Checking Whether a Condition is
True/False |
|
|
In some programming assignments, you must find out
whether a given situation bears a valid value. This is done by checking a
condition. To support this, the Visual Basic language provides a series of words that can be
combined to perform this checking. Checking a condition usually
produces a True or a False result.
|
Once the condition has been checked,
you can use the result (as True or False) to take action. Because there
are different ways to check a condition, there are also different types of
keywords to check different things. To use them, you must be aware of what
each does or cannot do so you would select the right one.
Practical
Learning: Introducing Conditional Statements
|
|
- Start Microsoft Visual Basic and create a Console Application named BCR1
- In the Solution Explorer, right-click Module1.vb and click Rename
- Type BethesdaCarRental.vb and press Enter
- Accept to change the file name and change the document as follows:
Module BethesdaCarRental
Public Function Main() As Integer
Dim EmployeeName As String
Dim StrHourlySalary As String, StrWeeklyHours As String
Dim HourlySalaryFormatter As String
Dim WeeklyHoursFormatter As String
Dim Payroll As String
HourlySalaryFormatter = "0.00"
WeeklyHoursFormatter = "0.00"
EmployeeName = InputBox("Enter Employee Name:",
"Bethesda Car Rental",
"John Doe")
StrHourlySalary = InputBox("Enter Employee Hourly Salary:",
"Bethesda Car Rental", "0.00")
HourlySalaryFormatter = FormatCurrency(CDbl(StrHourlySalary))
StrWeeklyHours = InputBox("Enter Employee Weekly Hours:",
"Bethesda Car Rental", "0.00")
WeeklyHoursFormatter = FormatNumber(CDbl(StrWeeklyHours))
Payroll = "======================" & vbCrLf &
"=//= BETHESDA CAR RENTAL =//=" & vbCrLf &
"==-=-= Employee Payroll =-=-==" & vbCrLf &
"-------------------------------------------" & vbCrLf &
"Employee Name:" & vbTab & EmployeeName & vbCrLf &
"Hourly Salary:" & vbTab &
HourlySalaryFormatter & vbCrLf &
"Weekly Hours:" & vbTab &
WeeklyHoursFormatter & vbCrLf &
"======================"
MsgBox(Payroll, MsgBoxStyle.Information Or MsgBoxStyle.OkOnly,
"Bethesda Car Rental")
Return 0
End Function
End Module
- To execute the exercise, press Ctrl + F5
- Enter the Employee name as Helene Mukoko, the hourly salary as 22.35,
and the weekly hours as 38
- Close the message box and the DOS window to return to your programming
environment
If a Condition is True/False,
Then What?
|
|
The If...Then statement examines
the truthfulness of an expression. Structurally, its formula is:
If ConditionToCheck Then Statement
Therefore, the program examines a condition, in
this case ConditionToCheck.
This ConditionToCheck can be a simple expression or a combination of
expressions. If the ConditionToCheck is true, then the program will execute
the Statement.
