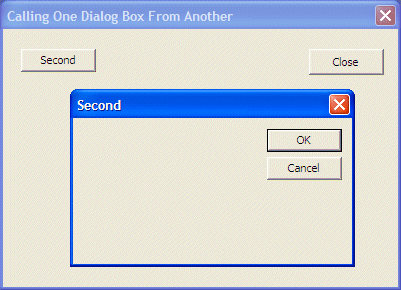
It is unusual to have a dialog-based application. This
is because a dialog box is usually made to complement a frame-based
application. In that case, after adding the dialog box to the application,
you would also provide the ability to call
the dialog from an SDI. In the same way, if you create an application made of various dialog
boxes, at one time, you may want the user to be able to call one dialog
box from another. To start, you would create the necessary dialog boxes.
Practical Learning: Calling One Dialog Box From Another
|
|
- Start your programming environment. For this example, we will use Borland
C++BuilderX.
Therefore, start Borland C++BuilderX and, on the main menu, click File ->
New...

- In the Object Gallery dialog box, click New GUI Application and click OK
- In the Name edit box, type Primary1 and click Next
- In the New GUI Application Project Wizard - Step 2 of 3, accept the
defaults and click Next
- In the New GUI Application Project Wizard - Step 3 of 3, click the check
box under Create
- Select Untitled under the Name column header. Type Exercise to
replace the name and press Tab
- Click Finish
- To create a resource header file, on the main menu, click File -> New
File...
- In the Create New File dialog box, in the Name, type Resource
- In the Type combo box, select h, and click OK
- In the file, type:
#define IDD_PRIMARY_DLG 102
#define IDD_SECOND_DLG 104
#define IDC_SECOND_BTN 106
|
- To create a resource script, on the main menu, click File -> New
File...
- In the Create New File dialog box, in the Name, type Primary1
- In the Type combo box, select rc, and click OK
- In the file, type:
#include "Resource.h"
/* -- Dialog Box: Primary -- */
IDD_PRIMARY_DLG DIALOGEX 0, 0, 263, 159
STYLE DS_SETFONT | DS_MODALFRAME | DS_FIXEDSYS | WS_POPUP | WS_VISIBLE |
WS_CAPTION | WS_SYSMENU
EXSTYLE WS_EX_APPWINDOW
CAPTION "Calling One Dialog Box From Another"
FONT 8, "MS Shell Dlg"
BEGIN
PUSHBUTTON "Close",IDCANCEL,204,12,50,16
PUSHBUTTON "Second",IDC_SECOND_BTN,12,12,50,14
END
/* -- Dialog Box: Second -- */
IDD_SECOND_DLG DIALOGEX 0, 0, 186, 90
STYLE DS_SETFONT | DS_MODALFRAME | DS_FIXEDSYS | WS_POPUP | WS_CAPTION |
WS_SYSMENU
CAPTION "Second"
FONT 8, "MS Shell Dlg"
BEGIN
DEFPUSHBUTTON "OK",IDOK,129,7,50,14
PUSHBUTTON "Cancel",IDCANCEL,129,24,50,14
END
|
- Display the Exercise.cpp file and change it as follows:
#include <windows.h>
#include "Resource.h"
#ifdef __BORLANDC__
#pragma argsused
#endif
//---------------------------------------------------------------------------
HWND hWnd;
HINSTANCE hInst;
const char *ClsName = "CallingDlg";
const char *WndName = "Calling One Dialog Box From Another";
LRESULT CALLBACK DlgProcPrimary(HWND hWndDlg, UINT Msg,
WPARAM wParam, LPARAM lParam);
LRESULT CALLBACK DlgProcSecondary(HWND hWndDlg, UINT Msg,
WPARAM wParam, LPARAM lParam);
//---------------------------------------------------------------------------
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
DialogBox(hInstance, MAKEINTRESOURCE(IDD_PRIMARY_DLG),
hWnd, (DLGPROC)DlgProcPrimary);
hInst = hInstance;
return FALSE;
}
//---------------------------------------------------------------------------
LRESULT CALLBACK DlgProcPrimary(HWND hWndDlg, UINT Msg,
WPARAM wParam, LPARAM lParam)
{
switch(Msg)
{
case WM_INITDIALOG:
return TRUE;
case WM_COMMAND:
switch(wParam)
{
case IDC_SECOND_BTN:
DialogBox(hInst, MAKEINTRESOURCE(IDD_SECOND_DLG),
hWnd, (DLGPROC)DlgProcSecondary);
return FALSE;
case IDCANCEL:
EndDialog(hWndDlg, 0);
return TRUE;
}
break;
}
return FALSE;
}
//---------------------------------------------------------------------------
LRESULT CALLBACK DlgProcSecondary(HWND hWndDlg, UINT Msg,
WPARAM wParam, LPARAM lParam)
{
switch(Msg)
{
case WM_INITDIALOG:
return TRUE;
case WM_COMMAND:
switch(wParam)
{
case IDOK:
EndDialog(hWndDlg, 0);
return TRUE;
case IDCANCEL:
EndDialog(hWndDlg, 0);
return TRUE;
}
break;
}
return FALSE;
}
//---------------------------------------------------------------------------
|
- Test the application
|
|