An MFC static library is a library file that includes MFC
functions or classes. Such a library natively supports MFC strings (such as
CString, CTime, etc), lists, and any other C/C++ or MFC global
functions. Because this library is an MFC type, it can be used only by an
MFC-based application.
Creation of an MFC Static Library |
|
To create an MFC static library, from the New dialog box, select Win32
Static Library and specify the directory. Then, in the Win32 Static
Library - Step 1 of 1, mark the MFC Support check box. You can also ask
Microsoft Visual C++ to generate precompiled header files:
- If you don't mark the Precompiled Header check box, an empty project would be created
- If you select the Precompiled Header radio button, the wizard will generate a StdAfx.h header file and a StdAfx.cpp source file for the project. The wizard will also include the afx.h and the afxwin.h files in the StdAfx.h header file. You can still add any cenessary header file in StdAfx.h
Practical Learning: Creating an MFC Static Library
|
|
- To start a new project, on the main menu, click File -> New…
- In the New dialog box, click the Projects property page
- In the list, click Win32 Static Library
- In the Project Name edit box, type MFCExt

- Click OK
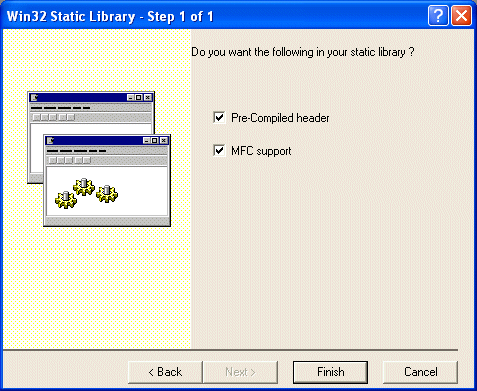
- In the Win32 Static Library – Step 1 of 1, click both check boxes and click Finish then click OK
- To add a new header file, on the main menu, click File -> New…
- In the Files property page of the New dialog box, click C/C++ Header File. In the File Name edit box, type mfcextent and click OK
- In the empty file, type the following:
#ifndef MFCEXT_H_
#define MFCEXT_H_
namespace MFCExtensions
{
BOOL IsNatural(const CString Str);
BOOL IsNumeric(const CString Str);
int StringToInt(const CString Str);
double StringToFloat(const CString Str);
}
#endif // MFCEXT_H
|
- To add a new source file, on the main menu, click File -> New… In the Files property page of the New dialog box, click C++ Source File. In the File Name edit box, type mfcextent and click OK
- In the empty file, type the following:
#include "stdafx.h"
#include " mfcextent.h"
namespace MFCExtensions
{
BOOL IsNatural(const CString Str)
{
// Check each character of the string
// If a character at a certain position is not a digit,
// then the string is not a valid natural number
for(int i = 0; i < Str.GetLength(); i++)
{
if( Str[i] < '0' || Str[i] > '9' )
return FALSE;
}
return TRUE;
}
BOOL IsNumeric(const CString Str)
{
// Make a copy of the original string to test it
CString WithoutSeparator = Str;
// Prepare to test for a natural number
// First remove the decimal separator, if any
WithoutSeparator.Replace(".", "");
// If this number were natural, test it
// If it is not even a natural number, then it can't be valid
if( IsNatural(WithoutSeparator) == FALSE )
return FALSE; // Return Invalid Number
// Set a counter to 0 to counter the number of decimal separators
int NumberOfSeparators = 0;
// Check each charcter in the original string
for(int i = 0; i < Str.GetLength(); i++)
{
// If you find a decimal separator, count it
if( Str[i] == '.' )
NumberOfSeparators++;
}
// After checking the string and counting the decimal separators
// If there is more than one decimal separator,
// then this cannot be a valid number
if( NumberOfSeparators > 1 )
return FALSE; // Return Invalid Number
else // Otherwise, this appears to be a valid decimal number
return TRUE;
}
int StringToInt(const CString Str)
{
// First check to see if this is a valid natural number
BOOL IsValid = IsNatural(Str);
// If this number is valid, then convert it
if( IsValid == TRUE )
return atoi(Str);
else
{
// Return 0 to be nice
return 0;
}
}
double StringToFloat(const CString Str)
{
// First check to see if this is a valid number
BOOL IsValid = IsNumeric(Str);
// If this number is valid, then convert it
if( IsValid == TRUE )
return atof(Str);
else
{
// Return 0 to be nice
return 0;
}
}
}
|
- To create the library, on the main menu, click Build -> Build MFCExt.lib
The Output window will indicate that a file with .lib extension was created
|
|