If-Condition Built-In Functions |
|
Using the Immediate If Function
|
|
The IIf()
function can also be used in place of an If...Then...ElseIf
scenario. When the function is called, the Expression is
checked. As we saw already, if the expression is true, the function returns the
value of the TruePart argument and ignores the last argument. To use this
function as an alternative to If...Then...ElseIf statement, if the
expression is false, instead of immediately returning the value of the FalsePart
argument, you can translate that part into a new IIf function. The pseudo-syntax
would become:
Public Function IIf( _
ByVal Expression As Boolean, _
ByVal TruePart As Object, _
Public Function IIf( _
ByVal Expression As Boolean, _
ByVal TruePart As Object, _
ByVal FalsePart As Object _
) As Object
) As Object
In this case, if the expression is false, the function
returns the TruePart and stops. If the expression is false, the compiler
accesses the internal IIf function and applies the same scenario. Here is
example:
Module Exercise
Public Function Main() As Integer
Dim MemberAge As Short
Dim MembershipCategory As String
MemberAge = 74
MembershipCategory = _
IIf(MemberAge <= 18, "Teen", IIf(MemberAge < 55, "Adult", "Senior"))
MsgBox("Membership: " & MembershipCategory)
Return 0
End Function
End Module
We saw that in an If...Then...ElseIf statement you
can add as many ElseIf conditions as you want. In the same, you can call
as many IIf functions in the subsequent FalsePart sections as you
judge necessary:
Public Function IIf(
ByVal Expression As Boolean,
ByVal TruePart As Object,
Public Function IIf(
ByVal Expression As Boolean,
ByVal TruePart As Object,
Public Function IIf(
ByVal Expression As Boolean,
ByVal TruePart As Object,
Public Function IIf(
ByVal Expression As Boolean,
ByVal TruePart As Object,
ByVal FalsePart As Object
) As Object
) As Object
) As Object
) As Object
Choose an Alternate Value
|
|
As we have seen so far, the Choose function takes a
list of arguments. To use it as an alternative to the If...Then...ElseIf...ElseIf
condition, you can pass as many values as you judge necessary for the second
argument. The index of the first member of the second argument would be 1. The
index of the second member of the second argument would be 2, and so on. When
the function is called, it would first get the value of the first argument, then
it would check the indexes of the available members of the second argument. The
member whose index matches the first argument would be executed. Here is an
example:
Module Exercise
Public Function Main() As Integer
Dim Status As UShort, EmploymentStatus As String
Status = 3
EmploymentStatus = Choose(Status,
"Full Time",
"Part Time",
"Contractor",
"Seasonal")
MsgBox("Employment Status: " & EmploymentStatus)
Return 0
End Function
End Module
This would produce:
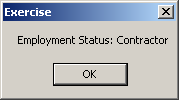
So far, we have used only strings for the values of the
second argument of the Choose() function. In reality, the values of the
second argument can be almost anything. One value can be a constant. Another
value can be a string. Yet another value can come from calling a function. Here
is an example:
Module Exercise
Private Function ShowContractors$()
Return "=-= List of Contractors =-=" & vbCrLf &
"Martin Samson" & vbCrLf &
"Geneviève Lam" & vbCrLf &
"Frank Viel" & vbCrLf &
"Henry Rickson" & vbCrLf &
"Samuel Lott"
End Function
Public Function Main() As Integer
Dim Status As UShort, Result$
Status = 3
Result = Choose(Status,
"Employment Status: Full Time",
"Employment Status: Part Time",
ShowContractors,
"Seasonal Employment")
MsgBox(Result)
Return 0
End Function
End Module
This would produce:
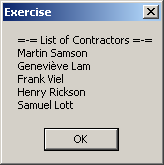
The values of the second argument can even be of different
types.
