- Create a new Dialog Based MFC Application named CarInventory2 without the About Box and set the Dialog Title to
Car Inventory
- Resize the dialog box to 430 x 230
- On the dialog box, delete the TODO line if it exists and the OK button
- Change the caption of the Cancel button to Close
- From the Controls toolbox, click the Tree Control button and click on the left area of the dialog box
- Change its ID to IDC_CAR_TREE
- Set its location to 7, 7 and its dimensions to 114 x 216
- On the dialog box, click the tree control to select it. In the Properties window set the
Has Buttons, the Has Lines, the Lines At Root, and the
Scroll properties to True
- Also set the Client Edge and the Modal Frame properties to
True
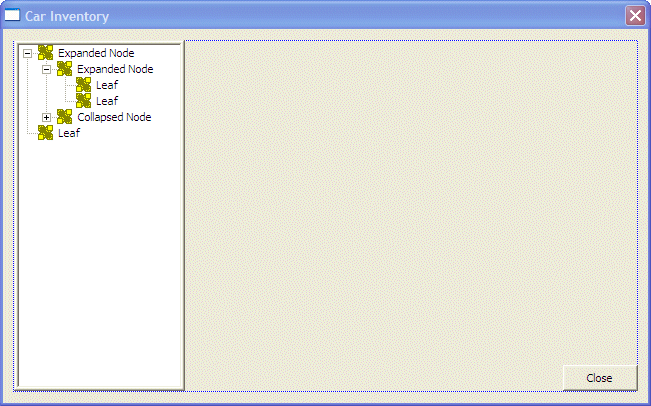
- Add a Control Variable to the tree object and name it m_CarTree
- Save All
- To create the items for the tree control, access the OnInitDialog() event of the CCarInventory2Dlg class and type the following:
BOOL CCarInventory2Dlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
HTREEITEM hItem, hCar;
hItem = m_CarTree.InsertItem("Car Listing", TVI_ROOT);
hCar = m_CarTree.InsertItem("Economy", hItem);
m_CarTree.InsertItem("BH-733", hCar);
m_CarTree.InsertItem("SD-397", hCar);
m_CarTree.InsertItem("JU-538", hCar);
m_CarTree.InsertItem("DI-285", hCar);
m_CarTree.InsertItem("AK-830", hCar);
hCar = m_CarTree.InsertItem("Compact", hItem);
m_CarTree.InsertItem("HG-490", hCar);
m_CarTree.InsertItem("PE-473", hCar);
hCar = m_CarTree.InsertItem("Standard", hItem);
m_CarTree.InsertItem("SO-398", hCar);
m_CarTree.InsertItem("DF-438", hCar);
m_CarTree.InsertItem("IS-833", hCar);
hCar = m_CarTree.InsertItem("Full Size", hItem);
m_CarTree.InsertItem("PD-304", hCar);
hCar = m_CarTree.InsertItem("Mini Van", hItem);
m_CarTree.InsertItem("ID-497", hCar);
m_CarTree.InsertItem("RU-304", hCar);
m_CarTree.InsertItem("DK-905", hCar);
hCar = m_CarTree.InsertItem("SUV", hItem);
m_CarTree.InsertItem("FE-948", hCar);
m_CarTree.InsertItem("AD-940", hCar);
hCar = m_CarTree.InsertItem("Truck", hItem);
m_CarTree.InsertItem("HD-394", hCar);
return TRUE; // return TRUE unless you set the focus to a control
}
|
- Execute the program to test the tree control
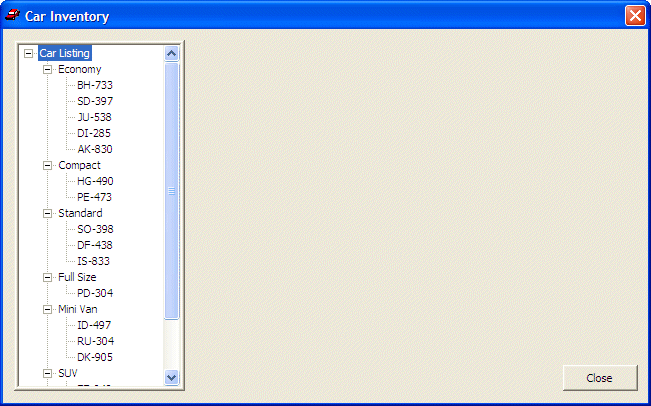
- Close the dialog box and return to MSVC
- Save the following cars to CarInventory2 folder of the current project:
 |
 |
 |
IDB_CIVIC |
IDB_FOCUS |
IDB_ACCENT |
 |
 |
 |
IDB_ESCORT |
IDB_ELANTRA |
IDB_MYSTIQUE |
 |
 |
 |
IDB_GRANDMARQUIS |
IDB_NAVIGATOR |
IDB_ESCAPE |
- On the main menu, click Project -> Add Resource...
