The Select...Case Statement |
|
|
If you have a large number of conditions to examine, the
If...Then...Else statement will go through each one of them.
The Visual Basic language offers
the alternative of jumping to the statement that applies to the
state of the condition. This is done with the Select and Case
keywords.
|
The formula of the Select Case
statement is:
Select Case Expression
Case Expression1
Statement1
Case Expression2
Statement2
Case ExpressionX
StatementX
End Select
The statement starts with Select Case and ends with End
Select. On the right side of Select Case, enter a value, the Expression
factor, that will be used as a tag. The value of Expression can be
Boolean value (a Boolean type), a character (Char type), a string
(a String type), a natural number (a Byte, an SByte, a Short,
a UShort, an Integer, a UInteger, a Long, or a ULong
type), a decimal number (a Single, a Double, or a Decimal
type), a date or time value (a Date type), an enumeration (an Enum type),
or else (an Object type). Inside the Select Case and the End
Select lines, you provide one or more sections that each contains a Case
keyword followed by a value. The value on the right side of Case, ExpresionX,
must be the same type as the value of Expression or it can be implied
from it. After the case and its expression, you can write a statement.
When this section of code is accessed, the value of Expression
is considered. Then the value of Expression is compared to each ExpressionX of
each case:
- If the value of Expression1 is equal to that of Expression,
then Statement1 is executed. If the value of Expression1 is
not equal to that of Expression, then the compiler moves to Expression2
- If the value of Expression2 is equal to that of Expression,
then Statement2 is executed
- This will continue down to the last ExpressionX
Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Answer As Byte
Answer = CByte(InputBox( _
"One of the following is not a Visual Basic keyword" & vbCrLf &
"1) Function" & vbCrLf &
"2) Except" & vbCrLf &
"3) ByRef" & vbCrLf &
"4) Each" & vbCrLf & vbCrLf &
"Your Answer? "))
Select Case Answer
Case 1
MsgBox("Wrong: Function is a Visual Basic keyword." & vbCrLf &
"It is used to create a procedure of a function type")
Case 2
MsgBox("Correct: Except is not a keyword in " & vbCrLf &
"Visual Basic but __except is a C++ " & vbCrLf &
"keyword used in Exception Handling")
Case 3
MsgBox("Wrong: ByRef is a Visual Basic keyword used " & vbCrLf &
"to pass an argument by reference to a procedure")
Case 4
MsgBox("Wrong: The ""Each"" keyword is used in " & vbCrLf &
"Visual Basic in a type of looping " & vbCrLf &
"used to ""scan"" a list of item.")
End Select
Return 0
End Function
End Module
Here is an example of running the program:
The above code supposes that one of the cases will match the
value of the Expression factor. This is not always so. If you anticipate that there could be no match
between the Expression and one of the Expressions, you can use a
Case Else statement at the end of the list. The statement would then
look like this:
Select Case Expression
Case Expression1
Statement1
Case Expression2
Statement2
Case Expressionk
Statementk
Case Else
Statementk
End Select
In this case, the statement after the Case Else
will
execute if none of the previous expressions matches the Expression
factor. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Answer As Byte
Answer = CByte(InputBox( _
"One of the following is not a Visual Basic keyword" & vbCrLf &
"1) Function" & vbCrLf &
"2) Except" & vbCrLf &
"3) ByRef" & vbCrLf &
"4) Each" & vbCrLf & vbCrLf &
"Your Answer? "))
Select Case Answer
Case 1
MsgBox("Wrong: Function is a Visual Basic keyword." & vbCrLf &
"It is used to create a procedure of a function type")
Case 2
MsgBox("Correct: Except is not a keyword in " & vbCrLf &
"Visual Basic but __except is a C++ " & vbCrLf &
"keyword used in Exception Handling")
Case 3
MsgBox("Wrong: ByRef is a Visual Basic keyword used " & vbCrLf &
"to pass an argument by reference to a procedure")
Case 4
MsgBox("Wrong: The ""Each"" keyword is used in " & vbCrLf &
"Visual Basic in a type of looping " & vbCrLf &
"used to ""scan"" a list of item.")