Practical
Learning: Using the Choose Function
|
|
- To use the Choose() function, change the document as follows:
Module BethesdaCarRental
Private Function GetEmployeeName(ByVal EmplNbr As Long) As String
Dim Name As String
If EmplNbr = 22804 Then
Name = "Helene Mukoko"
ElseIf EmplNbr = 92746 Then
Name = "Raymond Kouma"
ElseIf EmplNbr = 54080 Then
Name = "Henry Larson"
ElseIf EmplNbr = 86285 Then
Name = "Gertrude Monay"
Else
Name = "Unknown"
End If
Return Name
End Function
Public Function Main() As Integer
Dim EmployeeNumber As Long, EmployeeName As String
Dim CustomerName As String
Dim Tank As Integer, TankLevel As String
Dim RentStartDate As Date, RentEndDate As Date
Dim NumberOfDays As Integer
Dim RateType As String, RateApplied As Double
Dim OrderTotal As Double
Dim OrderInvoice As String
RateType = "Weekly Rate"
RateApplied = 0
OrderTotal = RateApplied
EmployeeNumber =
CLng(InputBox("Employee number (who processed this order):",
"Bethesda Car Rental", "00000"))
EmployeeName = GetEmployeeName(EmployeeNumber)
CustomerName = InputBox("Enter Customer Name:",
"Bethesda Car Rental", "John Doe")
Tank = CInt(InputBox("Enter Tank Level:" & vbCrLf &
"1. Empty" & vbCrLf &
"2. 1/4 Empty" & vbCrLf &
"3. 1/2 Full" & vbCrLf &
"4. 3/4 Full" & vbCrLf &
"5. Full",
"Bethesda Car Rental", 1))
TankLevel = Choose(Tank, "Empty", "1/4 Empty",
"1/2 Full", "3/4 Full", "Full")
RentStartDate = CDate(InputBox("Enter Rent Start Date:",
"Bethesda Car Rental", #1/1/1900#))
RentEndDate = CDate(InputBox("Enter Rend End Date:",
"Bethesda Car Rental", #1/1/1900#))
NumberOfDays = DateDiff(DateInterval.Day, RentStartDate, RentEndDate)
RateApplied = CDbl(InputBox("Enter Rate Applied:", _
"Bethesda Car Rental", 0))
OrderInvoice = "===========================" & vbCrLf &
"=//= BETHESDA CAR RENTAL =//=" & vbCrLf &
"==-=-= Order Processing =-=-==" & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Processed by:" & vbTab & EmployeeName & vbCrLf &
"Processed for:" & vbTab & CustomerName & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Car Selected:" & vbCrLf &
vbTab & "Tank:" & vbTab & TankLevel & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Start Date:" & vbTab & RentStartDate & vbCrLf &
"End Date:" & vbTab & RentEndDate & vbCrLf &
"Nbr of Days:" & vbTab & NumberOfDays & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Rate Type:" & vbTab & RateType & vbCrLf &
"Rate Applied:" & vbTab & RateApplied & vbCrLf &
"Order Total:" & vbTab & FormatCurrency(OrderTotal) & vbCrLf &
"==========================="
MsgBox(OrderInvoice,
MsgBoxStyle.Information Or MsgBoxStyle.OkOnly,
"Bethesda Car Rental")
Return 0
End Function
End Module
- Eexecute the application
- Enter the employee number as 22804, the customer name as George
Livingstone, the tank level as 2, the start date as 09/15/2008,
the end date as 09/19/2008, and the rate applied 65.75
- Close the message box and the DOS window to return to your programming
environment
Switching to an Alternate Value
|
|
The Switch() function is a prime alternative to the If...Then...ElseIf...ElseIf
condition. The argument to this function is passed as a list of values. As seen
previously, each value is passed as a combination of two values:
ConditionXToCheck, StatementX
As the function is accessed, the compiler checks each
condition. If a condition X is true, its statement is executed. If a condition Y
is false, the compiler skips it. You can provide as many of these combinations
as you want. Here is an
example:
Module Exercise
Private Enum EmploymentStatus
FullTime
PartTime
Contractor
Seasonal
End Enum
Public Function Main() As Integer
Dim Status As EmploymentStatus
Dim Result As String
Status = EmploymentStatus.Contractor
Result = "Unknown"
Result = Microsoft.VisualBasic.Switch(
Status = EmploymentStatus.FullTime, "Full Time",
Status = EmploymentStatus.PartTime, "Part Time",
Status = EmploymentStatus.Contractor, "Contractor",
Status = EmploymentStatus.Seasonal, "Seasonal")
MsgBox("Employment Status: " & Result)
Return 0
End Function
End Module
This would produce:
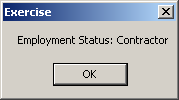
In a true If...Then...ElseIf...ElseIf condition, we
saw that there is a possibility that none of the conditions would fit, in which
case you can add a last Else statement. The Switch() function also
supports this situation if you are using a number, a character, or a string. To
provide this last alternative, instead of a ConditionXToCheck