- In the Add Resource dialog box, click Import...
- Locate the folder that contains the above pictures. Select each picture
and change their IDs as indicated in the above table
- Add another Bitmap resource and change its ID to IDB_EMPTY (design
it anyway you like or leave it empty)
- Design the rest of the dialog box with additional controls as follows:
 |
Control |
Caption |
ID |
Other Properties |
Picture |
|
|
|
Static Text |
Make: |
|
|
Edit Box |
|
IDC_MAKE |
|
Static Text |
Model: |
|
|
Edit Box |
|
IDC_MODEL |
|
Static Text |
Year: |
|
|
Edit Box |
|
IDC_YEAR |
|
Static Text |
Doors: |
|
|
Edit Box |
|
IDC_DOORS |
|
Static Text |
Mileage: |
|
|
Edit Box |
|
IDC_MILEAGE |
|
Static Text |
Transmission: |
|
|
Combo Box |
|
IDC_TRANSMISSION |
Data: Automatic;Manual |
Group Box |
Options |
|
|
Check Box |
Air Condition |
IDC_AC |
Left Text: True |
Check Box |
Air Bags |
IDC_AIR_BAGS |
Left Text: True |
Check Box |
Cruise Control |
IDC_CRUISE_CONTROL |
Left Text: True |
Check Box |
Convertible |
IDC_CONVERTIBLE |
Left Text: True |
Check Box |
Cassette |
IDC_CASSETTE |
|
Check Box |
CD Player |
IDC_CD_PLAYER |
Left Text: True |
Picture |
|
IDC_PICTURE |
Type: Bitmap
Image: IDB_CIVIC
Center Image: True |
|
- Add a variable to each non-static control as follows:
ID |
Value Variable |
Control Variable |
Type |
Name |
Type |
Name |
IDC_MAKE |
CString |
m_Make |
|
|
IDC_MODEL |
CString |
m_Model |
|
|
IDC_YEAR |
int |
m_Year |
|
|
IDC_DOORS |
int |
m_Doors |
|
|
IDC_MILEAGE |
long |
m_Mileage |
|
|
IDC_TRANSMISSION |
int |
m_Transmission |
|
|
IDC_AC |
BOOL |
m _AC |
|
|
IDC_AIR_BAGS |
BOOL |
m _AirBags |
|
|
IDC_CRUISE_CONTROL |
BOOL |
m _CruiseControl |
|
|
IDC_CONVERTIBLE |
BOOL |
m_Convertible |
|
|
IDC_CASSETTE |
BOOL |
m _Cassette |
|
|
IDC_CD_PLAYER |
BOOL |
m _CDPlayer |
|
|
IDC_PICTURE |
|
|
CStatic |
m_Picture |
- Save All
- On the dialog box, right-click the tree control and click Add Event Handler…
- In the Message Type list box, click TVN_SELCHANGED. In the Class List, make sure CCarInventory2Dlg is selected
- In the Function Handle Name, change the name to OnCarSelectedChange and click Add And Edit
- Implement the OnPaint and the OnCarSelectedChange events as follows:
void CCarInventory2Dlg::OnPaint()
{
CPaintDC dc(this); // device context for painting
// Change the car picture based on the selected tag
CTreeCtrl *pTree = reinterpret_cast<CTreeCtrl *>(GetDlgItem(IDC_CAR_TREE));
HTREEITEM hTree = pTree->GetSelectedItem();
CString ItemSelected;
CBitmap Bmp;
ItemSelected = pTree->GetItemText(hTree);
if( ItemSelected == "BH-733" )
{
Bmp.LoadBitmap(IDB_FOCUS);
m_Picture.SetBitmap(Bmp);
}
else if( ItemSelected == "FE-948" )
{
Bmp.LoadBitmap(IDB_NAVIGATOR);
m_Picture.SetBitmap(Bmp);
}
else if( ItemSelected == "HG-490" )
{
Bmp.LoadBitmap(IDB_ELANTRA);
m_Picture.SetBitmap(Bmp);
}
else if( ItemSelected == "DF-438" )
{
Bmp.LoadBitmap(IDB_MYSTIQUE);
m_Picture.SetBitmap(Bmp);
}
else if( ItemSelected == "SD-397" )
{
Bmp.LoadBitmap(IDB_ACCENT);
m_Picture.SetBitmap(Bmp);
}