Case Else
MsgBox("Invalid Selection")
End Select
Return 0
End Function
End Module
Here is an example of running the program:
Practical
Learning: Using a Select...Case Condition
|
|
- Start Microsoft Visual Basic and create a Console Application named BCR4
- In the Solution Explorer, right-click Module1.vb and click Rename
- Type BethesdaCarRental.vb and press Enter
- Accept to change the name of the module
- To use a Select...Case condition, change the document as follows:
Module BethesdaCarRental
Private Function GetEmployeeName(ByVal EmplNbr As Long) As String
Dim Name As String
If EmplNbr = 22804 Then
Name = "Helene Mukoko"
ElseIf EmplNbr = 92746 Then
Name = "Raymond Kouma"
ElseIf EmplNbr = 54080 Then
Name = "Henry Larson"
ElseIf EmplNbr = 86285 Then
Name = "Gertrude Monay"
Else
Name = "Unknown"
End If
Return Name
End Function
Public Function Main() As Integer
Dim EmployeeNumber As Long, EmployeeName As String
Dim CustomerName As String
Dim TagNumber As String, CarSelected As String
Dim CarStatus As Integer, CarCondition As String
Dim Tank As Integer, TankLevel As String
Dim RentStartDate As Date, RentEndDate As Date
Dim NumberOfDays As Integer
Dim RateType As String, RateApplied As Double
Dim OrderTotal As Double
Dim OrderInvoice As String
RateType = "Weekly Rate"
RateApplied = 0
OrderTotal = RateApplied
EmployeeNumber = CLng(InputBox("Employee number (who processed this order):",
"Bethesda Car Rental", "00000"))
EmployeeName = GetEmployeeName(EmployeeNumber)
CustomerName = InputBox("Enter Customer Name:",
"Bethesda Car Rental", "John Doe")
TagNumber = InputBox("Enter the tag number of the car to rent:",
"Bethesda Car Rental", "000000")
CarSelected = Microsoft.VisualBasic.Switch(
TagNumber = "297419", "BMW 335i",
TagNumber = "485M270", "Chevrolet Avalanche",
TagNumber = "247597", "Honda Accord LX",
TagNumber = "924095", "Mazda Miata",
TagNumber = "772475", "Chevrolet Aveo",
TagNumber = "M931429", "Ford E150XL",
TagNumber = "240759", "Buick Lacrosse",
True, "Unidentified Car")
CarStatus = CInt(InputBox("After inpecting it, enter car condition:" & vbCrLf &
"1. Excellent - No scratch, no damage, no concern" & vbCrLf &
"2. Good - Some concerns (scratches or missing something)." &
"Make sure the customer is aware." & vbCrLf &
"3. Drivable - The car is good enough to drive." &
"The customer must know the status of the car " &
"and agree to rent it",
"Bethesda Car Rental", 1))
Select Case CarStatus
Case 1
CarCondition = "Excellent"
Case 2
CarCondition = "Good"
Case 3
CarCondition = "Drivable"
Case Else
CarCondition = "Unknown"
End Select
Tank = CInt(InputBox("Enter Tank Level:" & vbCrLf &
"1. Empty" & vbCrLf &
"2. 1/4 Empty" & vbCrLf &
"3. 1/2 Full" & vbCrLf &
"4. 3/4 Full" & vbCrLf &
"5. Full",
"Bethesda Car Rental", 1))
TankLevel = Choose(Tank, "Empty", "1/4 Empty", "1/2 Full", "3/4 Full", "Full")
RentStartDate = CDate(InputBox("Enter Rent Start Date:",
"Bethesda Car Rental", #1/1/1900#))
RentEndDate = CDate(InputBox("Enter Rend End Date:",
"Bethesda Car Rental", #1/1/1900#))
NumberOfDays = DateDiff(DateInterval.Day, RentStartDate, RentEndDate)
RateApplied = CDbl(InputBox("Enter Rate Applied:",
"Bethesda Car Rental", 0))
OrderInvoice = "===========================" & vbCrLf &
"=//= BETHESDA CAR RENTAL =//=" & vbCrLf &
"==-=-= Order Processing =-=-==" & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Processed by:" & vbTab & EmployeeName & vbCrLf &
"Processed for:" & vbTab & CustomerName & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Car Selected:" & vbCrLf & _
vbTab & "Tag #:" & vbTab & TagNumber & vbCrLf &
vbTab & "Car:" & vbTab & CarSelected & vbCrLf &
vbTab & "Tank:" & vbTab & TankLevel & vbCrLf &
"Car Condition:" & vbTab & CarCondition & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Start Date:" & vbTab & RentStartDate & vbCrLf &
"End Date:" & vbTab & RentEndDate & vbCrLf &