expressionk, enter True, and include the necessary statement. Here is an
example:
Module Exercise
Public Function Main() As Integer
Dim Status As UShort
Dim Result As String
Status = 12
Result = Microsoft.VisualBasic.Switch(
Status = 1, "Full Time",
Status = 2, "Part Time",
Status = 3, "Contractor",
Status = 4, "Seasonal",
True, "Unknown")
MsgBox("Employment Status: " & Result)
Return 0
End Function
End Module
This would produce:
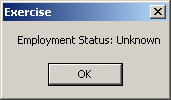
Remember that you can also use True with a character. Here
is an example:
Module Exercise
Public Function Main() As Integer
Dim Gender As Char
Dim Result As String
Gender = "H"
Result = Microsoft.VisualBasic.Switch(
Gender = "f", "Female",
Gender = "F", "Female",
Gender = "m", "Male",
Gender = "M", "Male",
True, "Unknown")
MsgBox("Gender: " & Result)
Return 0
End Function
End Module
This would produce:
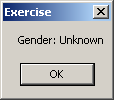
Practical
Learning: Using the Switch() Function
|
|
- To use the Switch() function, change the document as follows:
Module BethesdaCarRental
Private Function GetEmployeeName(ByVal EmplNbr As Long) As String
Dim Name As String
If EmplNbr = 22804 Then
Name = "Helene Mukoko"
ElseIf EmplNbr = 92746 Then
Name = "Raymond Kouma"
ElseIf EmplNbr = 54080 Then
Name = "Henry Larson"
ElseIf EmplNbr = 86285 Then
Name = "Gertrude Monay"
Else
Name = "Unknown"
End If
Return Name
End Function
Public Function Main() As Integer
Dim EmployeeNumber As Long, EmployeeName As String
Dim CustomerName As String
Dim TagNumber As String, CarSelected As String
Dim Tank As Integer, TankLevel As String
Dim RentStartDate As Date, RentEndDate As Date
Dim NumberOfDays As Integer
Dim RateType As String, RateApplied As Double
Dim OrderTotal As Double
Dim OrderInvoice As String
RateType = "Weekly Rate"
RateApplied = 0
OrderTotal = RateApplied
EmployeeNumber =
CLng(InputBox("Employee number (who processed this order):",
"Bethesda Car Rental", "00000"))
EmployeeName = GetEmployeeName(EmployeeNumber)
CustomerName = InputBox("Enter Customer Name:",
"Bethesda Car Rental", "John Doe")
TagNumber = InputBox("Enter the tag number of the car to rent:",
"Bethesda Car Rental", "000000")
CarSelected = Microsoft.VisualBasic.Switch(
TagNumber = "297419", "BMW 335i",
TagNumber = "485M270", "Chevrolet Avalanche",
TagNumber = "247597", "Honda Accord LX",
TagNumber = "924095", "Mazda Miata",
TagNumber = "772475", "Chevrolet Aveo",
TagNumber = "M931429", "Ford E150XL",
TagNumber = "240759", "Buick Lacrosse",
True, "Unidentified Car")
Tank = CInt(InputBox("Enter Tank Level:" & vbCrLf &
"1. Empty" & vbCrLf &
"2. 1/4 Empty" & vbCrLf &
"3. 1/2 Full" & vbCrLf &
"4. 3/4 Full" & vbCrLf &
"5. Full",
"Bethesda Car Rental", 1))
TankLevel = Choose(Tank, "Empty", "1/4 Empty",
"1/2 Full", "3/4 Full", "Full")
RentStartDate = CDate(InputBox("Enter Rent Start Date:",
"Bethesda Car Rental", #1/1/1900#))
RentEndDate = CDate(InputBox("Enter Rend End Date:", _
"Bethesda Car Rental", #1/1/1900#))
NumberOfDays = DateDiff(DateInterval.Day, RentStartDate, RentEndDate)
RateApplied = CDbl(InputBox("Enter Rate Applied:", _
"Bethesda Car Rental", 0))
OrderInvoice = "===========================" & vbCrLf &
"=//= BETHESDA CAR RENTAL =//=" & vbCrLf &
"==-=-= Order Processing =-=-==" & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Processed by:" & vbTab & EmployeeName & vbCrLf &
"Processed for:" & vbTab & CustomerName & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Car Selected:" & vbCrLf &
vbTab & "Tag #:" & vbTab & TagNumber & vbCrLf &
vbTab & "Car:" & vbTab & CarSelected & vbCrLf &
vbTab & "Tank:" & vbTab & TankLevel & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Start Date:" & vbTab & RentStartDate & vbCrLf &
"End Date:" & vbTab & RentEndDate & vbCrLf &
"Nbr of Days:" & vbTab & NumberOfDays & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Rate Type:" & vbTab & RateType & vbCrLf &
"Rate Applied:" & vbTab & RateApplied & vbCrLf &
"Order Total:" & vbTab & FormatCurrency(OrderTotal) & vbCrLf &
"==========================="
MsgBox(OrderInvoice,
MsgBoxStyle.Information Or MsgBoxStyle.OkOnly,
"Bethesda Car Rental")
Return 0
End Function
End Module
- Eexecute the application
- Enter the employee number as 86285, the customer name as Genevieve
H. Gomoore, the tag number as 924095, the tank level as 3, the
start date as 10/22/2008, the end date as 10/31/2008,
and the rate applied 55.50
- Close the message box and the DOS window to return to your programming
environment
|
|