | 3.50 | 1 | 2004 | 735475.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 17| 475974 | SingleFamily | Gaithersburg | MD | Unknown | 4 | 2.50 | 1 | 1965 | 615775.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 18| 927409 | Condominium | McLean | VA | Excellent | 1 | 1.00 | 12 | 2006 | 485900.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 19| 304750 | Condominium | Washington | DC | Unknown | 2 | 2.00 | 6 | 1992 | 388665.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
| 20| 207850 | Townhouse | Rockville | MD | Good | 3 | 2.50 | 2 | 1988 | 525995.00 |
+---+--------+--------------+---------------+-------+-----------+------+-------+-------+------+-----------+
Press any key to continue . . .
- Close the DOS window and return to your programming environment
|
|