Application:
Overloading Binary Operators
|
|
- Access the Rational.vb file and change it as follows:
Imports Mathematics
Public Class Rational
Private Num As Long
Private Den As Long
Public Sub New(ByVal n As Long, ByVal d As Long)
Num = n
Den = d
End Sub
Public Property Numerator() As String
Get
Return Num / Arithmetic.GreatestCommonDivisor(Num, Den)
End Get
Set(ByVal value As String)
Num = value
End Set
End Property
Public Property Denominator() As String
Get
Return Den / Arithmetic.GreatestCommonDivisor(Num, Den)
End Get
Set(ByVal value As String)
Den = value
End Set
End Property
Public Shared Operator +(ByVal First As Rational,
ByVal Second As Rational) As Rational
Dim Top As Long
Dim Bottom As Long
Top = (First.Numerator * Second.Denominator) +
(First.Denominator * Second.Numerator)
Bottom = First.Denominator * Second.Denominator
Return New Rational(Top, Bottom)
End Operator
Public Shared Operator -(ByVal First As Rational,
ByVal Second As Rational) As Rational
Dim Top As Long
Dim Bottom As Long
Top = (First.Numerator * Second.Denominator) -
(First.Denominator * Second.Numerator)
Bottom = First.Denominator * Second.Denominator
Return New Rational(Top, Bottom)
End Operator
Public Shared Operator *(ByVal First As Rational,
ByVal Second As Rational) As Rational
Dim Top As Long
Dim Bottom As Long
Top = First.Numerator * Second.Numerator
Bottom = First.Denominator * Second.Denominator
Return New Rational(Top, Bottom)
End Operator
Public Shared Operator /(ByVal First As Rational,
ByVal Second As Rational) As Rational
Dim Top As Long
Dim Bottom As Long
Top = First.Numerator * Second.Denominator
Bottom = First.Denominator * Second.Numerator
Return New Rational(Top, Bottom)
End Operator
Public Overrides Function ToString() As String
Dim Numer As Long
Dim Denom As Long
Numer = Num / Arithmetic.GreatestCommonDivisor(Num, Den)
Denom = Den / Arithmetic.GreatestCommonDivisor(Num, Den)
If Denom = 1 Then
Return Numer.ToString()
Else
Return String.Format("{0}/{1}", Numer, Denom)
End If
End Function
End Class
- Access the Algebra.vb file and change it as follows:
Module Algebra
Public Function Main() As Integer
Dim a, b, c, d As Long
Dim FirstFraction As Rational = Nothing
Dim SecondFraction As Rational = Nothing
MsgBox("This program allows you to perform " &
"an arithmetic operation on two fractions")
a = CLng(InputBox("=-= First Fraction =-=" & vbCrLf &
"Enter the numerator:"))
b = CLng(InputBox("=-= First Fraction =-=" & vbCrLf &
"Enter the denominator: "))
c = CLng(InputBox("=-= Second Fraction =-=" & vbCrLf &
"Enter the numerator:"))
d = CLng(InputBox("=-= Second Fraction =-=" & vbCrLf &
"Enter the denominator:"))
FirstFraction = New Rational(a, b)
MsgBox(String.Format("First Fraction: {0}/{1} => {2}", a, b, FirstFraction))
SecondFraction = New Rational(c, d)
MsgBox(String.Format("Second Fraction: {0}/{1} => {2}", c, d, SecondFraction))
Dim Addition As Rational
Dim Subtraction As Rational
Dim Multiplication As Rational
Dim Division As Rational
Addition = FirstFraction + SecondFraction
MsgBox(String.Format("Addition: {0}/{1} + {2}/{3} = {4}", a, b, c, d, Addition))
Subtraction = FirstFraction - SecondFraction
MsgBox(String.Format("Addition: {0}/{1} + {2}/{3} = {4}", a, b, c, d, Subtraction))
Multiplication = FirstFraction * SecondFraction
MsgBox(String.Format("Addition: {0}/{1} + {2}/{3} = {4}", a, b, c, d, Multiplication))
Division = FirstFraction / SecondFraction
MsgBox(String.Format("Addition: {0}/{1} + {2}/{3} = {4}", a, b, c, d, Division))
Return 0
End Function
End Module
- To execute the application, on the main menu, click Debug -> Start
Debugging
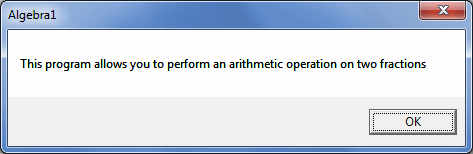
- Click OK
- When requested, enter the first numerator as 128
and press Enter
- Enter the first denominator as 54 and press Enter
- Enter the second numerator as 88 and press Enter
- Enter the second denominator as 36 and press Enter
Besides the traditional arithmetic operations, Visual
Basic support the ability to add or shift bits in the computer memory. These
operators are the bitwise conjunction operator & and its assignment
operator &=, the bitwise disjunction operator | and its assignment operator
|=, the bitwise exclusion operator ^ and its assignment operator ^=, the
left-shift operator << and its assigment operator <<=, the right-shift
operator >> and its assignment operator >>=. When necessary, overload only
the primary operator (&, |, ^, <<, >>) and its equivalent assignment
operator will be automatically provided by the compiler.