There are two ways you can use the If...Then
statement. If the conditional formula is short enough, you can write
it on one line, like this:
If ConditionToCheck Then Statement
Here is an example:
Module Exercise
Public Function Main() As Integer
Dim IsMarried As Boolean
Dim TaxRate As Double
TaxRate = 33.0
MsgBox("Tax Rate: " & TaxRate & "%")
IsMarried = True
If IsMarried = True Then TaxRate = 30.65
MsgBox("Tax Rate: " & TaxRate & "%")
Return 0
End Function
End Module
This would produce:
If there are many statements to execute as a
truthful result of the condition, you should write the statements on
alternate lines. Of course, you can use this technique even if the
condition you are examining is short. In this case, one very
important rule to keep is to terminate the conditional statement
with End If. The formula used is:
If ConditionToCheck Then
Statement
End If
Here is an example:
Module Exercise
Public Function Main() As Integer
Dim IsMarried As Boolean
Dim TaxRate As Double
TaxRate = 33.0
MsgBox("Tax Rate: " & TaxRate & "%")
IsMarried = True
If IsMarried = True Then
TaxRate = 30.65
MsgBox("Tax Rate: " & TaxRate & "%")
End If
Return 0
End Function
End Module
Practical
Learning: Using If...Then
|
|
- To use an If condition, change the document as follows:
Module BethesdaCarRental
Public Function Main() As Integer
Dim EmployeeName As String
Dim StrHourlySalary As String, StrWeeklyHours As String
Dim HourlySalaryFormatter As String
Dim WeeklyHoursFormatter As String
Dim Payroll As String
HourlySalaryFormatter = "0.00"
WeeklyHoursFormatter = "0.00"
EmployeeName = InputBox("Enter Employee Name:",
"Bethesda Car Rental", "John Doe")
StrHourlySalary = InputBox("Enter Employee Hourly Salary:",
"Bethesda Car Rental", "0.00")
If IsNumeric(StrHourlySalary) = True Then
HourlySalaryFormatter = FormatCurrency(CDbl(StrHourlySalary))
End If
StrWeeklyHours = InputBox("Enter Employee Weekly Hours:",
"Bethesda Car Rental", "0.00")
If IsNumeric(StrWeeklyHours) = True Then
WeeklyHoursFormatter = FormatNumber(CDbl(StrWeeklyHours))
End If
Payroll = "======================" & vbCrLf &
"=//= BETHESDA CAR RENTAL =//=" & vbCrLf &
"==-=-= Employee Payroll =-=-==" & vbCrLf &
"-------------------------------------------" & vbCrLf &
"Employee Name:" & vbTab & EmployeeName & vbCrLf &
"Hourly Salary:" & vbTab &
HourlySalaryFormatter & vbCrLf &
"Weekly Hours:" & vbTab &
WeeklyHoursFormatter & vbCrLf &
"======================"
MsgBox(Payroll, MsgBoxStyle.Information Or MsgBoxStyle.OkOnly,
"Bethesda Car Rental")
Return 0
End Function
End Module
- Execute the application
- Enter the Employee name as Helene Mukoko, the hourly salary as 2W.o5,
and the weekly hours as Thirty Eight
- Close the message box and the DOS window then return to your programming
environment
Using the Default Value of a Boolean Expression
|
|
In the previous lesson, we saw that when you declare a
Boolean variable, by default, it is initialized with the False value. Here is an
example:
Module Exercise
Public Function Main() As Integer
Dim IsMarried As Boolean
MsgBox("Employee Is Married? " & IsMarried)
Return 0
End Function
End Module
This would produce:
Based on this, if you want to check whether a newly declared
and uninitialized Boolean variable is false, you can omit the = False expression
applied to it. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim IsMarried As Boolean
Dim TaxRate As Double
TaxRate = 33.0
If IsMarried Then TaxRate = 30.65
MsgBox("Tax Rate: " & TaxRate & "%")
Return 0
End Function
End Module
This would produce:
Notice that there is no = after the If IsMarried expression.
In this case, the compiler assumes that the value of the variable is False. On
the other hand, if you want to check whether the variable is True, make sure you
include the = True expression. Overall, whenever in doubt, it is safer to always
initialize your variable and it is safer to include the = True or = False
expression when evaluating the variable:
Module Exercise
Public Function Main() As Integer
Dim IsMarried As Boolean
Dim TaxRate As Double
TaxRate = 36.45 ' %
IsMarried = True
If IsMarried = False Then TaxRate = 33.15
MsgBox("Tax Rate: " & TaxRate & "%")
Return 0
End Function
End Module
In the previous lesson, we introduced some Boolean-based
functions such IsNumeric and IsDate. The default value of these functions is
true. This means that when you call them, you can omit the = True
expression.