"Nbr of Days:" & vbTab & NumberOfDays & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Rate Type:" & vbTab & RateType & vbCrLf &
"Rate Applied:" & vbTab & RateApplied & vbCrLf &
"Order Total:" & vbTab & FormatCurrency(OrderTotal) & vbCrLf &
"==========================="
MsgBox(OrderInvoice,
MsgBoxStyle.Information Or MsgBoxStyle.OkOnly,
"Bethesda Car Rental")
Return 0
End Function
End Module
- Execute the application
- Enter the employee number as 92746, the customer name as Antoinette
D. Liel, the tag number as 297419, the car condition as 1,
the tank level as 5, the start date as 11/15/2008, the
end date as 11/17/2008, and the rate applied 65.75
- Close the message box and the DOS window to return to your programming
environment
As mentioned in our introduction, the Select Case can use a
value other than an integer. For example you can use a character. Here is an
example:
Module Exercise
Public Function Main() As Integer
Dim Gender As Char
Gender = "M"
Select Case Gender
Case "F"
MsgBox("Female")
Case "M"
MsgBox("Male")
Case Else
MsgBox("Unknown")
End Select
Return 0
End Function
End Module
This would produce:
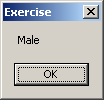
Notice that in this case we are using only upper case
characters. If want to validate lower case characters also, we may have to
create additional case sections for each. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Gender As Char
Gender = "f"
Select Case Gender
Case "f"
MsgBox("Female")
Case "F"
MsgBox("Female")
Case "m"
MsgBox("Male")
Case "M"
MsgBox("Male")
Case Else
MsgBox("Unknown")
End Select
Return 0
End Function
End Module
This would produce:
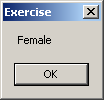
Instead of using one value for a case, you can
apply more than one. To do this, on the right side of the Case
keyword, you can separate the expressions with commas. Here are
examples:
Module Exercise
Public Function Main() As Integer
Dim Gender As Char
Gender = "F"
Select Case Gender
Case "f", "F"
MsgBox("Female")
Case "m", "M"
MsgBox("Male")
Case Else
MsgBox("Unknown")
End Select
Return 0
End Function
End Module
Validating a Range of Cases
|
|
You can use a
range of values for a case. To do this, on the right side of Case,
enter the lower value, followed by To, followed by the higher value.
Here is an example:
Imports System
Module Exercise
Public Function Main() As Integer
Dim Age As Integer
Age = 24
Select Case Age
Case 0 To 17
MsgBox("Teen")
Case 18 To 55
MsgBox("Adult")
Case Else
MsgBox("Senior")
End Select
End Sub
End Module
Checking Whether a Value IS
|
|
Consider the following program:
Module Exercise
Public Function Main() As Integer
Dim Number As Short
Number = 448
Select Case Number
Case -602
MsgBox("-602")
Case 24
MsgBox("24")
Case 0
MsgBox("0")
End Select
Return 0
End Function
End Module
Obviously this Select Case statement will work in rare cases
where the expression of a case exactly matches the value sought for. In
reality, for this type of scenario, you could validate a range of values. The
Visual Basic language provides an alternative. You can check whether the value
of the Expression responds to a criterion instead of an exact value. To create
it, you use the Is operator with the following formula:
Is Operator Value
You start with the Is keyword. It is followed by one
of the Boolean operators we saw in the previous lessons: =, <>, <, <=, >, or
>=. On the right side of the boolean operator, type the desired value. Here
are examples:
Module Exercise
Public Function Main() As Integer
Dim Number As Short
Number = -448
Select Case Number
Case Is < 0
MsgBox("The number is negative")
Case Is > 0
MsgBox("The number is positive")
Case Else
MsgBox("0")
End Select
Return 0
End Function
End Module
Although we used a natural number here, you can use any
appropriate logical comparison that can produce a True or a False result. You
can also combine it with the other alternatives we saw previously, such as
separating the expressions of a case with commas.