To overloaded a binary operator, pass two arguments to
the parentheses of the method. For the two bitwise shift operators, << and
>>, the first argument must be the class in which you are working and the
second argument must by a constant integer (type int). Here
is an example that overloads the left bitwise shifter <<:
Public Class Natural
Public Number As Integer
Public Sub New(ByVal N As Integer)
Number = N
End Sub
Public Shared Operator Not(ByVal Value As Natural) As Natural
Dim Nbr As Integer = Value.Number
Dim N As Integer = Not Nbr
Dim Nat As Natural = New Natural(N)
Return Nat
End Operator
Public Shared Operator +(ByVal Value As Natural, ByVal Add As Integer) As Natural
Dim Nbr As Integer = Value.Number
Dim N As Integer = Nbr + Add
Dim Nat As Natural = New Natural(N)
Return Nat
End Operator
Public Shared Operator <<(ByVal Value As Natural, ByVal Shifts As Integer) As Natural
Dim Nbr As Integer = Value.Number
Dim N As Integer = (Nbr << Shifts)
Dim Nat As Natural = New Natural(N)
Return Nat
End Operator
Public Overrides Function ToString() As String
Return Number.ToString()
End Function
End Class
Here is an example of using the operator:
Public Module Exercise
Public Function Main() As Integer
Dim Nbr As Natural
Nbr = New Natural(1248)
MsgBox("Shiftting the bits of " & Nbr.ToString() &
" to the left by 4 is " & (Nbr << 4).ToString())
Return 0
End Function
End Module
This would produce:

For the operators that join or disjoin two series of
bits:
- The first argument must be the class on which you are working. The
second argument can be an integer. Here is an example:
Public Shared Operator And(ByVal Value As Natural,
ByVal Constant As Integer) As Natural
Dim Result As Integer = Value.Number And Constant
Return New Natural(Result)
End Operator
-----------------------------------------------------------------------
Public Module Exercise
Public Function Main() As Integer
Dim Nbr As Natural
Nbr = New Natural(286)
MsgBox("286 AND 4075 = " & (Nbr And 4075).ToString())
Return 0
End Function
End Module

- The first argument must be the class on which you are working. The
second argument can be a class of the same type as the first argument.
Here is an example:
Public Class Natural
Public Number As Integer
Public Sub New(ByVal N As Integer)
Number = N
End Sub
Public Shared Operator Not(ByVal Value As Natural) As Natural
Dim Nbr As Integer = Value.Number
Dim N As Integer = Not Nbr
Dim Nat As Natural = New Natural(N)
Return Nat
End Operator
Public Shared Operator +(ByVal Value As Natural, ByVal Add As Integer) As Natural
Dim Nbr As Integer = Value.Number
Dim N As Integer = Nbr + Add
Dim Nat As Natural = New Natural(N)
Return Nat
End Operator
Public Shared Operator <<(ByVal Value As Natural, ByVal Shifts As Integer) As Natural
Dim Nbr As Integer = Value.Number
Dim N As Integer = (Nbr << Shifts)
Dim Nat As Natural = New Natural(N)
Return Nat
End Operator
Public Shared Operator And(ByVal Value As Natural,
ByVal Constant As Integer) As Natural
Dim Result As Integer = Value.Number And Constant
Return New Natural(Result)
End Operator
Public Shared Operator And(ByVal Operand1 As Natural,
ByVal Operand2 As Natural) As Natural
Dim Result As Integer = Operand1.Number And Operand2.Number
Return New Natural(Result)
End Operator
Public Overrides Function ToString() As String
Return Number.ToString()
End Function
End Class
---------------------------------------------------
Public Module Exercise
Public Function Main() As Integer
Dim Nbr1 As Natural = New Natural(52)
Dim Nbr2 As Natural = New Natural(2447)
MsgBox("52 AND 2447 = " & (Nbr1 And Nbr2).ToString())
Return 0
End Function
End Module
This would produce:
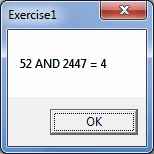
In the same way you can overload the other operators.
As its name implies, a comparison is used to find out
whether one of two values is higher than the other. The values must be of
the same type. Comparison operators go in pair. That is, if you want to
compare two values for equality, you must also be able to know when they are
different. For this reason, if you decide to overload a comparison operator,
you must also overload its opposite:
- If you overload =, you must also overload <> and vice versa
- If you overload <, you must also overload > and vice versa
- If you overload <=, you must also overload >= and vice versa
- If you overload IsTrue, you must also overload
IsFalse and vice versa
We know that the Object class is
equipped with a method named Equals. That method makes it
possible to find out whether one object is equal to another. And since all
classes in a Visual Basic application derive from Object,
if you decide to overload a couple of comparison operators, you should also
override the Equals() method.
Some operators either cannot be overloaded or depend on
other operators being overloaded. The following operators cannot be
overloaded: =, AddressOf, AndAlso,
AsType, GetType, Is,
IsNot, New, OrElse, and
TypeOf ... Is.
Application:
Ending the Lesson
|
|
- Close your programming environment
- When asked whether you want to save, click No
|
|