Due to the high level of support of XML in the .NET Framework, there are various ways you can create an XML
file. The most common technique consists of using a simple text editor. In
Microsoft Windows, this would be Notepad. An XML file is first of all a normal text-based
document that has a .xml extension. Therefore, however you create
it, you must specify that extension.
Many other applications allow creating an XML file or
generating one from an existing file. There are also commercial editors
you can get or purchase to create the XML file.
|
Practical
Learning: Introducing XML
|
|
- Start SharpDevelop
- To create a new project, on the main menu, click File -> New
-> Combine...
- In the Categories section of the New Project dialog box, click C# if
necessary.
In the Templates list, click Windows
Application
- Set the Name to CPAP1
- Specify the necessary path of the project in the Location text box
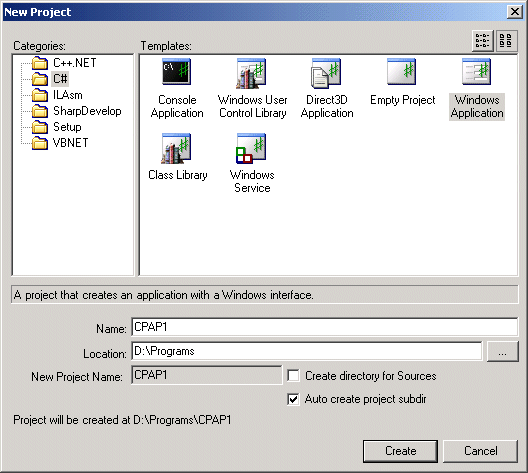
- Click Create
Creating an XML File With a Text Editor |
|
Probably the most common way to create an XML file in
Microsoft Windows consists of using Notepad or any other text editor.
After opening the text editor, you can enter the necessary lines of code. After creating the file, you must save it. When saving
it, you can include the name of the file in double-quotes. Here is an
example:
You can also first set the Save As Type combo box to
All Files and then enter the name of the file with the .xml extension |
Creating an XML File With SharpDevelop |
|
To create an XML file when working with SharpDevelop,
first display the New File dialog box available from the File menu. In the
New File dialog box, you can click the Empty XML File icon and click
Create. After clicking Create, a new file named Xml1.xml would
be created. You must then save it to make it part of your project.
To add a new XML file to a project you are working on
in SharpDevelop, in the Projects list, you can right-click any node,
position the mouse on Add, and click New File. This would prompt you to
select the type of file you want to create. In this case you would select
an XML file, give it a name, click Create.
|
Practical
Learning: Adding a New XML File
|
|
- On the main menu of SharpDevelop, click View -> Projects.
In the Projects list, right-click CPAP1, position the mouse on Add,
and click New File
- In the Categories list of the New File dialog box, click Misc
- In the Templates list, click Empty XML File
- In the File Name box, replace the string with Parts.xml
- Click Create
The Document Object Model and the XmlDocument |
|
To implement XML, the .NET Framework provides the System.Xml
namespace. When you create an XML file, there are standard rules you should
(must) follow in order to have a valid document. The standards for an XML file
are defined by the W3C Document Object Model (DOM). To support these standards,
the System.Xml namespace provides the XmlDocument class. This
class allows you to create an XML document, its contents, and many other related
operations you may want to perform on the contents of the file.
Creating XML Code Using XmlDocument |
|
To create XML code using XmlDocument, this
class has a method called LoadXml(). Its syntax is:
public virtual void LoadXml(string xml);
This method takes a string as argument.
The XmlDocument.LoadXml() method doesn't create an XML file, it
only allows you to provide or create XML code. The code can be created as
argument. You can also first declare and initialize a string
variable with the XML code, then pass
it as argument to the XmlDocument.LoadXml()
method.
The advantage of creating an XML file using
SharpDevelop is that the file, its name, and an extension are created
automatically. If you use the XmlDocument.LoadXml() method, only
the XML code is created, not the file. To actually create the Windows
file, you can call the XmlDocument.Save() method. This method is
provided in four versions. One of them takes as argument a string
value that would be the file name. The syntax of this method is:
public virtual void Save(string filename);
The argument must be a valid filename and must include
the .xml extension. If you pass a string without backlashes, the file
would be created in the same folder as the current project. If you want
the file to be created somewhere else, pass the whole path.
|
Practical
Learning: Creating an XML Document
|
|
- To display the form, in the top section of the Code Editor, click the
MainForm.cs tab and, in the lower section of the Code Editor, click the
Design tab
- In the Tools section, click Windows Forms. Click the Button control and click the
form
- In the Properties window, change its Text to Create Employees and its Name to btnCreateEmployees
- Double-click the newly added button to generate its Click event
- In the top section of the file, under the other using lines, type:
using System.Xml;
- Implement the Click event of the btnCreate button as
follows:
void BtnCreateClick(object sender, System.EventArgs e)
{
XmlDocument docXML = new XmlDocument();
docXML.LoadXml("");
}
|
- Save all
|
|