Practical
Learning: Using Options of Select...Case
|
|
- To use various options of the Select...Case condition, change the document as follows:
Module BethesdaCarRental
Private Function GetEmployeeName(ByVal EmplNbr As Long) As String
Dim Name As String
If EmplNbr = 22804 Then
Name = "Helene Mukoko"
ElseIf EmplNbr = 92746 Then
Name = "Raymond Kouma"
ElseIf EmplNbr = 54080 Then
Name = "Henry Larson"
ElseIf EmplNbr = 86285 Then
Name = "Gertrude Monay"
Else
Name = "Unknown"
End If
Return Name
End Function
Public Function Main() As Integer
Dim EmployeeNumber As Long, EmployeeName As String
Dim CustomerName As String
Dim TagNumber As String, CarSelected As String
Dim CarStatus As Integer, CarCondition As String
Dim Tank As Integer, TankLevel As String
Dim RentStartDate As Date, RentEndDate As Date
Dim NumberOfDays As Integer
Dim RateType As String, RateApplied As Double
Dim OrderTotal As Double
Dim OrderInvoice As String
RateType = "Weekly Rate"
RateApplied = 0
OrderTotal = RateApplied
EmployeeNumber = CLng(InputBox("Employee number (who processed this order):",
"Bethesda Car Rental", "00000"))
EmployeeName = GetEmployeeName(EmployeeNumber)
CustomerName = InputBox("Enter Customer Name:",
"Bethesda Car Rental", "John Doe")
TagNumber = InputBox("Enter the tag number of the car to rent:",
"Bethesda Car Rental", "000000")
CarSelected = Microsoft.VisualBasic.Switch(
TagNumber = "297419", "BMW 335i",
TagNumber = "485M270", "Chevrolet Avalanche",
TagNumber = "247597", "Honda Accord LX",
TagNumber = "924095", "Mazda Miata",
TagNumber = "772475", "Chevrolet Aveo",
TagNumber = "M931429", "Ford E150XL",
TagNumber = "240759", "Buick Lacrosse",
True, "Unidentified Car")
CarStatus = CInt(InputBox("After inpecting it, enter car condition:" & vbCrLf &
"1. Excellent - No scratch, no damage, no concern" & vbCrLf &
"2. Good - Some concerns (scratches or missing something)." &
"Make sure the customer is aware." & vbCrLf &
"3. Drivable - The car is good enough to drive." &
"The customer must know the status of the car " &
"and agree to rent it",
"Bethesda Car Rental", 1))
Select Case CarStatus
Case 1
CarCondition = "Excellent"
Case 2
CarCondition = "Good"
Case 3
CarCondition = "Drivable"
Case Else
CarCondition = "Unknown"
End Select
Tank = CInt(InputBox("Enter Tank Level:" & vbCrLf &
"1. Empty" & vbCrLf &
"2. 1/4 Empty" & vbCrLf &
"3. 1/2 Full" & vbCrLf &
"4. 3/4 Full" & vbCrLf &
"5. Full",
"Bethesda Car Rental", 1))
TankLevel = Choose(Tank, "Empty", "1/4 Empty", "1/2 Full", "3/4 Full", "Full")
RentStartDate = CDate(InputBox("Enter Rent Start Date:",
"Bethesda Car Rental", #1/1/1900#))
RentEndDate = CDate(InputBox("Enter Rend End Date:",
"Bethesda Car Rental", #1/1/1900#))
NumberOfDays = DateDiff(DateInterval.Day, RentStartDate, RentEndDate)
RateApplied = CDbl(InputBox("Enter Rate Applied:",
"Bethesda Car Rental", 0))
Dim TemporaryRate As Double
Select Case NumberOfDays
Case 0, 1
RateType = "Daily Rate"
OrderTotal = RateApplied
Case 2
RateType = "Weekend Rate"
TemporaryRate = RateApplied * 50 / 100
RateApplied = RateApplied - TemporaryRate
OrderTotal = RateApplied * 2
Case 2 To 7
RateType = "Weekly Rate"
TemporaryRate = RateApplied * 25 / 100
RateApplied = RateApplied - TemporaryRate
OrderTotal = RateApplied * NumberOfDays
Case Is > 8
RateType = "Monthly Rate"
TemporaryRate = RateApplied * 15 / 100
RateApplied = RateApplied - TemporaryRate
OrderTotal = RateApplied * NumberOfDays
End Select
OrderInvoice = "===========================" & vbCrLf &
"=//= BETHESDA CAR RENTAL =//=" & vbCrLf &
