A message box is a rectangle object that displays
short message to the user. The message can be made of one sentence, one
paragraph, or a few paragraphs. To make the creation of a message box
easy, the Win32 library provides a specific function that can be used to
for this purpose.
|
To create a message box, use the MessageBox()
function. Its syntax is:
int MessageBox(HWND hWnd, LPCTSTR lpText, LPCTSTR lpCaption, UINT uType);
The first argument, hWnd, can be a handle to the
window from where the message box will be called. Otherwise, it can NULL.
The second argument, lpText, is a null-terminated
string, such as an array of characters. This is the actual message that
will be presented to the user. As stated already, it can be one word, a
whole sentence, a paragraph, even a hew paragraphs.
The third argument, lpCaption, is the title that will
display on the title bar. It also can be a null-terminated string, if you
know what title you would like to display. Otherwise, it can be NULL, in
which case the title bar would display Error.
The simplest way you can create a message is by
calling the MessageBox() function with all arguments set to NULL, in which
case the message box would not make any sense:
MessageBox(NULL, NULL, NULL, NULL);

As stated already, the first argument is either a
handle of the window that is calling it, or NULL.
The simplest way to specify the second argument is by
including a word or a sentence in double-quotes. Here is an example: |
MessageBox(NULL, L"I am just trying my wedding dress", NULL, NULL);
|

|
If you want to display the message on various lines,
you can separate sections with the new line character '\n'. Here is an
example: |
MessageBox(NULL, L"It happened earlier\nDidn't it?", NULL, NULL);
|
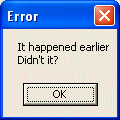
|
You can also use string editing techniques to create a
more elaborate message. This means that you can use functions of the C
string library to create your message.
The caption of the message can be any word or sentence
but convention wisdom would like this sentence to be in tune with the
actual message. After all, unless the message is about bad news, Error as
a title is not particularly cute.
The fourth argument actually does three things. First
it displays one or a few buttons. The buttons depend on the value
specified for the argument. If this argument is NULL, the message box
displays (only) OK. The values and their buttons can be as follows: |
Constant
Integer |
Buttons |
MB_OK |
 |
MB_OKCANCEL |
 |
MB_ABORTRETRYIGNORE |
 |
MB_YESNOCANCEL |
 |
MB_YESNO |
 |
MB_RETRYCANCEL |
 |
MB_CANCELTRYCONTINUE |
 |
MB_HELP |
 |
|
Besides the buttons, the message box can also display
a friendly icon that accompanies the message. Each icon is displayed by
specifying a constant integer. The values and their buttons are as
follows: |
Value |
Icon |
Suited
when |
MB_ICONEXCLAMATION
MB_ICONWARNING |
 |
Warning the user of an action performed on the
application |
MB_ICONINFORMATION
MB_ICONASTERISK |
 |
Informing the user of a non-critical situation |
MB_ICONQUESTION |
 |
Asking a question that expects a Yes or No, or a
Yes, No, or Cancel answer |
MB_ICONSTOP
MB_ICONERROR
MB_ICONHAND |
 |
A critical situation or error has occurred. This
icon is appropriate when informing the user of a termination or
deniability of an action |
|
The icons are used in conjunction with the buttons
constant. To combine these two flags, use the bitwise OR operator “|”.
|
The second thing this fourth argument does is to let
the user close the message box after selecting one of the buttons. Once
the user clicks one of the buttons, the message box is closed.
The third role of this fourth argument is to control
the result derived from the user dismissing the message box. For example,
clicking OK usually means that the user acknowledges what the message.
Clicking Cancel usually means the user is changing his or her mind about
the action performed previously. Clicking Yes instead of No usually
indicates that the user agrees to perform an action.
In reality, the message box only displays a message
and one or a few buttons. It is your responsibility as the programmer to
decide what to do when what button is clicked.
When a message box is configured to display more than
one button, the operating system is set to decide which button is the
default. The default button has a thick border that sets it apart from the
other button(s). If the user presses Enter, the message box would behave
as if the user had clicked the default button. Fortunately, if the message
box has more than one button, you can decide what button would be the
default. To specify the default button, use one of the following
constants:
|
Value |
If the
message box has more than one button, the default button would
be |
MB_DEFBUTTON1 |
The first button |
MB_DEFBUTTON2 |
The second button |
MB_DEFBUTTON3 |
The third button |
MB_DEFBUTTON4 |
The fourth button |
|
To specify the default button, use the bitwise OR operator
to combine the constant integer of the desired default button with the button's
constant and the icon. |
|
|