Practical
Learning: Using the Default Value of Boolean-Based Functions
|
|
- To use the default value of the IsNumeric function, change the
document as follows:
Module BethesdaCarRental
Public Function Main() As Integer
. . . No Change
If IsNumeric(StrHourlySalary) Then
HourlySalaryFormatter = FormatCurrency(CDbl(StrHourlySalary))
End If
. . . No Change
If IsNumeric(StrWeeklyHours) Then
WeeklyHoursFormatter = FormatNumber(CDbl(StrWeeklyHours))
End If
. . . No Change
Return 0
End Function
End Module
- Save the file
If-Condition Based Functions |
|
We have learned how to check whether a condition is True or
False and take an action. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Status As UShort, EmploymentStatus As String
Status = 1
EmploymentStatus = "Unknown"
If Status = 1 Then
EmploymentStatus = "Full Time"
End If
MsgBox("Employment Status: " & EmploymentStatus)
Return 0
End Function
End Module
To provide an alternative to this operation, the Visual Basic language provides a function
named Choose. Its syntax
is:
Public Function Choose( _
ByVal Index As Double, _
ByVal ParamArray Choice() As Object _
) As Object
This function takes two required arguments. The fist
argument is equivalent to the ConditionToCheck of our If...Then formula. For the Choose() function, this first argument must be a number (a Byte, an
SByte, a Short,
a UShort, an Integer, a UInteger, a Long, a ULong,
a Single, a Double, or a Decimal
value). This is the value against which the second argument will be
compared. Before calling the function, you must know the value of the first
argument. To take care of this, you can first declare a variable and initialize
it with the desired value. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Status As UShort
Status = 1
Choose(Status, ...)
Return 0
End Function
End Module
The second argument can be the Statement of our
formula. Here is an example:
Choose(Status, "Full Time")
We will see in the next sections that the second argument is
actually a list of values and each value has a specific position referred to as
its index. To use the function in an If...Then scenario, you pass only
one value as the second argument. This value/argument has an index of 1. When the Choose() function
is called in an If...Then implementation, if the first argument holds a value
of 1, the second argument is validated.
When the Choose() function has been called, it returns a
value of type Object. You can retrieve that value, store it in
a variable and use it as you see fit. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Status As UShort, EmploymentStatus As String
Status = 1
EmploymentStatus = Choose(Status, "Full Time")
MsgBox("Employment Status: " & EmploymentStatus)
Return 0
End Function
End Module
This would produce:
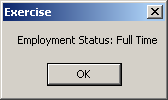
To give you another alternative to an If...Then
condition, the Visual Basic language provides a function named Switch.
Its syntax is:
Public Function Switch( _
ByVal ParamArray VarExpr() As Object _
) As Object
 |
In the .NET Framework, there is another Switch
implement that can cause a conflict when you call the Switch()
function in your program. Therefore, you must qualify this function when
calling it. To do this, use Microsoft.VisualBasic.Switch. |
This function takes one required argument. To use it in an If...Then
scenario, pass the argument as follows:
Switch(ConditionToCheck, Statement)
In the ConditionToCheck placeholder, pass a Boolean
expression that can be evaluated to True or False. If that condition is true,
the second argument would be executed.
When the Switch() function has been called, it
produces a value of type Object (such as a string) that you can use as
you see fit. For example, you can store it in a variable. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Status As UShort, EmploymentStatus As String
Status = 2
EmploymentStatus = "Unknown"
EmploymentStatus =
Microsoft.VisualBasic.Switch(Status = 1, "Full Time")
MsgBox("Employment Status: " & EmploymentStatus)
Return 0
End Function
End Module
In this example, we used a number as argument. You can also
use another type of value, such as an enumeration. Here is an example:
Module Exercise
Private Enum EmploymentStatus
FullTime
PartTime
Contractor
Seasonal
Unknown
End Enum
Public Function Main() As Integer
Dim Status As EmploymentStatus
Dim Result As String
Status = EmploymentStatus.FullTime
Result = "Unknown"
Result = Microsoft.VisualBasic.Switch( _
Status = EmploymentStatus.FullTime, "Full Time")
MsgBox("Employment Status: " & Result)
Return 0
End Function
End Module