"==-=-= Order Processing =-=-==" & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Processed by:" & vbTab & EmployeeName & vbCrLf &
"Processed for:" & vbTab & CustomerName & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Car Selected:" & vbCrLf &
vbTab & "Tag #:" & vbTab & TagNumber & vbCrLf &
vbTab & "Car:" & vbTab & CarSelected & vbCrLf &
vbTab & "Tank:" & vbTab & TankLevel & vbCrLf &
"Car Condition:" & vbTab & CarCondition & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Start Date:" & vbTab & RentStartDate & vbCrLf &
"End Date:" & vbTab & RentEndDate & vbCrLf &
"Nbr of Days:" & vbTab & NumberOfDays & vbCrLf &
"------------------------------------------------" & vbCrLf &
"Rate Type:" & vbTab & RateType & vbCrLf &
"Rate Applied:" & vbTab & RateApplied & vbCrLf &
"Order Total:" & vbTab & FormatCurrency(OrderTotal) & vbCrLf &
"==========================="
MsgBox(OrderInvoice, _
MsgBoxStyle.Information Or MsgBoxStyle.OkOnly,
"Bethesda Car Rental")
Return 0
End Function
End Module
- Execute the application
- Enter the employee number as 86285, the customer name as Franco
Bolero, the tag number as M931429, the car condition as 3,
the tank level as 1, the start date as 10/06/2008, the
end date as 11/07/2008, and the rate applied 85.50
- Close the message box and the DOS window to return to your programming
environment
Select...Case and the Conditional Built-In Functions
|
|
With the Select...Case statement, we saw how to check
different values against a central one and take action when one of those matches
the tag. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Number As UShort, MembershipType As String
Number = 2
Select Case Number
Case 1
MembershipType = "Teen"
Case 2
MembershipType = "Adult"
Case Else
MembershipType = "Senior"
End Select
MsgBox("Membership Type: " & MembershipType)
Return 0
End Function
End Module
We also saw that the Visual Basic language provides the Choose()
function that can check a condition and take an action. The Choose() function is
another alternative to a Select...Case statement. Once again, consider
the syntax of the Choose function:
Public Function Choose( _
ByVal Index As Double, _
ByVal ParamArray Choice() As Object _
) As Object
This function takes two required arguments. The first
argument is equivalent to the Expression of our Select Case
formula. As mentioned already, the first argument must be a number (a Byte, an
SByte, a Short,
a UShort, an Integer, a UInteger, a Long, a ULong,
a Single, a Double, or a Decimal
value). This is the central value against which the other values will be
compared. Instead of using Case sections, provide the equivalent ExpressionX
values as a list of values in place of the second argument. The
values are separated by commas. Here is an example:
Choose(Number, "Teen", "Adult", "Senior")
As mentioned already, the values of the second argument are
provided as a list. Each member of the list uses an index. The first member of the list, which is the second argument of this
function, has an index of 1. The second value of the argument, which is the third
argument of the function, has an index of 2. You can continue adding the values
of the second argument as you see fit.
When the Choose() function has been called, it
returns a value of type Object. You can retrieve that value, store it in
a variable and use it as you see fit. Here is an example:
Module Exercise
Public Function Main() As Integer
Dim Number As UShort, MembershipType As String
Number = 1
MembershipType = Choose(Number, "Teen", "Adult", "Senior")
MsgBox("Membership Type: " & MembershipType)
Return 0
End Function
End Module