else if( ItemSelected == "AD-940" )
{
Bmp.LoadBitmap(IDB_ESCAPE);
m_Picture.SetBitmap(Bmp);
}
else if( ItemSelected == "HD-394" )
{
Bmp.LoadBitmap(IDB_EMPTY);
m_Picture.SetBitmap(NULL);
}
else if( ItemSelected == "SD-397" )
{
Bmp.LoadBitmap(IDB_EMPTY);
m_Picture.SetBitmap(NULL);
}
else if( ItemSelected == "PD-304" )
{
Bmp.LoadBitmap(IDB_GRANDMARQUIS);
m_Picture.SetBitmap(Bmp);
}
else
{
Bmp.LoadBitmap(IDB_EMPTY);
m_Picture.SetBitmap(NULL);
}
if (IsIconic())
{
SendMessage(WM_ICONERASEBKGND, reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
// The system calls this function to obtain the cursor
// to display while the user drags
// the minimized window.
HCURSOR CCarInventory2Dlg::OnQueryDragIcon()
{
return static_cast<HCURSOR>(m_hIcon);
}
void CCarInventory2Dlg::OnCarSelectedChange(NMHDR *pNMHDR, LRESULT *pResult)
{
LPNMTREEVIEW pNMTreeView = reinterpret_cast<LPNMTREEVIEW>(pNMHDR);
// TODO: Add your control notification handler code here
*pResult = 0;
CTreeCtrl *pTree = reinterpret_cast<CTreeCtrl *>(GetDlgItem(IDC_CAR_TREE));
HTREEITEM hTree = pTree->GetSelectedItem();
CString ItemSelected;
CBitmap Bmp;
ItemSelected = pTree->GetItemText(hTree);
if( ItemSelected == "BH-733" )
{
m_Make.Format("%s", "Ford");
m_Model.Format("%s", "Focus");
m_Year = 2000;
m_Doors = 4;
m_Mileage = 72804;
m_Transmission = 1;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = FALSE;
m_CDPlayer = FALSE;
}
else if( ItemSelected == "SD-397" )
{
m_Make.Format("%s", "Hyundai");
m_Model.Format("%s", "Accent");
m_Year = 2000;
m_Doors = 4;
m_Mileage = 42775;
m_Transmission = 1;
m_AC = FALSE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = FALSE;
m_CDPlayer = FALSE;
}
else if( ItemSelected == "DF-438" )
{
m_Make.Format("%s", "Mercury");
m_Model.Format("%s", "Mystique");
m_Year = 2004;
m_Doors = 4;
m_Mileage = 48616;
m_Transmission = 0;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = FALSE;
m_CDPlayer = TRUE;
}
else if( ItemSelected == "AD-940" )
{
m_Make.Format("%s", "Ford");
m_Model.Format("%s", "Escape");
m_Year = 2005;
m_Doors = 5;
m_Mileage = 58225;
m_Transmission = 1;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = TRUE;
m_CDPlayer = FALSE;
}
else if( ItemSelected == "FE-948" )
{
m_Make.Format("%s", "Lincoln");
m_Model.Format("%s", "Navigator");
m_Year = 2003;
m_Doors = 5;
m_Mileage = 63820;
m_Transmission = 0;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = TRUE;
m_Convertible = FALSE;
m_Cassette = TRUE;
m_CDPlayer = TRUE;
}
else if( ItemSelected == "HG-490" )
{
m_Make.Format("%s", "Hyundai");
m_Model.Format("%s", "Elantra");
m_Year = 2001;
m_Doors = 4;
m_Mileage = 60116;
m_Transmission = 1;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = FALSE;
m_CDPlayer = FALSE;
}
else if( ItemSelected == "HD-394" )
{
m_Make.Format("%s", "Ford");
m_Model.Format("%s", "F150");
m_Year = 2002;
m_Doors = 4;
m_Mileage = 28845;
m_Transmission = 0;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = TRUE;
m_CDPlayer = FALSE;
}
else if( ItemSelected == "SD-397" )
{
m_Make.Format("%s", "Daewoo");
m_Model.Format("%s", "Lanos");
