Introduction to List Boxes
|
|
|
A list box presents a list of items to choose from. Each
item displays on a line:
|

The user makes a selection by clicking in the list.
Once clicked, the item or line on which the mouse landed becomes
highlighted, indicating that the item is the current choice. Once an item
is selected, to make a different selection, the user would click another.
The user can also press the up and down arrow keys to navigate through the
list and make a selection.
As far as item selection is concerned, there are two
types of list boxes: single selection and multiple selection.
One of the main reasons for using a list box is to
display a list of items to the user. Sometimes the list would be very
large. If the list is longer than the available space on the control, the
operating system would provide a scroll bar that allows the user to
navigate up and down to access all items of the list. Therefore, you will
have the option of deciding how many items to display on the list.
Practical
Learning: Introducing List Boxes
|
|
- Start Microsoft Visual Studio
- To start a new application, on the main menu, click File -> New
Project...
- In the middle list, click MFC Application and change the Name to
MeasuesOfCenter1
- In the first page of the wizard, click Next
- In the second page of the wizard, click Dialog Based and click
Next
- Click Finish
To include a list box in your application, from the
Toolbox, you can click the List Box button
and click on a parent window. After visually adding the control, if you
intend to refer to it in your code, you should create a member variable
for it.
The MFC list box is based on the CListBox
class. Therefore, if you want to programmatically create a list box,
declare a CListBox variable or pointer using its
constructor. To initialize the control, call its Create()
member function. Its syntax is:
virtual BOOL Create(DWORD dwStyle,
const RECT& rect,
CWnd* pParentWnd,
UINT nID);
Here is an example:
BOOL CExerciseDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CListBox *m_SimpleList = new CListBox;
m_SimpleList->Create(WS_CHILD | WS_VISIBLE,
CRect(20, 20, 120, 120),
this,
0x118);
return TRUE; // return TRUE unless you set the focus to a control
}
A newly added list box appears as a rectangular empty
box with a white background.
Practical
Learning: Creating a List Box
|
|
- Design the dialog box as follows:
 |
Control |
Caption |
ID |
Align Text |
Static Text |
 |
Value (x): |
|
|
Edit Box |
 |
|
IDC_VALUE |
Right |
Button |
 |
Add |
IDC_ADD |
|
List Box |
 |
|
IDC_VALUES |
|
Button |
 |
Reset |
IDC_RESET |
|
Static Text |
 |
Count: |
|
|
Edit Box |
 |
|
IDC_COUNT |
Right |
Static Text |
 |
Sum: |
|
|
Edit Box |
 |
|
IDC_SUM |
Right |
Static Text |
 |
Mean: |
|
|
Edit Box |
 |
|
IDC_MEAN |
|
Button |
 |
Close |
IDCANCEL |
|
|
- Right-click each of the edit controls and click Add Variable...
- Create the variables as follows:
ID |
Category |
Type |
Name |
IDC_VALUE |
Value |
double |
m_Value |
IDC_VALUES |
Control |
CListBox |
m_Values |
IDC_COUNT |
Value |
int |
m_Count |
IDC_SUM |
Value |
double |
m_Sum |
IDC_MEAN |
Value |
double |
m_Mean |
- In the Class View, expand the project and, in the top part,
double-click CMeasuresOfCenter1Dlg
- Declare a CList variable named Values and that takes double as
parameter:
// MeasuesOfCenter1Dlg.h : header file
//
#pragma once
#include "afxwin.h"
// CMeasuesOfCenter1Dlg dialog
class CMeasuesOfCenter1Dlg : public CDialogEx
{
// Construction
public:
CMeasuesOfCenter1Dlg(CWnd* pParent = NULL);// standard constructor
// Dialog Data
enum { IDD = IDD_MEASUESOFCENTER1_DIALOG };
protected:
virtual void DoDataExchange(CDataExchange* pDX);// DDX/DDV support
// Implementation
protected:
HICON m_hIcon;
// Generated message map functions
virtual BOOL OnInitDialog();
afx_msg void OnPaint();
afx_msg HCURSOR OnQueryDragIcon();
DECLARE_MESSAGE_MAP()
public:
double m_Value;
CListBox m_Values;
int m_Count;
double m_Sum;
double m_Mean;
private:
CArray<double, double> Values;
};
- To execute and preview the dialog box, press F5
- Close the dialog box and return to your programming environment
Adding a String to a List Box
|
|
After creating a list box, you can add items to it.
This is done by calling the AddString() member function
of the CListBox class. Its syntax is:
int AddString(LPCTSTR lpszItem);
This member function expects a null-terminated string
as argument and adds this argument to the control. To add more items, you
must call this member function for each desired item. Here is an example:
BOOL CExerciseDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
m_CollegeMajors.AddString(L"Biology");
m_CollegeMajors.AddString(L"Accounting");
m_CollegeMajors.AddString(L"Art Education");
m_CollegeMajors.AddString(L"Finance");
m_CollegeMajors.AddString(L"Computer Science");
return TRUE; // return TRUE unless you set the focus to a control
}
The items of a list box are arranged as a zero-based
array. The top item has an index of zero. The second item as an index of
1, etc. Although the items seem to be added randomly to the list, their
new position depends on whether the list is sorted or not. If the list is
sorted, each new item is added to its alphabetical position. If the list
is not sorted, each item is added on top of the list as if it were the
first item. The other items are then "pushed down".
The Number of Strings in a List Box
|
|
At any time, if you want to know the number of items
that a list box holds, call the GetCount() member
function of the CListBox class. Its syntax is:
int GetCount() const;
This member function simply returns a count of the
items in the list. Here is an example of calling it:
BOOL CExerciseDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
m_CollegeMajors.AddString(L"Biology");
m_CollegeMajors.AddString(L"Accounting");
m_CollegeMajors.AddString(L"Art Education");
m_CollegeMajors.AddString(L"Finance");
m_CollegeMajors.AddString(L"Computer Science");
m_Count = m_CollegeMajors.GetCount();
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
This would produce:

Practical
Learning: Populating a List Box
|
|
- On the main menu, click Project -> Class Wizard...
- In the Class Name combo box, select CMeasuresOfCenter1Dlg.
Click Methods
- Click Add Method...
- Set the Return Type to void
- Set the Function Name to ShowValues

- Click OK
- Click Commands
- In the Commands list, click IDC_ADD
- Click Add Handler...
- Accept the suggested name of the function and click OK
- Click Edit Code
- Implement the event as follows:
void CMeasuesOfCenter1Dlg::ShowValues(void)
{
UpdateData();
double Sum = 0, Mean, Median;
CString strValue;
// Check the values in the collection
for(int i = 0; i < Values.GetCount(); i++)
{
// Calculate the sum of values
Sum += Values[i];
// Since the value is a double, first convert it to a CString, ...
strValue.Format(_T("%.3f"), Values[i]);
// then add the string to the list box
m_Values.AddString(strValue);
}
// Calculate the average
Mean = Sum / Values.GetCount();
// Display the values in the edit boxes
m_Count = m_Values.GetCount();
m_Sum = Sum;
m_Mean = Mean;
UpdateData(FALSE);
}
void CMeasuesOfCenter1Dlg::OnClickedAdd()
{
// TODO: Add your control notification handler code here
UpdateData();
// Get the new value and store it in the collection
Values.Add(m_Value);
// Reset the Value edit box
m_Value = 0;
UpdateData(FALSE);
// Display the values
ShowValues();
}
Inserting a String Into a List Box
|
|
Inserting a string consists of putting inside the list
in a position of your choice. To support this operation, the
CListBox class is equipped with the InsertString()
member function whose syntax is:
int InsertString(int nIndex, LPCTSTR lpszItem);
The lpszItem argument is the string to be
added to the list. The nIndex argument specifies the new position
in the zero-based list. Here is an example:
BOOL CExerciseDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
m_CollegeMajors.AddString(L"Biology");
m_CollegeMajors.AddString(L"Accounting");
m_CollegeMajors.AddString(L"Art Education");
m_CollegeMajors.AddString(L"Finance");
m_CollegeMajors.AddString(L"Computer Science");
m_CollegeMajors.InsertString(1, L"Criminal Justice");
m_Count = m_CollegeMajors.GetCount();
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
This would produce:

If you pass it as 0, the lpszItem item would
be made the first in the list. If you pass it as -1, lpszItem
would be added at the end of the list, unless the list is empty.
Deleting a String From a List Box
|
|
To delete an item from the list, pass its index to the
DeleteString() member function of the CListBox class. Its
syntax is:
int DeleteString(UINT nIndex);
For example, to delete the second item of the list,
pass a 1 value to this member function.
If you want to delete all items of the control, call
the ResetContent() member function of the
CListBox class. Its syntax is:
void ResetContent();
This member function simply dismisses the whole
content of the list box.
Practical
Learning: Resetting a List Box
|
|
- Call the CListBox::ResetContent() member function at the beginning
of the ShowValues() member function:
void CMeasuesOfCenter1Dlg::ShowValues(void)
{
UpdateData();
double Sum = 0, Mean, Median;
CString strValue;
// Clear the list box before populating it with strings from the collection
m_Values.ResetContent();
// Check the values in the collection
for(int i = 0; i < Values.GetCount(); i++)
{
// Calculate the sum of values
Sum += Values[i];
// Since the value is a double, first convert it to a CString, ...
strValue.Format(_T("%.3f"), Values[i]);
// then add the string to the list box
m_Values.AddString(strValue);
}
// Calculate the average
Mean = Sum / Values.GetCount();
// Display the values in the edit boxes
m_Count = m_Values.GetCount();
m_Sum = Sum;
m_Mean = Mean;
UpdateData(FALSE);
}
- To execute, press F5
- Type each of the following values and click Add after each:
72604, 7592, 6314,
57086, 24885

- Close the dialog box and return to your programming environment
Selecting an Item From a List Box
|
|
Once a list has been created, you and your users can
use its items. For example, to select an item, the user clicks it. To
programmatically select an item (on a list box that allows only single
selections), call the SetCurSel() member function of the
CListBox class. Its syntax is:
int SetCurSel(int nSelect);
The nSelect argument specifies the item to
select. To select the fourth item from a list box, you can pass the
nSelect argument with a value of 3. Here is an example:
BOOL CExerciseDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
m_CollegeMajors.AddString(L"Biology");
m_CollegeMajors.AddString(L"Accounting");
m_CollegeMajors.AddString(L"Art Education");
m_CollegeMajors.AddString(L"Finance");
m_CollegeMajors.AddString(L"Computer Science");
m_CollegeMajors.InsertString(1, L"Criminal Justice");
m_CollegeMajors.SetCurSel(3);
m_Count = m_CollegeMajors.GetCount();
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
If an item is selected in the list and you want to
find out which one, you can call the GetCurSel() member function of
the CListBox class. Its syntax is:
int GetCurSel() const;
Here is an example of calling it:
void CExerciseDlg::OnBnClickedGetSelection()
{
// TODO: Add your control notification handler code here
m_Selected = m_CollegeMajors.GetCurSel();
UpdateData(FALSE);
}
This would produce:

As you can see, the CListBox::GetCurSel()
member function returns the index of the string that was selected. If you
want to get the actual string, you can call the GetText()
member function of the CListBox class. It is overloaded
with two versions whose syntaxes are:
int GetText(int nIndex, LPTSTR lpszBuffer) const;
void GetText(int nIndex, CString& rString) const;
The first argument represents the index of the item
whose string you want to find out. The second argument is the returned
string. Here is an example that returns a CString value by using the
second version of the function:
void CExerciseDlg::OnBnClickedGetSelection()
{
// TODO: Add your control notification handler code here
m_CollegeMajors.GetText(m_CollegeMajors.GetCurSel(), m_Selected);
UpdateData(FALSE);
}
Here is an example of running it:
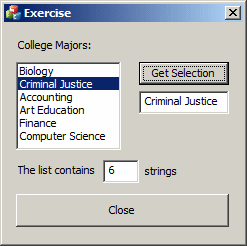