m_Year = 2000;
m_Doors = 4;
m_Mileage = 94508;
m_Transmission = 1;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = TRUE;
m_CDPlayer = FALSE;
}
else if( ItemSelected == "PD-304" )
{
m_Make.Format("%s", "Mercury");
m_Model.Format("%s", "Grand Marquis");
m_Year = 2000;
m_Doors = 4;
m_Mileage = 109442;
m_Transmission = 0;
m_AC = TRUE;
m_AirBags = TRUE;
m_CruiseControl = TRUE;
m_Convertible = FALSE;
m_Cassette = TRUE;
m_CDPlayer = FALSE;
}
else
{
m_Make.Format("%s", "");
m_Model.Format("%s", "");
m_Year = 0;
m_Doors = 0;
m_Mileage = 0;
m_Transmission = 0;
m_AC = FALSE;
m_AirBags = FALSE;
m_CruiseControl = FALSE;
m_Convertible = FALSE;
m_Cassette = FALSE;
m_CDPlayer = FALSE;
}
CRect RectPicture;
m_Picture.GetWindowRect(&RectPicture);
ScreenToClient(&RectPicture);
InvalidateRect(&RectPicture);
UpdateData(FALSE);
}
|
- Test the application
- Close it and return to MSVC
The List Control and Images |
|
As reviewed in the tree control, you can display an
icon on the left of the caption of a node of a tree control. To start, you can
create some icons or bitmaps and store them in a CImageList object.
Practical Learning: Using Images on a Tree List
|
|
- Display the Add Resource dialog box and double-click Bitmap
- On the Properties, change its ID to IDB_IMGTREE
- Design is as follows:

- In the header file of the dialog, declare a private CImageList variable and name it
ImgList
- To assign the pictures to the tree items, change the OnInitDialog() event of the dialog box as follows:
BOOL CCarInventory2Dlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
HTREEITEM hItem, hCar;
m_Picture.SetBitmap(NULL);
ImgList.Create(IDB_IMGTREE, 16, 6, RGB(0, 0, 0));
m_CarTree.SetImageList(&ImgList, TVSIL_NORMAL);
hItem = m_CarTree.InsertItem("Car Listing", 0, 1, TVI_ROOT);
hCar = m_CarTree.InsertItem("Economy", 2, 3, hItem);
m_CarTree.InsertItem("BH-733", 4, 5, hCar);
m_CarTree.InsertItem("SD-397", 4, 5, hCar);
m_CarTree.InsertItem("JU-538", 4, 5, hCar);
m_CarTree.InsertItem("DI-285", 4, 5, hCar);
m_CarTree.InsertItem("AK-830", 4, 5, hCar);
hCar = m_CarTree.InsertItem("Compact", 2, 3, hItem);
m_CarTree.InsertItem("HG-490", 4, 5, hCar);
m_CarTree.InsertItem("PE-473", 4, 5, hCar);
hCar = m_CarTree.InsertItem("Standard", 2, 3, hItem);
m_CarTree.InsertItem("SO-398", 4, 5, hCar);
m_CarTree.InsertItem("DF-438", 4, 5, hCar);
m_CarTree.InsertItem("IS-833", 4, 5, hCar);
hCar = m_CarTree.InsertItem("Full Size", 2, 3, hItem);
m_CarTree.InsertItem("PD-304", 4, 5, hCar);
hCar = m_CarTree.InsertItem("Mini Van", 2, 3, hItem);
m_CarTree.InsertItem("ID-497", 4, 5, hCar);
m_CarTree.InsertItem("RU-304", 4, 5, hCar);
m_CarTree.InsertItem("DK-905", 4, 5, hCar);
hCar = m_CarTree.InsertItem("SUV", 2, 3, hItem);
m_CarTree.InsertItem("FE-948", 4, 5, hCar);
m_CarTree.InsertItem("AD-940", 4, 5, hCar);
hCar = m_CarTree.InsertItem("Truck", 2, 3, hItem);
m_CarTree.InsertItem("HD-394", 4, 5, hCar);
return TRUE; // return TRUE unless you set the focus to a control
}
|
- Test the application
- Close it and return to MSVC
|
|